太依赖容器 这样的耦合程度较高
IoC type 2 setter injection
例子程序
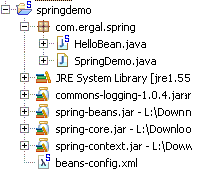
HelloBean.java
package
com.ergal.spring;

public
class
HelloBean

...
{
private String helloworld;
public void setHelloworld(String helloworld)

...{
this.helloworld=helloworld;
}
public String getHelloworld()

...{
return this.helloworld;
}
}
SpringDemo.java
package
com.ergal.spring;

import
org.springframework.core.io.FileSystemResource;
import
org.springframework.core.io.Resource;
import
org.springframework.beans.factory.BeanFactory;
import
org.springframework.beans.factory.xml.XmlBeanFactory;

public
class
SpringDemo

...
{
public static void main(String args[])

...{
Resource rs=new FileSystemResource("beans-config.xml");
BeanFactory factory=new XmlBeanFactory(rs);
HelloBean hello=(HelloBean)factory.getBean("hellobean");
System.out.println(hello.getHelloworld());
}
}
beans-config.xml
<?
xml version="1.0" encoding="UTF-8"
?>
<!
DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"
>

<
beans
>
<
bean
id
="hellobean"
class
="com.ergal.spring.HelloBean"
>
<
property
name
="helloworld"
>
<
value
>
Hello! Rainytooo!!
</
value
>
</
property
>
</
bean
>
</
beans
>
运行结果:
2006-9-27 5:18:34 org.springframework.core.CollectionFactory <clinit>
信息: JDK 1.4+ collections available
2006-9-27 5:18:35 org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions
信息: Loading XML bean definitions from file [D:/workspace/springdemo/beans-config.xml]
Hello! Rainytooo!!
IoC type 3 constructor injection
例子程序
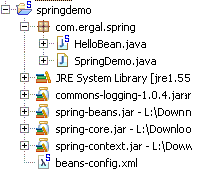
HelloBean.java
package
com.ergal.spring;

public
class
HelloBean

...
{
private String helloworld;
private String name;

public HelloBean()...{}
public HelloBean(String helloworld, String name)

...{
this.helloworld=helloworld;
this.name=name;
}
public void setHelloworld(String helloworld)

...{
this.helloworld=helloworld;
}
public String getHelloworld()

...{
return this.helloworld;
}
public void setName(String name)

...{
this.name=name;
}
public String getName()

...{
return this.name;
}
}
SpringDemo.java
package
com.ergal.spring;

import
org.springframework.context.ApplicationContext;
import
org.springframework.context.support.FileSystemXmlApplicationContext;



public
class
SpringDemo

...
{
public static void main(String args[])

...{
ApplicationContext context=new FileSystemXmlApplicationContext("beans-config.xml");
HelloBean hello=(HelloBean)context.getBean("hellobean");
System.out.println(hello.getHelloworld());
System.out.println(hello.getName());
}
}
beans-config.xml
<?
xml version="1.0" encoding="UTF-8"
?>
<!
DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"
>

<
beans
>
<
bean
id
="hellobean"
class
="com.ergal.spring.HelloBean"
>
<
constructor-arg
index
="0"
>
<
value
>
Hi guy !
</
value
>
</
constructor-arg
>
<
constructor-arg
index
="1"
>
<
value
>
Rainytooo
</
value
>
</
constructor-arg
>
</
bean
>
</
beans
>
BeanFactory
BeanFactory 负责读取Bean定义文件 管理对象的加载 生成并维护Bean对象之间的依赖关系 负责生命周期
ApplicationContext
有更简洁的获取资源方法 提供文字消息的解析方法 支持国际化 可以发布事件 对事件感兴趣的bean 可以接受到这些事情
常使用的三个类
org.springframework.context.support.FileSystemXmlApplicationContext
制定路径来读取定义文件
org.springframework.context.support.ClassPathXmlApplicationContext
从classpath中来读取XML定义文件
org.springframework.web.context.support.XmlWebApplicationContext
在web应用中 指定位置来读取定义文件
Bean的基本管理
属性参考
直接参考一个已经定义的bean
用<bean> 里的<ref bean=" " />
例子程序
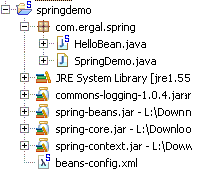
HelloBean.java
package
com.ergal.spring;

import
java.util.Date;

public
class
HelloBean

...
{
private String helloworld;
private String name;
private Date date;

public HelloBean()...{}
public HelloBean(String helloworld, String name)

...{
this.helloworld=helloworld;
this.name=name;
}
public void setHelloworld(String helloworld)

...{
this.helloworld=helloworld;
}
public String getHelloworld()

...{
return this.helloworld;
}
public void setName(String name)

...{
this.name=name;
}
public String getName()

...{
return this.name;
}
public Date getDate()

...{
return this.date;
}
public void setDate(Date date)

...{
this.date=date;
}
}
SpringDemo.java
package
com.ergal.spring;

import
org.springframework.context.ApplicationContext;
import
org.springframework.context.support.FileSystemXmlApplicationContext;



public
class
SpringDemo

...
{
public static void main(String args[])

...{
ApplicationContext context=new FileSystemXmlApplicationContext("beans-config.xml");
HelloBean hello=(HelloBean)context.getBean("hellobean");
System.out.println(hello.getHelloworld());
System.out.println(hello.getName());
System.out.println("Time is " + " " + hello.getDate());
}
}
beans-config.xml
<?
xml version="1.0" encoding="UTF-8"
?>
<!
DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"
>

<
beans
>
<
bean
id
="datebean"
class
="java.util.Date"
>
</
bean
>
<
bean
id
="hellobean"
class
="com.ergal.spring.HelloBean"
>
<
constructor-arg
index
="0"
>
<
value
>
Hi guy !
</
value
>
</
constructor-arg
>
<
constructor-arg
index
="1"
>
<
value
>
Rainytooo
</
value
>
</
constructor-arg
>
<
property
name
="date"
>
<
ref
bean
="datebean"
/>
</
property
>
</
bean
>
</
beans
>
自动绑定
<bean> autowire属性 有
byType 根据类型自动绑定
byName
autoselect 一切交给Spring来判断 spring是先constractor后byType
no
constractor
default
<bean> dependency-check属性 有
simple 只检查属性
object 只检查对象
all 都检查注入了没有
default
none
例子程序
同上
改beans-config.xml
<?
xml version="1.0" encoding="UTF-8"
?>
<!
DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"
>

<
beans
>
<
bean
id
="datebean"
class
="java.util.Date"
>
</
bean
>
<
bean
id
="hellobean"
class
="com.ergal.spring.HelloBean"
autowire
="byType"
>
<
constructor-arg
index
="0"
>
<
value
>
Hi guy !
</
value
>
</
constructor-arg
>
<
constructor-arg
index
="1"
>
<
value
>
Rainytooo
</
value
>
</
constructor-arg
>
</
bean
>
</
beans
>
集合对象的注入
对于List Set Map 等集合对象 在注入之前若必须填充一些对象到集合中 然后再将集合对象注入到bean里
例子程序
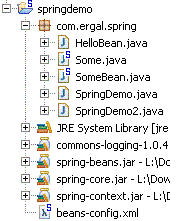
Some.java
package
com.ergal.spring;

public
class
Some

...
{
private String name;


public String getName() ...{
return name;
}


public void setName(String name) ...{
this.name = name;
}
public String toString()

...{
return name;
}
}
SomeBean.java
package
com.ergal.spring;

import
java.util.List;
import
java.util.Map;

public
class
SomeBean

...
{
private String[] someStrArray;
private Some[] someObjArray;
private List someList;
private Map someMap;

public List getSomeList() ...{
return someList;
}

public void setSomeList(List someList) ...{
this.someList = someList;
}

public Map getSomeMap() ...{
return someMap;
}

public void setSomeMap(Map someMap) ...{
this.someMap = someMap;
}

public Some[] getSomeObjArray() ...{
return someObjArray;
}

public void setSomeObjArray(Some[] someObjArray) ...{
this.someObjArray = someObjArray;
}

public String[] getSomeStrArray() ...{
return someStrArray;
}

public void setSomeStrArray(String[] someStrArray) ...{
this.someStrArray = someStrArray;
}
}
SpringDemo2.java
package
com.ergal.spring;

import
java.util.List;
import
java.util.Map;

import
org.springframework.context.ApplicationContext;
import
org.springframework.context.support.FileSystemXmlApplicationContext;

public
class
SpringDemo2

...
{
public static void main(String[] args)

...{
ApplicationContext context=new FileSystemXmlApplicationContext("beans-config.xml");
SomeBean somebean=(SomeBean)context.getBean("somebean");
String[] strs=(String[])somebean.getSomeStrArray();
Some[] somes=(Some[])somebean.getSomeObjArray();
for(int i=0; i <strs.length; i++)

...{
System.out.println(strs[i] + " " + somes[i].getName());
}
List someList=(List)somebean.getSomeList();
for(int i=0;i<someList.size();i++)

...{
System.out.println(someList.get(i));
}
Map someMap=(Map)somebean.getSomeMap();
System.out.println(someMap.get("MapTest"));
System.out.println(someMap.get("some1"));
}
}
beans-config.xml
<?
xml version="1.0" encoding="UTF-8"
?>
<!
DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"
>

<
beans
>
<
bean
id
="some1"
class
="com.ergal.spring.Some"
>
<
property
name
="name"
>
<
value
>
RainyTooo
</
value
>
</
property
>
</
bean
>
<
bean
id
="some2"
class
="com.ergal.spring.Some"
>
<
property
name
="name"
>
<
value
>
zhang
</
value
>
</
property
>
</
bean
>
<
bean
id
="somebean"
class
="com.ergal.spring.SomeBean"
>
<
property
name
="someStrArray"
>
<
list
>
<
value
>
Hello
</
value
>
<
value
>
welcome!
</
value
>
</
list
>
</
property
>
<
property
name
="someObjArray"
>
<
list
>
<
ref
bean
="some1"
/>
<
ref
bean
="some2"
/>
</
list
>
</
property
>
<
property
name
="someList"
>
<
list
>
<
value
>
ListTest
</
value
>
<
ref
bean
="some1"
/>
<
ref
bean
="some2"
/>
</
list
>
</
property
>
<
property
name
="someMap"
>
<
map
>
<
entry
key
="MapTest"
>
<
value
>
Hello hhahaha
</
value
>
</
entry
>
<
entry
key
="somekey1"
>
<
ref
bean
="some1"
/>
</
entry
>
</
map
>
</
property
>
</
bean
>
</
beans
>
结果
Hello RainyTooo
welcome! zhang
ListTest
RainyTooo
zhang
要注意的是map用 <entry key=""><value></value></entry>
List 可以用value 或者ref
另外
set
.......
<
set
>
<
value
></
value
>
<
ref bean
=
""
/>
<
ref bean
=
""
/>
</
set
>
java.util.properties
<
property name
=
""
>
<
props key
=
"
someProKey1
"
>
someProValue1
</
props
>
<
props key
=
"
someProKey2
"
>
someProValue2
</
props
>
</
property
>
BeanPostProcessor
org.springframework.beans.factory.config
Interface BeanPostProcessor
注入完成后 的初始化前后分别执行这两个方法
Method Summary |
---|
Object | postProcessAfterInitialization(Object bean, String beanName) Apply this BeanPostProcessor to the given new bean instance after any bean initialization callbacks (like InitializingBean's afterPropertiesSet or a custom init-method). |
Object | postProcessBeforeInitialization(Object bean, String beanName) Apply this BeanPostProcessor to the given new bean instance before any bean initialization callbacks (like InitializingBean's afterPropertiesSet or a custom init-method). |
例子:
大小写转换
BeanPostProcessorDemo.java
package
com.ergal.spring;

import
java.lang.reflect.Field;

import
org.springframework.beans.factory.config.BeanPostProcessor;

public
class
BeanPostProcessorDemo
implements
BeanPostProcessor

...
{
public Object postProcessAfterInitialization(Object bean, String beanName)

...{
Field[] fields=bean.getClass().getDeclaredFields();
for(int i=0; i<fields.length; i++)

...{
if(fields[i].getType().equals(String.class))

...{
fields[i].setAccessible(true);
try

...{
String original=(String)fields[i].get(bean);
fields[i].set(bean, original.toUpperCase());
}
catch(IllegalArgumentException e)

...{
e.printStackTrace();
}
catch(IllegalAccessException e)

...{
e.printStackTrace();
}
}
}
return bean;
}
public Object postProcessBeforeInitialization(Object bean, String beanName)

...{
return bean;
}
}
HelloBean.java
package
com.ergal.spring;

import
java.util.Date;

public
class
HelloBean

...
{
private String helloworld;
private String name;
private Date date;

public HelloBean()...{}
public HelloBean(String helloworld, String name)

...{
this.helloworld=helloworld;
this.name=name;
}
public void setHelloworld(String helloworld)

...{
this.helloworld=helloworld;
}
public String getHelloworld()

...{
return this.helloworld;
}
public void setName(String name)

...{
this.name=name;
}
public String getName()

...{
return this.name;
}
public Date getDate()

...{
return this.date;
}
public void setDate(Date date)

...{
this.date=date;
}
}
SpringDemo.java
package
com.ergal.spring;

import
org.springframework.context.ApplicationContext;
import
org.springframework.context.support.FileSystemXmlApplicationContext;



public
class
SpringDemo

...
{
public static void main(String args[])

...{
ApplicationContext context=new FileSystemXmlApplicationContext("beans-config.xml");
HelloBean hello=(HelloBean)context.getBean("hellobean");
System.out.println(hello.getHelloworld());
System.out.println(hello.getName());
System.out.println("Time is " + " " + hello.getDate());
}
}
beans-config.xml
<?
xml version="1.0" encoding="UTF-8"
?>
<!
DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"
>

<
beans
>
<
bean
id
="date"
class
="java.util.Date"
>
</
bean
>
<
bean
id
="hellobean"
class
="com.ergal.spring.HelloBean"
autowire
="byType"
>
<
constructor-arg
index
="0"
>
<
value
>
Hi guy !
</
value
>
</
constructor-arg
>
<
constructor-arg
index
="1"
>
<
value
>
Rainytooo
</
value
>
</
constructor-arg
>
</
bean
>
<
bean
id
="uppercaseModifier"
class
="com.ergal.spring.BeanPostProcessorDemo"
/>
</
beans
>
输出结果为
HI GUY !
RAINYTOOO
Time is Fri Sep 29 04:41:01 CST 2006
BeanFactoryPostProcessor
在BeanFactory加载Bean定义文件的所有内容,但还没有正式产生bean实力之前可以进行一些处理
1 PropertyPlacehholderConfiguer
籍由这个类可以将配置信息移出到一个或多个.properties文件中 可以用来客户化和分离功能设定
例子
将上面的xml改成
beans-config.xml
<?
xml version="1.0" encoding="UTF-8"
?>
<!
DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"
>

<
beans
>
<
bean
id
="date"
class
="java.util.Date"
>
</
bean
>
<
bean
id
="hellobean"
class
="com.ergal.spring.HelloBean"
autowire
="byType"
>
<
property
name
="helloworld"
>
<
value
>
${com.ergal.spring.HelloWorld}
</
value
>
</
property
>
<
property
name
="name"
>
<
value
>
Rainytooo
</
value
>
</
property
>
</
bean
>
<
bean
id
="configbBean"
class
="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer"
>
<
property
name
="location"
>
<
value
>
hello.properties
</
value
>
</
property
>
</
bean
>

</
beans
>
增加
hello.properties
com.ergal.spring.HelloWorld=Test config by properties
输出结果
Test config by properties
Rainytooo
Time is Fri Sep 29 05:16:47 CST 2006
2 CustomerEditComfigurer
将字符串值转换为指定类型的对象
例子
User.java
package
com.ergal.spring;

public
class
User

...
{
String name;
int number;

public String getName() ...{
return name;
}

public void setName(String name) ...{
this.name = name;
}

public int getNumber() ...{
return number;
}

public void setNumber(int number) ...{
this.number = number;
}
}
HellBean.java
package
com.ergal.spring;


public
class
HelloBean

...
{
private String helloworld;
private User user;

public String getHelloworld() ...{
return helloworld;
}

public void setHelloworld(String helloworld) ...{
this.helloworld = helloworld;
}

public User getUser() ...{
return user;
}

public void setUser(User user) ...{
this.user = user;
}
}
SpringDemo.java
package
com.ergal.spring;

import
org.springframework.context.ApplicationContext;
import
org.springframework.context.support.FileSystemXmlApplicationContext;

public
class
SpringDemo

...
{


/** *//**
* @param args
*/
public static void main(String[] args)

...{
// TODO Auto-generated method stub
ApplicationContext context=new FileSystemXmlApplicationContext("beans-config.xml");
HelloBean hello=(HelloBean)context.getBean("helloBean");
System.out.println(hello.getHelloworld() + " !");
System.out.println(hello.getUser().getName());
System.out.println(hello.getUser().getNumber());
}

}
beans-config.xml
<?
xml version="1.0" encoding="UTF-8"
?>
<!
DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"
>

<
beans
>
<
bean
id
="configBean"
class
="org.springframework.beans.factory.config.CustomEditorConfigurer"
>
<
property
name
="customEditors"
>
<
map
>
<
entry
key
="com.ergal.spring.User"
>
<
bean
id
="userEditor"
class
="com.ergal.spring.UserEditor"
></
bean
>
</
entry
>
</
map
>
</
property
>
</
bean
>
<
bean
id
="helloBean"
class
="com.ergal.spring.HelloBean"
>
<
property
name
="helloworld"
>
<
value
>
kkakaa
</
value
>
</
property
>
<
property
name
="user"
>
<
value
>
rainytooo,10889701
</
value
>
</
property
>
</
bean
>
</
beans
>
输出结果
kkakaa !
rainytooo
10889701
这个例子不能显示出威力和优点 只有在属性很多的时候 就能看出这种用来用指定方法来装还字符串为 指定对象的优点
资源的取得
Resource resource=context.getResource("classpath:admin.properties");
Resource resource=context.getResource("file:c:/asd/war/***/**");
Resource resource=context.getResource("WEB-INF/conf/admin.properties");
国际化
String getMessage(String code,
Object[] args,
Locale locale)
throws NoSuchMessageException
例子
beans-config.xml
<?
xml version="1.0" encoding="UTF-8"
?>
<!
DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"
>

<
beans
>
<
bean
id
="messageSource"
class
="org.springframework.context.support.ResourceBundleMessageSource"
>
<
property
name
="basename"
>
<
value
>
messages
</
value
>
</
property
>
</
bean
>
</
beans
>
SpringDemo2.java
package
com.ergal.spring;

import
java.util.Calendar;
import
java.util.Locale;

import
org.springframework.context.ApplicationContext;
import
org.springframework.context.support.FileSystemXmlApplicationContext;

public
class
SpringDemo2

...
{
public static void main(String[] args)

...{
ApplicationContext context=new FileSystemXmlApplicationContext("beans-config.xml");

Object[] arguments=new Object[]...{"Keith", Calendar.getInstance().getTime()};
System.out.println(context.getMessage("userLogin", arguments, Locale.US));
System.out.println(context.getMessage("userLogin", arguments, Locale.CHINA));
}

}
messages_en_US.properties
userLogin=User
{...}
{0}
login at
{...}
{1}
messages_zh_CN.properties
userLogin=使用者
{...}
{0}
于
{...}
{1}
登录
是用 native2ascii工具从txt转换而来
我在英文版win2003上直接用ANNI保存的txt
结果如下
User Keith login at 9/29/06 10:18 AM
使用者 Keith 于 06-9-29 上午10:18 登录