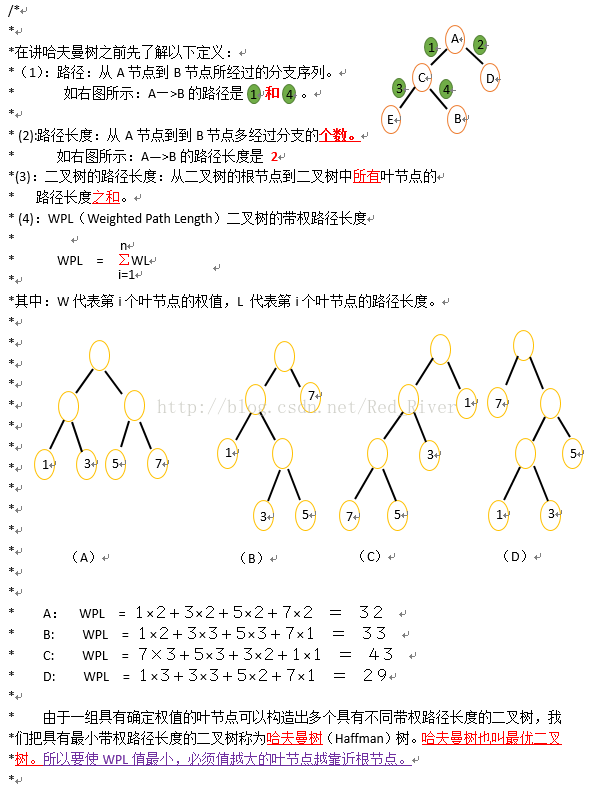
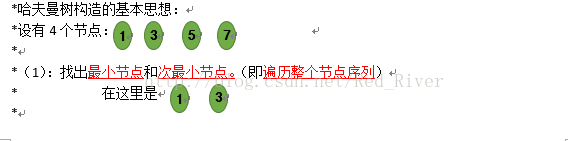
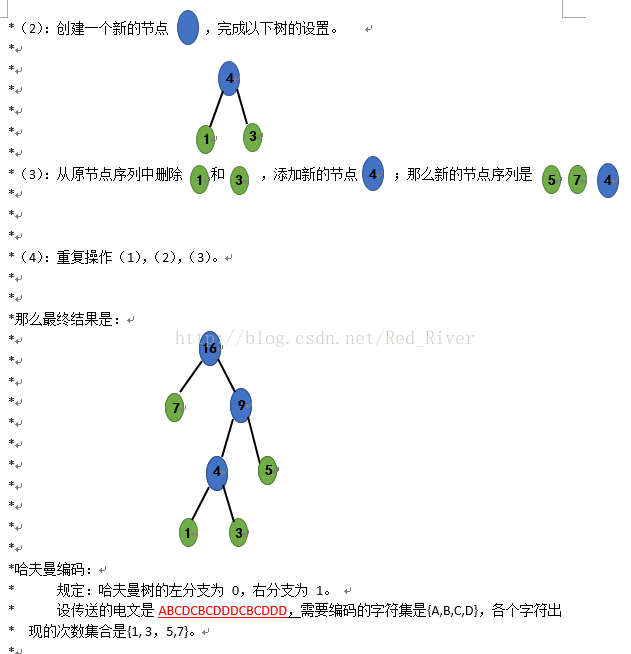
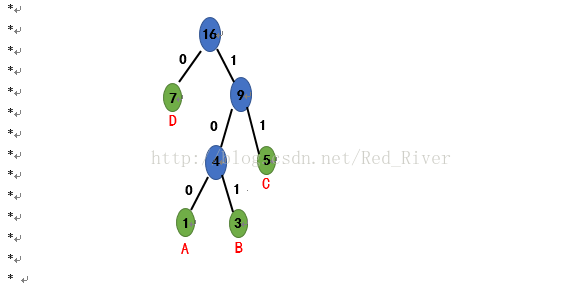
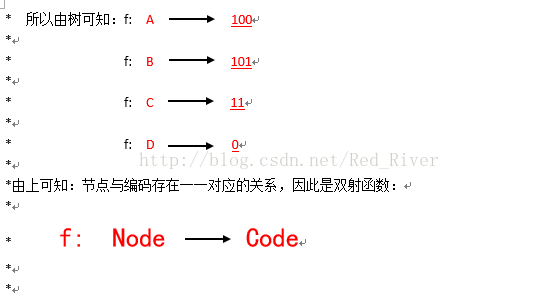
#ifndef _HAFFNODE_
#define _HAFFNODE_
class HaffNode
{
private:
int weight;//权值
int flag;//标记
int parent;
int leftChild;
int rightChild;
public:
HaffNode();
~HaffNode();
/*virtual*/ void SetWeight(int w);
/*virtual*/ int GetWeight()const;
/*virtual*/ void SetFlag(int f);
/*virtual*/ int GetFlag()const;
/*virtual*/ void SetParent(int p);
/*virtual*/ int GetParent()const;
/*virtual*/ void SetLeftChild(int l);
/*virtual*/ int GetLeftChild()const;
/*virtual*/ void SetRightChild(int r);
/*virtual*/ int GetRightChild()const;
};
#endif
#include "HaffNode.h"
HaffNode::HaffNode()
:parent(-1),leftChild(-1),rightChild(-1),
flag(0),weight(0)
{
}
HaffNode::~HaffNode()
{
}
void HaffNode::SetWeight(int w)
{
weight = w;
}
int HaffNode::GetWeight()const
{
return weight;
}
void HaffNode::SetFlag(int f)
{
flag = f;
}
int HaffNode::GetFlag()const
{
return flag;
}
void HaffNode::SetParent(int p)
{
parent = p;
}
int HaffNode::GetParent()const
{
return parent;
}
void HaffNode::SetLeftChild(int l)
{
leftChild = l;
}
int HaffNode::GetLeftChild()const
{
return leftChild;
}
void HaffNode::SetRightChild(int r)
{
rightChild = r;
}
int HaffNode::GetRightChild()const
{
return rightChild;
}
#ifndef _HAFFTREE_
#define _HAFFTREE_
#include "HaffNode.h"
#define MaxValue 100
class HaffTree/*:public HaffNode*/
{
public:
HaffNode* hafftree;
public:
HaffTree(int weight[], int lengthOfWeight);
~HaffTree();
};
#endif
#include "HaffTree.h"
HaffTree::HaffTree(int weight[], int lengthOfWeight)
{
hafftree = new HaffNode[2*lengthOfWeight-1];
int firMin;//最小值的下标
int secMin;//次最小的下标
int firMin_Weight;//最小值的权值
int secMin_Weight;//次最小值的权值
/
int i;
int j;
for(i = 0; i < lengthOfWeight; i++)
hafftree[i].SetWeight(weight[i]);
for(i = 0; i < lengthOfWeight-1; i++)
{
firMin_Weight = secMin_Weight = MaxValue;
firMin = secMin = 0;
for(j = 0; j < lengthOfWeight+i; j++)
{
if(hafftree[j].GetWeight() < firMin_Weight && !hafftree[j].GetFlag())
{
secMin_Weight = firMin_Weight;
secMin = firMin;
firMin_Weight = hafftree[j].GetWeight();
firMin = j;
}
else if(hafftree[j].GetWeight() < secMin_Weight && !hafftree[j].GetFlag())
{
secMin_Weight = hafftree[j].GetWeight();
secMin = j;
}
}
hafftree[firMin].SetParent(lengthOfWeight + i);
hafftree[secMin].SetParent(lengthOfWeight + i);
hafftree[firMin].SetFlag(1);
hafftree[secMin].SetFlag(1);
hafftree[lengthOfWeight + i].SetWeight(hafftree[firMin].GetWeight()+hafftree[secMin].GetWeight());
hafftree[lengthOfWeight+i].SetLeftChild(firMin);
hafftree[lengthOfWeight+i].SetRightChild(secMin);
}
}
HaffTree::~HaffTree()
{
delete []hafftree;
}
#ifndef _HAFFCODE_
#define _HAFFCODE_
#include <iostream>
#include "HaffTree.h"
class HaffCode
{
private:
int* bit;//编码数组,存储0/1
int weight;//节点的权值
int count;
int lengthOfWeight;
public:
HaffCode();
HaffCode(int lengthOfWeight);
void operator=(const HaffCode* code);
void operator=(const HaffCode& temp);
~HaffCode();
void SetWeight(int w);
int GetWeight()const;
void SetBit(int _x, bool value);
void HaffmanCode(const HaffTree& hafftree, int _x);
void Paint();
};
#endif
#include "HaffCode.h"
HaffCode::HaffCode():weight(0),count(0),lengthOfWeight(0)
{
}
HaffCode::HaffCode(int _lengthOfWeight)
:weight(0),count(0),lengthOfWeight(_lengthOfWeight)
{
bit = new int[_lengthOfWeight-1];
for(int i = 0; i < _lengthOfWeight-1; i++)
bit[i] = 0;
}
HaffCode::~HaffCode()
{
delete []bit;
}
void HaffCode::SetWeight(int w)
{
weight = w;
}
int HaffCode::GetWeight()const
{
return weight;
}
void HaffCode::SetBit(int _x, bool value)
{
bit[_x] = value;
}
void HaffCode::Paint()
{
for(int i = count-1; i >=0; i--)
std::cout<<bit[i];
std::cout<<std::endl;
}
void HaffCode::HaffmanCode(const HaffTree&hafftree, int _x)
{
int child = _x;
int parent = hafftree.hafftree[child].GetParent();
this->weight = hafftree.hafftree[child].GetWeight();
while(parent != -1)
{
if(hafftree.hafftree[parent].GetLeftChild() == child)
bit[count] = 0,count++;
else
bit[count] = 1,count++;
child = parent;
parent = hafftree.hafftree[child].GetParent();
}
}
void HaffCode::operator=(const HaffCode* code)
{
this->weight = code->weight;
this->count = code->count;
this->lengthOfWeight = code->lengthOfWeight;
bit = new int[this->lengthOfWeight-1];
for(int i = 0; i < this->lengthOfWeight-1; i++)
bit[i] = 0;
}
void HaffCode::operator=(const HaffCode &temp)
{
this->weight = temp.weight;
this->count = temp.count;
this->lengthOfWeight = temp.lengthOfWeight;
bit = new int[this->lengthOfWeight-1];
for(int i = 0; i < this->lengthOfWeight-1; i++)
bit[i] = 0;
}
#include "HaffCode.h"
#include "HaffTree.h"
#include <iomanip>
#define lengthOfWeight 10
int main(void)
{
int weight[lengthOfWeight] = {
1, 3, 5, 7, 9,
11,13,15,17,19};
HaffTree hafftree(weight, lengthOfWeight);
HaffCode* haffcode = new HaffCode[lengthOfWeight];
for(int i = 0; i < lengthOfWeight; i++)
{
//HaffCode* temp = new HaffCode(lengthOfWeight);
//haffcode[i] = temp;
//haffcode[i].HaffmanCode(hafftree, i);
//delete temp;
HaffCode temp(lengthOfWeight);
haffcode[i] = temp;
haffcode[i].HaffmanCode(hafftree, i);
}
std::cout.flags(std::ios::left);
for(int i = 0; i < lengthOfWeight; i++)
std::cout<<"Weight = "<<haffcode[i].GetWeight()<<" "<<"Code = ",haffcode[i].Paint();
return 0;
}