Notification能够使你的应用程序能够在不使用Activity的情况下通知用户。
虽然Notification与Toast都可以起到通知、提醒的作用。但它们的实现原理和表现形式却完全不一样。Toast其实相当于一个组件 (Widget)。有些类似于没有按钮的对话框。而Notification是显示在屏幕上方状态栏中的信息。还有就是Notification需要用 NotificationManager来管理,而Toast只需要简单地创建Toast对象即可。
“下载完毕”:
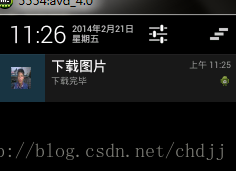
下面我总结了一些Notification的使用方法。
1.创建一个最简单的notification
创建notification只需要几个步骤:
(1)创建notification对象
Notification notification = new Notification(R.drawable.ic_launcher,"您有一条新通知", System.currentTimeMillis());
第一个参数为图标资源,第二个参数是通知到来时显示到顶部状态条上的内容,第三个是通知的时间。
这些参数也可以手动设置(如notification.icon等
)
(2)为notification配置一些参数
比如消息到来的声音,震动模式等,另外需要初始化一个PendingIntent用于指定点击通知所触发的事件,比如打开一个activity,启动service或者发送广播等。
notification.flags = Notification.FLAG_AUTO_CANCEL;//当用户点击通知时,自动删除通知
PendingIntent contentIntent = PendingIntent.getActivity(this, 0, new Intent(this,SecondActivity.class),PendingIntent.FLAG_UPDATE_CURRENT);
3)调用setLatestEventInfo方法设置通知的标题,内容,以及点击通知栏所触发的事件等。
notification.setLatestEventInfo(this,"通知标题", "点我跳转到一个activity", contentIntent);
第一个参数是上下文context,第二个参数是标题,第三个是内容,最后一个是PendingIntent对象.
(4)经过以上三步notification就已经被创建出来的,但是如果想让通知显示,还必须使用NotificationManager类:
NotificationManager manager = (NotificationManager)this.getSystemService(Context.NOTIFICATION_SERVICE);
manager.notify(1, notification);//第一个参数是通知的id
全部代码如下:
/**
* 显示一个通知,点击通知会跳转到一个Activity
* 注:跳转的这个activity必须先在清单文件中注册,否则点击将不会产生效果,而且不抛异常
*/
@SuppressWarnings("deprecation")
public void sendNotification()
{
NotificationManager manager = (NotificationManager) this.getSystemService(Context.NOTIFICATION_SERVICE);
Notification notification = new Notification(R.drawable.ic_launcher,"您有一条新通知", System.currentTimeMillis());
notification.flags = Notification.FLAG_AUTO_CANCEL;//当用户点击通知时,自动删除通知
// 相当于调用了startActivity方法
PendingIntent contentIntent = PendingIntent.getActivity(this, 0, new Intent(this,SecondActivity.class),PendingIntent.FLAG_UPDATE_CURRENT);
notification.setLatestEventInfo(this,"通知标题", "点我跳转到一个activity", contentIntent);
manager.notify(1, notification);
}
显示效果:
----------------------------------------------------------------------------------
2.android3.0发布之后,官方废弃掉了上面那种显示通知的方式,改用Notification.Builder类来创建通知了。但是考虑到兼容性,官方又发布了support.v4包,这里面有个叫
NotificationCompat.Builder的类,这个类用于创建通知,其实效果跟
Notification.Builder是一样的。
示例代码如下:
/**
* 普通的通知
*点击通知会跳转到另一个activity
*/
public void show_normal()
{
NotificationCompat.Builder mBuilder = new NotificationCompat.Builder(
this).setSmallIcon(R.drawable.ic_launcher)//设置图标
.setContentTitle("这是标题")//设置标题
.setContentText("这是内容");//设置内容
/*设置PendingIntent,当用户点击通知跳转到另一个界面,当退出该界面,直接回到HOME*/
Intent resultIntent = new Intent(this, ResultActivity.class);
TaskStackBuilder stackBuilder = TaskStackBuilder.create(this);
stackBuilder.addParentStack(ResultActivity.class);
stackBuilder.addNextIntent(resultIntent);
PendingIntent resultPendingIntent = stackBuilder.getPendingIntent(0,
PendingIntent.FLAG_UPDATE_CURRENT);
mBuilder.setContentIntent(resultPendingIntent);//设置PendingIntent
//创建NotificationManager 对象
NotificationManager mNotificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
Notification notification = mBuilder.build();//生成Notification对象
notification.flags = Notification.FLAG_AUTO_CANCEL;//点击后自动关闭通知
mNotificationManager.notify(1,notification);
}
显示效果:
另外还有一种特殊的通知,就是通知上有一个进度条(比如图片下载进度)。这个时候可以使用
builder.setProgress(max,progress,boolean inDeterminate);
第一个参数是进度条总长,第二个是当前进度,第三个参数表示当前的进度是否是最终的状态,如果为true,则进度条将不会走。
具体请看下面的示例代码:
/**
* 带进度条的通知
*/
public void show_progress()
{
final NotificationManager manager = (NotificationManager) this.getSystemService(NOTIFICATION_SERVICE);
final NotificationCompat.Builder builder = new NotificationCompat.Builder(this);
builder.setSmallIcon(R.drawable.ic_launcher);
builder.setLargeIcon(BitmapFactory.decodeResource(getResources(), R.drawable.me));
builder.setContentTitle("下载图片");
builder.setContentText("下载进度");
new Thread(new Runnable()
{
@Override
public void run()
{
for(int i = 0;i<=100;i++)
{
builder.setProgress(100,i, false);
manager.notify(0,builder.build());
try
{
Thread.sleep(50);
} catch (InterruptedException e)
{
e.printStackTrace();
}
}
// 注:setprogress设置为true后进度条进度将会停止,并且进度条不消失
// -- 进度条走完之后更新显示文本--
builder.setContentText("下载完毕").setProgress(0, 0, false);
Notification notification = builder.build();
// 下载完成后来一个声音提示
notification.defaults = Notification.DEFAULT_SOUND;
manager.notify(0, notification);
}
}).start();
}
显示效果:
“下载完毕”:
---------------------------------------------------------------------------------完------------------------------------------------
-----------------------------------------------------
详细信息参见:api guides中的Notifications
-----------------------------------------------------