13:用双链表结构实现双端队列
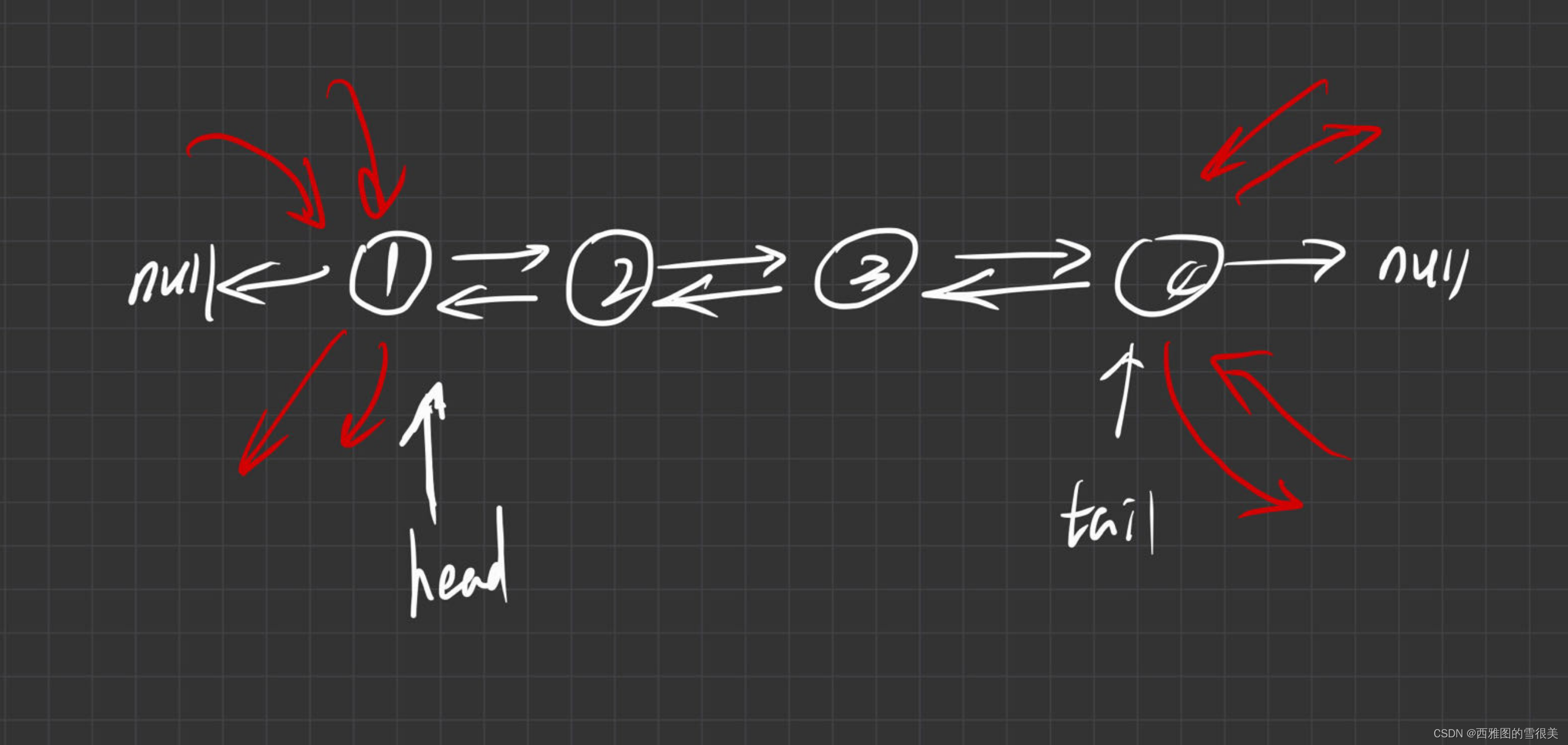
public class DoubleLinkedListToDeque<V> {
public static class Node<V> {
V value;
Node<V> next;
Node<V> last;
public Node(V value) {
this.value = value;
}
}
Node<V> head;
Node<V> tail;
int usedSize;
public DoubleLinkedListToDeque() {
head = null;
tail = null;
usedSize = 0;
}
public boolean isEmpty() {
return this.usedSize == 0;
}
public int size() {
return this.usedSize;
}
public void pushHead(V value) {
Node<V> cur = new Node<>(value);
if(head == null) {
tail = cur;
head = cur;
}else {
cur.next = head;
head.last = cur;
head = cur;
}
usedSize++;
}
public void pushTail(V value) {
Node<V> cur = new Node<>(value);
if(head == null) {
tail = cur;
head = cur;
}else {
tail.next = cur;
cur.last = tail;
tail = cur;
}
usedSize++;
}
public V popHead() {
if(this.head == null) {
return null;
}
V cur = this.head.value;
if(this.head == this.tail) {
this.head = null;
this.tail = null;
}else {
head = head.next;
head.last = null;
}
usedSize--;
return cur;
}
public V popTail() {
if(this.head == null) {
return null;
}
V cur = this.tail.value;
if(this.head == this.tail) {
this.head = null;
this.tail = null;
}else {
tail = tail.last;
tail.next = null;
}
usedSize--;
return cur;
}
public V peekHead() {
return this.isEmpty() ? null : this.head.value;
}
public V peekTail() {
return this.isEmpty() ? null : this.tail.value;
}
}