一、 基础数字操作
1.加减乘除以及内置函数: min(), max(), sum(), abs(), len() math库: math.pi math.e, math.sin math.sqrt math.pow()

# -*- coding:UTF-8 -*-
import math, random
a, b = 1, 100 # 整形 integer
result = []
for i in range(20):
number = random.randint( a, b )
# 生成不同的分数
if number not in result:
result.append( number )
minimum = min(result) # result找到最小和最大分数
maximum = max(result)
result.remove(minimum) # 移除最大最小值
result.remove(maximum)
length = len(result)
num = sum(result)
average = num / length # 浮点型, float 求20个数的均值
year = math.pow(2, 11) - (2**5) + 3
print( average, type(average) ) # float
print( length, average, average % year, num / b, num // b ) # /为真除法 5/2=2.5 ;//为截断除法,5//2=2类似于C语言的除法
print( random.choice( [1, 2, 3, 4, 5] ), average + random.random() )
2.数字的不同进制表示和转换。 十进制 八进制 十六进制 二进制 int(), oct(), hex(), bin(),eval()
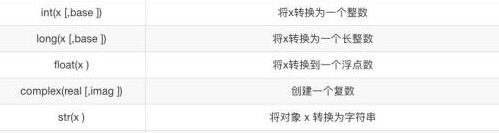
figs = [ 64, 0o11, 0x11, 0b11]
print(figs) # 输出十进制结果 [64, 9, 17, 3]
# eval -> input str transform into digit字符串转数字
print( 'eval: ', eval('64'), eval('0b111'), eval('0x140') )
# int -> str turn to num on base基于进制进行字符串数字转换
print( 'int: ', int('64'), int('100', 8), int('40', 16) )
格式化输出八、十六和二进制 '%o, %x, %b' % (64, 64, 64)
print( 'format printf 64-> ','{0:o}, {1:b}, {2:x}'.format(64, 64, 64) )
digit = int( input("input a digit:") )
print( "{} {} {} {} in different literal".format( digit, oct(digit), hex(digit), bin(digit) ) )
# b_len(x) == x.bit_length() 二进制位数
def b_len( num ):
return len( bin(num) ) - 2
print( 'bit length of num = %d' % ( b_len(figs[0])) )
3.小数decimal 和分数fraction
# temporary precision set
from decimal import Decimal
Deciaml.getcontext().prec = 4
precision dNum = Decimal('0.1') + Decimal('1.3')
# decimal 小数
import decimal
with decimal.localcontext() as ctx:
ctx.prec = 2 #小数精度
dnum = deciaml.Decimal('2.123') / deciaml.Decimal('7')
fraction 分数
from fraction import Fraction
a_frac = Fraction(1, 10) + Fraction(1, 10) - Fraction(2, 10)
a_frac = Fraction(1, 3) + Fraction( 5, 14)
print(a_frac)
def singleNumber(nums):
""" find single number type
nums: List:[int]
retype: int
"""
numSet = set(nums)
for num in numSet:
if nums.count(num) == 1:
return num
else:
print("We can't found it!")
print( singleNumber([4,1,2,1,2]) )
二、 Python 基础字符
1. 字符串 " " and ' ' and """ """ 转义字符 \n \t \' \\ 原始字符串 raw_str-> r"\now" "\\n" 2. s = "hello world" -> s.find('hello') s.rstrip()移除空格 s.replace("me","you") s.split(',')分隔 s.index("world") 3. s.isdigit() s.isspace() s.islower s.endswith('spam') s.upper() 4. for x in s: print(x)迭代 'spam' in s [c for c in s] map( ord ,s )映射
# 新建字符对象,headline变量指向此对象
headline = "knight" + " glory " + "sword"
print("Meaning " "of" "programming")
index = headline.find("sword") # rfind 从左到右
# 字符串常量不可修改,为不可变类型
title = "SpaM" * 4
print("lens:", len(title) )
title = title.lower()
title = title.replace("m", 'rk ')
print(title)
s1 = "your"
s2 = " name"
s = s1 + s2
print( s1 * 2, s1[1] ,s[:] , s[0:] ,s[::-1]) # 切片
# S.split(c) 使用符号c分割S字符串
line = "aaa bbb ccc 111"
cols = line.split(" ") # ['aaa', 'bbb', 'ccc', '111' ]
# rstrip() 移除尾部空白
line = "The dawn before the day!\n"
if line.startswith("The") and line.endswith("day!\n"):
print( line.rstrip() )
# S.join(iterable) -> str 还原字符串,S字符串作为分隔符插入到各迭代字符串间
strList = list(s)
print(strList) # ['y', 'o', 'u', 'r', ' ', 'n', 'a', 'm', 'e']
s = "".join(strList)
# ""作为分隔符,则连接单个字符
testStr = "spam".join(['egg', 'sausage', 'ham', 'sandwich'])
print(s, testStr)
字符串格式化方法
home = "this\' is our new home\n"
# 格式化字符->"%s meets %s" % (s1, s2) "{0} and {1}".format(s1, s2)
hotspot = "%s have no idea how %s i was." % ( "you", "worried" )
hitbox = "That is %d %s bird!" % ( 1, 'dead' )
# 基于字典键值格式化
response = "%(n)d %(x)s" % {"n":100, "x":"start"} #字符串格式符引用字典键值
print( home , hotspot, hitbox, response)
values = {'name':'David', "age":34}
reply = """
Greetings...
Hello %(name)s!
Your age roughly is %(age)s years old
"""
print( reply % values )
格式化方法调用 format
template = "{} {} and {}".format('green', 'red', 'purple' )
textline = "{name} wants to eat {food} \n".format( name="Bob", food="meat")
pi = 3.14159
# 类似C语言printf 格式输出 [06.2f]前补0 ,6字符宽度.2f浮点数输出小数点后2位
line = "\n{0:f} {1:.2f} {2:06.2f}\n".format( pi, pi , pi)
print( template, textline, line,'{0:X}, {1:o}, {2:b}'.format(255,255,255) )
test = random.choice( ['apple', 'pear', 'banana', 'orange'] )
test += str(123) # str(object)--> string
for animal in ["dog", "cat", "piglet"]:
print( "%s is a mammal" % animal )
字符转换chr、ord, int
alpha = []
c = 'a' # chr() ord()->trans char into int [ASCII]
for i in range(26):
alpha.append( chr( ord(c)+i ) )
print(alpha)
binDigit = '11010011' # equal to int( B, 2 )
B = binDigit[:]
I = 0
while B != '':
I = I * 2 + ( ord(B[0]) - ord('0') )
B = B[1:]
print( "\'%s\' -> %d" % ( binDigit, I ) )
| 参考 《python学习手册》第4版