综合实战:多对多数据映射(管理员-角色-组-权限)
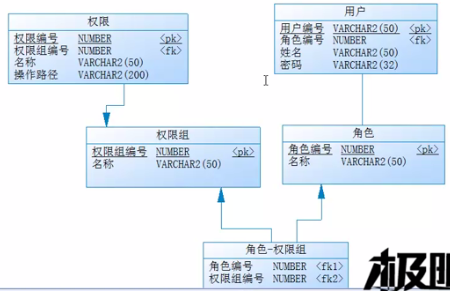
开发要求:

第一步:数据表转换为简单Java类
class User{
private String userid;
private String name;
private String password;
public User(String userid,String name,String password){
this.userid=userid;
this.name=name;
this.password=password;
}
public String getInfo(){
return "用户编号:"+this.userid+",姓名:"+this.name+",密码:"+this.password;
}
}
class Role{//角色
private int rid;
private String title;
public Role(int rid,String title){
this.rid=rid;
this.title=title;
}
public String getInfo(){
return "角色编号:"+this.rid+",角色名称:"+this.title;
}
}
class Group{//权限组
private int gid;
private String title;
public Group(int gid,String title){
this.gid=gid;
this.title=title;
}
public String getInfo(){
return "组编号:"+this.gid+",组名称:"+this.title;
}
}
class Action{//权限
private int aid;
private String title;
private String url;
public Action(int aid,String title,String url){
this.aid=aid;
this.title=title;
this.url=url;
}
public String getInfo(){
return "权限编号:"+this.aid+",权限名称:"+this.title+",路径:"+this.url;
}
}
class User{
private String userid;
private String name;
private String password;
private Role role;
public User(String userid,String name,String password){
this.userid=userid;
this.name=name;
this.password=password;
}
public void setRole(Role role){
this.role=role;
}
public Role getRole(){
return this.role;
}
public String getInfo(){
return "用户编号:"+this.userid+",姓名:"+this.name+",密码:"+this.password;
}
}
class Role{//角色
private int rid;
private String title;
public User users [];
private Group groups[];
public Role(int rid,String title){
this.rid=rid;
this.title=title;
}
public void setUser(User users[]){
this.users=users;
}
public User[] getUsers(){
return this.users;
}
public void setGroups(Group [] groups){
this.groups=groups;
}
public Group [] getGroups(){
return this.groups;
}
public String getInfo(){
return "角色编号:"+this.rid+",角色名称:"+this.title;
}
}
class Group{//权限组
private int gid;
private String title;
private Action actions[];
private Role roles[];
public Group(int gid,String title){
this.gid=gid;
this.title=title;
}
public void setActions(Action actions[]){
this.actions=actions;
}
public Action [] getActions(){
return this.actions;
}
public void setRoles(Role roles[]){
this.roles=roles;
}
public Role[] getRoles(){
return this.roles;
}
public String getInfo(){
return "组编号:"+this.gid+",组名称:"+this.title;
}
}
class Action{//权限
private int aid;
private String title;
private String url;
private Group group;
public Action(int aid,String title,String url){
this.aid=aid;
this.title=title;
this.url=url;
}
public void setGroup(Group group){
this.group=group;
}
public Group getGroup(){
return this.group;
}
public String getInfo(){
return "权限编号:"+this.aid+",权限名称:"+this.title+",路径:"+this.url;
}
}
第三步:设置关系
public class Hello {
public static void main(String args[]){
//根据表结构设置关系
//1.定义单独的类对象
User ua= new User("usera","用户A","hello");
User ub= new User("userb","用户B","hello");
User uc= new User("userc","用户C","hello");
//定义权限
Action act1=new Action(1,"新闻管理","http://www.mdln.cn/news");
Action act2=new Action(2,"用户管理","http://www.mdln.cn/users");
Action act3=new Action(3,"备份管理","http://www.mdln.cn/bks");
Action act4=new Action(4,"缓存管理","http://www.mdln.cn/caches");
Action act5=new Action(5,"数据管理","http://www.mdln.cn/datas");
//3.定义权限组信息
Group g1 =new Group(1,"数据管理");
Group g2 =new Group(2,"人事管理");
Group g3 =new Group(3,"信息管理");
//4.定义角色信息
Role r1=new Role(10,"超级管理员");
Role r2=new Role(10,"普通管理员");
//5.设置权限组与权限的关系,一对多关系
act1.setGroup(g1);
act2.setGroup(g1);
act3.setGroup(g2);
act4.setGroup(g2);
act5.setGroup(g3);
g1.setActions(new Action[]{act1,act2});
g2.setActions(new Action[]{act3,act4});
g3.setActions(new Action[]{act5});
//6.权限组与角色的关系
r1.setGroups(new Group[]{g1,g2,g3});
r2.setGroups(new Group[]{g2,g3});
g1.setRoles(new Role[]{r1});
g2.setRoles(new Role[]{r1,r2});
g3.setRoles(new Role[]{r1,r2});
//7.定义用户与角色的关系
ua.setRole(r1);
ub.setRole(r2);
uc.setRole(r2);
r1.setUser(new User[]{ua});
r2.setUser(new User[]{ub,uc});
//第二步:根据要求通过关系取出数据
//2.1
System.out.println(ua.getInfo());
System.out.println("\t|-【角色】"+ua.getRole().getInfo());
for(int x=0;x<ua.getRole().getGroups().length;x++){
System.out.println("\t\t|-【权限组】"+ua.getRole().getGroups()[x].getInfo());
for(int y=0;y<ua.getRole().getGroups()[x].getActions().length;y++){
System.out.println("\t\t\t|-【权限】"+ua.getRole().getGroups()[x].getActions()[y].getInfo());
}
}
System.out.println("==============================================================================================");
//2.2
System.out.println(act1.getInfo());
//通过权限找到权限对应的权限组,一个权限组有多个角色
for(int x=0;x<act1.getGroup().getRoles().length;x++){
System.out.println("\t\t|-【角色】"+act1.getGroup().getRoles()[x].getInfo());
for(int y=0;y<act1.getGroup().getRoles()[x].getUsers().length;y++){
System.out.println("\t\t\t|-【用户】"+act1.getGroup().getRoles()[x].getUsers()[y].getInfo());
}
}
System.out.println("==============================================================================================");
//2.3
System.out.println(r1.getInfo());
for(int x=0;x<r1.getUsers().length;x++){
System.out.println("\t|-【用户】"+r1.getUsers()[x].getInfo());
for(int y=0;y<r1.getGroups().length;y++){
System.out.println("\t\t|-【权限组】"+r1.getGroups()[y].getInfo());
for(int z=0;z<r1.getGroups()[y].getActions().length;z++){
System.out.println("\t\t\t|-【权限】"+r1.getGroups()[y].getActions()[z].getInfo());
}
}
}
}
}
引用是Java基础的核心!!!