java 使用jacob实现word、excle、ppt、图片转pdf
IDEA Maven 微服务项目示例
示例:
步骤:
1.jarcob jar包、插件下载
SaveAsPDFandXPS 下载地址:
http://www.microsoft.com/zh-cn/download/details.aspx?id=7
(若访问不了,百度搜索SaveAsPDFandXPS)
jacob 包下载地址:
http://sourceforge.net/projects/jacob-project/
2.jacob jar包和dll存放路径
下载 jacob 解压后存放路径:
jacob.jar 放在 C:\Program Files\Java\jdk1.8.0_171\jre\lib\ext目录下
jacob.dll 放在 C:\Program Files\Java\jdk1.8.0_171\jre\bin 目录下
3. 项目里src/main/resources/lib 下放jar包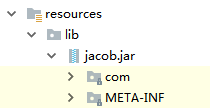
五、pom文件中添加依赖
<dependency>
<groupId>com.jacob</groupId>
<artifactId>jacob</artifactId>
<version>1.20</version>
<scope>system</scope>
<systemPath>${basedir}/src/main/resources/lib/jacob.jar</systemPath>
</dependency>
六、代码实现
import java.io.File;
import com.jacob.activeX.ActiveXComponent;
import com.jacob.com.ComFailException;
import com.jacob.com.ComThread;
import com.jacob.com.Dispatch;
import com.jacob.com.Variant;
public class ToPDF {
private static final int wdFormatPDF = 17; // PDF 格式
private static final int xlTypePDF = 0; // xls格式
public boolean toPDF(String sfileName, String toFileName) {
System.out.println("------开始转换------");
String suffix = getFileSufix(sfileName);
File file = new File(sfileName);
if (!file.exists()) {
System.out.println("文件不存在!");
return false;
}
if (suffix.equals("pdf")) {
System.out.println("PDF not need to convert!");
return false;
}
if (suffix.equals("doc") || suffix.equals("docx") || suffix.equals("txt")) {
return word2PDF(sfileName, toFileName);
} else if (suffix.equals("ppt") || suffix.equals("pptx")) {
return ppt2PDF(sfileName, toFileName);
} else if (suffix.equals("xls") || suffix.equals("xlsx")) {
return excel2PDF(sfileName, toFileName);
} else {
System.out.println("文件格式不支持转换!");
return false;
}
}
//截取文件后缀方法
public static String getFileSufix(String fileName) {
int splitIndex = fileName.lastIndexOf(".");
return fileName.substring(splitIndex + 1);
}
//转换word文档
public boolean word2PDF(String sfileName, String toFileName) {
long start = System.currentTimeMillis();
ActiveXComponent app = null;
Dispatch doc = null;
boolean result = true;
try {
app = new ActiveXComponent("Word.Application");
app.setProperty("Visible", new Variant(false));
Dispatch docs = app.getProperty("Documents").toDispatch();
doc = Dispatch.call(docs, "Open", sfileName).toDispatch();
System.out.println("打开文档..." + sfileName);
System.out.println("转换文档到 PDF..." + toFileName);
File tofile = new File(toFileName);
if (tofile.exists()) {
tofile.delete();
}
Dispatch.call(doc, "SaveAs", toFileName, wdFormatPDF);
long end = System.currentTimeMillis();
System.out.println("转换完成..用时:" + (end - start) + "ms.");
result = true;
} catch (Exception e) {
// TODO: handle exception
System.out.println("========Error:文档转换失败:" + e.getMessage());
result = false;
} finally {
Dispatch.call(doc, "Close", false);
System.out.println("关闭文档");
if (app != null) {
app.invoke("Quit", new Variant[] {});
}
}
ComThread.Release();
return result;
}
//转换excel文档
public boolean excel2PDF(String inputFile, String pdfFile) {
ActiveXComponent app = null;
Dispatch excel = null;
boolean result = true;
try {
app = new ActiveXComponent("Excel.Application");
app.setProperty("Visible", false);
Dispatch excels = app.getProperty("Workbooks").toDispatch();
excel = Dispatch.call(excels, "Open", inputFile, false, true).toDispatch();
Dispatch.call(excel, "ExportAsFixedFormat", xlTypePDF, pdfFile);
System.out.println("打开文档..." + inputFile);
System.out.println("转换文档到 PDF..." + pdfFile);
result = true;
} catch (Exception e) {
result = false;
} finally {
if (excel != null) {
Dispatch.call(excel, "Close");
}
if (app != null) {
app.invoke("Quit");
}
}
return result;
}
//转换ppt文档
public boolean ppt2PDF(String srcFilePath, String pdfFilePath) {
ActiveXComponent app = null;
Dispatch ppt = null;
boolean result = true;
try {
ComThread.InitSTA();
app = new ActiveXComponent("PowerPoint.Application");
Dispatch ppts = app.getProperty("Presentations").toDispatch();
// 因POWER.EXE的发布规则为同步,所以设置为同步发布
ppt = Dispatch.call(ppts, "Open", srcFilePath, true, // ReadOnly
true, // Untitled指定文件是否有标题
false// WithWindow指定文件是否可见
).toDispatch();
Dispatch.call(ppt, "SaveAs", pdfFilePath, 32); // ppSaveAsPDF为特定值32
System.out.println("转换文档到 PDF..." + pdfFilePath);
result = true; // set flag true;
} catch (ComFailException e) {
result = false;
} catch (Exception e) {
result = false;
} finally {
if (ppt != null) {
Dispatch.call(ppt, "Close");
}
if (app != null) {
app.invoke("Quit");
}
ComThread.Release();
}
return result;
}
public static void main(String[] args) {
ToPDF d = new ToPDF();
d.toPDF("E:\\poi-test\\一个.excle.xlsx", "E:\\poi-test\\一个.excle.pdf");
}
}
七、结果:(例)
附:图片转pdf(要用的话,上边的toPDF方法加上图片的后缀判断)
else if (suffix.equals("jpg") || suffix.equals("jpeg")||suffix.equals("png") || suffix.equals("gif") || suffix.equals("bmp")){}
public String imgToPdf(String filename)throws IOException {
String[] strings=filename.split("\\.");
String str= strings[strings.length-1];
String toFileName=filename.replace(str,"pdf");//新的文件名pdf格式
File file=new File(filename);
if(file.exists()){
Document document = new Document();
FileOutputStream fos = null;
try {
fos = new FileOutputStream(toFileName);
PdfWriter.getInstance(document, fos);
// 添加PDF文档的某些信息,比如作者,主题等等
// document.addAuthor("arui");
//document.addSubject("test pdf.");
// 设置文档的大小
document.setPageSize(PageSize.A4);
// 打开文档
document.open();
// 写入一段文字
//document.add(new Paragraph("JUST TEST ..."));
// 读取一个图片
Image image = Image.getInstance(filename);
float imageHeight=image.getScaledHeight();
float imageWidth=image.getScaledWidth();
int i=0;
while(imageHeight>500||imageWidth>500){
image.scalePercent(100-i);
i++;
imageHeight=image.getScaledHeight();
imageWidth=image.getScaledWidth();
System.out.println("imageHeight->"+imageHeight);
System.out.println("imageWidth->"+imageWidth);
}
image.setAlignment(Image.ALIGN_CENTER);
// //设置图片的绝对位置
// image.setAbsolutePosition(0, 0);
// image.scaleAbsolute(500, 400);
// 插入一个图片
document.add(image);
} catch (DocumentException de) {
System.out.println(de.getMessage());
} catch (IOException ioe) {
System.out.println(ioe.getMessage());
}
//向FiletopdfImagetopdf表添加数据
FiletopdfImagetopdf filetopdfImagetopdf=new FiletopdfImagetopdf();
filetopdfImagetopdf.setFilename(filename);
filetopdfImagetopdf.setTofilename(toFileName);
document.close();
fos.flush();
fos.close();
return toFileName;
}else{
return "图片不存在!";
}
}
总结
这里对文章进行总结:
以上就是今天要讲的内容,本文介绍了怎样使用jacob实现word、excle、ppt、图片转换为pdf的过程。其中核心代码实现可根据读者自己的使用情况稍作拆分或调整。