在 pom.xml 文件中添加依赖:
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency>
由于使用 HikariCP 连接池,添加下面依赖:
<dependency> <groupId>com.zaxxer</groupId> <artifactId>HikariCP</artifactId> </dependency>
在 application.yml 文件中添加配置:
spring: datasource: driver-class-name: com.mysql.jdbc.Driver url: jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&useSSL=false username: root password: type: com.zaxxer.hikari.HikariDataSource jpa: database: mysql database-platform: MYSQL show-sql: true format-sql: true hibernate: ddl-auto: update naming: strategy: org.springframework.boot.orm.jpa.hibernate.SpringNamingStrategy
编写配置类:
import com.mj.wcs.domain.User; import com.mj.wcs.repository.UserRepository; import org.springframework.boot.autoconfigure.domain.EntityScan; import org.springframework.context.annotation.Configuration; import org.springframework.data.jpa.repository.config.EnableJpaRepositories; import org.springframework.transaction.annotation.EnableTransactionManagement; /** * @author smileorsilence * @date 2018/03/28 */ @Configuration @EntityScan(basePackageClasses = User.class) @EnableJpaRepositories(basePackageClasses = UserRepository.class) @EnableTransactionManagement public class HibernateJpaConfiguration { }
编写实体类:
impor
Spring Boot 学习笔记 5 : JPA
最新推荐文章于 2024-07-20 20:09:56 发布
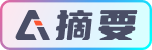