ES6 Class
和 ES5 的对比和基本说明
function Point(x, y) {
this.x = x;
this.y = y;
}
Point.prototype.toString = function () {
return '(' + this.x + ', ' + this.y + ')';
};
const p = new Point(1, 2);
// 使用 class 之后
class Point {
constructor(x, y) {
this.x = x;
this.y = y;
}
toString() {
return '(' + this.x + ', ' + this.y + ')';
}
}
const p = new Point(1, 2);
上面的代码中定义了一个“类” 类名是 Point
。constructor
是它的方法体。所以 p.constructor === Point.prototype.constructor
Class 基本语法
class Point {
toString() {
...
}
toValue() {
...
}
toKeys() {
...
}
// ES7 中的写法 ES6 不支持
name = "ccc"
}
var const = new Point(1, 2);
// 等同于
Point.prototype = {
toString(){},
toValue(){},
toKeys(){}
}
上面的 class 里面定义了一个 toString()
方法,在 Point
里面写的方法会直接写在原型上。在 ES6 在 class 里面直接赋值会报错,在 ES7 中不会。
静态方法
在前面加上 static 关键字,该方法不会被实例继承,只能使用。这种就叫静态方法
class Fun {
// 在前面加上 static 关键字,该方法不会被实例继承,只能使用
static name (){
return 'MyFun';
}
}
Fun.name(); // 'MyFun'
let fun = new Fun();
fun.name();
// TypeError: fun.name is not a function
上面的代码中,name
方法前有 static
关键字,表示 name
是一个静态方法,可以 Fun.name()
调用,而不是在 fun
调用。
继承和 super
class Fun {
static name (){
return 'MyFun';
}
}
class Box extends Fun {
}
Box.name() // 'MyFun'
上面代码中,Fun
有一个静态方法,Box
继承 Fun
之后可以调用这个方法。
可以使用 super
,调用父类的静态方法。
class Fun {
static name (){
return 'MyFun';
}
}
class Box extends Fun {
static name () {
console.log(super.name() + ', ccc')
}
}
Box.name() // 'MyFun,ccc'
ES7 Class 的新特性
- static prop = xxx; 静态属性
- property = xxx; 私有属性
- @decortor 装饰器
静态属性和私有属性
我在上面文章说了,在 ES7
中可以直接 static prop = xxx
写静态属性,也可以 myName = 'ccc'
写私有属性
class Fun {
static name = 'ccc'; // 静态属性
constructor(){
this.keyValue = ''
}
myName = 'ccc'; // 私有属性
getCount(){
console.log('发送请求')
}
}
var fun = new Fun()
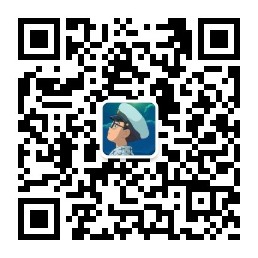