1:冒泡排序
<script>
function bubbleSort(arr) {
const len = arr.length;
for (let i = 0; i < len - 1; i++) {
for (let j = 0; j < len - 1 - i; j++) {
if (arr[j] > arr[j + 1]) {
const t = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = t;
}
}
}
return arr;
}
const arr = [66, 99, 25, 10, 22, 55, 11];
console.log("排序前:", arr);
console.log("排序后:", bubbleSort(arr));
</script>
最后效果:
2:选择排序
<script>
function selectSort(arr) {
const len = arr.length;
for (let i = 0; i < len - 1; i++) {
let min = i;
for (let j = i + 1; j < len; j++) {
if (arr[j] < arr[min]) {
min = j;
}
}
if (min !== i) {
const t = arr[i];
arr[i] = arr[min];
arr[min] = t;
}
}
return arr;
}
const arr = [64, 99, 25, 12, 78, 11, 90];
console.log("排序前:", arr);
console.log("排序后:", selectSort(arr));
</script>
效果:
3:插入排序法
<script>
function insertSort(arr) {
const len = arr.length;
for (let i = 1; i < len; i++) {
let current = arr[i];
let j = i - 1;
while (j >= 0 && arr[j] > current) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = current;
}
return arr;
}
const arr = [64, 78, 90, 55, 15, 11, 27, 117];
console.log("排序前:", arr);
console.log("排序后:", insertSort(arr));
</script>
效果:
4:驼峰法变换
<script>
function toCamelCase(str) {
let words = ['get', 'element', 'by', 'id'];
return words.map((word, index) => {
if (index === 0) {
return word.toLowerCase();
} else {
return word[0].toUpperCase() + word.slice(1);
}
}).join('');
}
const originalStr = "getelementbyid";
const camelCaseStr = toCamelCase(originalStr);
console.log(camelCaseStr);
</script>
效果:
5:抽奖
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<style type="text/css">
* {
margin: 0;
padding: 0;
}
.container {
width: 800px;
height: 800px;
border: 1px dashed red;
position: absolute;
left: 50%;
margin-left: -400px;
text-align: center;
line-height: 100px;
}
.container .box, .box2 {
width: 300px;
height: 300px;
background: red;
border-radius: 50%;
margin: auto;
margin-top: 50px;
text-align: center;
line-height: 300px;
}
.box2 {
background: deepskyblue;
}
#show {
font-size: 30px;
color: white;
font-weight: bold;
}
#start {
width: 300px;
height: 50px;
background: palevioletred;
}
</style>
</head>
<body>
<div class="container">
<div class="box" id="box">
<span id="show">
奖品
</span>
</div>
<button id="start" onclick="start()">开始抽奖</button>
</div>
<script type="text/javascript">
var flag = false;
var goods = ["Fujifilm X100vi", "iphone13pro", "Nikon Z9", "哈苏907x100c", "MacBook", "Leica M8", "二十代金劵", "网易云黑胶一年"];
var show = document.getElementById("show");
var _start = document.getElementById("start");
var _box = document.getElementById("box")
var timer;
function start() {
if (!flag) {
flag = true;
_start.innerHTML = "停止抽奖"
timer = setInterval(function() {
var index = Math.floor(Math.random()*goods.length);
var good = goods[index]
show.innerText = good;
_box.className = "box2";
}, 10)
} else {
flag = false;
_start.innerHTML = "开始抽奖";
clearInterval(timer);
_box.setAttribute("class", "box");
}
}
</script>
</body>
</html>
效果:
6:星星点灯
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script type="text/javascript">
window.onload = test;
function test() {
document.onclick = start;
}
function start(e) {
var x = e.pageX;
var y = e.pageY;
var image = document.createElement("img");
image.src = "./img/star.jpeg";
image.style.position = "absolute";
var w = getRandom(20,200);
var h = getRandom(20,200);
image.style.width = w +"px";
image.style.top = (y - (h/2))+"px";
image.style.left = (x - (w/2) ) +"px";
document.body.appendChild(image);
}
function getRandom(min,max) {
return parseInt(Math.random()*(max - min + 1)) + min;
}
</script>
</body>
</html>
7: 全选与返选
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Checkbox Select/Deselect Example</title>
<style type="text/css">
li {
height: 30px;
}
</style>
<script type="text/javascript">
function change(node) {
var all_check = document.getElementsByClassName("all_check");
for (var i = 0; i < all_check.length; i++) {
if (node.checked == true) {
all_check[i].checked = true;
} else {
all_check[i].checked = false;
}
}
}
</script>
</head>
<body>
<label>
<input type="checkbox" onclick="change(this)"> 全选
</label>
<label>
<input type="checkbox" class="all_check"> 选项1
</label>
<label>
<input type="checkbox" class="all_check"> 选项2
</label>
<label>
<input type="checkbox" class="all_check"> 选项3
</label>
</body>
</html>
效果:
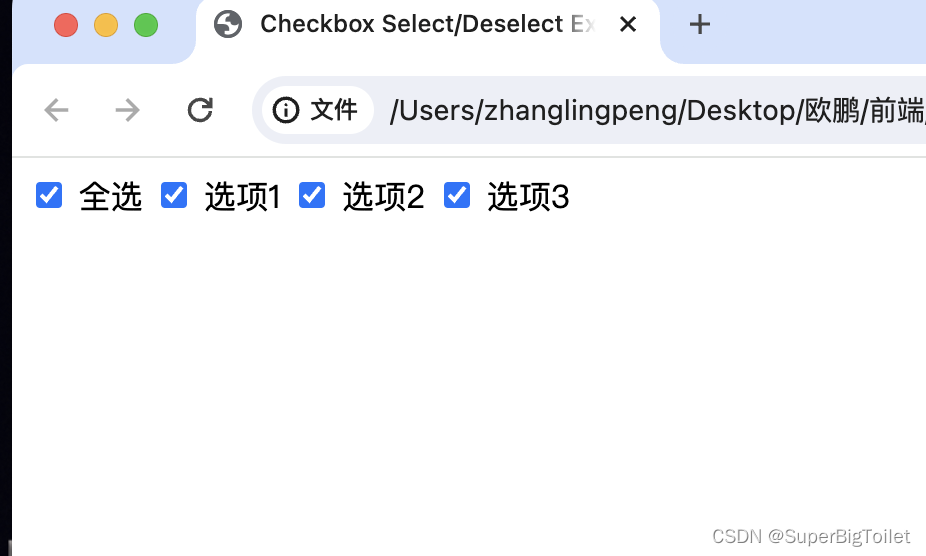