1、思维导图
2、从堆区申请能存5个结构体变量的数组的空间,完成数组中成员的输入,根据学生成绩,用选择排序的方式,对学生排序并输出。
代码:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
//从堆区申请5个结构体变量的数组空间,完成数组成员的输入
//根据学生成绩,用选择排序的方式,对学生排序并输出
typedef struct
{
char name[50];
float score;
}Stu;
void Input(Stu a[]);
void Sort(Stu a[]);
void Output(Stu a[]);
int main(int argc, const char *argv[])
{
//申请数组空间
Stu *p=(Stu*)malloc(sizeof(Stu)*5);
Input(p);
Sort(p);
Output(p);
free(p);//释放空间
p=NULL;
return 0;
}
void Sort(Stu a[]) //简单排序函数
{
int max,i,j;
for(i=0;i<5;i++)
{
max=i;
for(j=i;j<5;j++)
{
if(a[max].score<a[j].score)
max=j;
}
if(max!=i)
{
Stu t=a[i];
a[i]=a[max];
a[max]=t;
}
}
}
void Input(Stu a[]) //输入函数
{
for(int i=0;i<5;i++)
{
printf("输入第%d个学生的姓名:",i+1);
// gets(a[i].name);
scanf("%s",a[i].name);
printf("输入第%d个学生的成绩:",i+1);
scanf("%f",&a[i].score);
}
}
void Output(Stu a[]) //输出函数
{
for(int i=0;i<5;i++)
printf("第%d名的学生姓名是%s,成绩=%.2f\n",i+1,a[i].name,a[i].score);
}
运行结果:
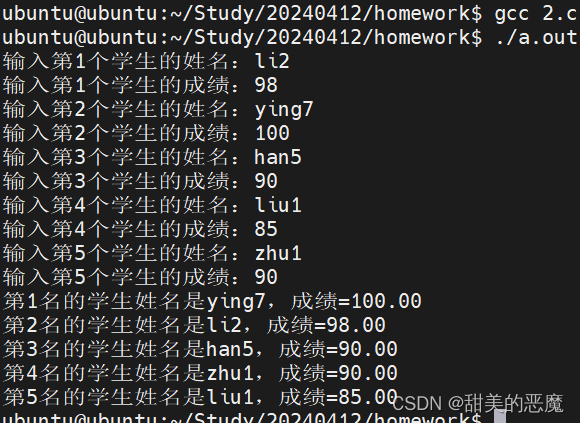
3、求以下结构体的大小
typedef struct {
int id; //4
char name[50]; //50 54-->56
char grade[3]; //3 59-->60
} Student; //60
typedef struct {
int id; //4
char name[50];//50 54-->56
Student student; //60--8 116-->120
} Teacher; //120
typedef struct {
int id; //4
char name[50];//50 54-->56
Teacher teacher;//120--8 176
} Course;//176