目录
1、编写一个简单的JavaFX程序
package demo;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
public class MyJavaFX extends Application {
public void start(Stage primaryStage) {
Button btOK = new Button("OK");
Scene scene = new Scene(btOK, 400, 250);
primaryStage.setTitle("MyJavaFX");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
2、将一个按钮置于一个面板中
package demo;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
public class ButtonInPane extends Application {
public void start(Stage primaryStage) {
StackPane pane = new StackPane();
pane.getChildren().add(new Button("OK"));
Scene scene = new Scene(pane, 400, 250);
primaryStage.setTitle("Button in a pane");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
3、在面板中央显示圆
package demo;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
public class ShowCircle extends Application{
public void start(Stage primaryStage) {
Circle circle = new Circle();
circle.setCenterX(100);
circle.setCenterY(100);
circle.setRadius(50);
circle.setStroke(Color.RED);
circle.setFill(Color.BLUE);
Pane pane = new Pane();
pane.getChildren().add(circle);
Scene scene = new Scene(pane, 200, 200);
primaryStage.setTitle("ShowCircle");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
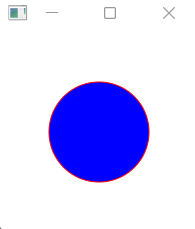
4、窗体改变大小后圆依然显示在中央
圆心的 x 坐 标 和 y坐 标 要重新设置到面板的中央。可以通过将 centerX 和 centerY 分别绑定到面板的 width/2 以及 height/2 上面实现
package demo;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
public class ShowCircleCentered extends Application {
public void start(Stage primaryStage) {
Pane pane = new Pane();
Circle circle = new Circle();
circle.centerXProperty().bind(pane.widthProperty().divide(2));
circle.centerYProperty().bind(pane.heightProperty().divide(2));
circle.setRadius(50);
circle.setStroke(Color.RED);
circle.setFill(Color.BLUE);
pane.getChildren().add(circle);
Scene scene = new Scene(pane, 400, 400);
primaryStage.setTitle("ShowCircle");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
5、创建一个按钮,设置它的样式
并将它加人到一个面板中然后将面板旋转 45°, 设置它的样式为边框颜色为红色,背景颜色为淡灰色
package demo;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.control.Button;
public class NodeStyleRotateDemo extends Application {
public void start(Stage primaryStage) {
StackPane pane = new StackPane();
Button btOK = new Button("OK");
btOK.setStyle("-fx-border-color:blue;");
pane.getChildren().add(btOK);
pane.setRotate(45);
pane.setStyle("sfx-border-color:red; -fx-background-color:lightgray;");
Scene scene = new Scene(pane, 400, 250);
primaryStage.setTitle("NodeStyleRotateDemo");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
6、使用字体Times New Roman
加粗、斜体和大小为 20 来显示一个标签
package demo;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.scene.control.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.text.*;
public class FrontDemo extends Application {
public void start(Stage primaryStage) {
Pane pane = new StackPane();
Circle circle = new Circle();
circle.setCenterX(100);
circle.setCenterY(100);
circle.setRadius(50);
circle.setStroke(Color.RED);
circle.setFill(Color.WHITE);
pane.getChildren().add(circle);
Label label = new Label("JavaFX");
label.setFont(Font.font("Times Nre Roman", FontWeight.BOLD, FontPosture.ITALIC, 20));
pane.getChildren().add(label);
Scene scene = new Scene(pane, 400, 200);
primaryStage.setTitle("FontDemo");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
7、演示 FlowPane 用法的程序。
程序添加标签和文本域到一个 FlowPane 中
package demo;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class ShowFlowPane extends Application {
public void start(Stage primaryStage) {
FlowPane pane = new FlowPane();
pane.setPadding(new Insets(11, 12, 13, 14));
pane.setHgap(5);
pane.setVgap(5);
pane.getChildren().addAll(new Label("First Name"), new TextField(), new Label("MI:"));
TextField tfMi = new TextField();
tfMi.setPrefColumnCount(1);
pane.getChildren().addAll(tfMi, new Label("Last Name:"), new TextField());
Scene scene = new Scene(pane, 400, 100);
primaryStage.setTitle("ShowFlowPane");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
8、演示 GHdPane 的程序
将三个标签、三个文本域,以及一个按钮添加到一个网格的特定位置
package demo;
import javafx.application.Application;
import javafx.geometry.HPos;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class ShowGridPane extends Application {
public void start(Stage primaryStage) {
GridPane pane = new GridPane();
pane.setAlignment(Pos.CENTER);
pane.setPadding(new Insets(11.5, 12.5, 13.5, 14.5));
pane.setHgap(5.5);
pane.setVgap(5.5);
pane.add(new Label("First Name"), 0, 0);
pane.add(new TextField(), 1, 0);
pane.add(new Label("MI:"), 0, 1);
pane.add(new TextField(), 1, 1);
pane.add(new Label("Last Name"), 0, 2);
pane.add(new TextField(), 1, 2);
Button btAdd = new Button("Add Name:");
pane.add(btAdd, 1, 3);
GridPane.setHalignment(btAdd, HPos.RIGHT);
Scene scene = new Scene(pane);
primaryStage.setTitle("ShowGridPane");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
9、演示 BorderPane的使用
程序将五个按钮分别放置 在面板的五个区域
package demo;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ShowBorderPane extends Application {
public void start(Stage primaryStage) {
BorderPane pane = new BorderPane();
pane.setTop(new CustomPane("Top"));
pane.setRight(new CustomPane("Right"));
pane.setBottom(new CustomPane("Bottom"));
pane.setLeft(new CustomPane("Left"));
pane.setCenter(new CustomPane("Center"));
Scene scene = new Scene(pane);
primaryStage.setTitle("ShowBorderPane");
primaryStage.setScene(scene);
primaryStage.show();
}
class CustomPane extends StackPane {
public CustomPane(String title) {
getChildren().add(new Label(title));
setStyle("-fx-border-color:black");
setPadding(new Insets(11.5, 12.5, 13.5, 14.5));
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
10、演示 HBox 和 VBox 的程序
程序将两个按钮放在一个 HBox 中,将五个标签放在一个 VBox 中
package demo;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
public class ShowHBoxVBox extends Application {
public void start(Stage primaryStage) {
BorderPane pane = new BorderPane();
pane.setTop(getHBox());
pane.setLeft(getVBox());
Scene scene = new Scene(pane,400,300);
primaryStage.setTitle("ShowHBoxVBox");
primaryStage.setScene(scene);
primaryStage.show();
}
private HBox getHBox() {
HBox hBox = new HBox(15);
hBox.setPadding(new Insets(15, 15, 15, 15));
hBox.setStyle("-fx-background-color:gold");
hBox.getChildren().add(new Button("Computer Science"));
hBox.getChildren().add(new Button("Chemistry"));
return hBox;
}
private VBox getVBox() {
VBox vBox = new VBox(15);
vBox.setPadding(new Insets(15, 5, 5, 5));
vBox.getChildren().add(new Label("Courses"));
Label[] courses = { new Label("CSCI 1301"), new Label("CSCI 1302"), new Label("CSCI 2410"),
new Label("CSCI 3720") };
for (Label course : courses) {
VBox.setMargin(course, new Insets(0, 0, 0, 15));
vBox.getChildren().add(course);
}
return vBox;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
12、演示文本
package demo;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.geometry.Insets;
import javafx.stage.Stage;
import javafx.scene.text.Text;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.FontPosture;
public class ShowText extends Application {
public void start(Stage primaryStage) {
Pane pane = new Pane();
pane.setPadding(new Insets(5, 5, 5, 5));
Text text1 = new Text(20, 20, "Programming is fun");
text1.setFont(Font.font("Courier", FontWeight.BOLD, FontPosture.ITALIC, 15));
pane.getChildren().add(text1);
Text text2 = new Text(10, 60, "Programming is fun\nDisplay text");
pane.getChildren().add(text2);
Text text3 = new Text(10, 100, "Programming is fun\nDisplay text");
text3.setFill(Color.BLUE);
text3.setUnderline(true);
text3.setStrikethrough(true);
pane.getChildren().add(text3);
Scene scene = new Scene(pane, 400, 300);
primaryStage.setTitle("ShowText");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
13、演示直线
package demo;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.scene.shape.Line;
public class ShowLine extends Application {
public void start(Stage primaryStage) {
Scene scene = new Scene(new LinePane(), 400, 300);
primaryStage.setTitle("ShowLine");
primaryStage.setScene(scene);
primaryStage.show();
}
class LinePane extends Pane {
public LinePane() {
Line line1 = new Line(10, 10, 10, 10);
line1.endXProperty().bind(widthProperty().subtract(10));
line1.endYProperty().bind(heightProperty().subtract(10));
line1.setStrokeWidth(5);
line1.setStroke(Color.PINK);
getChildren().add(line1);
Line line2 = new Line(10, 10, 10, 10);
line2.startXProperty().bind(widthProperty().subtract(10));
line2.endYProperty().bind(heightProperty().subtract(10));
line2.setStrokeWidth(5);
line2.setStroke(Color.GOLD);
getChildren().add(line2);
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
14、演示矩形
package demo;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.scene.text.Text;
import javafx.scene.shape.Rectangle;
public class ShowRectangle extends Application {
public void start(Stage primaryStage) {
Pane pane = new Pane();
Rectangle r1 = new Rectangle(25, 10, 60, 30);
r1.setStroke(Color.BLACK);
r1.setFill(Color.PINK);
pane.getChildren().add(new Text(10, 27, "r1"));
pane.getChildren().add(r1);
Rectangle r2 = new Rectangle(25, 50, 60, 30);
pane.getChildren().add(new Text(10, 67, "r2"));
pane.getChildren().add(r2);
Rectangle r3 = new Rectangle(25, 90, 60, 30);
r3.setArcWidth(15);
r3.setArcHeight(25);
pane.getChildren().add(new Text(10, 107, "r3"));
pane.getChildren().add(r3);
for (int i = 0; i < 4; i++) {
Rectangle r = new Rectangle(100, 50, 100, 30);
r.setRotate(i * 360 / 8);
r.setStroke(Color.color(Math.random(), Math.random(), Math.random()));
r.setFill(Color.GOLD);
pane.getChildren().add(r);
}
Scene scene = new Scene(pane, 400, 300);
primaryStage.setTitle("ShowRectangle");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
15、演示椭圆
package demo;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.scene.shape.Ellipse;
public class ShowEllipse extends Application {
public void start(Stage primaryStage) {
Pane pane = new Pane();
for (int i = 0; i < 16; i++) {
Ellipse e1 = new Ellipse(150, 100, 100, 50);
e1.setStroke(Color.BLACK);
e1.setFill(Color.GOLD);
e1.setRotate(i * 180 / 16);
pane.getChildren().add(e1);
}
Scene scene = new Scene(pane, 400, 300);
primaryStage.setTitle("ShowEllipse");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
16、演示弧
package demo;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.scene.shape.Arc;
import javafx.scene.shape.ArcType;
import javafx.scene.text.Text;
public class ShowArc extends Application {
public void start(Stage primaryStage) {
Pane pane = new Pane();
Arc arc1 = new Arc(150, 100, 80, 80, 30, 35);
arc1.setFill(Color.RED);
arc1.setType(ArcType.ROUND);
pane.getChildren().add(new Text(210, 40, "arc1:round"));
pane.getChildren().add(arc1);
Arc arc2 = new Arc(150, 100, 80, 80, 30 + 90, 35);
arc2.setFill(Color.WHITE);
arc2.setType(ArcType.OPEN);
arc2.setStroke(Color.BLACK);
pane.getChildren().add(new Text(20, 40, "arc2:open"));
pane.getChildren().add(arc2);
Arc arc3 = new Arc(150, 100, 80, 80, 30 + 180, 35);
arc3.setFill(Color.WHITE);
arc3.setType(ArcType.CHORD);
arc3.setStroke(Color.BLACK);
pane.getChildren().add(new Text(20, 170, "arc3:chord"));
pane.getChildren().add(arc3);
Arc arc4 = new Arc(150, 100, 80, 80, 30 + 270, 35);
arc4.setFill(Color.WHITE);
arc4.setType(ArcType.CHORD);
arc4.setStroke(Color.BLACK);
pane.getChildren().add(new Text(210, 170, "arc4:chord"));
pane.getChildren().add(arc4);
Scene scene = new Scene(pane, 400, 300);
primaryStage.setTitle("ShowArc");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果
17、创建六边形
package demo;
import javafx.application.Application;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.scene.shape.Polygon;
public class ShowPolyon extends Application {
public void start(Stage primaryStage) {
Pane pane = new Pane();
Polygon polygon = new Polygon();
pane.getChildren().add(polygon);
polygon.setFill(Color.WHITE);
polygon.setStroke(Color.BLACK);
ObservableList<Double> list = polygon.getPoints();
final double WIDTH = 200, HEIGHT = 200;
double centerX = WIDTH / 2, centerY = HEIGHT / 2;
double radius = Math.min(WIDTH, HEIGHT) * 0.4;
for (int i = 0; i < 6; i++) {
list.add(centerX + radius * Math.cos(2 * i * Math.PI / 6));
list.add(centerX + radius * Math.sin(2 * i * Math.PI / 6));
}
Scene scene = new Scene(pane, 400, 300);
primaryStage.setTitle("ShowPolygon");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Application.launch(args);
}
}
运行结果