1.WebServer项目
1.1项目目录
1.1.1Java目录
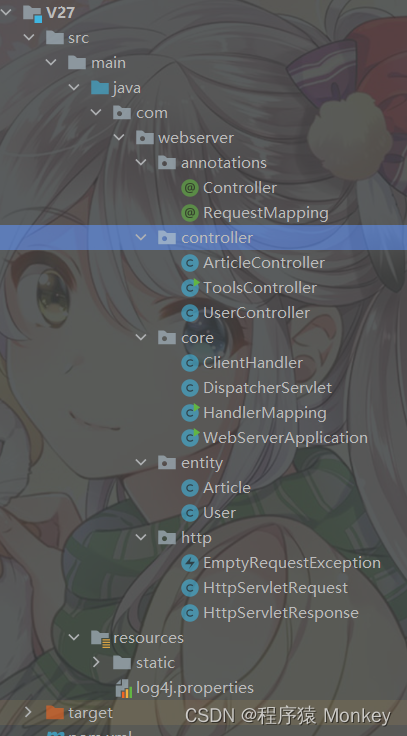
1.1.2HTML目录
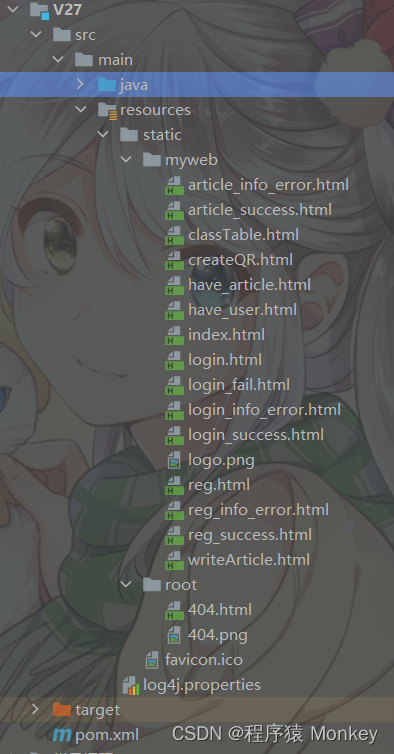
1.2Java代码
1.2.1Controller(注解)
package com.webserver.annotations;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
public @interface Controller {
}
1.2.2RequestMapping(注解)
package com.webserver.annotations;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface RequestMapping {
String value();
}
1.2.3ArticleController(文章业务)
package com.webserver.controller;
import com.webserver.annotations.Controller;
import com.webserver.annotations.RequestMapping;
import com.webserver.core.ClientHandler;
import com.webserver.entity.Article;
import com.webserver.http.HttpServletRequest;
import com.webserver.http.HttpServletResponse;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.net.URISyntaxException;
@Controller
public class ArticleController {
private static File rootDir;
private static File staticDir;
//表示articles目录
private static File articleDir;
static{
try {
rootDir = new File(
ClientHandler.class.getClassLoader()
.getResource(".").toURI()
);
staticDir = new File(rootDir,"static");
} catch (URISyntaxException e) {
e.printStackTrace();
}
articleDir = new File("articles");
if(!articleDir.exists()){
articleDir.mkdirs();
}
}
@RequestMapping("/myweb/writeArticle")
public void writeArticle(HttpServletRequest request, HttpServletResponse response){
//1获取表单数据
String title = request.getParameter("title");
String author = request.getParameter("author");
String content = request.getParameter("content");
if(title==null||author==null||content==null){
File file = new File(staticDir,"/myweb/article_info_error.html");
response.setContentFile(file);
return;
}
//2写入文件
File articleFile = new File(articleDir,title+".obj");
if(articleFile.exists()){//文件存在,说明重复的标题,不能发表(需求过于严苛,后期数据库可通过ID避免该问题).
File file = new File(staticDir,"/myweb/have_article.html");
response.setContentFile(file);
return;
}
Article article = new Article(title,author,content);
try (
FileOutputStream fos = new FileOutputStream(articleFile);
ObjectOutputStream oos = new ObjectOutputStream(fos);
){
oos.writeObject(article);
File file = new File(staticDir,"/myweb/article_success.html");
response.setContentFile(file);
} catch (IOException e) {
e.printStackTrace();
}
//3响应页面
}
}
1.2.4ToolsController(二维码,验证码业务)
package com.webserver.controller;
import com.webserver.annotations.Controller;
import com.webserver.annotations.RequestMapping;
import com.webserver.http.HttpServletRequest;
import com.webserver.http.HttpServletResponse;
import qrcode.QRCodeUtil;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Random;
@Controller
public class ToolsController {
@RequestMapping("/myweb/createQR")
public void createQR(HttpServletRequest request, HttpServletResponse response){
String line = request.getParameter("content");
try {
response.setContentType("image/jpeg");
QRCodeUtil.encode(
line, //二维码上显示的文字
"./logo.jpg", //二维码中间的logo图
response.getOutputStream(),//生成的二维码数据会通过该流写出
true);//是否压缩logo图片的尺寸
System.out.println("二维码已生成");
} catch (Exception e) {
e.printStackTrace();
}
}
@RequestMapping("/myweb/random.jpg")
public void createRandomImage(HttpServletRequest request,HttpServletResponse response){
response.setContentType("image/jpeg");
//1创建一张空白图片,同时指定宽高 理解为:搞一张白纸,准备画画
BufferedImage image = new BufferedImage(
70,30,BufferedImage.TYPE_INT_RGB
);
//2通过图片获取绘制该图片的画笔,通过这个画笔就可以往该图片上绘制了
Graphics g = image.getGraphics();
Random random = new Random();
//3随机生成个颜色涂满整张画布
Color color = new Color(
random.nextInt(256),
random.nextInt(256),
random.nextInt(256));
g.setColor(color);//设置画笔颜色
g.fillRect(0,0,70,30);
//4向画布上绘制文字
String line = "abcdefghjiklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
g.setFont(new Font(null,Font.BOLD,20));
for(int i=0;i<4;i++) {
color = new Color(
random.nextInt(256),
random.nextInt(256),
random.nextInt(256));
g.setColor(color);//设置画笔颜色
String str = line.charAt(random.nextInt(line.length())) + "";
g.drawString(str, i*15+5, 18+ random.nextInt(11)-5);
}
//5随机生成5条干扰线
for(int i=0;i<5;i++){
color = new Color(
random.nextInt(256),
random.nextInt(256),
random.nextInt(256));
g.setColor(color);//设置画笔颜色
g.drawLine(random.nextInt(71), random.nextInt(31),
random.nextInt(71), random.nextInt(31));
}
try {
ImageIO.write(image,"jpg",
response.getOutputStream());
System.out.println("图片已生成");
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
//1创建一张空白图片,同时指定宽高 理解为:搞一张白纸,准备画画
BufferedImage image = new BufferedImage(
70,30,BufferedImage.TYPE_INT_RGB
);
//2通过图片获取绘制该图片的画笔,通过这个画笔就可以往该图片上绘制了
Graphics g = image.getGraphics();
Random random = new Random();
//3随机生成个颜色涂满整张画布
Color color = new Color(
random.nextInt(256),
random.nextInt(256),
random.nextInt(256));
g.setColor(color);//设置画笔颜色
g.fillRect(0,0,70,30);
//4向画布上绘制文字
String line = "abcdefghjiklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
g.setFont(new Font(null,Font.BOLD,20));
for(int i=0;i<4;i++) {
color = new Color(
random.nextInt(256),
random.nextInt(256),
random.nextInt(256));
g.setColor(color);//设置画笔颜色
String str = line.charAt(random.nextInt(line.length())) + "";
g.drawString(str, i*15+5, 18+ random.nextInt(11)-5);
}
//5随机生成5条干扰线
for(int i=0;i<5;i++){
color = new Color(
random.nextInt(256),
random.nextInt(256),
random.nextInt(256));
g.setColor(color);//设置画笔颜色
g.drawLine(random.nextInt(71), random.nextInt(31),
random.nextInt(71), random.nextInt(31));
}
try {
ImageIO.write(image,"jpg",
new FileOutputStream("./random.jpg"));
System.out.println("图片已生成");
} catch (IOException e) {
e.printStackTrace();
}
}
// public static void main(String[] args) {
// try {
// String line = "扫你妹!";
// //参数1:二维码上显示的文字,参数2:二维码图片生成的位置
QRCodeUtil.encode(line,"./qr.jpg");
//
// //参数1:二维码上显示的文字,参数2:二维码中间的logo图,
// //参数3:二维码生成的位置,参数4:是否压缩logo图片的尺寸
QRCodeUtil.encode(line,"./logo.jpg",
"./qr.jpg",true);
//
// QRCodeUtil.encode(
// line, //二维码上显示的文字
// "./logo.jpg", //二维码中间的logo图
// new FileOutputStream("./qr.jpg"),//生成的二维码数据会通过该流写出
// true);//是否压缩logo图片的尺寸
//
// System.out.println("二维码已生成");
// } catch (Exception e) {
// e.printStackTrace();
// }
// }
}
1.2.5UserController(用户业务)
package com.webserver.controller;
import com.webserver.annotations.Controller;
import com.webserver.annotations.RequestMapping;
import com.webserver.entity.User;
import com.webserver.http.HttpServletRequest;
import com.webserver.http.HttpServletResponse;
import org.apache.log4j.Logger;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
/**
* 处理与用户相关的业务操作
*/
@Controller
public class UserController {
private static Logger logger = Logger.getLogger(UserController.class);
//表示users目录
private static File userDir;
static {
userDir = new File("users");
if (!userDir.exists()) {
userDir.mkdirs();
}
}
/**
* 生成展示所有用户信息的动态页面
*
* @param request
* @param response
*/
@RequestMapping("/myweb/showAllUser")
public void showAllUser(HttpServletRequest request, HttpServletResponse response) {
/*
1:通过读取users目录下所有的obj文件,进行反序列化
得到所有的注册用户信息(若干的User对象)将他们存入一个List集合备用
2:拼接一个HTML页面,并将所有用户信息拼接到table表格中用于展示给用户
3:将拼接好的HTML代码作为正文给浏览器响应回去
*/
//1
List<User> userList = new ArrayList<>();
/*
1.1:通过File的listFiles方法,从userDir目录下获取所有的obj文件
要使用文件过滤器
1.2:遍历每一个obj文件,并利用对象流与文件流的连接,将obj文件中的
User对象反序列化出来
1.3:将反序列化的User对象存入userList集合中
*/
//1.1
File[] subs = userDir.listFiles(f -> f.getName().endsWith(".obj"));
//1.2
for (File userFile : subs) {
try (
FileInputStream fis = new FileInputStream(userFile);
ObjectInputStream ois = new ObjectInputStream(fis);
) {
User user = (User) ois.readObject();
//1.3
userList.add(user);
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
System.out.println(userList);
//2将数据拼接到html生成页面
response.setContentType("text/html");
PrintWriter pw = response.getWriter();
pw.println("<!DOCTYPE html>");
pw.println("<html lang=\"en\">");
pw.println("<head>");
pw.println("<meta charset=\"UTF-8\">");
pw.println("<title>用户列表</title>");
pw.println("</head>");
pw.println("<body>");
pw.println("<center>");
pw.println("<h1>用户列表</h1>");
pw.println("<table border=\"1\">");
pw.println("<tr>");
pw.println("<td>用户名</td>");
pw.println("<td>密码</td>");
pw.println("<td>昵称</td>");
pw.println("<td>年龄</td>");
pw.println("</tr>");
for (User user : userList) {
pw.println("<tr>");
pw.println("<td>" + user.getUsername() + "</td>");
pw.println("<td>" + user.getPassword() + "</td>");
pw.println("<td>" + user.getNickname() + "</td>");
pw.println("<td>" + user.getAge() + "</td>");
pw.println("</tr>");
}
pw.println("</table>");
pw.println("</center>");
pw.println("</body>");
pw.println("</html>");
System.out.println("页面已生成");
}
@RequestMapping("/myweb/login")
public void login(HttpServletRequest request, HttpServletResponse