import numpy as np
x1 = np.array([0, 0, 1, 1])
x2 = np.array([0, 1, 0, 1])
# and运算
y = np.array([0, 0, 0, 1])
# or运算
y1 = np.array([0, 1, 1, 1])
# 设初始值
w0 = -0.8
w1 = 0.5
w2 = 0.5
alpha = 0.001
# 阶跃函数
def fx(x1, x2):
return w1*x1+w2*x2+w0
# 激活函数
def hy(xx1, xx2):
if(fx(xx1,xx2)>0.5):
return 1
else:
return 0
# and运算循环5000次
for i in range(5000):
w0 = w0 - (fx(x1[i % 4], x2[i % 4]) - y[i % 4]) * alpha
w1 = w1 - (fx(x1[i % 4], x2[i % 4]) - y[i % 4]) * x1[i % 4] * alpha
w2 = w2 - (fx(x1[i % 4], x2[i % 4]) - y[i % 4]) * x2[i % 4] * alpha
# 如果偏导小于0.001,输出此时权重及偏导
if (abs(fx(x1[i % 4], x2[i % 4]) - y[i % 4]) < 0.001 and abs(
(fx(x1[i % 4], x2[i % 4]) - y[i % 4]) * x1[i % 4]) < 0.001 and abs(
(fx
python实现感知器
于 2022-09-29 21:37:27 首次发布
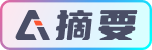