package cn.tedu;
//模拟Servlet解析请求参数的对象Request
public class MyRequest {
public static void main(String[] args) {
//this是代表的本类对象,static里不能出现this,原因就是加载顺序
// this.getParamter();
MyRequest my = new MyRequest();
my.getParamter();
}
//1,getParamter()获取每个请求参数
public void getParamter(){
String url="http://localhost:8090/cgb2109javaweb03_war_exploded/ServletDemo6" +
"?user=jack&pwd=123" ;
// 1,按照?切割字符串,切出来两部分,存入数组
//[http://localhost:8090/cgb2109javaweb03/ServletDemo6,user=jack&pwd=123]
String[] strs = url.split("\\?");//转义
// 2,重点解析数组中的第二个元素,下标为1的元素
String datas = strs[1];//user=jack&pwd=123
// 3,把第二步的结果,按照&切割
//[user=jack,pwd=123]
String[] data = datas.split("&");
// 4,遍历数组,获取每个数据
for(String s : data){//遍历两次,第一次s是user=jack,第二次s是pwd=123
// 5,按照=切割,得到数组 [user,jack],只要第二个元素
// String params = s.split("=")[1];//和下面两行等效,只是简写形式
String[] ss = s.split("=");
String params = ss[1];
System.out.println(params);
}
}
}
二,Servlet 解析post方式提交的数据
–1,测试
package cn.tedu.request;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/ServletDemo6")
public class ServletDemo6 extends HttpServlet {
//总结1:Servlet什么时候会自动调用doPost()?什么时候会自动调用doGet()?
// Servlet会调用getMethod()来获取用户的访问方式,如果是POST会调用doPost()
// Servlet会调用getMethod()来获取用户的访问方式,如果是GET会调用doGet()
//总结2:中文乱码问题?
// get方式提交数据没有乱码问题,Tomcat已经配置好了(URIEncoding="utf-8"),不必关心
// post方式提交的数据如果有中文一定乱码,request.setCharacterEncoding("utf-8");
//TODO 处理post方式提交的数据--如果请求参数包含中,一定乱码!!
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//验证,请求方式是post吗?
String method = request.getMethod();
//指定请求的方式使用的编码--解决中文乱码
request.setCharacterEncoding("utf-8");
String name=request.getParameter("user");
String pwd = request.getParameter("pwd");
System.out.println("doPost..."+name+pwd+"..."+method);
}
//ServletDemo6?user=jack&pwd=123
//处理get方式提交的数据:如果出现中文不会乱码,Tomcat7.0以上都处理过了
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String method = request.getMethod();
String user = request.getParameter("user");//获取user的值
String pwd = request.getParameter("pwd");//获取pwd的值
System.out.println("doGet..."+user+pwd+"..."+method);
}
}
–2,前端HTML页面
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<form action="http://localhost:8090/cgb2109javaweb03_war_exploded/ServletDemo6">
<h3>测试 get提交数据</h3>
姓名:<input type="text" placeholder="请输入姓名..." name="user"/> <br />
密码:<input type="password" placeholder="请输入密码..." name="pwd"/> <br />
<input type="submit" value="提交"/>
</form>
<form method="post"
action="http://localhost:8090/cgb2109javaweb03_war_exploded/ServletDemo6">
<h3>测试 post提交数据</h3>
姓名:<input type="text" placeholder="请输入姓名..." name="user"/> <br />
密码:<input type="password" placeholder="请输入密码..." name="pwd"/> <br />
<input type="submit" value="提交"/>
</form>
</body>
</html>
三,综合案例
–1,需求
综合案例:
1,准备前端HTML网页,让用户输入部门数据(编号,名称,地址)
2,准备DeptServlet,接受请求,并且解析请求参数
3,把解析成功的请求参数,进行入库
3.1,准备jar包
3.2,准备dept表(deptno,dname,loc)
3.3,准备jdbc代码,进行insert的SQL语句操作
–2,创建HTML网页
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>前后端大融合</title>
<style>
input[type='text']{
width: 200px;/* 宽度*/
height: 30px;/* 高度 */
background-color: pink;/* 背景色 */
border-color: pink;/* 边框色 */
border-radius: 10px;/* 圆角边框 */
}
input[type="submit"]{
width: 80px;/* 宽度*/
height: 40px;/* 高度 */
background-color: gray;/* 背景色 */
border-color: gray;/* 边框色 */
color: white;/* 文字的颜色 */
font-size: 20px;/* 字号大小 */
}
</style>
</head>
<body>
<form method="post" action="http://localhost:8090/cgb2109javaweb03_war_exploded/DeptServlet">
<h1>添加部门数据</h1>
<h3>编号:<input type="text" name="deptno"/></h3>
<h3>名称:<input type="text" name="dname"/></h3>
<h3>地址:<input type="text" name="loc"/></h3>
<input type="submit" value="保存" />
</form>
</body>
</html>
–3,创建DeptServlet类
package cn.tedu.dept;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
@WebServlet("/DeptServlet")
public class DeptServlet extends HttpServlet {
//用户以post方式提交的数据,doPost()解析请求参数
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try{
//防止中文乱码
request.setCharacterEncoding("utf-8");
//用来获取网页中传递来的参数,
//getParameter()的参数是网页中name属性的值
String a = request.getParameter("deptno");
String b = request.getParameter("dname");
String c = request.getParameter("loc");
//TODO jdbc--把解析到的参数入库,,insert
//获取驱动
Class.forName("com.mysql.jdbc.Driver");
//获取连接
String url="jdbc:mysql:///cgb2109?characterEncoding=utf8";
Connection con=DriverManager.getConnection(url,"root","root");
//获取传输器Statement不安全低效,PreparedStatement安全防止了SQL攻击高效
String sql="insert into dept values(?,?,?)";//sql骨架,?是占位符
PreparedStatement p = con.prepareStatement(sql);
//设置SQL的参数,执行SQL
p.setObject(1,a);
p.setObject(2,b);
p.setObject(3,c);
p.executeUpdate();
//关闭资源
p.close();
con.close();
}catch (Exception e){
System.out.println("插入失败,请检查数据~");
}
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//post提交的,不能写在这里,
}
}
–4,测试
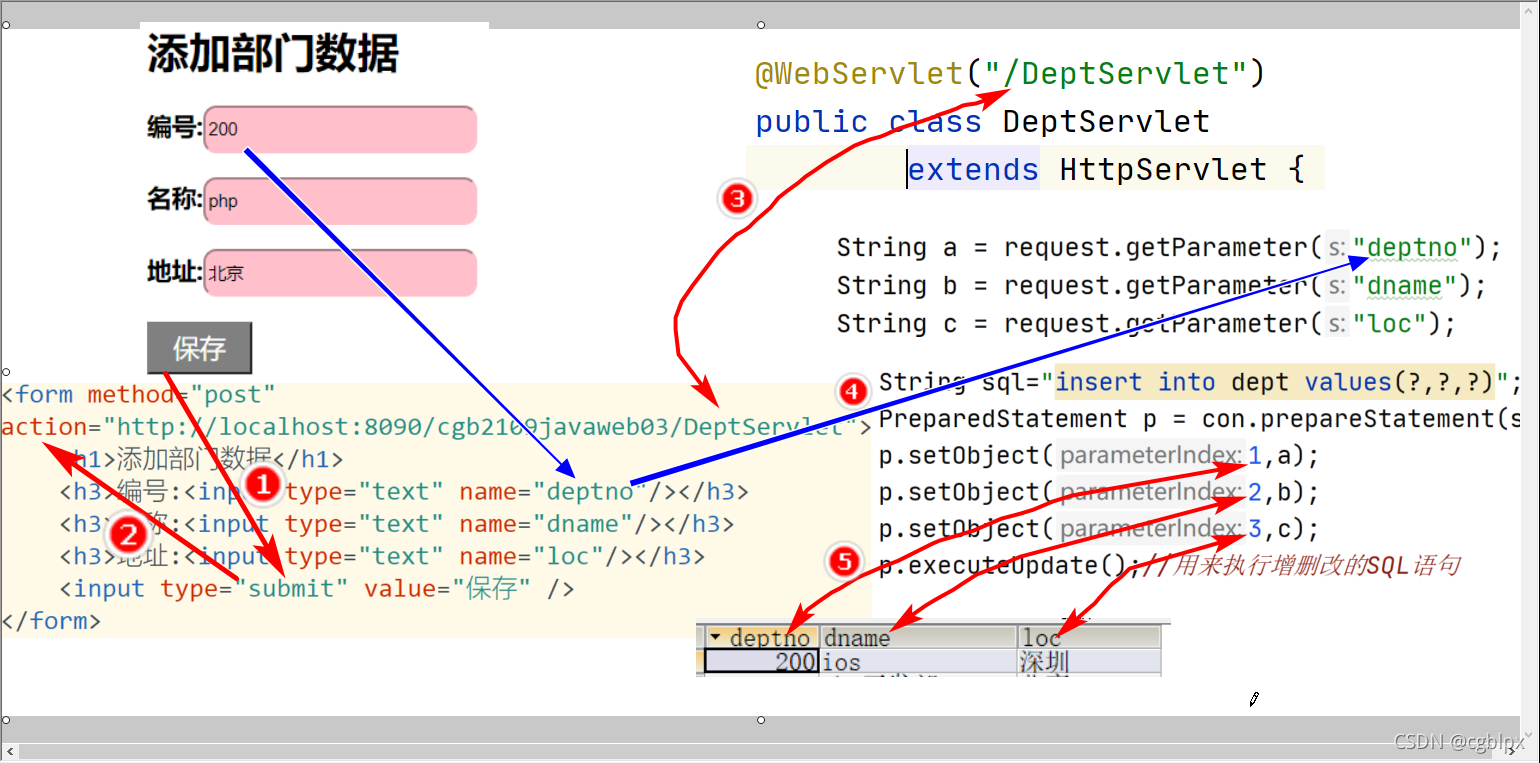
利用Jdbc将前端所设置的值 输入到数据库内