一.创建更新DDL
NHibernate.config配置文件
<?xml version="1.0" encoding="utf-8" ?>
<hibernate-configuration xmlns="urn:nhibernate-configuration-2.2" >
<session-factory>
<property name="connection.connection_string">
Data Source=.\SQLINSTANCE;Initial Catalog=NHibernateDemo;Persist Security Info=True;User ID=sa;Password=123456
</property>
<property name="connection.driver_class">NHibernate.Driver.SqlClientDriver</property>
<property name="dialect">NHibernate.Dialect.MsSql2008Dialect</property>
<property name='proxyfactory.factory_class'>NHibernate.ByteCode.Castle.ProxyFactoryFactory, NHibernate.ByteCode.Castle</property>
<mapping assembly='Model'/>
</session-factory>
</hibernate-configuration>
Model类库如下:
Blog.cs类和Blog.hbm.xml实体映射文件
public class Blog
{
public Blog()
{
Tags = new HashSet<Tag>();
Users = new HashSet<User>();
Posts = new HashSet<Post>();
SecurityKey = Guid.NewGuid();
}
public virtual int Id { get; set; }
public virtual Guid SecurityKey { get; set; }
public virtual ICollection<Post> Posts { get; set; }
public virtual ICollection<User> Users { get; set; }
public virtual ICollection<Tag> Tags { get; set; }
[Field]
public virtual string Title { get; set; }
[Field]
public virtual string Subtitle { get; set; }
public virtual string LogoImage { get; set; }
public virtual bool AllowsComments { get; set; }
public virtual DateTime CreatedAt { get; set; }
}
<?xml version="1.0" encoding="utf-8" ?>
<hibernate-mapping xmlns="urn:nhibernate-mapping-2.2"
assembly="Model"
namespace="Model">
<class name="Blog"
table="Blogs">
<cache usage="read-write"/>
<id name="Id">
<generator class="identity"/>
</id>
<property name="Title"/>
<property name="Subtitle"/>
<property name="SecurityKey"/>
<property name="AllowsComments"/>
<property name="CreatedAt"/>
<set name="Users"
table="UsersBlogs">
<key column="BlogId"/>
<many-to-many class="User"
column="UserId"/>
</set>
<set name="Posts"
table="Posts">
<key column ="BlogId"/>
<one-to-many class="Post"/>
</set>
<set name="Tags"
where="ISNULL(ItemType,2) = 2"
table="Tags">
<cache usage="read-write"/>
<key column="ItemId"/>
<one-to-many class="Tag"/>
</set>
</class>
<sql-query name="test">
<return-scalar column="Id"
type="System.Int32"/>
select b.id as id from Blogs b
</sql-query>
</hibernate-mapping>
Category.cs类和Category.hbm.xml实体映射文件
public class Category
{
public Category()
{
Posts = new HashSet<Post>();
}
public virtual string Name { get; set; }
public virtual int Id { get; set; }
public virtual ICollection<Post> Posts { get; set; }
}
<?xml version="1.0" encoding="utf-8" ?>
<hibernate-mapping xmlns="urn:nhibernate-mapping-2.2"
assembly="Model"
namespace="Model">
<class name="Category"
table="Categories">
<id name="Id">
<generator class ="assigned"/>
</id>
<property name="Name"/>
<set name="Posts"
table="CategoriesPosts">
<key column="CategoryId"/>
<many-to-many class="Post"
column="PostId" />
</set>
</class>
</hibernate-mapping>
public class Comment
{
public virtual int Id { get; set; }
public virtual Post Post { get; set; }
public virtual string Name { get; set; }
public virtual string Email { get; set; }
public virtual string HomePage { get; set; }
public virtual int Ip { get; set; }
public virtual string Text { get; set; }
}
<?xml version="1.0" encoding="utf-8" ?>
<hibernate-mapping xmlns="urn:nhibernate-mapping-2.2"
assembly="Model"
namespace="Model">
<class name="Comment"
table="Comments">
<id name="Id">
<generator class="identity"/>
</id>
<property name="Name"/>
<property name="Email"/>
<property name="HomePage"/>
<property name="Ip"/>
<property name="Text"/>
<many-to-one name="Post" class="Post"
column="PostId"/>
</class>
</hibernate-mapping>
Post.cs类和Post.hbm.xml实体映射文件
[Indexed]
public class Post
{
public Post()
{
Tags = new HashSet<Tag>();
Categories = new HashSet<Category>();
Comments = new HashSet<Comment>();
}
[DocumentId]
public virtual int Id { get; set; }
[IndexedEmbedded]
public virtual Blog Blog { get; set; }
[IndexedEmbedded]
public virtual User User { get; set; }
[Field]
public virtual string Title { get; set; }
[Field]
public virtual string Text { get; set; }
[Field]
public virtual DateTime PostedAt { get; set; }
public virtual ICollection<Comment> Comments { get; set; }
public virtual ICollection<Category> Categories { get; set; }
public virtual ICollection<Tag> Tags { get; set; }
}
<?xml version="1.0" encoding="utf-8" ?>
<hibernate-mapping xmlns="urn:nhibernate-mapping-2.2"
assembly="Model"
namespace="Model">
<class name="Post"
table="Posts">
<id name="Id">
<generator class="identity"/>
</id>
<property name="Title"/>
<property name="Text"/>
<property name="PostedAt"/>
<many-to-one name="Blog" class="Blog"
column="BlogId"/>
<many-to-one name="User" class="User"
column="UserId"/>
<set name="Comments"
table="Comments">
<key column="PostId"/>
<one-to-many class="Comment"/>
</set>
<set name="Categories"
table="CategoriesPosts">
<key column="PostId"/>
<many-to-many class="Category"
column="CategoryId"/>
</set>
<set name="Tags"
where="ISNULL(ItemType,1) = 1"
table="Tags">
<key column="ItemId"/>
<one-to-many class="Tag"/>
</set>
</class>
</hibernate-mapping>
ReadOnlyBlog.cs类和ReadOnlyBlog.hbm.xml实体映射文件
public class ReadOnlyBlog
{
public virtual int Id { get; set; }
public virtual string Title { get; set; }
}
<?xml version="1.0" encoding="utf-8" ?>
<hibernate-mapping xmlns="urn:nhibernate-mapping-2.2"
assembly="Model"
namespace="Model">
<class name="ReadOnlyBlog"
mutable="true"
table="Blogs">
<cache usage="read-only"/>
<id name="Id">
<generator class="native"/>
</id>
<property name="Title"/>
</class>
</hibernate-mapping>
Tag.cs类和Tag.hbm.xml实体映射文件
public class Tag
{
public virtual int Id { get; set; }
public virtual object Entity { get; set; }
public virtual string Name { get; set; }
}
<?xml version="1.0" encoding="utf-8" ?>
<hibernate-mapping xmlns="urn:nhibernate-mapping-2.2"
assembly="Model"
namespace="Model">
<class name="Tag" table="Tags">
<id name="Id">
<generator class="identity"/>
</id>
<property name="Name"/>
<any name="Entity"
id-type="Int32"
meta-type="Int32">
<meta-value class="Post" value="1"/>
<meta-value class="Blog" value ="2"/>
<column name="ItemId"/>
<column name="ItemType"/>
</any>
</class>
</hibernate-mapping>
User.cs类和User.hbm.xml实体映射文件
public class User : IUser
{
public User()
{
Posts = new HashSet<Post>();
Blogs = new HashSet<Blog>();
}
public virtual ICollection<Blog> Blogs { get; set; }
public virtual ICollection<Post> Posts { get; set; }
public virtual int Id { get; set; }
public virtual byte[] Password { get; set; }
[Field]
public virtual string Email { get; set; }
[Field]
public virtual string Username { get; set; }
public virtual DateTime CreatedAt { get; set; }
public virtual string Bio { get; set; }
public virtual SecurityInfo SecurityInfo
{
get { return new SecurityInfo(Username, Id); }
}
}
<?xml version="1.0" encoding="utf-8" ?>
<hibernate-mapping xmlns="urn:nhibernate-mapping-2.2"
assembly="Model"
namespace="Model">
<class name="User"
table="Users">
<id name="Id">
<generator class="identity"/>
</id>
<property name="Password"/>
<property name="Username"/>
<property name="Email"/>
<property name="CreatedAt"/>
<property name="Bio"/>
<set name="Posts"
table="Posts">
<key column="UserId"/>
<one-to-many class="Post"/>
</set>
<set name="Blogs"
inverse="true"
table="UsersBlogs">
<key column="UserId"/>
<many-to-many class="Blog"
column="BlogId"/>
</set>
</class>
</hibernate-mapping>
Program.cs代码如下:
public class Program
{
public static void Main(string[] args)
{
NHibernateProfiler.Initialize();
try
{
var configuration = new Configuration().Configure("NHibernate.config");
new SchemaExport(configuration).Create(true, true);
var factory = configuration.BuildSessionFactory();
using(var s = factory.OpenSession())
using(s.BeginTransaction())
{
for (int i = 0; i < 30; i++)
{
s.Save(new Blog
{
CreatedAt = DateTime.Now,
Title = "a"
});
}
s.Transaction.Commit();
}
}
catch (Exception e)
{
Console.WriteLine(e);
}
LogManager.Shutdown();
}
}
结果如图:
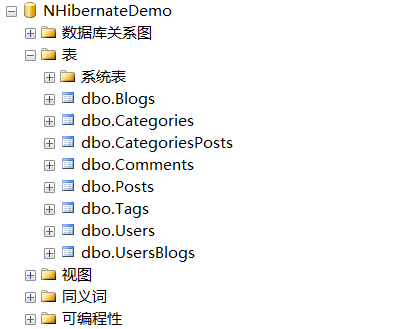