HomeController.cs
using RedisMonitor.Core.Model;
using RedisMonitor.Core.RedisServer;
using System.Linq;
using System.Web.Mvc;
namespace RedisManager
{
public class HomeController : Controller
{
#region 视图
public ActionResult Index()
{
var redisServers = RedisServerConfig.RedisServers;
ViewBag.RedisServers = redisServers;
return View();
}
public ActionResult Details(string serverId)
{
return View();
}
public ActionResult SimpleDetails(string serverId)
{
return PartialView();
}
public ActionResult RichDetails(string serverId)
{
var result = RedisServerHelp.GetRedisInfo(serverId);
ViewBag.InfoMsg = result;
return PartialView();
}
public ActionResult InfoInstructions()
{
return View();
}
public ActionResult Clients(string serverId)
{
return PartialView();
}
public ActionResult GetClients(string serverId)
{
var result = RedisServerHelp.GetClients(serverId);
ViewBag.Clients = result;
return PartialView();
}
public ActionResult BaseRedisStatus(string serverId)
{
RedisServerModel model = RedisServerConfig.RedisServers.FirstOrDefault(p => p.ServerId == serverId);
var serverResponse = RedisServerHelp.GetRedisServerResponse(serverId);
ViewBag.RedisServer = model;
ViewBag.ServerResponse = serverResponse;
return PartialView();
}
#endregion 视图
#region 方法
public ActionResult GetRedisStatus(string serverId)
{
var model = RedisServerHelp.GetRedisServerResponse(serverId);
return Json(model, JsonRequestBehavior.AllowGet);
}
public ActionResult SimpleDetailsData(string serverId)
{
var result = RedisServerHelp.GetRedisInfoRow(serverId);
return Json(result, JsonRequestBehavior.AllowGet);
}
public ActionResult RichDetailsData(string serverId)
{
var result = RedisServerHelp.GetRedisInfo(serverId);
return Json(result, JsonRequestBehavior.AllowGet);
}
public ActionResult RefreshServerConfig()
{
RedisServerConfig.LoadConfig();
return Content("刷新成功");
}
#endregion 方法
}
}
RedisServiceConfig.json
[
{
"ServerHost": "127.0.0.1:6379,allowadmin=true,password=123456",
"ServerDescribe": "本地"
},
{
"ServerHost": "192.168.1.104:6379,allowadmin=true,password=123456",
"ServerDescribe": "测试环境"
},
{
"ServerHost": "192.168.1.141:6379,allowadmin=true",
"ServerDescribe": "开发环境"
}
]
_Layout.cshtml:
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>@ViewBag.Title</title>
@Styles.Render("~/Content/css")
@Scripts.Render("~/bundles/modernizr")
</head>
<body>
<div class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
@Html.ActionLink("监控列表", "Index", "Home", new { area = "" }, new { @class = "navbar-brand" })
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li>@Html.ActionLink("Info命令说明", "InfoInstructions", "Home")</li>
</ul>
</div>
</div>
</div>
<div class="container body-content">
@RenderBody()
<hr />
</div>
@Scripts.Render("~/bundles/jquery")
@Scripts.Render("~/bundles/bootstrap")
@RenderSection("scripts", required: false)
</body>
</html>
Index.cshtml:
@using RedisMonitor.Core.Model
@{
ViewBag.Title = "监控列表";
}
@{
var redisServers = ViewBag.RedisServers as List<RedisServerModel>;
}
<br />
<div class="panel panel-primary">
<div class="panel-heading">
<h3 class="panel-title">操作</h3>
</div>
<div class="panel-body" style="padding-bottom: 2px;">
<form class="form-horizontal" role="form">
<div class="form-group form-group-lg has-success has-feedback">
<label class="col-sm-2 control-label" for="select_time">刷新频率:</label>
<div class="col-sm-4">
<select class="form-control" id="select_time">
<option value="2">2s</option>
<option value="5" selected>5s</option>
<option value="20">20s</option>
<option value="40">40s</option>
<option value="60">1分钟</option>
<option value="300">5分钟</option>
<option value="600">10分钟</option>
<option value="1800">30分钟</option>
</select>
</div>
<label class="col-sm-2 control-label" for="select_time">修改了配置文件,请戳</label>
<div class="col-sm-4">
<button type="button" class="btn btn-primary" onclick="refreshList()">
<span class="glyphicon glyphicon-refresh"></span>点击刷新
</button>
</div>
</div>
</form>
</div>
</div>
<div class="panel panel-primary">
<div class="panel-heading">
<h3 class="panel-title">监控列表</h3>
</div>
<div class="panel-body">
<div class="table-responsive" style="min-height:500px;">
<table class="table table-bordered table-hover" id="table_redis">
<thead>
<tr>
<th>ServerId</th>
<th>配置地址</th>
<th style="width:10%">描述</th>
<th>状态</th>
<th>响应时间</th>
</tr>
</thead>
<tbody>
@if (redisServers != null)
{
foreach (var item in redisServers)
{
<tr class="active">
<td class="ServerId">@item.ServerId</td>
<td class="ServerHost"><a title="查看详情" target="_blank" href="/Home/Details?ServerId=@item.ServerId">@item.ServerHost</a></td>
<td class="ServerDescribe">@item.ServerDescribe</td>
<td class="Status">--</td>
<td class="ResponseTime">--</td>
</tr>
}
}
else
{
<tr>
<td>暂无配置的Redis服务器列表</td>
</tr>
}
</tbody>
</table>
</div>
</div>
</div>
@section scripts{
<script>
$(function () {
updateStatus();
});
function updateStatus() {
var $serverIds = $(".ServerId");
$serverIds.each(function () {
var $td = $(this);
$.ajax({
type: "get",
url: "/Home/GetRedisStatus",
data: {
serverId: $td.text()
},
dataType: "json",
success: function (data) {
if (data != null) {
var $tr = $td.parent();
$tr.removeClass("active");
if (data.IsConnection) {
$tr.addClass("success");
} else {
$tr.addClass("danger");
}
$td.nextAll(".Status").text(data.Status);
$td.nextAll(".ResponseTime").text(data.ResponseTime + "ms");
}
}
});
});
setTimeout(updateStatus, $("#select_time").val() * 1000);
}
function refreshList() {
$.ajax({
type: "get",
url: "/Home/RefreshServerConfig",
success: function (data) {
location.reload(true);
}
});
}
</script>
}
视图页面:
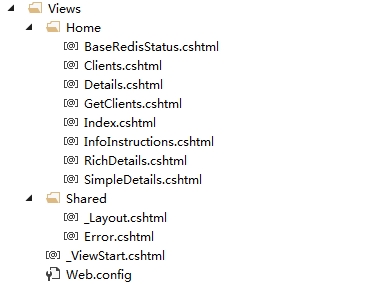
Dll:
web项目所需dll
运行结果如图:
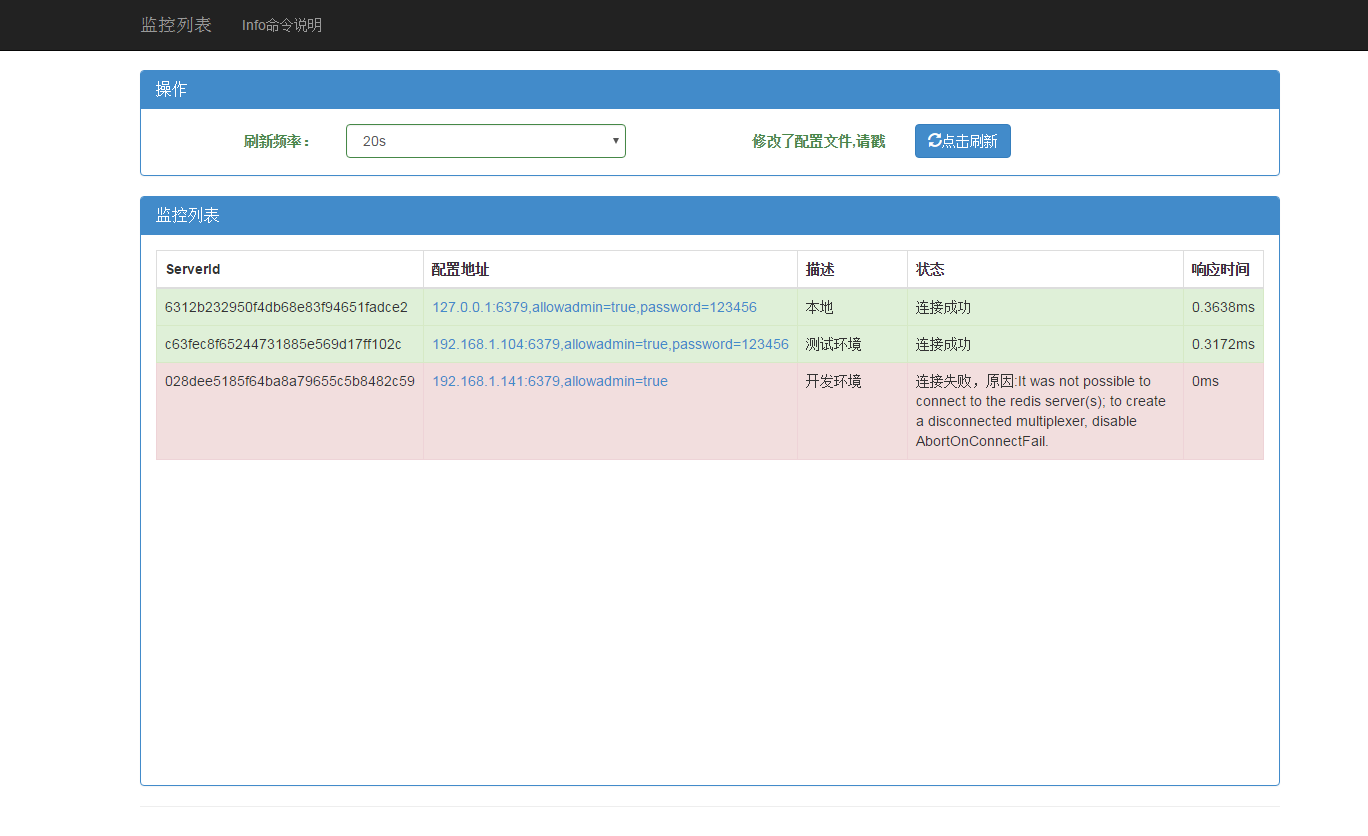
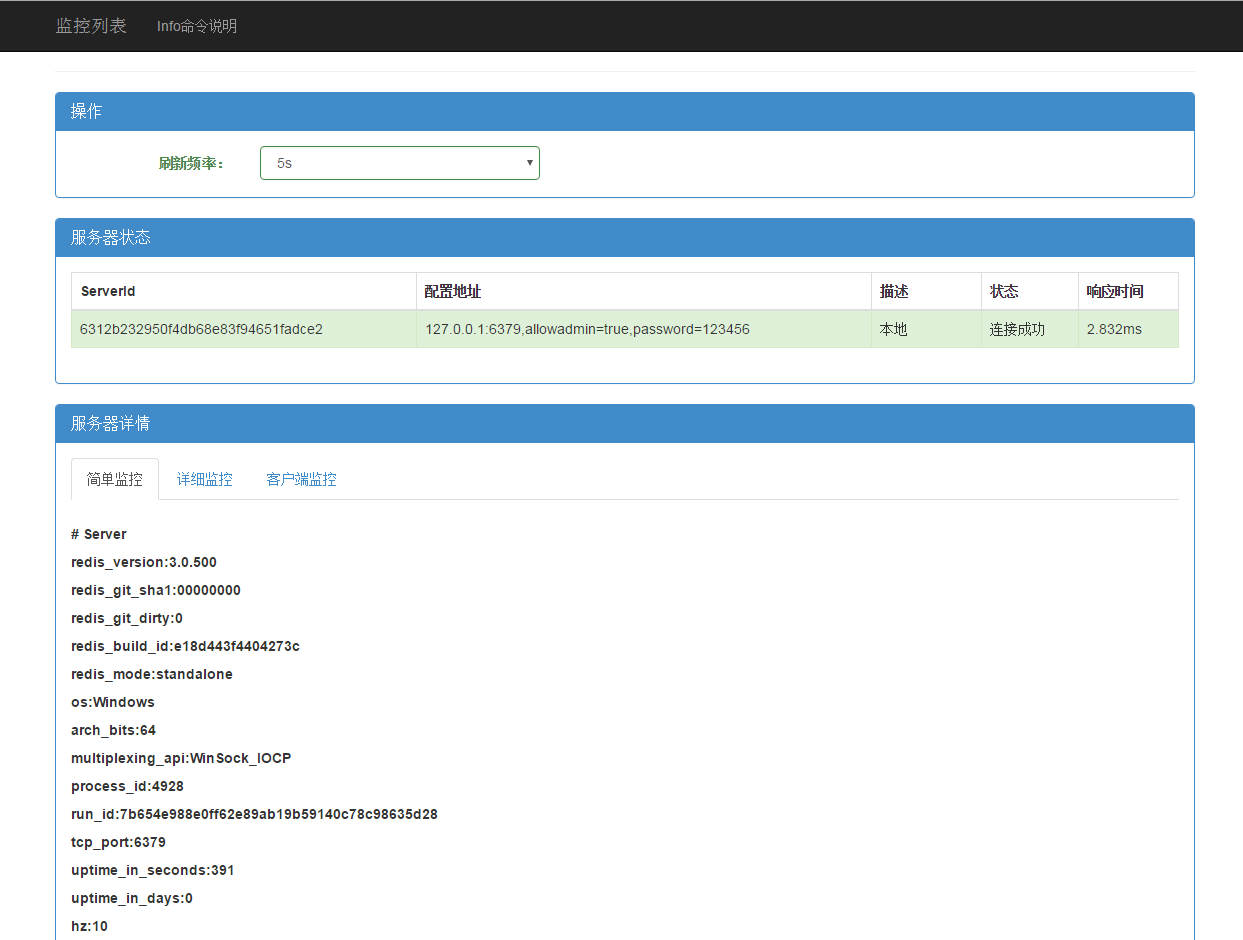
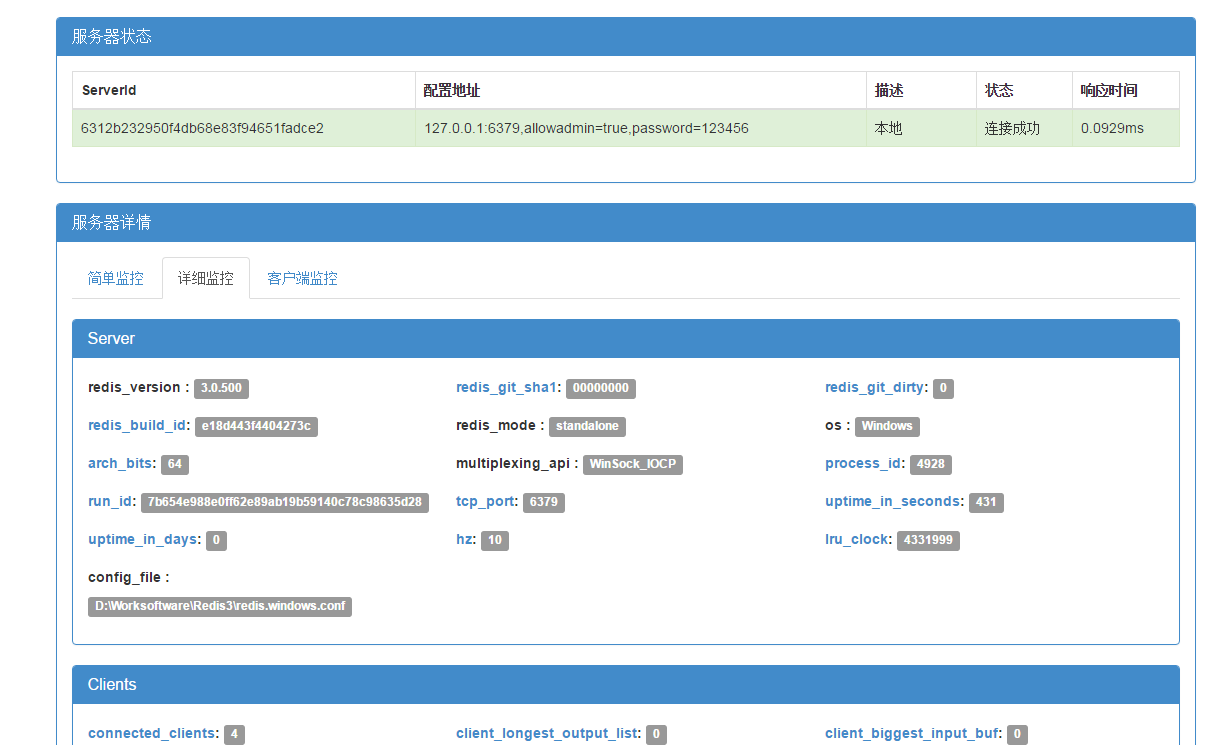
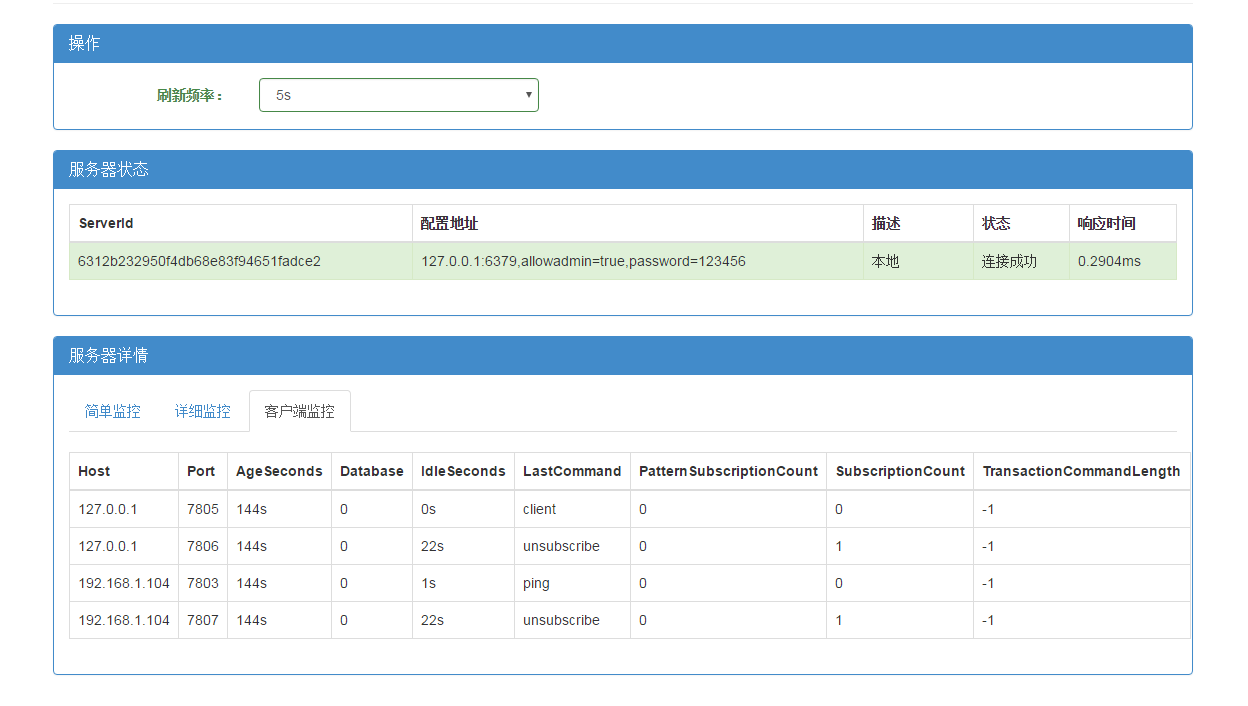