Map
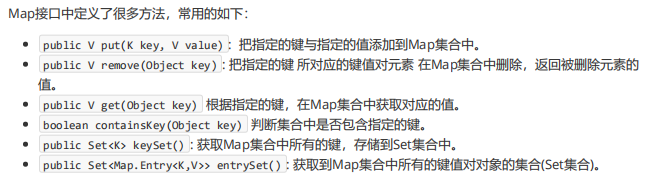
import com.xiye.bean.Student;
import com.xiye.utils.HashMapUtil;
import java.util.HashMap;
import java.util.Set;
/**
* Create by xiye on 2019/11/28 15:13
*/
public class Demo1_Map {
/*
* HashSet内部实现还是HashMap
* */
public static void main(String[] args) {
HashMap<Student, String> map = HashMapUtil.put(Student.class, String.class,
new Student("幻海", 20), "广州亨德路",
new Student("孟涛", 25), "广州德顺路",
new Student("空羽", 20), "广州天空路",
new Student("幻海", 20), "广州亨德路");
Set<Student> set = map.keySet();
System.out.println(set);
}
}
结果:[Student{name='幻海', age=20}, Student{name='空羽', age=20}, Student{name='孟涛', age=25}]
package com.xiye.bean;
import java.util.Objects;
/**
* Create by xiye on 2019/11/28 16:13
*/
public class Student {
private String name;
private Integer age;
public Student() {}
public Student(String name, Integer age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Student student = (Student) o;
return Objects.equals(name, student.name) &&
Objects.equals(age, student.age);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
备注:重写hashCode和equals是为了自定义集合去重元素规则,例如上面是名字和年龄相同即为同一个对象
package com.xiye.utils;
import java.util.HashMap;
/**
* Create by xiye on 2019/11/28 13:24
*/
public class HashMapUtil<K, V> extends HashMap<K, V> {
public static<K, V> HashMap put(Class<K> k, Class<V> v, Object... params) {
HashMap<K, V> map = new HashMap<>();
if (params.length % 2 == 0) {
for (int i = 0; i < params.length; i++) {
if (params[i].getClass().equals(k)) {
K key = (K) params[i];
if (params[++i].getClass().equals(v)) {
V value = (V) params[i];
//
map.put(key, value);
}
}
}
}
return map;
}
}
备注:自定义的工具类,指定键和值的类型,再传入偶数个参数(除一二参数,往后每两个参数是一个键值对)
异常
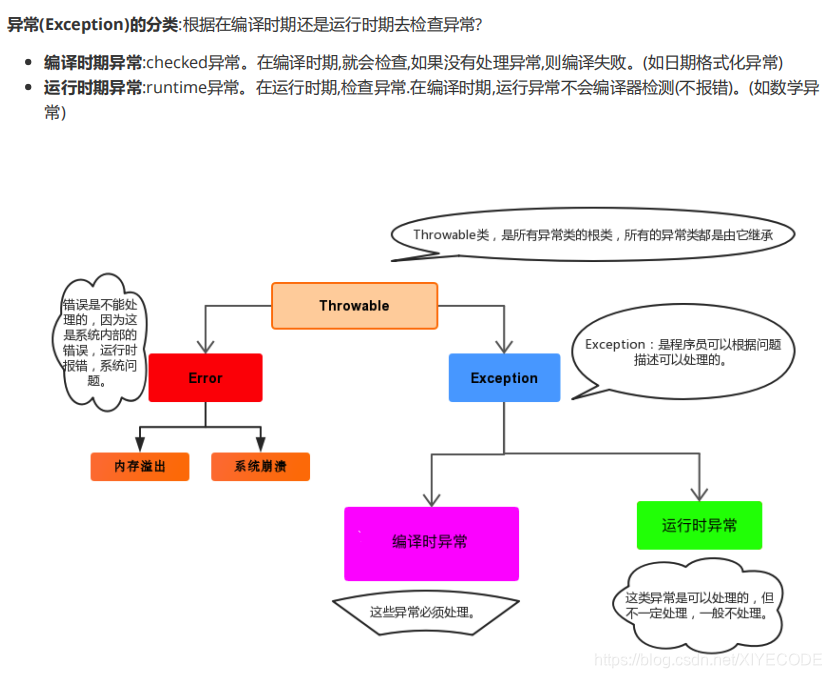
异常处理方式:
- 抛出异常throw,在方法内:throw new 异常类();
- 声明异常throws,在方法定义上: 修饰符 返回值类型 方法名(参数) throws 异常类名1,异常类名2…{ }
- 捕获异常try…catch,在方法内使用:try {//可能出现的异常或者编译异常} catch {//异常说明或者写入日志}
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
/**
* Create by xiye on 2019/11/28 16:50
*/
public class Demo2_异常 {
/*异常
* 运行时异常:声明抛出异常(throws Exception),调用时可以不做处理;或者主动抛出(throw new Exception())
*
* 编译时异常:声明抛出异常(throws Exception),调用时要做处理;或者捕获异常
*
*
* try...catch...finally:捕获编译时异常
* */
public static void main(String[] args) {
runTimeExceptionMethod();
throwMethod();
checkedExceptionMethod();
}
private static void checkedExceptionMethod() {
// 异常需要捕获,选中代码块,快捷键ctrl+alt+T选择try
try {
DateFormat df = new SimpleDateFormat("yyyy-MM-dd");
Date date = df.parse("2019-11-28");
System.out.println(date);
} catch (ParseException e) {
e.printStackTrace();
}
}
private static void runTimeExceptionMethod() {
System.out.println(1/0); // 属于运行时异常
}
private static void throwMethod() {
/*
* 如果主动抛出异常属于编译时异常,那么还要方法体之前声明异常(throws 异常类名)
*
* */
System.out.println("123");
throw new ArrayIndexOutOfBoundsException(); // 主动抛出异常
}
}