-
目录结构
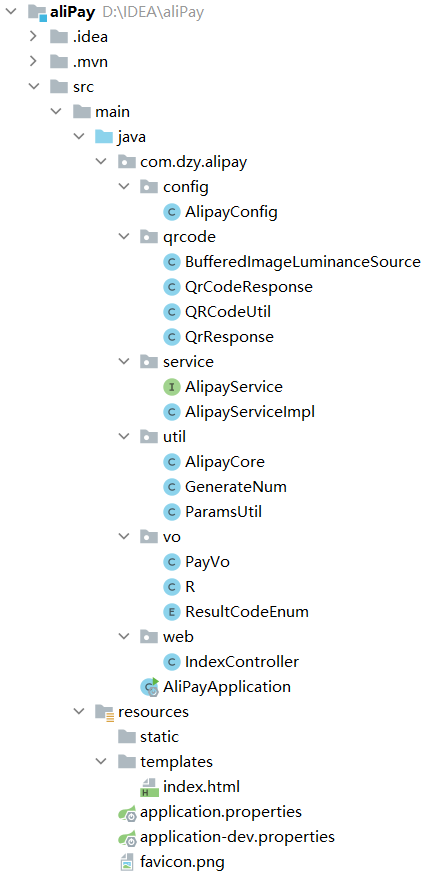
-
BufferedImageLuminanceSource.java
package com.dzy.alipay.qrcode;
import com.google.zxing.LuminanceSource;
import java.awt.*;
import java.awt.geom.AffineTransform;
import java.awt.image.BufferedImage;
public class BufferedImageLuminanceSource extends LuminanceSource {
private final BufferedImage image;
private final int left;
private final int top;
public BufferedImageLuminanceSource(BufferedImage image) {
this(image, 0, 0, image.getWidth(), image.getHeight());
}
public BufferedImageLuminanceSource(BufferedImage image, int left, int top, int width, int height) {
super(width, height);
int sourceWidth = image.getWidth();
int sourceHeight = image.getHeight();
if (left + width > sourceWidth || top + height > sourceHeight) {
throw new IllegalArgumentException("Crop rectangle does not fit within image data.");
}
for (int y = top; y < top + height; y++) {
for (int x = left; x < left + width; x++) {
if ((image.getRGB(x, y) & 0xFF000000) == 0) {
image.setRGB(x, y, 0xFFFFFFFF);
}
}
}
this.image = new BufferedImage(sourceWidth, sourceHeight, BufferedImage.TYPE_BYTE_GRAY);
this.image.getGraphics().drawImage(image, 0, 0, null);
this.left = left;
this.top = top;
}
public byte[] getRow(int y, byte[] row) {
if (y < 0 || y >= getHeight()) {
throw new IllegalArgumentException("Requested row is outside the image: " + y);
}
int width = getWidth();
if (row == null || row.length < width) {
row = new byte[width];
}
image.getRaster().getDataElements(left, top + y, width, 1, row);
return row;
}
public byte[] getMatrix() {
int width = getWidth();
int height = getHeight();
int area = width * height;
byte[] matrix = new byte[area];
image.getRaster().getDataElements(left, top, width, height, matrix);
return matrix;
}
public boolean isCropSupported() {
return true;
}
public LuminanceSource crop(int left, int top, int width, int height) {
return new BufferedImageLuminanceSource(image, this.left + left, this.top + top, width, height);
}
public boolean isRotateSupported() {
return true;
}
public LuminanceSource rotateCounterClockwise() {
int sourceWidth = image.getWidth();
int sourceHeight = image.getHeight();
AffineTransform transform = new AffineTransform(0.0, -1.0, 1.0, 0.0, 0.0, sourceWidth);
BufferedImage rotatedImage = new BufferedImage(sourceHeight, sourceWidth, BufferedImage.TYPE_BYTE_GRAY);
Graphics2D g = rotatedImage.createGraphics();
g.drawImage(image, transform, null);
g.dispose();
int width = getWidth();
return new BufferedImageLuminanceSource(rotatedImage, top, sourceWidth - (left + width), getHeight(), width);
}
}
-
QrCodeResponse.java
package com.dzy.alipay.qrcode;
public class QrCodeResponse {
private String code;
private String msg;
private String out_trade_no;
private String qr_code;
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
public String getOut_trade_no() {
return out_trade_no;
}
public void setOut_trade_no(String out_trade_no) {
this.out_trade_no = out_trade_no;
}
public String getQr_code() {
return qr_code;
}
public void setQr_code(String qr_code) {
this.qr_code = qr_code;
}
@Override
public String toString() {
return "QrCodeResponse{" +
"code='" + code + '\'' +
", msg='" + msg + '\'' +
", out_trade_no='" + out_trade_no + '\'' +
", qr_code='" + qr_code + '\'' +
'}';
}
}
-
QRCodeUtil.java
package com.dzy.alipay.qrcode;
import com.google.zxing.*;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.common.HybridBinarizer;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import org.springframework.util.ResourceUtils;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.geom.RoundRectangle2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.OutputStream;
import java.util.Hashtable;
public class QRCodeUtil {
private static final String CHARSET = "utf-8";
private static final String FORMAT_NAME = "JPG";
private static final int QRCODE_SIZE = 300;
private static final int WIDTH = 90;
private static final int HEIGHT = 90;
private static BufferedImage createImage(String content, String imgPath, boolean needCompress) throws Exception {
Hashtable hints = new Hashtable();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET, CHARSET);
hints.put(EncodeHintType.MARGIN, 1);
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, QRCODE_SIZE, QRCODE_SIZE,
hints);
int width = bitMatrix.getWidth();
int height = bitMatrix.getHeight();
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, bitMatrix.get(x, y) ? 0xFF000000 : 0xFFFFFFFF);
}
}
if (imgPath == null || "".equals(imgPath)) {
return image;
}
QRCodeUtil.insertImage(image, imgPath, needCompress);
return image;
}
private static void insertImage(BufferedImage source, String imgPath, boolean needCompress) throws Exception {
File file = new File(imgPath);
if (!file.exists()) {
System.err.println("" + imgPath + " 该文件不存在!");
return;
}
Image src = ImageIO.read(new File(imgPath));
int width = src.getWidth(null);
int height = src.getHeight(null);
if (needCompress) {
if (width > WIDTH) {
width = WIDTH;
}
if (height > HEIGHT) {
height = HEIGHT;
}
Image image = src.getScaledInstance(width, height, Image.SCALE_SMOOTH);
BufferedImage tag = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics g = tag.getGraphics();
g.drawImage(image, 0, 0, null);
g.dispose();
src = image;
}
Graphics2D graph = source.createGraphics();
int x = (QRCODE_SIZE - width) / 2;
int y = (QRCODE_SIZE - height) / 2;
graph.drawImage(src, x, y, width, height, null);
Shape shape = new RoundRectangle2D.Float(x, y, width, width, 6, 6);
graph.setStroke(new BasicStroke(3f));
graph.draw(shape);
graph.dispose();
}
public static void encode(String content, String imgPath, String destPath, boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
mkdirs(destPath);
ImageIO.write(image, FORMAT_NAME, new File(destPath));
}
public static BufferedImage encode(String content, String imgPath, boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
return image;
}
public static void mkdirs(String destPath) {
File file = new File(destPath);
if (!file.exists() && !file.isDirectory()) {
file.mkdirs();
}
}
public static void encode(String content, String imgPath, String destPath) throws Exception {
QRCodeUtil.encode(content, imgPath, destPath, false);
}
public static void encode(String content, String destPath) throws Exception {
QRCodeUtil.encode(content, null, destPath, false);
}
public static void encode(String content, String imgPath, OutputStream output, boolean needCompress)
throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath, needCompress);
ImageIO.write(image, FORMAT_NAME, output);
}
public static void encode(String content, OutputStream output) throws Exception {
QRCodeUtil.encode(content, null, output, false);
}
public static String decode(File file) throws Exception {
BufferedImage image;
image = ImageIO.read(file);
if (image == null) {
return null;
}
BufferedImageLuminanceSource source = new BufferedImageLuminanceSource(image);
BinaryBitmap bitmap = new BinaryBitmap(new HybridBinarizer(source));
Result result;
Hashtable hints = new Hashtable();
hints.put(DecodeHintType.CHARACTER_SET, CHARSET);
result = new MultiFormatReader().decode(bitmap, hints);
String resultStr = result.getText();
return resultStr;
}
public static String decode(String path) throws Exception {
return QRCodeUtil.decode(new File(path));
}
}
-
QrResponse.java
package com.dzy.alipay.qrcode;
public class QrResponse {
private QrCodeResponse alipay_trade_precreate_response;
private String sign;
public QrCodeResponse getAlipay_trade_precreate_response() {
return alipay_trade_precreate_response;
}
public void setAlipay_trade_precreate_response(QrCodeResponse alipay_trade_precreate_response) {
this.alipay_trade_precreate_response = alipay_trade_precreate_response;
}
public String getSign() {
return sign;
}
public void setSign(String sign) {
this.sign = sign;
}
}
-
AlipayService.java
package com.dzy.alipay.service;
import com.dzy.alipay.vo.PayVo;
public interface AlipayService {
byte[] alipay(PayVo payVo);
}
-
PayVo.java
package com.dzy.alipay.vo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.ToString;
@Data
@NoArgsConstructor
@AllArgsConstructor
@ToString
public class PayVo implements java.io.Serializable{
private String userId;
private String courseid;
}
-
R.java
package com.dzy.alipay.vo;
import lombok.Data;
import java.util.HashMap;
import java.util.Map;
@Data
public class R {
private Boolean success;
private Integer code;
private String message;
private Map<String, Object> data = new HashMap<String, Object>();
private R(){}
public static R ok(){
R r = new R();
r.setSuccess(ResultCodeEnum.SUCCESS.getSuccess());
r.setCode(ResultCodeEnum.SUCCESS.getCode());
r.setMessage(ResultCodeEnum.SUCCESS.getMessage());
return r;
}
public static R error(){
R r = new R();
r.setSuccess(ResultCodeEnum.UNKNOWN_REASON.getSuccess());
r.setCode(ResultCodeEnum.UNKNOWN_REASON.getCode());
r.setMessage(ResultCodeEnum.UNKNOWN_REASON.getMessage());
return r;
}
public static R setResult(ResultCodeEnum resultCodeEnum){
R r = new R();
r.setSuccess(resultCodeEnum.getSuccess());
r.setCode(resultCodeEnum.getCode());
r.setMessage(resultCodeEnum.getMessage());
return r;
}
public R success(Boolean success){
this.setSuccess(success);
return this;
}
public R message(String message){
this.setMessage(message);
return this;
}
public R code(Integer code){
this.setCode(code);
return this;
}
public R data(String key, Object value){
this.data.put(key, value);
return this;
}
public R data(Map<String, Object> map){
this.setData(map);
return this;
}
}
-
ResultCodeEnum.java
package com.dzy.alipay.vo;
import lombok.Getter;
@Getter
public enum ResultCodeEnum {
SUCCESS(true, 20000,"成功"),
UNKNOWN_REASON(false, 20001, "未知错误"),
LOGIN_PHONE_ERRROR(false, 20002, "手机号码不能为空"),
ACCOUNT_PHONE_ERRROR(false, 20002, "账号信息不能为空"),
LOGIN_PHONE_PATTARN_ERRROR(false, 20003, "手机号码格式不正确"),
VALIDATION_CODE_ERROR(false, 20004, "验证码不正确"),
LOGIN_CODE_ERROR(false, 20005, "短信验证码不能为空"),
LOGIN_CAPATA_ERROR(false, 20006, "图形验证码不能为空"),
LOGIN_CODE_FAIL_ERROR(false, 20007, "短信验证码失效,请重新发送"),
LOGIN_CODE_INPUT_ERROR(false, 20008, "输入的短信码有误"),
PHONE_ERROR_MSG(false, 20009, "该手机号未绑定账户"),
USER_FORBIDDEN(false, 20010, "该用户已被禁用,请联系平台客服"),
LOGIN_PWD_ERROR(false, 200011, "密码不允许为空"),
LOGIN_PWD_INPUT_ERROR(false, 200012, "密码输入有误"),
LOGIN_PWD_NO_INPUT_ERROR(false, 200013, "检测到没有完善密码信息"),
BAD_SQL_GRAMMAR(false, 21001, "sql语法错误"),
JSON_PARSE_ERROR(false, 21002, "json解析异常"),
PARAM_ERROR(false, 21003, "参数不正确"),
USER_PWD_ERROR(false, 21003, "尚未找到对应的用户信息");
private Boolean success;
private Integer code;
private String message;
private ResultCodeEnum(Boolean success, Integer code, String message) {
this.success = success;
this.code = code;
this.message = message;
}
}
-
AlipayServiceImpl.java
package com.dzy.alipay.service;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.alipay.api.AlipayApiException;
import com.alipay.api.AlipayClient;
import com.alipay.api.DefaultAlipayClient;
import com.alipay.api.domain.AlipayTradePrecreateModel;
import com.alipay.api.request.AlipayTradePrecreateRequest;
import com.alipay.api.response.AlipayTradePrecreateResponse;
import com.dzy.alipay.config.AlipayConfig;
import com.dzy.alipay.qrcode.QRCodeUtil;
import com.dzy.alipay.qrcode.QrCodeResponse;
import com.dzy.alipay.qrcode.QrResponse;
import com.dzy.alipay.util.GenerateNum;
import com.dzy.alipay.vo.PayVo;
import lombok.extern.log4j.Log4j2;
import org.springframework.stereotype.Service;
import org.springframework.util.FileCopyUtils;
import org.springframework.util.ResourceUtils;
import javax.imageio.ImageIO;
import javax.imageio.stream.ImageOutputStream;
import java.awt.image.BufferedImage;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
@Service
@Log4j2
public class AlipayServiceImpl implements AlipayService {
private final AlipayConfig alipayConfig;
public AlipayServiceImpl(AlipayConfig alipayConfig) {
this.alipayConfig = alipayConfig;
}
@Override
public byte[] alipay(PayVo payVo) {
try {
String userId = payVo.getUserId();
String money = "1";
String title = "java面向对象";
String orderNumber = GenerateNum.generateOrder();
JSONObject json = new JSONObject();
json.put("userId", userId);
json.put("orderNumber", orderNumber);
json.put("money", money);
String params = json.toString();
AlipayTradePrecreateModel model = new AlipayTradePrecreateModel();
model.setOutTradeNo(orderNumber);
model.setTotalAmount(money);
model.setSubject(title);
model.setBody(params);
model.setTimeoutExpress("30m");
model.setStoreId(userId+"");
QrCodeResponse qrCodeResponse = qrcodePay(model);
ByteArrayOutputStream output = new ByteArrayOutputStream();
String logoPath = ResourceUtils.getFile("classpath:favicon.png").getAbsolutePath();
BufferedImage buffImg = QRCodeUtil.encode(qrCodeResponse.getQr_code(), logoPath, false);
ImageOutputStream imageOut = ImageIO.createImageOutputStream(output);
ImageIO.write(buffImg, "JPEG", imageOut);
imageOut.close();
ByteArrayInputStream input = new ByteArrayInputStream(output.toByteArray());
return FileCopyUtils.copyToByteArray(input);
} catch (Exception ex) {
ex.printStackTrace();
return null;
}
}
public QrCodeResponse qrcodePay(AlipayTradePrecreateModel model) {
AlipayClient alipayClient = getAlipayClient();
AlipayTradePrecreateRequest request = new AlipayTradePrecreateRequest();
request.setBizModel(model);
request.setNotifyUrl(alipayConfig.getNotify_url());
request.setReturnUrl(alipayConfig.getReturn_url());
AlipayTradePrecreateResponse alipayTradePrecreateResponse = null;
try {
alipayTradePrecreateResponse = alipayClient.execute(request);
} catch (AlipayApiException e) {
e.printStackTrace();
}
assert alipayTradePrecreateResponse != null;
QrResponse qrResponse = JSON.parseObject(alipayTradePrecreateResponse.getBody(), QrResponse.class);
return qrResponse.getAlipay_trade_precreate_response();
}
private AlipayClient getAlipayClient() {
return new DefaultAlipayClient(alipayConfig.getGatewayUrl(), alipayConfig.getApp_id(), alipayConfig.getMerchant_private_key(), "JSON", alipayConfig.getCharset(), alipayConfig.getAlipay_public_key(), alipayConfig.getSign_type());
}
}
-
AlipayCore.java
package com.dzy.alipay.util;
import org.apache.commons.codec.digest.DigestUtils;
import org.apache.commons.httpclient.methods.multipart.FilePartSource;
import org.apache.commons.httpclient.methods.multipart.PartSource;
import java.io.File;
import java.io.IOException;
import java.util.*;
public class AlipayCore {
public static Map<String, String> paraFilter(Map<String, String> sArray) {
Map<String, String> result = new HashMap<String, String>();
if (sArray == null || sArray.size() <= 0) {
return result;
}
for (String key : sArray.keySet()) {
String value = sArray.get(key);
if (value == null || value.equals("") || key.equalsIgnoreCase("sign")
|| key.equalsIgnoreCase("sign_type")) {
continue;
}
result.put(key, value);
}
return result;
}
public static String createLinkString(Map<String, String> params) {
List<String> keys = new ArrayList<String>(params.keySet());
Collections.sort(keys);
String prestr = "";
for (int i = 0; i < keys.size(); i++) {
String key = keys.get(i);
String value = params.get(key);
if (i == keys.size() - 1) {
prestr = prestr + key + "=" + value;
} else {
prestr = prestr + key + "=" + value + "&";
}
}
return prestr;
}
public static String getAbstract(String strFilePath, String file_digest_type) throws IOException {
PartSource file = new FilePartSource(new File(strFilePath));
if (file_digest_type.equals("MD5")) {
return DigestUtils.md5Hex(file.createInputStream());
} else if (file_digest_type.equals("SHA")) {
return DigestUtils.sha256Hex(file.createInputStream());
} else {
return "";
}
}
}
-
GenerateNum.java
package com.dzy.alipay.util;
import java.text.SimpleDateFormat;
import java.util.Date;
public class GenerateNum {
private static int count = 0;
private static final int total = 99;
private static final SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMddHHmmss");
private static String getNowDateStr() {
return sdf.format(new Date());
}
private static String now = null;
public static String generateOrder() {
String datastr = getNowDateStr();
if (datastr.equals(now)) {
count++;
} else {
count = 1;
now = datastr;
}
int countInteger = String.valueOf(total).length() - String.valueOf(count).length();
String bu = "";
for (int i = 0; i < countInteger; i++) {
bu += "0";
}
bu += String.valueOf(count);
if (count >= total) {
count = 0;
}
return datastr + bu;
}
}
-
ParamsUtil.java
package com.dzy.alipay.util;
import javax.servlet.http.HttpServletRequest;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class ParamsUtil {
public static Map<String, String> ParamstoMap(HttpServletRequest request) {
Map<String, String> params = new HashMap<String, String>();
Map<String, String[]> requestParams = request.getParameterMap();
for (Iterator<String> iter = requestParams.keySet().iterator(); iter.hasNext();) {
String name = (String) iter.next();
String[] values = (String[]) requestParams.get(name);
String valueStr = "";
for (int i = 0; i < values.length; i++) {
valueStr = (i == values.length - 1) ? valueStr + values[i] : valueStr + values[i] + ",";
}
params.put(name, valueStr);
}
return params;
}
}