C++下OpenCV学习笔记
----Mat类数据结构
一.简介
Mat不仅是非常有用的图像容器类,也是一个通用的矩阵类。
- attention
(1)不必再手动为Mat类开辟空间
(2)不必在不需要时立即释放空间 - 组成
(1)矩阵头:包含矩阵尺寸、存储方法、存储地址等信息
(2)一个指向存储所有像素值的矩阵的指针。其中,根据所选的存储方法的不同,矩阵可以是不同的维数。 - 矩阵复制
(1)浅拷贝:=和()
引用计数机制,即让每个Mat对象拥有不同的信息头,但共享同一矩阵。通过让矩阵指针指向同一地址实现。使用拷贝构造函数时只复制信息头和矩阵指针,而不复制矩阵。
1>代码实现
#include<opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat a, c; //仅创建信息头
a = imread("C:\\Users\\441\\Desktop\\ZL\\夏目\\1.jpg"); //为矩阵开辟内存
Mat b(a); //使用拷贝构造函数
c = a; //使用赋值运算符
imshow("a", a);
imshow("b", b);
imshow("c", c);
waitKey(0);
return 0;
}
2>运行结果
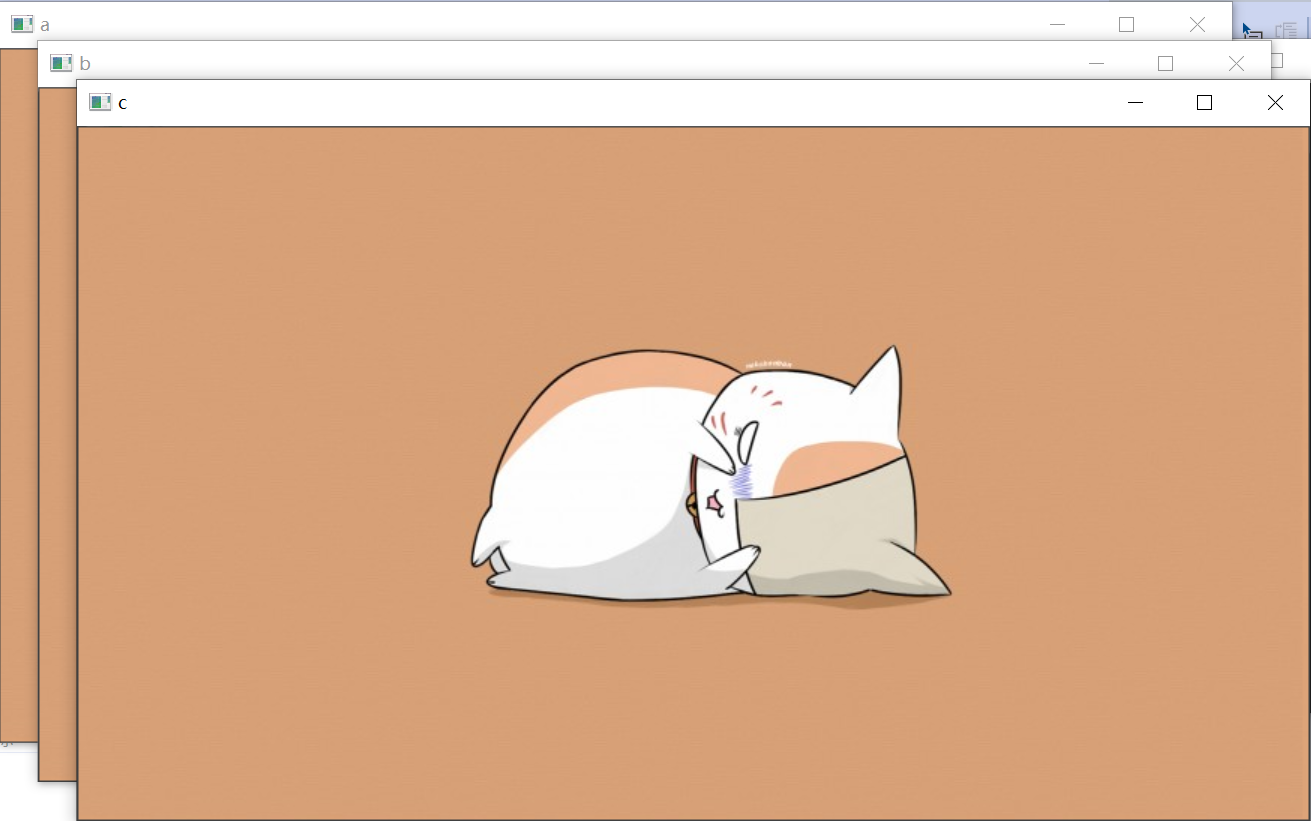
3>attention
通过对任何一个对象作出改变也会影响其他对象。
(2)创建只引用部分数据的信息头(以创建感兴趣区域ROI为例)
a.使用矩阵界定
1>代码实现
#include<opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat a;
a = imread("C:\\Users\\441\\Desktop\\ZL\\夏目\\1.jpg");
Mat b(a, Rect(250, 125, 250, 150));
imshow("a", a);
imshow("b", b);
waitKey(0);
return 0;
}
2>运行结果
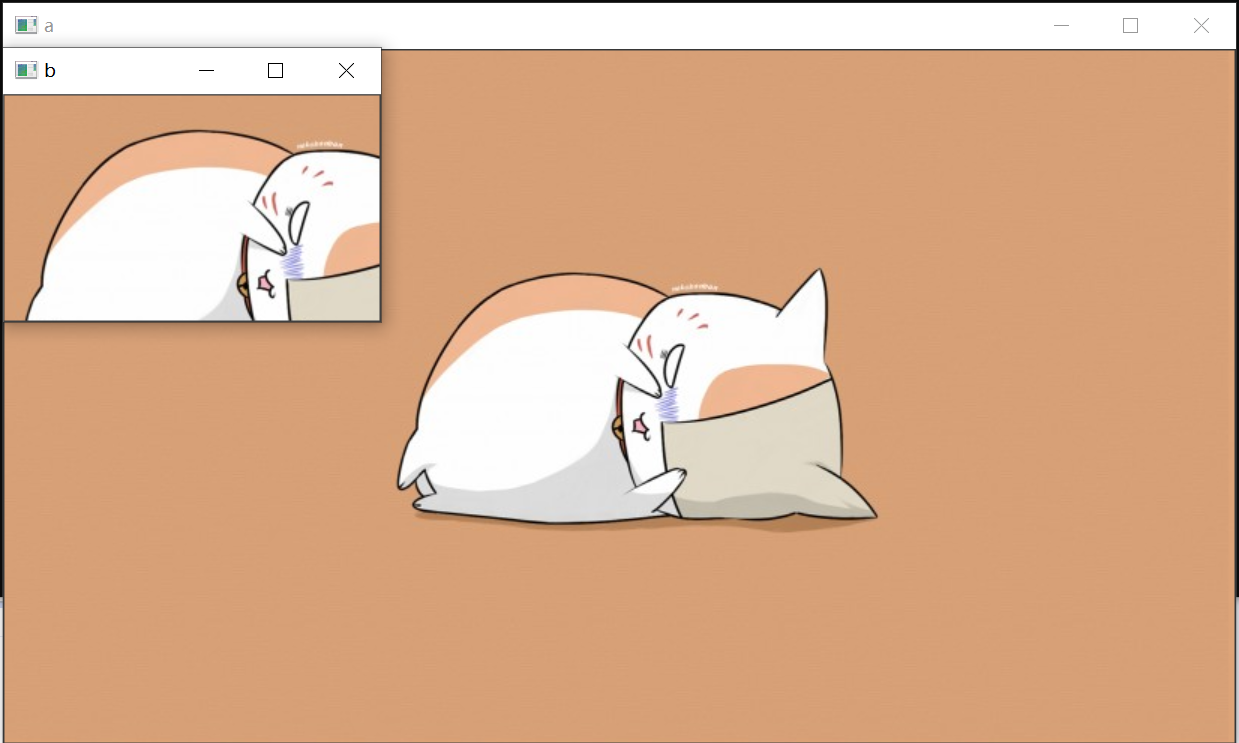
b.用行和列界定
1>代码实现
#include<opencv2/opencv.hpp>
using namespace cv;
int main()
{
Mat a;
a = imread("C:\\Users\\441\\Desktop\\ZL\\夏目\\1.jpg");
Mat b = a(Range(125, 275), Range::all());
imshow("a", a);
imshow