模板代码:
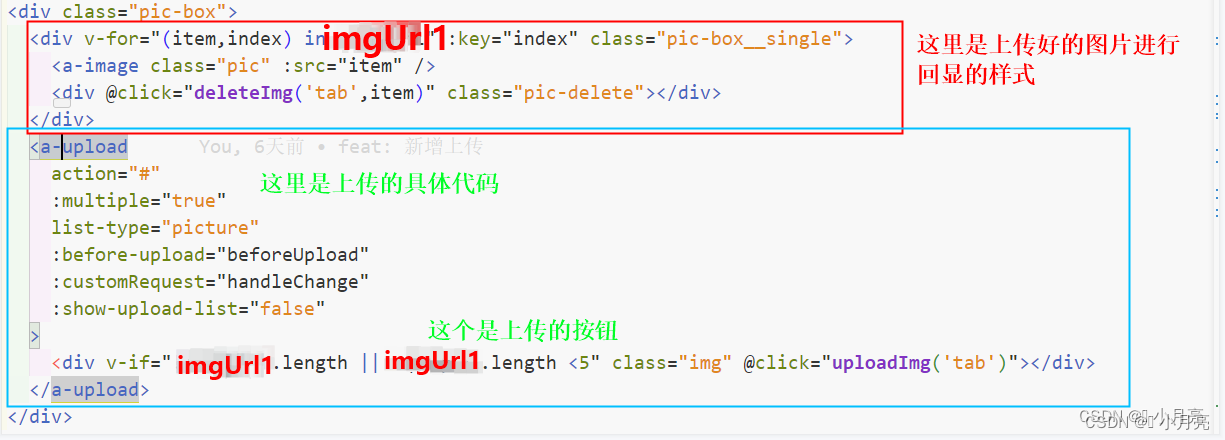
定义变量:
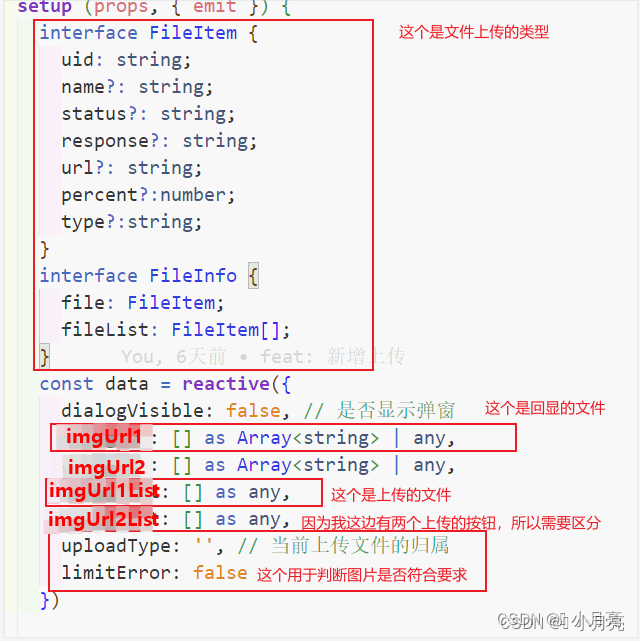
文件限制的函数:

上传的函数:
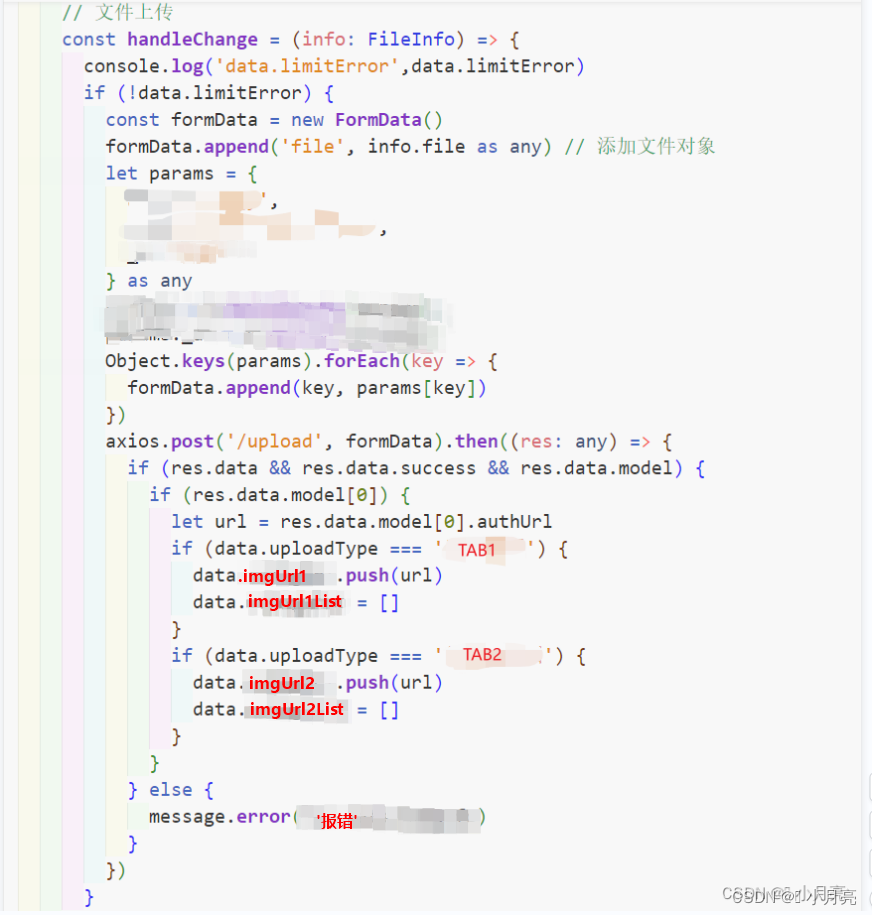
样式函数:
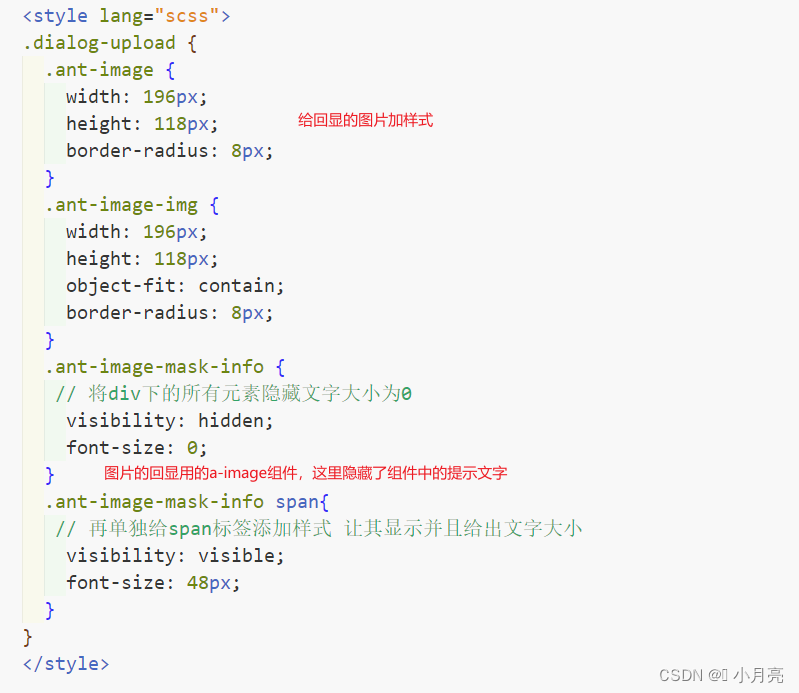
完整代码:
<template>
<div class="dialog-upload" v-if="showUploadDialog">
<div class="dialog-upload__head">
<div class="title">上传图片</div>
<div class="close" @click="closeDialog"></div>
</div>
<div class="dialog-upload__body">
<div class="upload-box">
<span class="text">tab:</span>
<div class="pic-box">
<div v-for="(item,index) in imgUrl1" :key="index" class="pic-box__single">
<a-image class="pic" :src="item" />
<div @click="deleteImg('tab',item)" class="pic-delete"></div>
</div>
<a-upload
action="#"
:multiple="true"
list-type="picture"
:before-upload="beforeUpload"
:customRequest="handleChange"
:show-upload-list="false"
>
<div v-if="!imgUrl1.length || imgUrl1.length <5" class="img" @click="uploadImg('tab')"></div>
</a-upload>
</div>
</div>
<div class="upload-box">
<span class="text">tab1:</span>
<div class="pic-box">
<div v-for="(item,index) in imgUrl2" :key="index" class="pic-box__single">
<a-image class="pic" :src="item" />
<div @click="deleteImg('tab1',item)" class="pic-delete"></div>
</div>
<a-upload
action="#"
:multiple="true"
list-type="picture"
:before-upload="beforeUpload"
:customRequest="handleChange"
:show-upload-list="false"
>
<div v-if="!imgUrl2.length || imgUrl2.length <5" class="img" @click="uploadImg('tab1')"></div>
</a-upload>
</div>
</div>
</div>
<div class="dialog-upload__foot">
<span class="sure" @click="sure">确定</span>
<span class="cancle" @click="closeDialog">取消</span>
</div>
</div>
</template>
<script lang="ts">
import {
defineComponent, reactive, toRefs, watch, nextTick } from 'vue'
import {
message } from 'ant-design-vue'
import request from '@/request/request'
import axios from 'axios'
export default defineComponent