//gcc aes_128.c -lssl -lcrypto
#include <string.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <openssl/evp.h>
#include <openssl/bio.h>
#include <openssl/buffer.h>
#include <openssl/aes.h>
int openssl_base64_encode(const unsigned char* in, int inlen, char* out, int* outlen)
{
BIO* b64 = BIO_new(BIO_f_base64());
BIO* bmem = BIO_new(BIO_s_mem());
if (!b64 || !bmem) {
fprintf(stderr, "fail to BIO_new\n");
return -1;
}
b64 = BIO_push(b64, bmem);
BIO_set_flags(b64, BIO_FLAGS_BASE64_NO_NL); // ignore newlines, write everything in one line
*outlen = BIO_write(b64, in, inlen);
if (*outlen <= 0 || *outlen != inlen) {
fprintf(stderr, "fail to BIO_write\n");
return -1;
}
BIO_flush(b64);
BUF_MEM* buf = NULL;
BIO_get_mem_ptr(b64, &buf);
*outlen = buf->length;
memcpy(out, buf->data, *outlen);
BIO_free_all(b64);
return 0;
}
int openssl_base64_decode(const char* in, int inlen, unsigned char* out, int* outlen)
{
BIO* b64 = BIO_new(BIO_f_base64());
BIO* bmem = BIO_new_mem_buf(in, inlen);
if (!b64 || !bmem) {
fprintf(stderr, "fail to BIO_new\n");
return -1;
}
b64 = BIO_push(b64, bmem);
BIO_set_flags(b64, BIO_FLAGS_BASE64_NO_NL); // ignore newlines, write everything in one line
*outlen = BIO_read(b64, out, inlen);
if (*outlen <= 0) {
fprintf(stderr, "fail to BIO_read\n");
return -1;
}
BIO_free_all(b64);
return 0;
}
int aes_encrypt(char* in, char* key, char* out)
{
if (!in || !key || !out)
{
return 0;
}
AES_KEY aes;
if (AES_set_encrypt_key((unsigned char*)key, 128, &aes) < 0)
{
return 0;
}
int len = strlen(in), en_len = 0;
while (en_len < len)
{
AES_encrypt((unsigned char*)in, (unsigned char*)out, &aes);
in += AES_BLOCK_SIZE;
out += AES_BLOCK_SIZE;
en_len += AES_BLOCK_SIZE;
}
return 1;
}
int aes_decrypt(char* in, char* key, char* out)
{
if (!in || !key || !out)
{
return 0;
}
AES_KEY aes;
if (AES_set_decrypt_key((unsigned char*)key, 128, &aes) < 0)
{
return 0;
}
int len = strlen(in), en_len = 0;
while (en_len < len)
{
AES_decrypt((unsigned char*)in, (unsigned char*)out, &aes);
in += AES_BLOCK_SIZE;
out += AES_BLOCK_SIZE;
en_len += AES_BLOCK_SIZE;
}
return 1;
}
int main()
{
//秘钥长度16位,最多一次转字符长度512个字节
char *data="116.375309";
char *key = "ABCD1234ABCD1234";
char encrypt_buffer[2048] = {'\0'}, decrypt_buffer[2048] = {'\0'};
char enbase64[256] = {'\0'};
char debase64[256] = {'\0'};
int len = 0;
len = strlen(data);
printf("明文长度:%d\n", len);
time_t start_time = time(NULL);
int count = 100000;
int count_p = 100000;
while(count--){
aes_encrypt(data, key, encrypt_buffer);
openssl_base64_encode(encrypt_buffer, strlen(encrypt_buffer), enbase64,&len);
openssl_base64_decode(enbase64, strlen(enbase64), debase64,&len);
aes_decrypt(debase64, key, decrypt_buffer);
printf("value:%s\n", decrypt_buffer);
}
time_t end_time = time(NULL);
printf("aes_128; %d字节;循环%d次, %d秒\n",len, count_p,(end_time-start_time));
return 0;
}
aes_128加密使用+base64编码(linux c语言)
最新推荐文章于 2024-05-19 19:23:18 发布
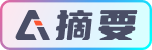