简单工厂模式(Static Factory Method)
简单工厂其实不是一个设计模式,反而比较像是一种编程习惯。但经常被使用,可以将客户程序从具体类解耦,所以就拿它来做一个设计模式的入门吧!
简单工厂模式是属于创建型模式,又叫做静态工厂方法(Static Factory Method)模式,但不属于23种GOF设计模式之一。简单工厂模式是由一个工厂对象决定创建出哪一种产品类的实例。简单工厂模式是工厂模式家族中最简单实用的模式,可以理解为是不同工厂模式的一个特殊实现。
代码
我们来实现一个简单的计算器功能来阐述简单工厂模式:
1.首先我们先定义一个运算符的基类,代码如下:
Opertaion运算类
using System;
namespace StaticFactoryMethod
{
public class Operation
{
//数值1
private double numberA=0;
//数值2
private double numberB = 0;
public double NumberA
{
get { return numberA; }
set { numberA = value; }
}
public double NumberB
{
get { return numberB; }
set { numberB = value; }
}
//计算结果
public virtual double GetResult()
{
double result = 0;
return result;
}
}
}
2.实现具体的加减乘除类,代码如下:
加法类
using System;
namespace StaticFactoryMethod
{
public class OperationAdd:Operation
{
public override double GetResult()
{
double result = 0;
result = NumberA + NumberB;
return result;
}
}
}
减法类
using System;
namespace StaticFactoryMethod
{
public class OperationSub:Operation
{
public override double GetResult()
{
double result = 0;
result = NumberA - NumberB;
return result;
}
}
}
乘法类
using System;
namespace StaticFactoryMethod
{
public class OperationMul:Operation
{
public override double GetResult()
{
double result = 0;
result = NumberA * NumberB;
return result;
}
}
}
除法类
using System;
namespace StaticFactoryMethod
{
public class OperationDiv:Operation
{
public override double GetResult()
{
double result = 0;
if (NumberB == 0)
throw new Exception ("除数不能为0。");
result = NumberA / NumberB;
return result;
}
}
}
3.简单运算工厂类,代码如下:
简单运算工厂类
using System;
namespace StaticFactoryMethod
{
public class OperationFactory
{
//根据具体的运算符创建相应的类的实例
public static Operation CreateOperate(String operate)
{
Operation operation=null;
switch (operate) {
case "+":
operation = new OperationAdd ();
break;
case "-":
operation = new OperationSub ();
break;
case "*":
operation = new OperationMul ();
break;
case "/":
operation = new OperationDiv ();
break;
}
return operation;
}
}
}
4.客户端代码,代码如下:
客户端代码
using System;
namespace StaticFactoryMethod
{
public class Program
{
static void Main(string[] args)
{
Operation opertaion;
opertaion = OperationFactory.CreateOperate ("+");
opertaion.NumberA = 1;
opertaion.NumberB = 2;
double result = opertaion.GetResult ();
Console.WriteLine (result);
}
}
}
运行结果
UML图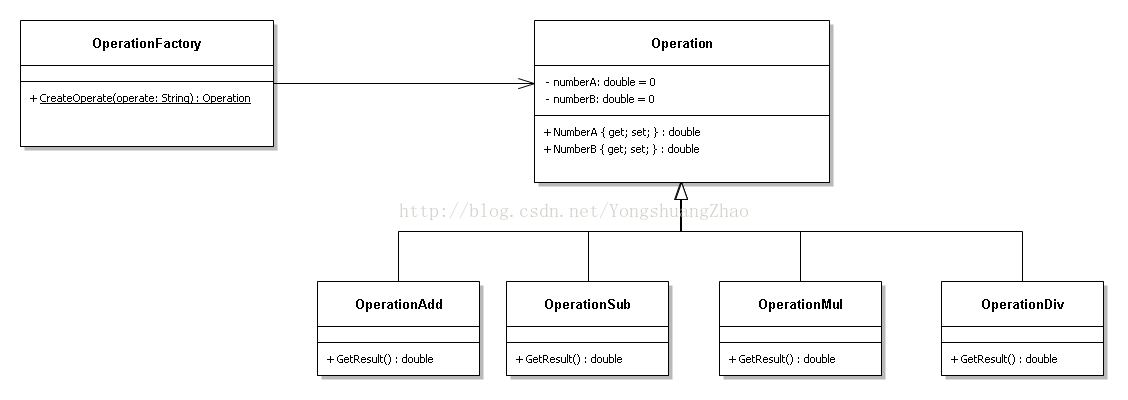
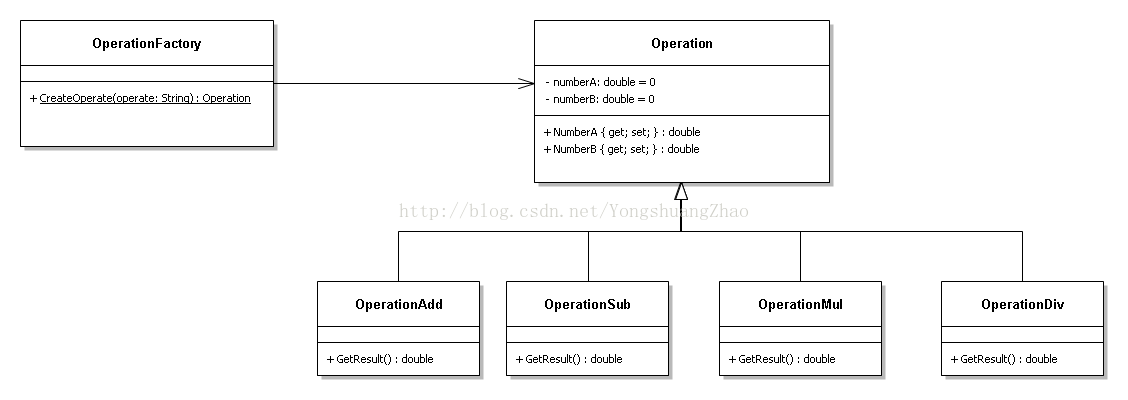
源码下载地址:https://gitee.com/ZhaoYongshuang/DesignPattern.git