- 在 NIO 中,Buffer 是一个顶层父类,它是一个抽象类, 类的层级关系图:
-
Buffer类定义了所有的缓冲区都具有的四个属性来提供关于其所包含的数据元素的信息:
-
Buffer类相关方法一览 就是我们所说的常用的api
其实这些都蛮简单的,只要我们记住几个常用的,其他的 用到了我们再来找就好了
- 从前面可以看出对于 Java 中的基本数据类型(boolean除外),都有一个 Buffer 类型与之相对应,最常用的自然是ByteBuffer 类(二进制数据),该类的主要方法如下:
通道(Channel)
基本介绍
- NIO的通道类似于流,但有些区别如下:
- 通道可以同时进行读写,而流只能读或者只能写
- 通道可以实现异步读写数据
- 通道可以从缓冲读数据,也可以写数据到缓冲:
-
BIO 中的 stream 是单向的,例如 FileInputStream 对象只能进行读取数据的操作,而 NIO 中的通道(Channel)是双向的,可以读操作,也可以写操作。
-
Channel在NIO中是一个接口public interface Channel extends Closeable{} 常用的 Channel 类有:FileChannel、DatagramChannel、ServerSocketChannel 和 SocketChannel。【ServerSocketChanne 类似 ServerSocket , SocketChannel 类似 Socket】
-
FileChannel 用于文件的数据读写,DatagramChannel 用于 UDP 的数据读写,ServerSocketChannel 和 SocketChannel 用于 TCP 的数据读写。
FileChannel 类
FileChannel主要用来对本地文件进行 IO 操作,常见的方法有
- public int read(ByteBuffer dst) ,从通道读取数据并放到缓冲区中
- public int write(ByteBuffer src) ,把缓冲区的数据写到通道中
- public long transferFrom(ReadableByteChannel src, long position, long count),从目标通道中复制数据到当前通道
- public long transferTo(long position, long count, WritableByteChannel target),把数据从当前通道复制给目标通道
例子
用FileChannel来写文件
package com.itheima.mq.rocketmq.nio;
import java.io.FileOutputStream;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
/**
- @author
- @version 1.0
- @date 2020/8/2 15:37
*/
public class NIOFileChannel01 {
public static void main(String[] args) throws Exception {
String name=“hello”;
//生成一个输出流
FileOutputStream fileOutputStream = new FileOutputStream(“d:\file01.txt”);
//通过 fileOutputStream 获取 对应的 FileChannel
//这个 fileChannel 真实 类型是 FileChannelImpl
FileChannel channel = fileOutputStream.getChannel();
ByteBuffer byteBuffer = ByteBuffer.allocate(1024);
//把数据放到缓存区中
byteBuffer.put(name.getBytes());
//转换
byteBuffer.flip();
channel.write(byteBuffer);
fileOutputStream.close();
}
}
总结一个和BIO的区别,其实很简单 ,就是把数据写到buffer里面 ,然后输入输出流从buffer里面拿数据
应用实例2-本地文件读数据
package com.itheima.mq.rocketmq.nio;
import java.io.File;
import java.io.FileInputStream;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
/**
- @author
- @version 1.0
- @date 2020/8/2 15:37
*/
public class NIOFileChannel02 {
public static void main(String[] args) throws Exception {
//创建文件流
File file = new File(“d:\file01.txt”);
FileInputStream fileInputStream = new FileInputStream(file);
FileChannel channel = fileInputStream.getChannel();
ByteBuffer allocate = ByteBuffer.allocate((int) file.length());
channel.read(allocate);
System.out.println(new String(allocate.array()));
fileInputStream.close();
}
}
应用实例3-使用一个Buffer完成文件读取
实例要求:
-
使用 FileChannel(通道) 和 方法 read , write,完成文件的拷贝
-
拷贝一个文本文件 1.txt , 放在项目下即可
代码演示
package com.itheima.mq.rocketmq.nio;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
/**
- @author
- @version 1.0
- @date 2020/8/2 15:37
*/
public class NIOFileChannel03 {
public static void main(String[] args) throws Exception {
FileInputStream fileInputStream = new FileInputStream(“1.txt”);
FileChannel fileChannel01 = fileInputStream.getChannel();
FileOutputStream fileOutputStream = new FileOutputStream(“2.txt”);
FileChannel fileChannel02 = fileOutputStream.getChannel();
ByteBuffer byteBuffer = ByteBuffer.allocate(512);
//下面就是数据读写的流程了
while (true){
byteBuffer.clear(); //清空buffer
int read = fileChannel01.read(byteBuffer);
System.out.println(“read =” + read);
if(read == -1) { //表示读完
break;
}
//将buffer 中的数据写入到 fileChannel02 – 2.txt
byteBuffer.flip();
fileChannel02.write(byteBuffer);
//将buffer 中的数据写入到 fileChannel02 – 2.txt
byteBuffer.flip();
fileChannel02.write(byteBuffer);
}
//关闭相关的流
fileInputStream.close();
fileOutputStream.close();
}
}
#继续
NIO 上文把介绍,Buffer,Channel 等讲了,我们再来粗略的分析一个selector(本文争对Java层面,如果要分析到内核的select函数的话,很懵逼)
基本介绍
- Java的NIO,用的非阻塞的IO方式。可以用一个客户端处理多个客户端的连接,其实这个就是我们前面讲的Uinx IO 模型中的I/O多路复用了,然后我们来讲讲我们最初的方式Selector选择器
- Selector 能够检测多个注册的通道上是否有事件发生(注意:多个Channel以事件的方式可以注册到同一个Selector),如果有事件发生,便获取事件然后针对每个事件进行相应的处理。这样就可以只用一个单线程去管理多个通道,也就是管理多个连接和请求
- 只有在 连接/通道 真正有读写事件发生时,才会进行读写,就大大地减少了系统开销,并且不必为每个连接都创建一个线程,不用去维护多个线程,避免了多线程之间的上下文切换导致的开销
Selector示意图和特点说明
- Netty 的 IO 线程 NioEventLoop(小六六觉得这个蛮重要的) 聚合了 Selector(选择器,也叫多路复用器),可以同时并发处理成百上千个客户端连接。
- 当线程从某客户端 Socket 通道进行读写数据时,若没有数据可用时,该线程可以进行其他任务。
- 线程通常将非阻塞 IO 的空闲时间用于在其他通道上执行 IO 操作,所以单独的线程可以管理多个输入和输出通道。
- 由于读写操作都是非阻塞的,这就可以充分提升 IO 线程的运行效率,避免由于频繁 I/O 阻塞导致的线程挂起。
- 一个 I/O 线程可以并发处理 N 个客户端连接和读写操作,这从根本上解决了传统同步阻塞 I/O 一连接一线程模型,架构的性能、弹性伸缩能力和可靠性都得到了极大的提升。
Selector相关的方法
小编也是截图的,大家如果看到这里了,应该去JDK里面找到这个类,先大致的过一下,具体的我们后面再看,有个大概映像。
说Netty 其实也就是两大类,第一大类是网络编程,第二类就是IO操作, IO操作的话再上面2节的Buffer和Channel 我们已经了解到了,那么现在我们来看看NIO的网络编程和我们前面的BIO的编程有啥区别呗,
NIO 非阻塞 网络编程原理分析图
对上面的解析
- 当客户端连接时,会通过ServerSocketChannel 得到 SocketChannel(如果是BIO的话我们得到的是Socket,就是一个客户端连接)
- Selector 进行监听 select 方法, 返回有事件发生的通道的个数.
- 将socketChannel注册到Selector上, register(Selector sel, int ops), 一个selector上可以注册多个SocketChannel
- 注册后返回一个 SelectionKey, 会和该Selector 关联(集合)
- 进一步得到各个 SelectionKey (有事件发生)
- 在通过 SelectionKey 反向获取 SocketChannel , 方法 channel()
- 可以通过 得到的 channel , 完成业务处理
NIO 网络编程案例
服务端代码
package com.liuliu.nio;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.*;
import java.util.Iterator;
import java.util.Set;
/**
- @author
- @version 1.0
- @date 2020/8/8 11:55
*/
public class NIOServer {
public static void main(String[] args) throws Exception {
// 传统io ServerSocket serverSocket = new ServerSocket(6666);
//获取一个nio的网络socket
ServerSocketChannel serverSocketChannel = ServerSocketChannel.open();
// 获取一个selector
Selector selector = Selector.open();
//绑定一个端口6666, 在服务器端监听
serverSocketChannel.socket().bind(new InetSocketAddress(6666));
//设置为非阻塞
serverSocketChannel.configureBlocking(false);
//把 serverSocketChannel 注册到 selector 关心 事件为 OP_ACCEPT
serverSocketChannel.register(selector, SelectionKey.OP_ACCEPT);
System.out.println(“注册后的selectionkey 数量=” + selector.keys().size());
//循环等待客户端连接
while (true) {
if (selector.select(1000) == 0) {
//说明这1秒内 没有事件发生,那么我们就自己让他跳出循环
continue;
}
//如果返回的>0, 就获取到相关的 selectionKey集合
//1.如果返回的>0, 表示已经获取到关注的事件
//2. selector.selectedKeys() 返回关注事件的集合
// 通过 selectionKeys 反向获取通道
//说明有事件通知了
Set selectionKeys = selector.selectedKeys();
//使用迭代器遍历
Iterator iterator = selectionKeys.iterator();
while (iterator.hasNext()) {
// 获取它的key
SelectionKey key = iterator.next();
//根据key 对应的通道发生的事件做相应处理
if (key.isAcceptable()) {
//如果是 OP_ACCEPT, 有新的客户端连接]
//生成一个socket连接,对应BIO中的 Socket socket=ServerSocket.accept
SocketChannel socketChannel = serverSocketChannel.accept();
System.out.println("客户端连接成功 生成了一个 socketChannel " + socketChannel.hashCode());
//将 SocketChannel 设置为非阻塞
socketChannel.configureBlocking(false);
//将socketChannel 注册到selector, 关注事件为 OP_READ, 同时给socketChannel
//生成一个buffter
ByteBuffer buffer = ByteBuffer.allocate(1024);
//把channel 和buffer关联
socketChannel.register(selector, SelectionKey.OP_READ, buffer);
System.out.println(“客户端连接后 ,注册的selectionkey 数量=” + selector.keys().size());
}
if (key.isReadable()) {
//发送的是读事件
//通过key 反向获取到对应channel
SocketChannel channel = (SocketChannel) key.channel();
//获取到该channel关联的buffer
ByteBuffer buffer = (ByteBuffer) key.attachment();
//把数据读到buffer中去
channel.read(buffer);
//打印从客户端发出来的数据
System.out.println("form 客户端 " + new String(buffer.array()));
}
// 手动从集合中移动当前的selectionKey, 防止重复操作
iterator.remove();
}
}
}
}
客户端代码
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
/**
- @author
- @version 1.0
- @date 2020/8/8 14:49
- NIO 网络编程的客户端
*/
public class NIOClient {
public static void main(String[] args) throws Exception {
// 得到一个网络通道
SocketChannel socketChannel = SocketChannel.open();
//设置非阻塞的操作
socketChannel.configureBlocking(false);
//提供服务器端的ip 和 端口
InetSocketAddress inetSocketAddress = new InetSocketAddress(“127.0.0.1”, 6666);
// 去服务端去连接
if (!socketChannel.connect(inetSocketAddress)) {
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
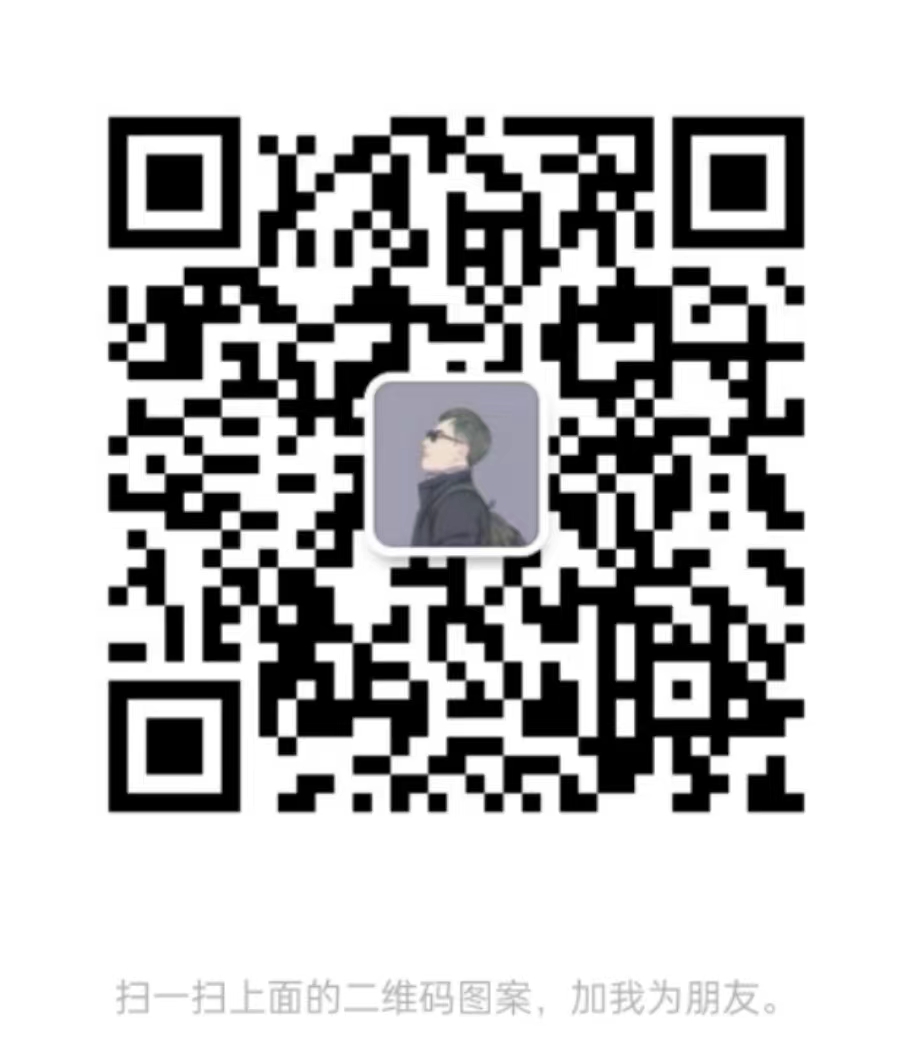
最后
经过日积月累, 以下是小编归纳整理的深入了解Java虚拟机文档,希望可以帮助大家过关斩将顺利通过面试。
由于整个文档比较全面,内容比较多,篇幅不允许,下面以截图方式展示 。
由于篇幅限制,文档的详解资料太全面,细节内容太多,所以只把部分知识点截图出来粗略的介绍,每个小节点里面都有更细化的内容!
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
098)]
[外链图片转存中…(img-ILe0hIUQ-1713701932098)]
[外链图片转存中…(img-JNVfaxbQ-1713701932098)]
[外链图片转存中…(img-0aPLJ2yT-1713701932098)]
[外链图片转存中…(img-kpMAYJLN-1713701932099)]
[外链图片转存中…(img-KwG9ceCV-1713701932099)]
由于篇幅限制,文档的详解资料太全面,细节内容太多,所以只把部分知识点截图出来粗略的介绍,每个小节点里面都有更细化的内容!
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!