红黑树是一棵二叉搜索树,它在每个节点上增加了一个存储位来表示节点的颜色,可以是Red或Black。通过对任何一条从根到叶子简单路径上的颜色来约束,红黑树保证最长路径不超过最短路径的两倍,因而近似于平衡
红黑树是满足下面红黑性质的二叉搜索树:
(1)每个节点,不是红色就是黑色的
(2)根节点是黑色的
(3)如果一个节点是红色的,则它的两个子节点是黑色的(没有连续的红节点)
(4)对每个节点,从该节点到其所有后代叶节点的简单路径上,均包含相同数目的黑色节点。(每条路径的黑色节点 的数量相等)
红黑树保证最长路径不超过最短路径的两倍,解释:
根据性质的第三点与第四点得:红结点出现最多的情况是间隔出现,所以一条路径上黑结点最少出现一半,为了满足第四点性质,所以最长路径不超过最短路径的两倍
所以为了保证红黑树的性质,当插入节点时,需要考虑颜色是否要调整。
当插入一个结点时,总体可分为三种情况:
ps:cur为当前节点,p为父节点,g为祖父节点,u为叔叔节点
(1)cur为红,p为红,g为黑,u存在且为红
则将p,u改为黑,g改为红,然后把g当成cur,继续向上调整。
(2)cur为红,p为红,g为黑,u不存在/u为黑
p为g的左孩子,cur为p的左孩子,则进行右单旋转;相反,p为g的右孩子,cur为p的右孩子,则进行左单旋转
p、g变色--p变黑,g变红
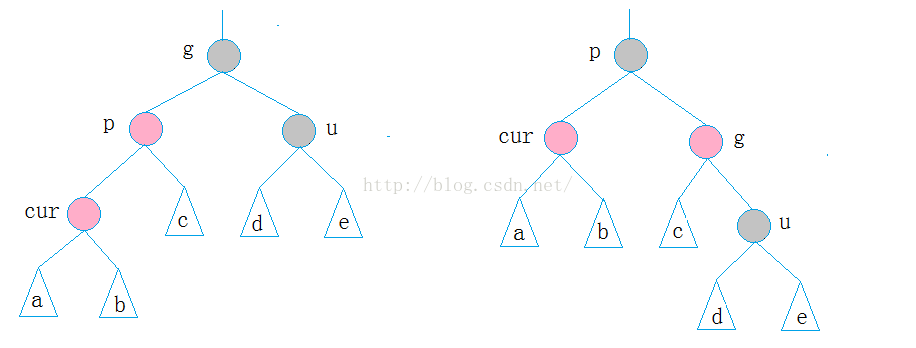
(3)cur为红,p为红,g为黑,u不存在/u为黑
p为g的左孩子,cur为p的右孩子,则针对p做左单旋转;相反,
p为g的右孩子,cur为p的左孩子,则针对p做右单旋转
则转换成了情况2
红黑树的数据插入操作:
#pragma once
#include<iostream>
using namespace std;
enum Color
{
RED,
BLACK,
};
template<class K, class V>
struct BSTreeNode
{
BSTreeNode<K, V>* _parent;
BSTreeNode<K, V>* _left;
BSTreeNode<K, V>* _right;
K _key;
V _value;
Color _color;
BSTreeNode<K, V>(const K& key, const V& value)
: _parent(NULL)
, _left(NULL)
, _right(NULL)
, _key(key)
, _value(value)
, _color(RED)
{}
};
template<class K, class V>
class RBTree
{
typedef BSTreeNode<K, V> Node;
public:
RBTree()
:_root(NULL)
{}
bool Insert(const K& key, const V& value)
{
if (_root == NULL)
{
_root = new Node(key, value);
_root->_color = BLACK;//根结点必须为黑色
return true;
}
Node* cur = _root;
Node* parent = NULL;
//1.找到结点的插入位置
while (cur)
{
if (cur->_key < key)
{
parent = cur;
cur = cur->_right;
}
else if (cur->_key > key)
{
parent = cur;
cur = cur->_left;
}
else
return false;
}
cur = new Node(key, value);
if (parent->_key < key)
parent->_right = cur;
else
parent->_left = cur;
cur->_parent = parent;
//2、开始调整颜色
while (cur != _root&&parent->_color == RED)
{
Node* grandfather = parent->_parent;
if (grandfather->_left == parent)
{
Node* uncle = grandfather->_right;
/*uncle 存在且为红色,调整方法:父与叔调黑,祖父调红。完成后,
再依次向上继续调整*/
if (uncle&&uncle->_color == RED)
{
parent->_color = uncle->_color = BLACK;
grandfather->_color = RED;
cur = grandfather;
parent = cur->_parent;
}
else//不存在或为黑色
{
if (cur == parent->_right)
{
RorateL(parent);
swap(cur, parent);
}
RorateR(grandfather);
parent->_color = BLACK;
grandfather->_color = RED;
break;
}
}
else//grandfather->_right == parent
{
Node* uncle = grandfather->_left;
/*uncle 存在且为红色,调整方法:父与叔调黑,祖父调红。完成后,
再依次向上继续调整*/
if (uncle&&uncle->_color == RED)
{
parent->_color = uncle->_color = BLACK;
grandfather->_color = RED;
cur = grandfather;
parent = cur->_parent;
}
else//不存在或为黑色
{
if (cur == parent->_left)
{
RorateR(parent);
swap(cur, parent);
}
RorateL(grandfather);
parent->_color = BLACK;
grandfather->_color = RED;
break;
}
}
}
_root->_color = BLACK;
return true;
}
bool IsBlance()
{
if (_root == NULL)
return true;
if (_root->_color == RED)
return false;
int BlackNum = 0;//一条路径上黑色结点的数目,与其他路径上黑色结点的数目进行比较
int count = 0;
//以最左边的路径上黑色结点的数目作为判断依据(每条路径上的黑色结点数目相等)
Node* cur = _root;
while (cur)
{
if (cur->_color == BLACK)
BlackNum++;
cur = cur->_left;
}
return _isBlance(_root, BlackNum,count);
}
protected:
bool _isBlance(Node*root, int BlackNum,int count)
{
if (root == NULL)
return true;
if (root->_color == RED)
{
Node*parent = root->_parent;
//不能有连续的红色结点
if (parent->_color == RED)
return false;
}
else
count++;
//判断一条路径是否已经走到叶子结点
if (root->_left == NULL&&root->_right == NULL)
{
if (count == BlackNum)
return true;
else
return false;
}
return _isBlance(root->_left, BlackNum, count)\
&&_isBlance(root->_right, BlackNum, count);
}
void RorateR(Node*parent)
{
Node* subL = parent->_left;
Node* subLR = subL->_right;
Node*ppNode = parent->_parent;
parent->_left = subLR;
if (subLR)
subLR->_parent = parent;
subL->_right = parent;
parent->_parent = subL;
if (ppNode == NULL)
{
_root = subL;
subL->_parent = NULL;
}
else
{
if (ppNode->_left == parent)
ppNode->_left = subL;
else
ppNode->_right = subL;
subL->_parent = ppNode;
}
}
void RorateL(Node*parent)
{
Node* subR = parent->_right;
Node* subRL = subR->_left;
Node* ppNode = parent->_parent;
parent->_right = subRL;
if (subRL)
subRL->_parent = parent;
subR->_left = parent;
parent->_parent = subR;
if (ppNode == NULL)
{
_root = subR;
subR->_parent = NULL;
}
else
{
if (ppNode->_left == parent)
ppNode->_left = subR;
else
ppNode->_right = subR;
subR->_parent = ppNode;
}
}
private:
Node*_root;
};