<html>
<head>
<meta charset="utf-8">
<style type="text/css">
p{
color:red;
}
.f20{
font-size:20px;
}
</style>
<link rel="stylesheet" href="css/demo01.css">
</head>
<body>
<p>这里是段落一</p>
<p>这里是段落二</p>
<p class="f20">这里是段落三</p>
<p id="p4">这里是段落四</p>
<div>
<p><span style="font-size:60px;font-weight:bolder;color:yellow;">HELLO</span></p>
<span class="f32">World</span>
<p class="f32">!!!</p>
</div>
</body>
</html>
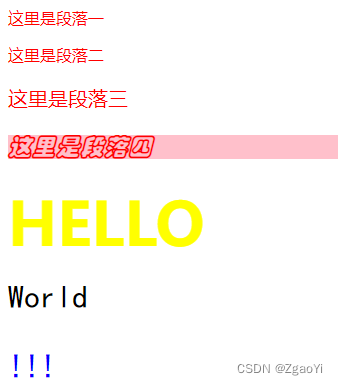
<html>
<head>
<meta charset="utf-8">
<style type="text/css">
#div1{
width:400px;
height:400px;
background-color:greenyellow;
border-width:1px;
border-style:solid;
border-color:blue;
}
#div2{
width:150px;
height:150px;
background-color:darkorange;
margin-top:100px;
margin-left:100px;
padding-top:50px;
padding-left:50px;
}
#div3{
width:100px;
height:100px;
background-color:aquamarine;
}
#div4{
width:200px;
height:200px;
margin-left:100px;
background-color:greenyellow;
}
body{
margin:0;
padding:0;
}
</style>
</head>
<body>
<div id="div1">
<div id="div2">
<div id="div3"> </div>
</div>
</div>
<div id="div4"> </div>
</body>
</html>
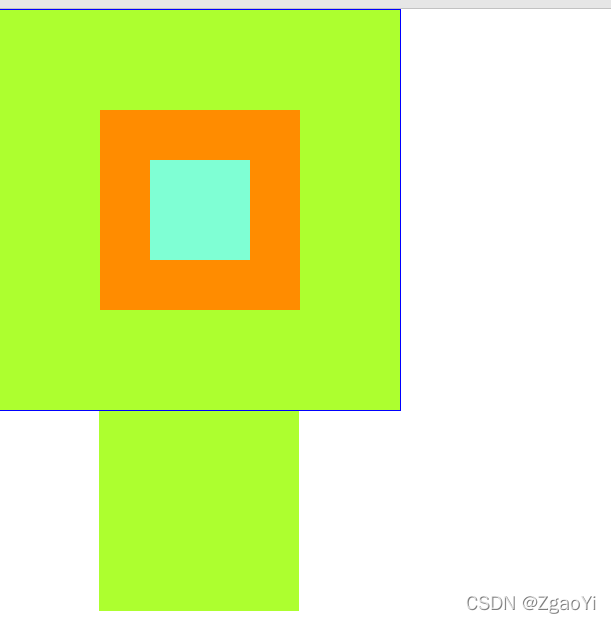
<html>
<head>
<meta charset="utf-8">
<style type="text/css">
body{
margin:0;
padding:0;
}
#div1{
width:200px;
height:50px;
background-color:greenyellow;
position:absolute;
left:100px;
top:100px;
}
#div2{
width:200px;
height:50px;
background-color:pink;
position:relative;
float:left;
margin-left:20px;
}
#div3{
height:50px;
background-color:darkorange;
}
#div4{
width:200px;
height:50px;
background-color:aqua;
float:left;
}
#div5{
width:200px;
height:50px;
background-color:olivedrab;
float:left;
}
div{
position:relative;
}
</style>
</head>
<body>
<div id="div3">
<div id="div4"> </div>
<div id="div5"> </div>
</div>
</body>
</html>

<html>
<head>
<meta charset="utf-8">
<style type="text/css">
body{
margin:0;
padding:0;
background-color:#808080;
}
div{
position:relative;
}
#div_top{
background-color: orange;
height:20%;
}
#div_left{
background-color: greenyellow;
height:80%;
width:15%;
float:left;
}
#div_main{
background-color: whitesmoke;
height:70%;
float:left;
width:85%;
}
#div_bottom{
background-color: sandybrown;
height:10%;
width:85%;
float:left;
}
#div_container{
width:80%;
height:100%;
border:0px solid blue;
margin-left:10%;
float:left;
}
</style>
</head>
<body>
<div id="div_container">
<div id="div_top">div_top</div>
<div id="div_left">div_left</div>
<div id="div_main">div_main</div>
<div id="div_bottom">div_bottom</div>
</div>
</body>
</html>
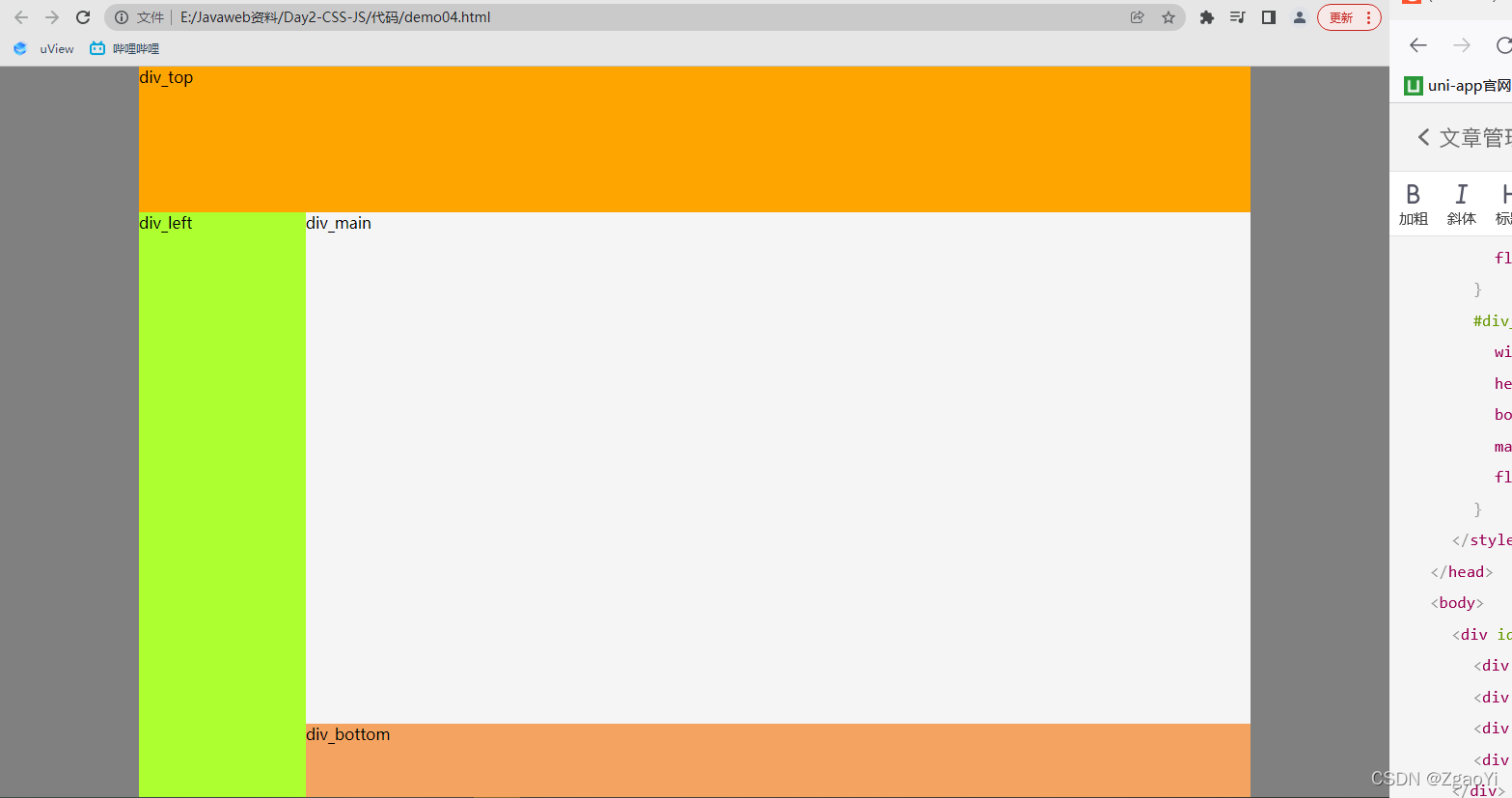
*{
color: threeddarkshadow;
}
body{
margin:0;
padding:0;
background-color:#808080;
}
div{
position:relative;
float:left;
}
#div_container{
width:80%;
height:100%;
border:0px solid blue;
margin-left:10%;
float:left;
background-color: honeydew;
border-radius:8px;
}
#div_fruit_list{
width:100%;
border:0px solid red;
}
#tbl_fruit{
width:60%;
line-height:28px;
margin-top:120px;
margin-left:20%;
}
#tbl_fruit , #tbl_fruit tr , #tbl_fruit th , #tbl_fruit td{
border:1px solid gray;
border-collapse:collapse;
text-align:center;
font-size:16px;
font-family:"黑体";
font-weight:lighter;
}
.w20{
width:20%;
}
.delImg{
width:24px;
height:24px;
}
.btn{
border:1px solid lightgray;
width:80px;
height:24px;
}
<html>
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="css/demo05.css">
</head>
<body>
<div id="div_container">
<div id="div_fruit_list">
<table id="tbl_fruit">
<tr>
<th class="w20">名称</th>
<th class="w20">单价</th>
<th class="w20">数量</th>
<th class="w20">小计</th>
<th>操作</th>
</tr>
<tr>
<td>苹果</td>
<td>5</td>
<td>20</td>
<td>100</td>
<td><img src="imgs/del.jpg" class="delImg"/></td>
</tr>
<tr>
<td>西瓜</td>
<td>3</td>
<td>20</td>
<td>60</td>
<td><img src="imgs/del.jpg" class="delImg"/></td>
</tr>
<tr>
<td>菠萝</td>
<td>4</td>
<td>25</td>
<td>100</td>
<td><img src="imgs/del.jpg" class="delImg"/></td>
</tr>
<tr>
<td>榴莲</td>
<td>3</td>
<td>30</td>
<td>90</td>
<td><img src="imgs/del.jpg" class="delImg"/></td>
</tr>
<tr>
<td>总计</td>
<td colspan="4">999</td>
</tr>
</table>
</div>
</div>
</body>
</html>
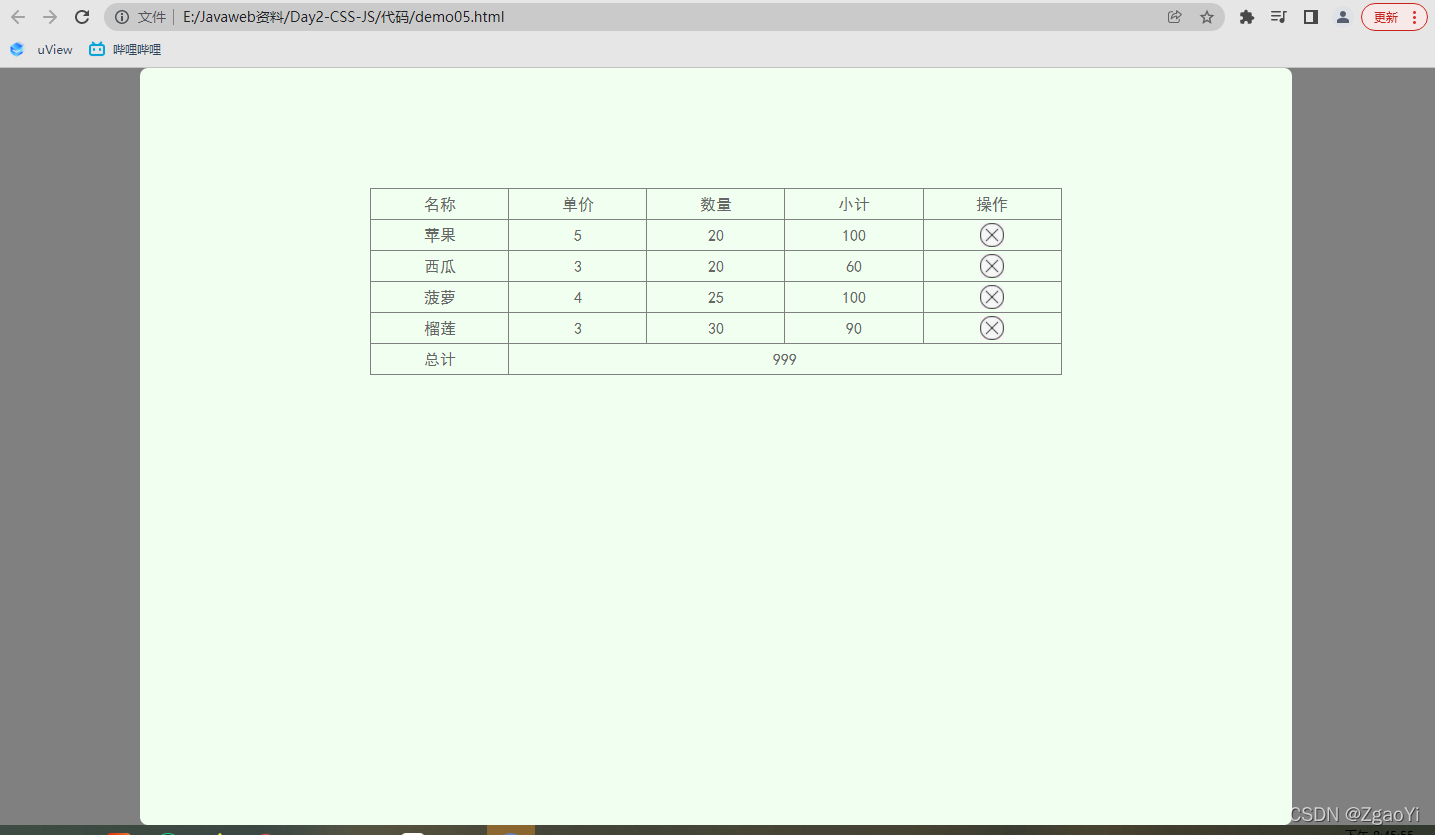