1 标准网格材质
import * as THREE from "three";
import { OrbitControls } from "three/examples/jsm/controls/OrbitControls";
import gsap from "gsap";
import * as dat from "dat.gui";
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
camera.position.set(0, 0, 10);
scene.add(camera);
const textureLoader = new THREE.TextureLoader();
const doorColorTexture = textureLoader.load("./textures/door/color.jpg");
const doorAplhaTexture = textureLoader.load("./textures/door/alpha.jpg");
const doorAoTexture = textureLoader.load(
"./textures/door/ambientOcclusion.jpg"
);
const doorHeightTexture = textureLoader.load("./textures/door/height.jpg");
const cubeGeometry = new THREE.BoxBufferGeometry(1, 1, 1, 100, 100, 100);
const material = new THREE.MeshStandardMaterial({
color: "#ffff00",
map: doorColorTexture,
alphaMap: doorAplhaTexture,
transparent: true,
aoMap: doorAoTexture,
aoMapIntensity: 1,
displacementMap: doorHeightTexture,
displacementScale: 0.1,
});
material.side = THREE.DoubleSide;
const cube = new THREE.Mesh(cubeGeometry, material);
scene.add(cube);
cubeGeometry.setAttribute(
"uv2",
new THREE.BufferAttribute(cubeGeometry.attributes.uv.array, 2)
);
const planeGeometry = new THREE.PlaneBufferGeometry(1, 1, 200, 200);
const plane = new THREE.Mesh(planeGeometry, material);
plane.position.set(1.5, 0, 0);
scene.add(plane);
planeGeometry.setAttribute(
"uv2",
new THREE.BufferAttribute(planeGeometry.attributes.uv.array, 2)
);
const light = new THREE.AmbientLight(0xffffff, 0.5);
scene.add(light);
const directionalLight = new THREE.DirectionalLight(0xffffff, 0.5);
directionalLight.position.set(10, 10, 10);
scene.add(directionalLight);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
const controls = new OrbitControls(camera, renderer.domElement);
controls.enableDamping = true;
const axesHelper = new THREE.AxesHelper(5);
scene.add(axesHelper);
const clock = new THREE.Clock();
function render() {
controls.update();
renderer.render(scene, camera);
requestAnimationFrame(render);
}
render();
window.addEventListener("resize", () => {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
renderer.setPixelRatio(window.devicePixelRatio);
});
2.粗糙度与粗糙度贴图/金属贴图、法线贴图
import * as THREE from "three";
import { OrbitControls } from "three/examples/jsm/controls/OrbitControls";
import gsap from "gsap";
import * as dat from "dat.gui";
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
camera.position.set(0, 0, 10);
scene.add(camera);
const textureLoader = new THREE.TextureLoader();
const doorColorTexture = textureLoader.load("./textures/door/color.jpg");
const doorAplhaTexture = textureLoader.load("./textures/door/alpha.jpg");
const doorAoTexture = textureLoader.load(
"./textures/door/ambientOcclusion.jpg"
);
const doorHeightTexture = textureLoader.load("./textures/door/height.jpg");
const roughnessTexture = textureLoader.load("./textures/door/roughness.jpg");
const metalnessTexture = textureLoader.load("./textures/door/metalness.jpg");
const normalTexture = textureLoader.load("./textures/door/normal.jpg");
const cubeGeometry = new THREE.BoxBufferGeometry(1, 1, 1, 100, 100, 100);
const material = new THREE.MeshStandardMaterial({
color: "#ffff00",
map: doorColorTexture,
alphaMap: doorAplhaTexture,
transparent: true,
aoMap: doorAoTexture,
aoMapIntensity: 1,
displacementMap: doorHeightTexture,
displacementScale: 0.1,
roughness: 1,
roughnessMap: roughnessTexture,
metalness: 1,
metalnessMap: metalnessTexture,
normalMap: normalTexture,
});
material.side = THREE.DoubleSide;
const cube = new THREE.Mesh(cubeGeometry, material);
scene.add(cube);
cubeGeometry.setAttribute(
"uv2",
new THREE.BufferAttribute(cubeGeometry.attributes.uv.array, 2)
);
const planeGeometry = new THREE.PlaneBufferGeometry(1, 1, 200, 200);
const plane = new THREE.Mesh(planeGeometry, material);
plane.position.set(1.5, 0, 0);
scene.add(plane);
planeGeometry.setAttribute(
"uv2",
new THREE.BufferAttribute(planeGeometry.attributes.uv.array, 2)
);
const light = new THREE.AmbientLight(0xffffff, 0.5);
scene.add(light);
const directionalLight = new THREE.DirectionalLight(0xffffff, 0.5);
directionalLight.position.set(10, 10, 10);
scene.add(directionalLight);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
const controls = new OrbitControls(camera, renderer.domElement);
controls.enableDamping = true;
const axesHelper = new THREE.AxesHelper(5);
scene.add(axesHelper);
const clock = new THREE.Clock();
function render() {
controls.update();
renderer.render(scene, camera);
requestAnimationFrame(render);
}
render();
window.addEventListener("resize", () => {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
renderer.setPixelRatio(window.devicePixelRatio);
});
3.纹理加载进度情况
import * as THREE from "three";
import { OrbitControls } from "three/examples/jsm/controls/OrbitControls";
import gsap from "gsap";
import * as dat from "dat.gui";
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
camera.position.set(0, 0, 10);
scene.add(camera);
var div = document.createElement("div");
div.style.width = "200px";
div.style.height = "200px";
div.style.position = "fixed";
div.style.right = 0;
div.style.top = 0;
div.style.color = "#fff";
document.body.appendChild(div);
let event = {};
event.onLoad = function () {
console.log("图片加载完成");
};
event.onProgress = function (url, num, total) {
console.log("图片加载完成:", url);
console.log("图片加载进度:", num);
console.log("图片总数:", total);
let value = ((num / total) * 100).toFixed(2) + "%";
console.log("加载进度的百分比:", value);
div.innerHTML = value;
};
event.onError = function (e) {
console.log("图片加载出现错误");
console.log(e);
};
const loadingManager = new THREE.LoadingManager(
event.onLoad,
event.onProgress,
event.onError
);
const textureLoader = new THREE.TextureLoader(loadingManager);
const doorColorTexture = textureLoader.load(
"./textures/door/color.jpg"
);
const doorAplhaTexture = textureLoader.load("./textures/door/alpha.jpg");
const doorAoTexture = textureLoader.load(
"./textures/door/ambientOcclusion.jpg"
);
const doorHeightTexture = textureLoader.load("./textures/door/height.jpg");
const roughnessTexture = textureLoader.load("./textures/door/roughness.jpg");
const metalnessTexture = textureLoader.load("./textures/door/metalness.jpg");
const normalTexture = textureLoader.load("./textures/door/normal.jpg");
const cubeGeometry = new THREE.BoxBufferGeometry(1, 1, 1, 100, 100, 100);
const material = new THREE.MeshStandardMaterial({
color: "#ffff00",
map: doorColorTexture,
alphaMap: doorAplhaTexture,
transparent: true,
aoMap: doorAoTexture,
aoMapIntensity: 1,
displacementMap: doorHeightTexture,
displacementScale: 0.1,
roughness: 1,
roughnessMap: roughnessTexture,
metalness: 1,
metalnessMap: metalnessTexture,
normalMap: normalTexture,
});
material.side = THREE.DoubleSide;
const cube = new THREE.Mesh(cubeGeometry, material);
scene.add(cube);
cubeGeometry.setAttribute(
"uv2",
new THREE.BufferAttribute(cubeGeometry.attributes.uv.array, 2)
);
const planeGeometry = new THREE.PlaneBufferGeometry(1, 1, 200, 200);
const plane = new THREE.Mesh(planeGeometry, material);
plane.position.set(1.5, 0, 0);
scene.add(plane);
planeGeometry.setAttribute(
"uv2",
new THREE.BufferAttribute(planeGeometry.attributes.uv.array, 2)
);
const light = new THREE.AmbientLight(0xffffff, 0.5);
scene.add(light);
const directionalLight = new THREE.DirectionalLight(0xffffff, 0.5);
directionalLight.position.set(10, 10, 10);
scene.add(directionalLight);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
const controls = new OrbitControls(camera, renderer.domElement);
controls.enableDamping = true;
const axesHelper = new THREE.AxesHelper(5);
scene.add(axesHelper);
const clock = new THREE.Clock();
function render() {
controls.update();
renderer.render(scene, camera);
requestAnimationFrame(render);
}
render();
window.addEventListener("resize", () => {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
renderer.setPixelRatio(window.devicePixelRatio);
});
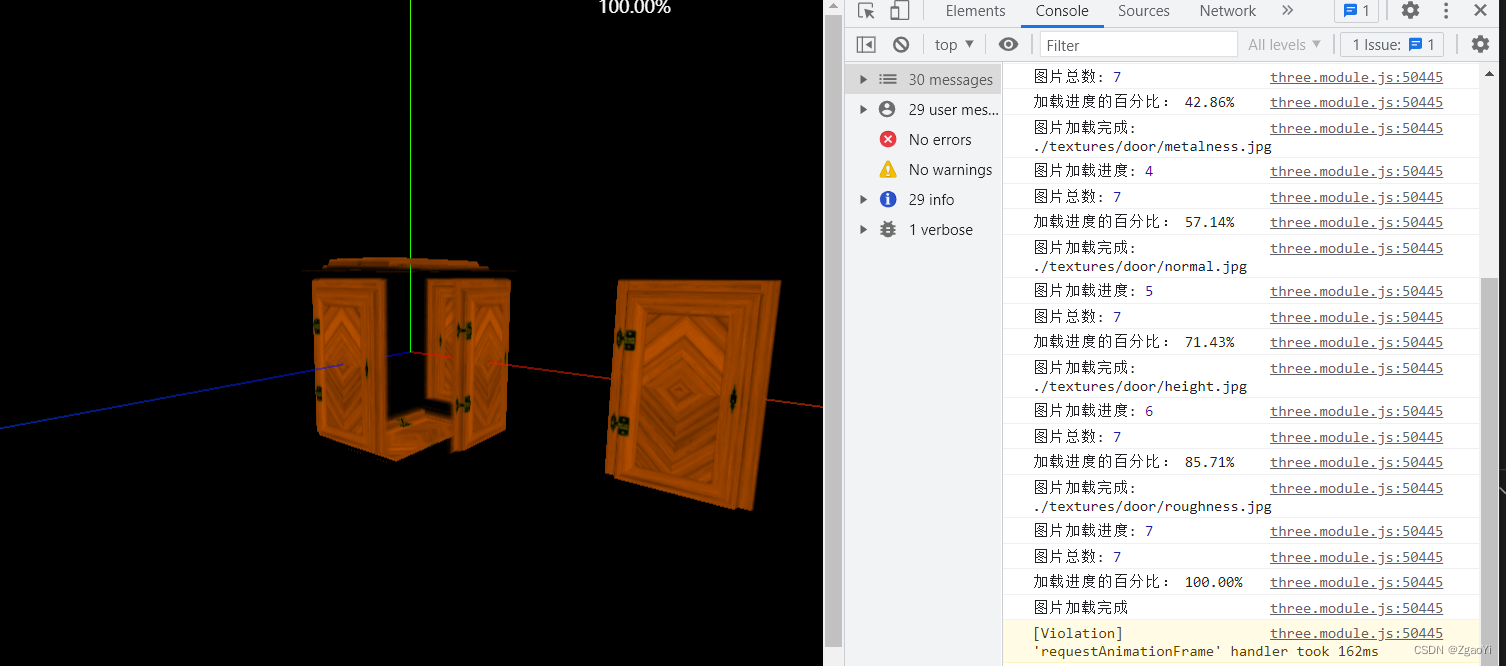
4 详解环境贴图
import * as THREE from "three";
import { OrbitControls } from "three/examples/jsm/controls/OrbitControls";
import gsap from "gsap";
import * as dat from "dat.gui";
import { RGBELoader } from "three/examples/jsm/loaders/RGBELoader";
const rgbeLoader = new RGBELoader();
rgbeLoader.loadAsync("textures/hdr/002.hdr").then((texture) => {
texture.mapping = THREE.EquirectangularReflectionMapping;
scene.background = texture;
scene.environment = texture;
});
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
camera.position.set(0, 0, 10);
scene.add(camera);
const cubeTextureLoader = new THREE.CubeTextureLoader();
const envMapTexture = cubeTextureLoader.load([
"textures/environmentMaps/1/px.jpg",
"textures/environmentMaps/1/nx.jpg",
"textures/environmentMaps/1/py.jpg",
"textures/environmentMaps/1/ny.jpg",
"textures/environmentMaps/1/pz.jpg",
"textures/environmentMaps/1/nz.jpg",
]);
const sphereGeometry = new THREE.SphereBufferGeometry(1, 20, 20);
const material = new THREE.MeshStandardMaterial({
metalness: 0.7,
roughness: 0.1,
});
const sphere = new THREE.Mesh(sphereGeometry, material);
scene.add(sphere);
scene.background = envMapTexture;
scene.environment = envMapTexture;
const light = new THREE.AmbientLight(0xffffff, 0.5);
scene.add(light);
const directionalLight = new THREE.DirectionalLight(0xffffff, 0.5);
directionalLight.position.set(10, 10, 10);
scene.add(directionalLight);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
const controls = new OrbitControls(camera, renderer.domElement);
controls.enableDamping = true;
const axesHelper = new THREE.AxesHelper(5);
scene.add(axesHelper);
const clock = new THREE.Clock();
function render() {
controls.update();
renderer.render(scene, camera);
requestAnimationFrame(render);
}
render();
window.addEventListener("resize", () => {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
renderer.setPixelRatio(window.devicePixelRatio);
});
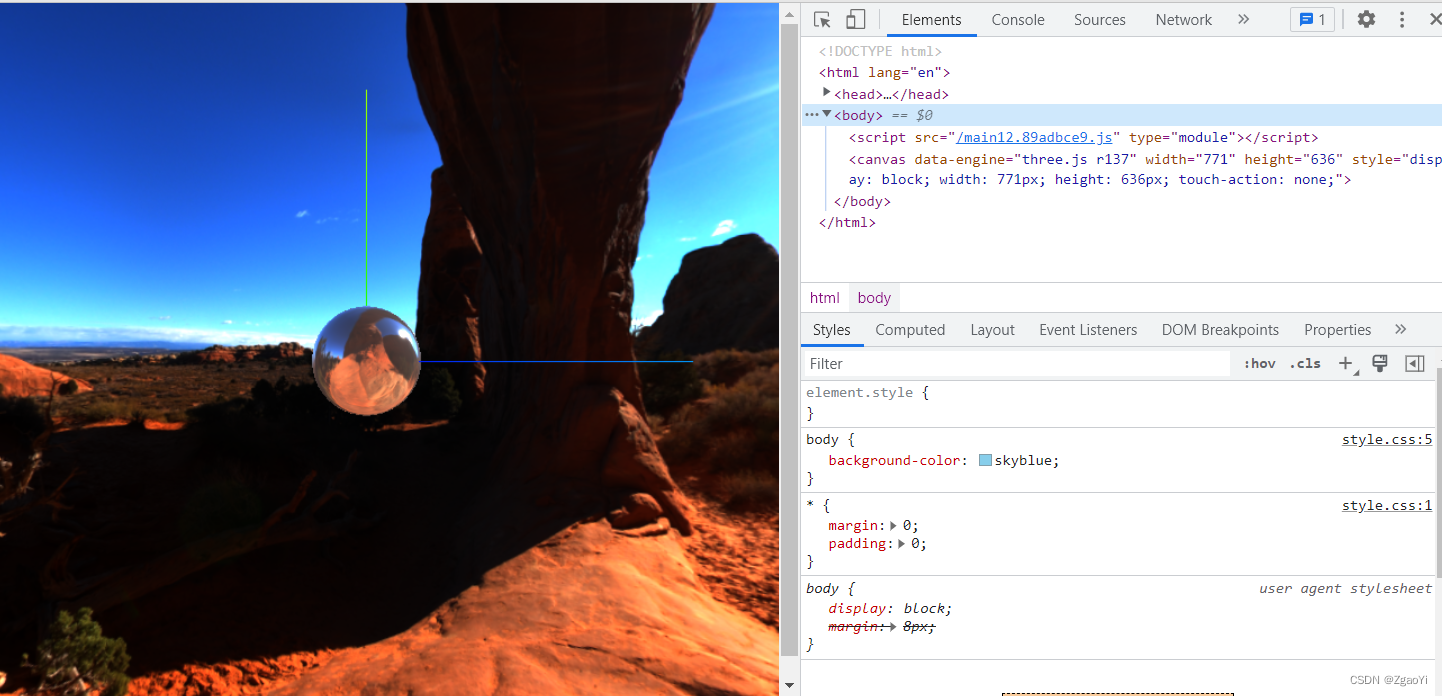