#include<iostream>
#include<string>
#include<iomanip>
#include<fstream>
using namespace std;
class person
{
public:
person();
person(int nu, int ty, string na, string se, int ag, string par, string st, double pa, person * next);
person(int nu, int ty, string na, string se, int ag, string par, string st, double pa);
int getnum() {return num;}
string getname() {return name;}
string getsex() { return sex; }
int getage() { return age; }
void setage(int sag) { age = sag; }
string getparty() { return party; }
string getstudy() { return study; }
void setpay(double spa) { pay = spa; }
int gettype() { return type; }
person* getnext() { return next; }
void setnext(person* next) { this->next = next; }
virtual double getpay();//计算员工工资函数
void display();
protected:
int num;
int type;
string name;
string sex;
int age;
string party;//政治面貌
string study;//学历
double pay;
person* next;
};
person::person()
{
num = 0;
type = 0;
name = " ";
sex = " ";
age = 0;
party = " ";
study = " ";
pay = 0.0;
next = NULL;
}
person::person(int nu, int ty, string na, string se, int ag, string par, string st, double pa,person*next)
{
this->num = nu;
this->type = ty;
this->name = na;
this->sex = se;
this->age = ag;
this->party = par;
this->study = st;
this->pay = pa;
this->next = next;
}
person::person(int nu, int ty, string na, string se, int ag, string par, string st, double pa)
{
this->num = nu;
this->type = ty;
this->name = na;
this->sex = se;
this->age = ag;
this->party = par;
this->study = st;
this->pay = pa;
this->next = NULL;
}
void person::display()
{
cout << "编号:" << num << endl;
cout << "类型:" << type << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "年龄:" << age << endl;
cout << "政治面貌:" << party << endl;
cout << "学历:" << study << endl;
cout << "工资:" << pay << endl;
}
double person::getpay()
{
return pay;
}
class teacher :virtual public person
{
public:
double getpay();
void getteacher();
protected:
string teachpos;//职称
double coursefee;//课时费
double coursenum;//基本工作量
};
void teacher::getteacher()
{
cout << "请输入授课教师的职称:";
cin >> teachpos;
cout << endl;
coursefee = 30.0;
cout << "请输入授课教师完成的工作量:";
cin >> coursenum;
}
double teacher::getpay()
{
if (teachpos == "教授")
pay = 1600;
else if (teachpos == "副教授")
pay = 1200;
else if (teachpos == "讲师")
pay = 800;
pay = pay + (coursenum - 120) * coursefee;
return pay;
}
class tester :virtual public person
{
public:
void gettester();
double getpay();
protected:
string testerclass;
};
void tester::gettester()
{
cout << "请输入实验员的级别:";
cin >> testerclass;
cout << endl;
}
double tester::getpay()
{
if (testerclass == "助理实验师")
pay = 650;
if (testerclass == "实验师")
pay = 850;
if (testerclass == "高级实验师")
pay = 1050;
pay = pay + 150;
return pay;
}
class staff :virtual public person
{
public:
void getstaff();
double getpay();
protected:
string staffclass;
};
void staff::getstaff()
{
cout << "请输入行政人员的职务级别:";
cin >> staffclass;
cout << endl;
}
double staff::getpay()
{
if (staffclass == "处级")
pay = 2500;
if (staffclass == "科级")
pay = 2000;
if (staffclass == "科员")
pay = 1500;
pay = pay + 250;
return pay;
}
class staffteacher :public staff, public teacher
{
public:
void getstaffteacher();
double getpay();
};
void staffteacher::getstaffteacher()
{
coursefee = 20.0;
}
double staffteacher::getpay()
{
if (staffclass=="处级")
{
if (teachpos=="教授")
pay = 2000;
else if (teachpos=="副教授")
pay = 1800;
else
pay = 1600;
}
if (staffclass=="科级")
{
if (teachpos=="教授")
pay = 1800;
else if (teachpos=="副教授")
pay = 1600;
else
pay = 1400;
}
if (staffclass=="科员")
{
if (teachpos=="教授")
pay = 1600;
else if (teachpos=="副教授")
pay = 1400;
else
pay = 1200;
}
pay = pay + (coursenum-70) * coursefee + 250;
return pay;
}
class testerteacher :public tester, public teacher
{
public:
void gettesterteacher();
double getpay();
};
void testerteacher::gettesterteacher()
{
coursefee = 30.0;
}
double testerteacher::getpay()
{
if (teachpos == "教授")
{
if (testerclass == "助理实验师")
pay = 2000;
else if (testerclass == "实验师")
pay = 2300;
else
pay = 2600;
}
if (teachpos=="副教授")
{
if (testerclass == "助理实验师")
pay = 1900;
else if (testerclass == "实验师")
pay = 2200;
else
pay = 2500;
}
if (teachpos=="讲师")
{
if (testerclass == "助理实验师")
pay = 1800;
else if (testerclass == "实验师")
pay = 2100;
else
pay = 2400;
}
pay = pay + (coursenum - 120) * coursefee + 150;
return pay;
}
class college
{
public:
college() { myfirst = NULL; }
college(int nenum, int netype, string nename, string nesex, int nage, string neparty, string nestudy, double nepay)
{
myfirst = new person(nenum, netype, nename, nesex, nage, neparty, nestudy, nepay);
}
~college();
void load();
void add();
void input(int number);
void insert(int nnum, int ntype,string nname, string nsex, int nage, string nparty, string nstudy, double npay); //学校员工链表中插入新员工结点
bool findnum();
bool findname();
bool modify(); //修改员工信息
bool deleteperson();
void count();
void save(); //员工信息存盘
void show();
private:
person* myfirst;
};
college::~college()
{
person* next = myfirst, * temp;
while (next != NULL)
{
temp = next;
next = next->getnext();
delete temp;
}
myfirst = NULL;
}
void college::load()
{
double npay;
int nnum, nage, ntype;
string nname, nsex, nparty, nstudy;
ifstream infile("person.txt", ios::in | ios::out);
if (!infile)
{
cerr << "open person.txt error!" << endl;
}
cout << "请输入编号,类型,姓名,性别,政治面貌,学历:" << endl;
cin >> nnum >> ntype >> nname >> nsex >> nage >> nparty >> nstudy >> npay;
infile >> nnum >> ntype >> nname >> nsex >> nage >> nparty >> nstudy >> npay;
infile.close();
cout << endl << "存储在文件中的学校人员信息已加载到系统中" << endl;
}
void college::add()
{
int tmpnum, number1, number2;
person* p = myfirst;
if (p == NULL)
{
cout << "目前学校无员工,请输入新员工的编号:";
cin >> tmpnum;
input(tmpnum);
}
else
{
if (p->getnext() == NULL)
{
number1 = p->getnum() + 1;
input(number1);
}
else
{
while (p->getnext() != NULL)
{
p = p->getnext();
}
number2 = p->getnum() + 1;
input(number2);
}
}
}
void college::input(int number)
{
int nage, ntype;
double npay;
string nname, nsex, nparty, nstudy;
cout << "请选择是任课教师(输入1),实验员(输入2),行政人员(输入3),教师兼职实验人员(输入4),还是行政人员兼职教师(输入5):" << endl;
cin >> ntype;
cout << "请输入编号为" << number << "的员工信息" << endl;
cout << "输入姓名:" << endl;
cin >> nname;
cout << "输入性别:" << endl;
cin >> nsex;
cout << "输入年龄:" << endl;
cin >> nage;
cout << "输入政治面貌:群众,团员还是党员?" << endl;
cin >> nparty;
cout << "输入学历:小学,初中,高中,专科,本科,硕士,博士:" << endl;
cin >> nstudy;
if (ntype == 1)
{
teacher t1;
t1.getteacher();
npay = t1.getpay();
}
else if (ntype == 2)
{
tester te;
te.gettester();
npay = te.getpay();
}
else if (ntype == 3)
{
staff s1;
s1.getstaff();
npay = s1.getpay();
}
else if (ntype == 4)
{
testerteacher tt;
tt.gettesterteacher();
npay = tt.getpay();
}
else if (ntype == 5)
{
staffteacher st1;
st1.getstaffteacher();
npay = st1.getpay();
}
cout << "该任课老师的工资为:" << npay << endl;
insert(number,ntype, nname, nsex, nage, nparty, nstudy, npay);
}
void college::insert(int nnum, int ntype,string nname, string nsex, int nage, string nparty, string nstudy, double npay)
{
person* p = myfirst;
if (p == NULL)
{
myfirst = new person(nnum, ntype, nname, nsex, nage, nparty, nstudy, npay);
}
else
{
while (p->getnext() != NULL)
p = p->getnext();
p->setnext(new person(nnum, ntype, nname, nsex, nage, nparty, nstudy, npay, p->getnext()));
}
}
bool college::findname()
{
string tmpname;
person* ahead = myfirst;
person* follow = ahead;
cout << "请输入员工的姓名:";
cin >> tmpname;
if (ahead == NULL)
{
cout << "本校暂无员工信息!" << endl;
return false;
}
else
{
while (ahead != NULL)
{
if (ahead->getname()==tmpname)
{
ahead->display();
return true;
}
else
{
follow = ahead;
ahead = ahead->getnext();
}
}
cout << "本校无此员工信息!" << endl;
return false;
}
}
bool college::findnum()
{
int id;
person* ahead = myfirst;
person* follow = ahead;
cout << "请输入员工的编号:" << endl;
cin >> id;
if (ahead == NULL)
{
cout << "本校暂无员工信息!" << endl;
return false;
}
else
{
while (ahead != NULL)
{
if (ahead->getnum() == id)
{
ahead->display();
return true;
}
else
{
follow = ahead;
ahead = ahead->getnext();
}
}
cout << "本校无此员工信息!" << endl;
return false;
}
}
bool college::modify() //修改员工信息
{
int number;
person* ahead = myfirst;
person* follow = ahead;
cout << "请输入要修改的学校员工编号:";
cin >> number;
if (ahead == NULL)
{
cout << "本校无员工!" << endl;
return false;
}
else
{
while (ahead != NULL)
{
if (ahead->getnum() == number)
{
ahead->display();
while (1)
{
int i;
int tmpnumber;
string temp;
cout << "请选择要修改的员工信息:" << endl;
cout << " 1:姓名 2:性别 3:年龄 4:政治面貌 5:学历 6:工资 " << endl;
cout << " 请选择(1~6)中的选项:";
cin >> i;
switch (i)
{
case 1: { cout << "输入修改姓名:"; cin >> temp; ahead->getname()==temp; }; break;
case 2: { cout << "输入修改性别:"; cin >> temp; ahead->getsex()==temp; }; break;
case 3: { cout << "输入修改年龄:"; cin >> tmpnumber; ahead->setage(tmpnumber); }; break;
case 4: { cout << "输入修改政治面貌:"; cin >> temp; ahead->getparty()==temp; }; break;
case 5: { cout << "输入修改学历:"; cin >> temp; ahead->getstudy()==temp; }; break;
case 6: { cout << "输入修改工资:"; cin >> tmpnumber; ahead->setpay(tmpnumber); }; break;
}
return true;
}
}
else
{
ahead = ahead->getnext(); follow = ahead;
}
}
cout << "本校没有此工作编号的员工!" << endl;
return false;
}
}
bool college::deleteperson() //删除员工信息
{
int i;
person* ahead = myfirst;
person* follow = ahead;
cout << "请输入要删除学校人员的工作编号:";
cin >> i;
if (ahead == NULL)
{
cout << "无员工可以删除";
return false;
}
else if (ahead->getnum() == i)
{
myfirst = myfirst->getnext();
cout << "工作编号为" << i << "的学校员工已被删除了!" << endl;
delete ahead;
return true;
}
else
{
ahead = ahead->getnext();
while (ahead != NULL)
{
if (ahead->getnum() == i)
{
follow->setnext(ahead->getnext());
cout << "编号为" << i << "的成员以被删除\n";
delete ahead;
return true;
}
follow = ahead;
ahead = ahead->getnext();
}
cout << "要删除的学校员工不存在,无法删除!" << endl;
return false;
}
}
void college::count() //统计员工信息
{
int i, amount = 0;
cout << " ***********************************************" << '\n'
<< " * *" << '\n'
<< " * 1.统计学校职工中的党员人数 *" << '\n'
<< " * *" << '\n'
<< " * 2.统计学校中女职工人数 *" << '\n'
<< " * *" << '\n'
<< " *************************************************" << '\n'
<< "请您选择上面的选项:" << endl;
cin >> i;
person* ahead = myfirst;
person* follow = ahead;
if (ahead == NULL)
{
cout << "学校无人员信息" << endl;
}
else
{
switch (i)
{
case 1:
{ while (ahead != NULL)
{
if (ahead->getparty()=="党员")
{
ahead = ahead->getnext();
amount++;
}
else
ahead = ahead->getnext();
}
cout << "学校中的党员人数:" << amount << endl;
};
break;
case 2:
{ while (ahead != NULL)
{
if (ahead->getsex()=="女")
{
ahead = ahead->getnext();
amount++;
}
else
ahead = ahead->getnext();
}
cout << "学校中的女员工人数:" << amount << endl; };
break;
}
}
}
void college::save() //员工信息存盘
{
ofstream outfile("person.txt", ios::out);
person* p = myfirst;
if (!outfile)
{
cerr << "open outfile error!" << endl;
exit(1);
}
while (p)
{
outfile << p->getnum() << "\t" << p->getname() << "\t" << p->getsex() << "\t" << p->getage() << "\t"
<< p->getparty() << "\t" << p->getstudy() << "\t" << p->getpay() << "\t" << p->gettype() << endl;
p = p->getnext();
}
outfile.close();
cout << "数据已保存" << endl;
}
void college::show() //显示学校所有员工信息
{
person* ahead = myfirst;
cout << setw(8) << "编号" << setw(8) << "姓名" << setw(8) << "性别" << setw(8) << "年龄" << setw(8)
<< "政治面貌" << setw(8) << "学历" << setw(8) << "工资" << setw(8) << "人员类型" << endl;
while (ahead != NULL)
{
cout << setw(4) << ahead->getnum() << setw(6) << ahead->getname() << setw(5) << ahead->getsex()
<< setw(4) << ahead->getage() << setw(10) << ahead->getparty() << setw(6) << ahead->getstudy()
<< setw(12) << ahead->getpay() << setw(12) << ahead->gettype() << endl;
ahead = ahead->getnext();
}
}
int main()
{
college co;;
int i;
while (1)
{
cout << "========高等院校人员管理系统======" << endl;
cout << " 1. 从文件中加载员工信息 " << endl;
cout << " 2. 增加学校员工信息 " << endl;
cout << " 3. 按编号查询学校员工信息 " << endl;
cout << " 4. 按姓名查询学校员工信息 " << endl;
cout << " 5. 修改学校员工信息 " << endl;
cout << " 6. 删除学校员工信息 " << endl;
cout << " 7. 统计学校员工信息 " << endl;
cout << " 8. 学校员工信息存盘 " << endl;
cout << " 9. 显示所有员工信息 " << endl;
cout << " 10. 退出系统 " << endl;
cout << "================================" << endl;
cout << "请选择上面的选项(1~10):" << endl;
cin >> i;
switch (i)
{
case 1:co.load(); break;
case 2:co.add(); break;
case 3:co.findnum(); break;
case 4:co.findname(); break;
case 5:co.modify(); break;
case 6:co.deleteperson(); break;
case 7:co.count(); break;
case 8:co.save(); break;
case 9:co.show(); break;
case 10:exit(1); break;
}
}
system("pause");
return 0;
}
课程设计:高校工资管理系统
于 2022-05-07 14:55:04 首次发布
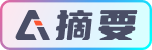