Solitaire
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submission(s): 4606 Accepted Submission(s): 1400
Problem Description
Solitaire is a game played on a chessboard 8x8. The rows and columns of the chessboard are numbered from 1 to 8, from the top to the bottom and from left to right respectively.
There are four identical pieces on the board. In one move it is allowed to:
> move a piece to an empty neighboring field (up, down, left or right),
> jump over one neighboring piece to an empty field (up, down, left or right).
There are 4 moves allowed for each piece in the configuration shown above. As an example let's consider a piece placed in the row 4, column 4. It can be moved one row up, two rows down, one column left or two columns right.
Write a program that:
> reads two chessboard configurations from the standard input,
> verifies whether the second one is reachable from the first one in at most 8 moves,
> writes the result to the standard output.
There are four identical pieces on the board. In one move it is allowed to:
> move a piece to an empty neighboring field (up, down, left or right),
> jump over one neighboring piece to an empty field (up, down, left or right).
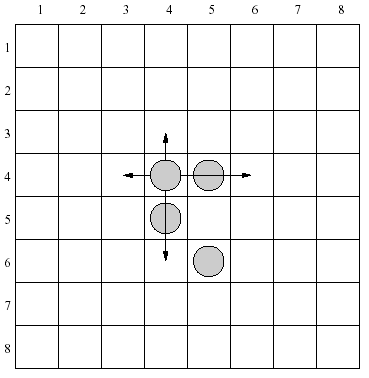
There are 4 moves allowed for each piece in the configuration shown above. As an example let's consider a piece placed in the row 4, column 4. It can be moved one row up, two rows down, one column left or two columns right.
Write a program that:
> reads two chessboard configurations from the standard input,
> verifies whether the second one is reachable from the first one in at most 8 moves,
> writes the result to the standard output.
Input
Each of two input lines contains 8 integers a1, a2, ..., a8 separated by single spaces and describes one configuration of pieces on the chessboard. Integers a2j-1 and a2j (1 <= j <= 4) describe the position of one piece - the row number and the column number respectively. Process to the end of file.
Output
The output should contain one word for each test case - YES if a configuration described in the second input line is reachable from the configuration described in the first input line in at most 8 moves, or one word NO otherwise.
Sample Input
4 4 4 5 5 4 6 5 2 4 3 3 3 6 4 6
Sample Output
YES
Source
Recommend
Ignatius.L
题意:给你四个棋子的初始位置和需要到的位置,每个棋子可以上下左右走,但如果走的方向旁边有一个棋子要跳过这个棋子,问能不能不大于八步走到要走的地方
解题思路:bfs
#include <iostream>
#include <cstdio>
#include <cstring>
#include <string>
#include <algorithm>
#include <cmath>
#include <map>
#include <cmath>
#include <set>
#include <stack>
#include <queue>
#include <vector>
#include <bitset>
#include <functional>
using namespace std;
#define LL long long
const int INF = 0x3f3f3f3f;
struct node
{
int x[4], y[4], step;
}s, e;
bool mp[10][10];
bool visit[8][8][8][8][8][8][8][8];
int dir[4][2] = { { 1,0 },{ -1,0 },{ 0,1 },{ 0,-1 } };
int check(node a)
{
for (int i = 0; i<4; i++)
if (!mp[a.x[i]][a.y[i]]) return 0;
return 1;
}
int check1(node p, int a)
{
for (int i = 0; i<4; i++)
if (i != a&&p.x[i] == p.x[a] && p.y[i] == p.y[a]) return 0;
return 1;
}
int bfs()
{
queue<node>q;
node pre, next;
s.step = 0;
q.push(s);
memset(visit, false, sizeof visit);
visit[s.x[0]][s.y[0]][s.x[1]][s.y[1]][s.x[2]][s.y[2]][s.x[3]][s.y[3]] = true;
while (!q.empty())
{
pre = q.front();
q.pop();
if (pre.step >= 8) return 0;
for (int i = 0; i<4; i++)
{
for (int j = 0; j<4; j++)
{
next = pre;
next.x[i] += dir[j][0];
next.y[i] += dir[j][1];
next.step++;
if (next.x[i]<0 || next.x[i] >= 8 || next.y[i]<0 || next.y[i] >= 8) continue;
if (check1(next, i))
{
if (check(next)) return 1;
if (!visit[next.x[0]][next.y[0]][next.x[1]][next.y[1]][next.x[2]][next.y[2]][next.x[3]][next.y[3]])
{
visit[next.x[0]][next.y[0]][next.x[1]][next.y[1]][next.x[2]][next.y[2]][next.x[3]][next.y[3]] = true;
q.push(next);
}
}
else
{
next.x[i] += dir[j][0];
next.y[i] += dir[j][1];
if (!check1(next, i)) continue;
if (next.x[i]<0 || next.x[i] >= 8 || next.y[i]<0 || next.y[i] >= 8) continue;
if (check(next)) return 1;
if (!visit[next.x[0]][next.y[0]][next.x[1]][next.y[1]][next.x[2]][next.y[2]][next.x[3]][next.y[3]])
{
visit[next.x[0]][next.y[0]][next.x[1]][next.y[1]][next.x[2]][next.y[2]][next.x[3]][next.y[3]] = true;
q.push(next);
}
}
}
}
}
return 0;
}
int main()
{
while (~scanf("%d %d", &s.x[0], &s.y[0]))
{
s.x[0]--; s.y[0]--;
for (int i = 1; i<4; i++)
{
scanf("%d %d", &s.x[i], &s.y[i]);
s.x[i]--; s.y[i]--;
}
memset(mp, false, sizeof mp);
for (int i = 0; i<4; i++)
{
scanf("%d %d", &e.x[i], &e.y[i]);
e.x[i]--; e.y[i]--;
mp[e.x[i]][e.y[i]] = true;
}
int flag = bfs();
if (flag == 1) printf("YES\n");
else printf("NO\n");
}
return 0;
}