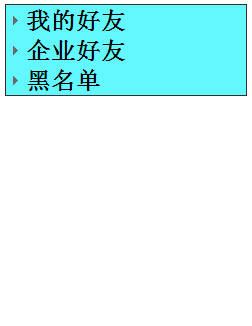
实现这样的效果。
直接看代码,html部分:


<body> <div class="box"> <div class="lists" id="qq"> <div id="div1"> <span class="normal"></span> <h2>我的好友</h2> </div> <ul> <li>张三</li> <li>张三</li> <li>李三</li> <li>李三</li> </ul> <div id="div2"> <span class="normal"></span> <h2>企业好友</h2> </div> <ul> <li>李四</li> <li>李四</li> <li>李四</li> </ul> <div id="div3"> <span class="normal"></span> <h2>黑名单</h2> </div> <ul> <li>王五</li> <li>王五</li> </ul> </div> </div> </body>
css部分:


<style> *{ margin:0; padding: 0; } body{ background: #000; list-style: none; } .box{ width: 255px; height: 330px; background-color: #fff; margin: 155px auto; position: relative; } .box .lists{ border: 1px solid #000; margin: 5px 8px 0 8px; width: 239px; position: absolute; } .lists div{ background-color: #00ffff; cursor: pointer; } .lists span.normal{ display:inline-block; width: 0; height: 0; border: 5px solid transparent; /*设置边框无色*/ border-left-color: #333; margin-left: 2px; line-height: 40px; } .lists span.active{ display:inline-block; width: 0; height: 0; border: 5px solid transparent; /*设置边框无色*/ border-top-color: #333; margin-left: 2px; line-height: 40px; } .lists h2{ display: inline-block; font:500 20px/40px "宋体"; cursor: pointer; } .lists ul{ /* background-color: #00ffff; */ display: none; } .lists ul li{ border-bottom: 1px solid #000; border-top:none; } </style>
js部分:


<script> window.onload = function(){ var oQQ = document.getElementById('qq'); var aDiv = oQQ.getElementsByTagName('div'); var aUl = document.getElementsByTagName('ul'); var aLi = document.getElementsByTagName('li'); // console.log(aLi); var aSpan = oQQ.getElementsByTagName('span'); var aH2 = oQQ.getElementsByTagName('h2'); for(var i = 0;i< aDiv.length;i++){ aDiv[i].index = i; aDiv[i].onOff = true; aDiv[i].onclick = function(){ if(this.onOff){ for(var j = 0;j<aUl.length;j++){ aSpan[j].className = 'normal'; aUl[j].style.display = 'none'; aDiv[j].style.background = '#00ffff'; aDiv[j].onOff =true; } aUl[this.index].style.display = 'block'; aDiv[this.index].style.background = 'yellow'; aSpan[this.index].className = 'active'; aDiv[this.index].onOff = false; }else{ aUl[this.index].style.display = 'none'; aDiv[this.index].style.background = '#00ffff'; aSpan[this.index].className = 'normal'; aDiv[this.index].onOff = true; } } } for (var i = 0; i < aLi.length; i++) { aLi[i].index = i; aLi[i].onclick = function () { aLi[this.index].style.background = 'red'; for (var j = 0; j < aLi.length; j++) { aLi[j].style.background = ''; } aLi[this.index].style.background = 'red'; } } } </script>
整体代码:


1 <!DOCTYPE html> 2 <html lang="en"> 3 <head> 4 <meta charset="UTF-8"> 5 <meta name="viewport" content="width=device-width, initial-scale=1.0"> 6 <meta http-equiv="X-UA-Compatible" content="ie=edge"> 7 <title>Document</title> 8 <style> 9 *{ 10 margin:0; 11 padding: 0; 12 } 13 body{ 14 background: #000; 15 list-style: none; 16 } 17 .box{ 18 width: 255px; 19 height: 330px; 20 background-color: #fff; 21 margin: 155px auto; 22 position: relative; 23 } 24 .box .lists{ 25 border: 1px solid #000; 26 margin: 5px 8px 0 8px; 27 width: 239px; 28 position: absolute; 29 } 30 .lists div{ 31 background-color: #00ffff; 32 cursor: pointer; 33 } 34 .lists span.normal{ 35 display:inline-block; 36 width: 0; 37 height: 0; 38 border: 5px solid transparent; /*设置边框无色*/ 39 border-left-color: #333; 40 margin-left: 2px; 41 line-height: 40px; 42 } 43 .lists span.active{ 44 display:inline-block; 45 width: 0; 46 height: 0; 47 border: 5px solid transparent; /*设置边框无色*/ 48 border-top-color: #333; 49 margin-left: 2px; 50 line-height: 40px; 51 } 52 .lists h2{ 53 display: inline-block; 54 font:500 20px/40px "宋体"; 55 cursor: pointer; 56 } 57 .lists ul{ 58 /* background-color: #00ffff; */ 59 display: none; 60 } 61 .lists ul li{ 62 border-bottom: 1px solid #000; 63 border-top:none; 64 } 65 </style> 66 <script> 67 window.onload = function(){ 68 var oQQ = document.getElementById('qq'); 69 var aDiv = oQQ.getElementsByTagName('div'); 70 var aUl = document.getElementsByTagName('ul'); 71 var aLi = document.getElementsByTagName('li'); 72 // console.log(aLi); 73 var aSpan = oQQ.getElementsByTagName('span'); 74 var aH2 = oQQ.getElementsByTagName('h2'); 75 76 77 for(var i = 0;i< aDiv.length;i++){ 78 aDiv[i].index = i; 79 aDiv[i].onOff = true; 80 aDiv[i].onclick = function(){ 81 if(this.onOff){ 82 for(var j = 0;j<aUl.length;j++){ 83 aSpan[j].className = 'normal'; 84 aUl[j].style.display = 'none'; 85 aDiv[j].style.background = '#00ffff'; 86 aDiv[j].onOff =true; 87 } 88 aUl[this.index].style.display = 'block'; 89 aDiv[this.index].style.background = 'yellow'; 90 aSpan[this.index].className = 'active'; 91 aDiv[this.index].onOff = false; 92 }else{ 93 aUl[this.index].style.display = 'none'; 94 aDiv[this.index].style.background = '#00ffff'; 95 aSpan[this.index].className = 'normal'; 96 aDiv[this.index].onOff = true; 97 } 98 } 99 } 100 for (var i = 0; i < aLi.length; i++) { 101 aLi[i].index = i; 102 aLi[i].onclick = function () { 103 aLi[this.index].style.background = 'red'; 104 for (var j = 0; j < aLi.length; j++) { 105 aLi[j].style.background = ''; 106 } 107 aLi[this.index].style.background = 'red'; 108 } 109 110 } 111 112 } 113 </script> 114 </head> 115 <body> 116 <div class="box"> 117 <div class="lists" id="qq"> 118 <div id="div1"> 119 <span class="normal"></span> 120 <h2>我的好友</h2> 121 </div> 122 <ul> 123 <li>张三</li> 124 <li>张三</li> 125 <li>李三</li> 126 <li>李三</li> 127 </ul> 128 <div id="div2"> 129 <span class="normal"></span> 130 <h2>企业好友</h2> 131 </div> 132 <ul> 133 <li>李四</li> 134 <li>李四</li> 135 <li>李四</li> 136 </ul> 137 <div id="div3"> 138 <span class="normal"></span> 139 <h2>黑名单</h2> 140 </div> 141 <ul> 142 <li>王五</li> 143 <li>王五</li> 144 </ul> 145 146 </div> 147 </div> 148 </body> 149 </html>