Moving Tables
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submission(s): 15528 Accepted Submission(s): 5348
Problem Description
The famous ACM (Advanced Computer Maker) Company has rented a floor of a building whose shape is in the following figure.
The floor has 200 rooms each on the north side and south side along the corridor. Recently the Company made a plan to reform its system. The reform includes moving a lot of tables between rooms. Because the corridor is narrow and all the tables are big, only one table can pass through the corridor. Some plan is needed to make the moving efficient. The manager figured out the following plan: Moving a table from a room to another room can be done within 10 minutes. When moving a table from room i to room j, the part of the corridor between the front of room i and the front of room j is used. So, during each 10 minutes, several moving between two rooms not sharing the same part of the corridor will be done simultaneously. To make it clear the manager illustrated the possible cases and impossible cases of simultaneous moving.
For each room, at most one table will be either moved in or moved out. Now, the manager seeks out a method to minimize the time to move all the tables. Your job is to write a program to solve the manager’s problem.
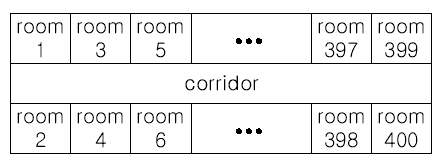
The floor has 200 rooms each on the north side and south side along the corridor. Recently the Company made a plan to reform its system. The reform includes moving a lot of tables between rooms. Because the corridor is narrow and all the tables are big, only one table can pass through the corridor. Some plan is needed to make the moving efficient. The manager figured out the following plan: Moving a table from a room to another room can be done within 10 minutes. When moving a table from room i to room j, the part of the corridor between the front of room i and the front of room j is used. So, during each 10 minutes, several moving between two rooms not sharing the same part of the corridor will be done simultaneously. To make it clear the manager illustrated the possible cases and impossible cases of simultaneous moving.
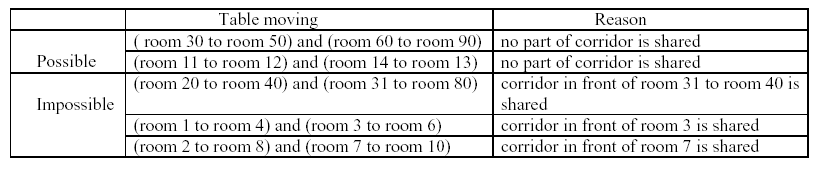
For each room, at most one table will be either moved in or moved out. Now, the manager seeks out a method to minimize the time to move all the tables. Your job is to write a program to solve the manager’s problem.
Input
The input consists of T test cases. The number of test cases ) (T is given in the first line of the input. Each test case begins with a line containing an integer N , 1<=N<=200 , that represents the number of tables to move. Each of the following N lines contains two positive integers s and t, representing that a table is to move from room number s to room number t (each room number appears at most once in the N lines). From the N+3-rd line, the remaining test cases are listed in the same manner as above.
Output
The output should contain the minimum time in minutes to complete the moving, one per line.
Sample Input
3 4 10 20 30 40 50 60 70 80 2 1 3 2 200 3 10 100 20 80 30 50
Sample Output
10 20 30
时间太晚了,困死了。题目大意改天在写吧。
思路见AC代码,细节很重要,调试一个多小时...
#include<stdio.h>
#include<string.h>
#include<algorithm>
using namespace std;
struct node
{
int begin; //记录每次输入的房间开始和终止
int end;
int cou; //这个房间的椅子是否搬过
};
node a[210];
//贪心原则:就是按照处理过的开始房间和终止房间位置,从小到大排序,如果起点相同,按终止从小到大排序。
// 从第一个房间依次向右扫描。扫描完后,接着与第一个相交房间号扫描...
// 复杂度为n^2...数据量比较小...够了。估计有O(n)算法...我是没想出来
bool cmp(node x,node y)
{
if(x.begin!=y.begin)
return x.begin<y.begin;
else
return x.end<y.end;
}
int main()
{
int t;
int n,i,j;
int c1,c2;
int en,ans,f,icou;
int sum,ccc;
int xxx;
scanf("%d",&t);
while(t--)
{
scanf("%d",&n);
for(i=0; i<n; i++) //处理输入数据时候得小心,WA一次,害的我调试1个小时...
{
xxx=1;
scanf("%d%d",&c1,&c2);
if(c1%2&&c2%2&&xxx) //如果都是奇数
{
c1=(c1+1)/2;
c2=(c2+1)/2;
xxx=0;
}
if(c1%2&&c2%2==0&&xxx) //如果c1的是奇数
{
c1=(c1+1)/2;
c2=c2/2;
xxx=0;
}
if(c1%2==0&&c2%2&&xxx) //如果c1的是偶数
{
c1=c1/2;
c2=(c2+1)/2;
xxx=0;
}
if(c1%2==0&&c2%2==0&&xxx) //如果都是偶数
{
c1=c1/2;
c2=c2/2;
xxx=0;
}
if(c1>c2) //不容忽视哦~~~
{
c1=c1+c2;
c2=c1-c2;
c1=c1-c2;
}
a[i].begin=c1;
a[i].end=c2;
a[i].cou=0;
}
sort(a,a+n,cmp);
ans=1; //需要的时间
a[0].cou=1; //记录区间已经访问
sum=1; //访问的区间数
ccc=0; //是否全部访问
icou=0; //标记与当前房价号相交的房间位置,即下一次扫描的开始房间
for(j=0; j<n; j++)
{
if(ccc)
break;
en=a[icou].end;
f=1;
for(i=1; i<n; i++)
{
if(a[i].begin>en&&a[i].cou==0) //扫描,注意判断区间是否用过
{
en=a[i].end;
a[i].cou=1;
sum++;
if(sum==n) //所有区间是否被扫描
{
ccc=1;
break;
}
}
else
{
if(f&&a[i].cou==0) //注意判断区间是否用过
{
icou=i; //记录下第一个相交区间位置
a[i].cou=1;
f=0;
ans++; //别忘总数++
sum++;
if(sum==n) //所有区间是否被扫描
{
ccc=1;
break;
}
}
}
}
}
printf("%d\n",ans*10);
}
return 0;
}
今天又重新看看,顺便补充一下题意.参考其他人,发现有种思路好实现。
题目大意:一个走廊两旁全是房间的公司,需要搬桌子。规则如下:走廊太小,一次只能一个桌子过去。从i房间到j房间需要10分钟。在i房间搬到j房间过程中,这个段走廊被占用,其他搬桌子的不允许通过。但不影响其他区域房间搬桌子。问把n个房间桌子搬走最少需要多少时间。
另一种AC代码:
#include<stdio.h>
#include<string.h>
#include<algorithm>
using namespace std;
int main()
{
int a[210];
int t;
int n,i,j;
int s,e;
int min;
scanf("%d",&t);
while(t--)
{ //贪心原则:就是求每个走廊的最多覆盖区间.
memset(a,0,sizeof(a)); //记得初始化
scanf("%d",&n);
for(i=0;i<n;i++) //复杂度同样N^2...
{
scanf("%d%d",&s,&e);
s=(s-1)/2;
e=(e-1)/2;
if(s>e)
{
s=s+e;
e=s-e;
s=s-e;
}
//记录每个走廊的覆盖区间数目
for(j=s;j<=e;j++)
{
a[j]=a[j]+1;
}
}
min=0;
for(i=0;i<200;i++)
{ //求走廊的最大覆盖区间
if(a[i]>min)
{
min=a[i];
}
}
printf("%d\n",min*10);
}
return 0;
}