完整原文地址见简书https://www.jianshu.com/p/8fa37a3c8e6e
createApp()、mount()、MVVM、根组件实例
-【createApp()】Vue.createApp()
,创建Vue应用实例,开始使用Vue;
-【mount()】.mount()
指定在哪个组件上使用Vue
(这个在《Vue3 | 基本特性概念 与语法的 应用与案例》中已经讲过了);
-传入createApp()
的参数,描述了这个Vue应用实例的展示内容,包括数据、UI、各种事件等;
-【MVVM】
我们说Vue架构使用了MVVM的设计思想,其中data()
是M层,template
是V层,
VM层视图数据连接层,由Vue组件
帮我们完成;
-【Vue应用实例
的根组件
实例】
以下代码中,const vm = heheApp.mount('#root_div');
这里,vm
接收的组件实例,就是这个Vue应用实例
的根组件
;
我们可以使用这个根组件实例
访问到组件内相关的 数据(data)等字段(详见下面案例):
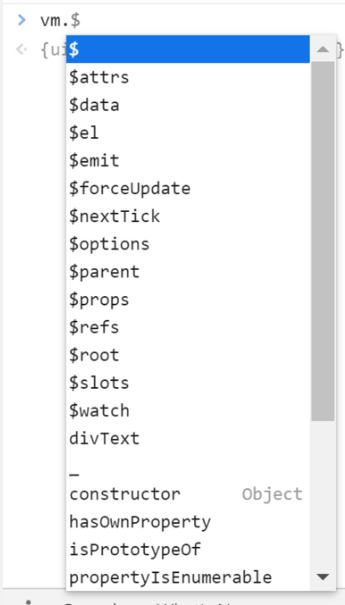
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello World! heheheheheheda</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<div id="root_div"></div>
</body>
<script>
const heheApp = Vue.createApp({
data(){
return{
divText:'hehehedadada'
}
},
template:`
<div>{{divText}}</div>
`
});
const vm = heheApp.mount('#root_div');
</script>
</html>
运行效果:
![]()
可以打开控制台进行终端操作:
输入刚刚案例代码中的Vue实例(
heheApp
),查看实例内容:查看![]()
根组件实例vm
及其内容:
查看vm的字段:
查看vm的data字段:
对data中的![]()
数据字段
赋值,
触发Vue的ViewModel层效果,UI数据双向绑定,
使得绑定这个数据字段
的对应的UI
发生改变:再尝试:![]()
![]()
生命周期
先贴上案例代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello World! heheheheheheda</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<div id="heheApp">
<h1>{{heheString + ' ———— string in outer HTML'}}</h1>
</div>
</body>
<script>
var vm = Vue.createApp({
el:'#heheApp',
data() {
return{
heheString: 'Vue的生命周期'
}
},
beforeCreate: function() {
console.group('------beforeCreate创建前状态------');
console.log("%c%s", "color:red" , "el : " + this.$el); //undefined
console.log("%c%s", "color:red","data : " + this.$data); //undefined
console.log("%c%s", "color:red","heheString: " + this.heheString)
},
created: function() {
console.group('------created创建完毕状态------');
console.log("%c%s", "color:red","el : " + this.$el); //undefined
console.log("%c%s", "color:red","data : " + this.$data); //已被初始化
console.log("%c%s", "color:red","heheString: " + this.heheString); //已被初始化
},
beforeMount: function() {
console.group('------beforeMount挂载前状态------');
console.log("%c%s", "color:red","el : " + (this.$el)); //已被初始化
console.log(this.$el);
console.log("%c%s", "color:red","data : " + this.$data); //已被初始化
console.log("%c%s", "color:red","heheString: " + this.heheString); //已被初始化
console.log("%c%s", "color:red",
document.getElementById('heheApp').innerHTML, " ———— beforeMount");
},
mounted: function() {
console.group('------mounted 挂载结束状态------');
console.log("%c%s", "color:red","el : " + this.$el); //已被初始化
console.log(this.$el);
console.log("%c%s", "color:red","data : " + this.$data); //已被初始化
console.log("%c%s", "color:red","heheString: " + this.heheString); //已被初始化
console.log("%c%s", "color:red",
document.getElementById('heheApp').innerHTML, " ———— mounted");
},
beforeUpdate: function () {
console.group('beforeUpdate 更新前状态===============》');
console.log("%c%s", "color:red","el : " + this.$el);
console.log(this.$el);
console.log("%c%s", "color:red","data : " + this.$data);
console.log("%c%s", "color:red","heheString: " + this.heheString);
console.log("%c%s", "color:red",
document.getElementById('heheApp').innerHTML, " ———— beforeUpdate");
},
updated: function () {
console.group('updated 更新完成状态===============》');
console.log("%c%s", "color:red","el : " + this.$el);
console.log(this.$el);
console.log("%c%s", "color:red","data : " + this.$data);
console.log("%c%s", "color:red","heheString: " + this.heheString);
console.log("%c%s", "color:red",
document.getElementById('heheApp').innerHTML, " ———— updated");
},
beforeUnmount: function () {
console.group('beforeDestroy 销毁前状态===============》');
console.log("%c%s", "color:red","el : " + this.$el);
console.log(this.$el);
console.log("%c%s", "color:red","data : " + this.$data);
console.log("%c%s", "color:red","heheString: " + this.heheString);
console.log("%c%s", "color:red",
document.getElementById('heheApp').innerHTML, " ———— beforeUnmount");
},
unmounted: function () {
console.group('destroyed 销毁完成状态===============》');
console.log("%c%s", "color:red","el : " + this.$el);
console.log(this.$el);
console.log("%c%s", "color:red","data : " + this.$data);
console.log("%c%s", "color:red","heheString: " + this.heheString);
console.log("%c%s", "color:red",
document.getElementById('heheApp').innerHTML, " ———— unmounted");
},
template: "<h1>{{this.heheString +' ———— string in template'}}</h1>"
})
const vmR = vm.mount('#heheApp');
</script>
</html>
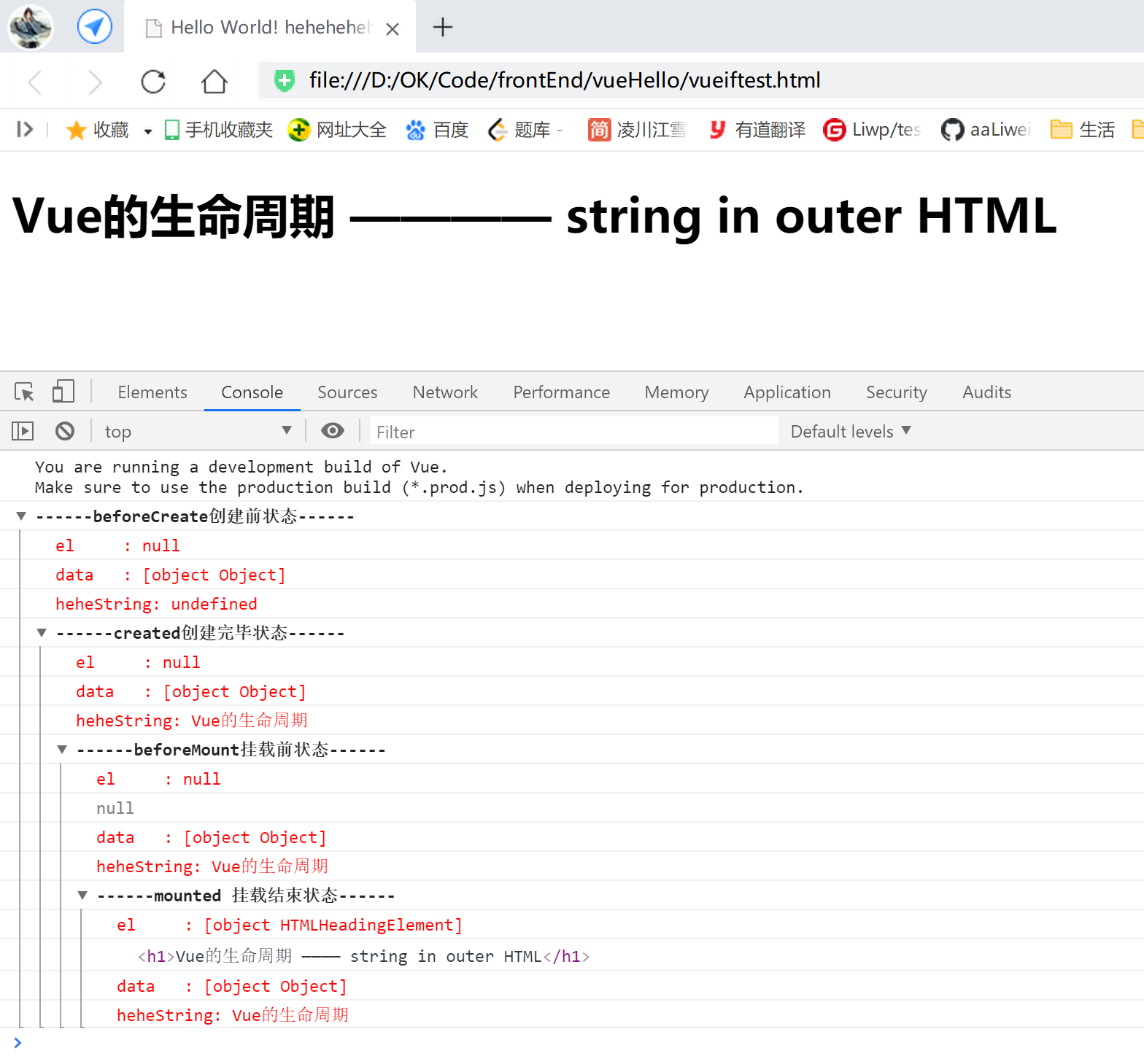