根据数据库的设计,编写相应的Model对象
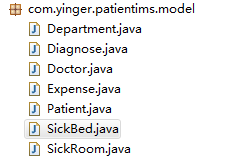
病人Patient类
package com.yinger.patientims.model;
import java.util.Date;
public class Patient {
private Long id; // id编号,对应数据库中bigint
private String name; // 姓名,varchar
private String sex; // 性别,varchar
private int age; // 年龄,int
private String phone; // 电话,varchar
private Date logtime; // 登记时间,datetime
private String address; // 地址,varchar
private SickBed sickBed = new SickBed(); // 病床,bigint
private SickRoom sickRoom = new SickRoom(); // 病房
private Doctor doctor = new Doctor(); // 医生
private Diagnose diagnose = new Diagnose(); // 诊断
private Department department = new Department(); // 科室
private Expense expense = new Expense(); // 费用
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge( int age) {
this.age = age;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public Date getLogtime() {
return logtime;
}
public void setLogtime(Date logtime) {
this.logtime = logtime;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public SickBed getSickbed() {
return sickBed;
}
public void setSickbed(SickBed sickbed) {
this.sickBed = sickbed;
}
public SickRoom getSickroom() {
return sickRoom;
}
public void setSickroom(SickRoom sickroom) {
this.sickRoom = sickroom;
}
public Doctor getDoctor() {
return doctor;
}
public void setDoctor(Doctor doctor) {
this.doctor = doctor;
}
public Diagnose getDiagnose() {
return diagnose;
}
public void setDiagnose(Diagnose diagnose) {
this.diagnose = diagnose;
}
public Department getDepartment() {
return department;
}
public void setDepartment(Department department) {
this.department = department;
}
public Expense getExpense() {
return expense;
}
public void setExpense(Expense expense) {
this.expense = expense;
}
}
import java.util.Date;
public class Patient {
private Long id; // id编号,对应数据库中bigint
private String name; // 姓名,varchar
private String sex; // 性别,varchar
private int age; // 年龄,int
private String phone; // 电话,varchar
private Date logtime; // 登记时间,datetime
private String address; // 地址,varchar
private SickBed sickBed = new SickBed(); // 病床,bigint
private SickRoom sickRoom = new SickRoom(); // 病房
private Doctor doctor = new Doctor(); // 医生
private Diagnose diagnose = new Diagnose(); // 诊断
private Department department = new Department(); // 科室
private Expense expense = new Expense(); // 费用
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge( int age) {
this.age = age;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public Date getLogtime() {
return logtime;
}
public void setLogtime(Date logtime) {
this.logtime = logtime;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public SickBed getSickbed() {
return sickBed;
}
public void setSickbed(SickBed sickbed) {
this.sickBed = sickbed;
}
public SickRoom getSickroom() {
return sickRoom;
}
public void setSickroom(SickRoom sickroom) {
this.sickRoom = sickroom;
}
public Doctor getDoctor() {
return doctor;
}
public void setDoctor(Doctor doctor) {
this.doctor = doctor;
}
public Diagnose getDiagnose() {
return diagnose;
}
public void setDiagnose(Diagnose diagnose) {
this.diagnose = diagnose;
}
public Department getDepartment() {
return department;
}
public void setDepartment(Department department) {
this.department = department;
}
public Expense getExpense() {
return expense;
}
public void setExpense(Expense expense) {
this.expense = expense;
}
}
医生Doctor类
package com.yinger.patientims.model;
import java.util.Date;
public class Doctor {
private Long id; // id编号,bigint
private String name; // 姓名
private String title; // 头衔,职称
private String username; // 用户名
private String password; // 密码
private int age; // 年龄
private String sex; // 性别
private String phone; // 电话
private Date logtime; // 登记时间
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public int getAge() {
return age;
}
public void setAge( int age) {
this.age = age;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public Date getLogtime() {
return logtime;
}
public void setLogtime(Date logtime) {
this.logtime = logtime;
}
}
import java.util.Date;
public class Doctor {
private Long id; // id编号,bigint
private String name; // 姓名
private String title; // 头衔,职称
private String username; // 用户名
private String password; // 密码
private int age; // 年龄
private String sex; // 性别
private String phone; // 电话
private Date logtime; // 登记时间
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public int getAge() {
return age;
}
public void setAge( int age) {
this.age = age;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public Date getLogtime() {
return logtime;
}
public void setLogtime(Date logtime) {
this.logtime = logtime;
}
}
科室类
package com.yinger.patientims.model;
public class Department {
private Long id; // id编号,bigint
private String name; // 名称,varchar
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
public class Department {
private Long id; // id编号,bigint
private String name; // 名称,varchar
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
诊断类
package com.yinger.patientims.model;
import java.util.Date;
public class Diagnose {
private Long id; //id编号,bigint
private String illness; //诊断结果
private String treatment; //诊断方案
private Date diagnoseDate; //诊断时间
private Patient patient; //病人
private Doctor doctor; //医生
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getIllness() {
return illness;
}
public void setIllness(String illness) {
this.illness = illness;
}
public String getTreatment() {
return treatment;
}
public void setTreatment(String treatment) {
this.treatment = treatment;
}
public Date getDiagnoseDate() {
return diagnoseDate;
}
public void setDiagnoseDate(Date diagnoseDate) {
this.diagnoseDate = diagnoseDate;
}
public Patient getPatient() {
return patient;
}
public void setPatient(Patient patient) {
this.patient = patient;
}
public Doctor getDoctor() {
return doctor;
}
public void setDoctor(Doctor doctor) {
this.doctor = doctor;
}
}
import java.util.Date;
public class Diagnose {
private Long id; //id编号,bigint
private String illness; //诊断结果
private String treatment; //诊断方案
private Date diagnoseDate; //诊断时间
private Patient patient; //病人
private Doctor doctor; //医生
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getIllness() {
return illness;
}
public void setIllness(String illness) {
this.illness = illness;
}
public String getTreatment() {
return treatment;
}
public void setTreatment(String treatment) {
this.treatment = treatment;
}
public Date getDiagnoseDate() {
return diagnoseDate;
}
public void setDiagnoseDate(Date diagnoseDate) {
this.diagnoseDate = diagnoseDate;
}
public Patient getPatient() {
return patient;
}
public void setPatient(Patient patient) {
this.patient = patient;
}
public Doctor getDoctor() {
return doctor;
}
public void setDoctor(Doctor doctor) {
this.doctor = doctor;
}
}
费用类
package com.yinger.patientims.model;
import java.util.Date;
public class Expense {
private Long id; //id编号,bigint
private String expenseDesc; //费用说明
private String expenseName; //费用名称
private float unitPrice; //单价
private int number; //数量
private float expenseSum; //实际的费用
private Date takeDate; //费用发生时间
private Patient patient; //病人
private Doctor doctor; //医生
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getExpenseDesc() {
return expenseDesc;
}
public void setExpenseDesc(String expenseDesc) {
this.expenseDesc = expenseDesc;
}
public String getExpenseName() {
return expenseName;
}
public void setExpenseName(String expenseName) {
this.expenseName = expenseName;
}
public float getUnitPrice() {
return unitPrice;
}
public void setUnitPrice( float unitPrice) {
this.unitPrice = unitPrice;
}
public int getNumber() {
return number;
}
public void setNumber( int number) {
this.number = number;
}
public float getExpenseSum() {
return expenseSum;
}
public void setExpenseSum( float expenseSum) {
this.expenseSum = expenseSum;
}
public Date getTakeDate() {
return takeDate;
}
public void setTakeDate(Date takeDate) {
this.takeDate = takeDate;
}
public Patient getPatient() {
return patient;
}
public void setPatient(Patient patient) {
this.patient = patient;
}
public Doctor getDoctor() {
return doctor;
}
public void setDoctor(Doctor doctor) {
this.doctor = doctor;
}
}
import java.util.Date;
public class Expense {
private Long id; //id编号,bigint
private String expenseDesc; //费用说明
private String expenseName; //费用名称
private float unitPrice; //单价
private int number; //数量
private float expenseSum; //实际的费用
private Date takeDate; //费用发生时间
private Patient patient; //病人
private Doctor doctor; //医生
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getExpenseDesc() {
return expenseDesc;
}
public void setExpenseDesc(String expenseDesc) {
this.expenseDesc = expenseDesc;
}
public String getExpenseName() {
return expenseName;
}
public void setExpenseName(String expenseName) {
this.expenseName = expenseName;
}
public float getUnitPrice() {
return unitPrice;
}
public void setUnitPrice( float unitPrice) {
this.unitPrice = unitPrice;
}
public int getNumber() {
return number;
}
public void setNumber( int number) {
this.number = number;
}
public float getExpenseSum() {
return expenseSum;
}
public void setExpenseSum( float expenseSum) {
this.expenseSum = expenseSum;
}
public Date getTakeDate() {
return takeDate;
}
public void setTakeDate(Date takeDate) {
this.takeDate = takeDate;
}
public Patient getPatient() {
return patient;
}
public void setPatient(Patient patient) {
this.patient = patient;
}
public Doctor getDoctor() {
return doctor;
}
public void setDoctor(Doctor doctor) {
this.doctor = doctor;
}
}
病房类
package com.yinger.patientims.model;
public class SickRoom {
private Long id; //id编号,bigint
private int sickRoomNo; //病房编号,int
private Department department = new Department(); //科室,病房所在的科室,bigint
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public int getSickRoomNo() {
return sickRoomNo;
}
public void setSickRoomNo( int sickRoomNo) {
this.sickRoomNo = sickRoomNo;
}
public Department getDepartment() {
return department;
}
public void setDepartment(Department department) {
this.department = department;
}
}
public class SickRoom {
private Long id; //id编号,bigint
private int sickRoomNo; //病房编号,int
private Department department = new Department(); //科室,病房所在的科室,bigint
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public int getSickRoomNo() {
return sickRoomNo;
}
public void setSickRoomNo( int sickRoomNo) {
this.sickRoomNo = sickRoomNo;
}
public Department getDepartment() {
return department;
}
public void setDepartment(Department department) {
this.department = department;
}
}
病床类
package com.yinger.patientims.model;
public class SickBed {
private Long id; //id编号,bigint
private int sickBedNo; //病床编号,int
private SickRoom sickRoom = new SickRoom(); //病房,病床所在的病房
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public int getSickBedNo() {
return sickBedNo;
}
public void setSickBedNo( int sickBedNo) {
this.sickBedNo = sickBedNo;
}
public SickRoom getSickRoom() {
return sickRoom;
}
public void setSickRoom(SickRoom sickRoom) {
this.sickRoom = sickRoom;
}
}
public class SickBed {
private Long id; //id编号,bigint
private int sickBedNo; //病床编号,int
private SickRoom sickRoom = new SickRoom(); //病房,病床所在的病房
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public int getSickBedNo() {
return sickBedNo;
}
public void setSickBedNo( int sickBedNo) {
this.sickBedNo = sickBedNo;
}
public SickRoom getSickRoom() {
return sickRoom;
}
public void setSickRoom(SickRoom sickRoom) {
this.sickRoom = sickRoom;
}
}