// calculate 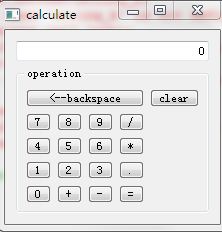
1 # coding = gbk
2 import sys
3 from PyQt4 import QtCore,QtGui
4
5 class Button(QtGui.QToolButton):
6 def __init__ (self,text,parent = None):
7 super(Button,self). __init__ (parent)
8 self.setSizePolicy(QtGui.QSizePolicy.Expanding,QtGui.QSizePolicy.Preferred)
9 self.setText(text)
10
11 class Calculate(QtGui.QWidget):
12 number_digit_buttons = 10
13 def __init__ (self,parent = None):
14 super(Calculate,self). __init__ (parent)
15 self.setWindowTitle( ' calculate ' )
16
17 self.x = ''
18 self.y = ''
19 self.sum_sofar = 0.0
20 self.factor_sofar = 0.0
21 self.wait_for_operator = True
22
23 self.line_edit = QtGui.QLineEdit( ' 0 ' )
24 self.line_edit.setAlignment(QtCore.Qt.AlignRight)
25 self.line_edit.setReadOnly(True)
26 self.line_edit.setMaxLength( 12 )
27
28 self.group_box = QtGui.QGroupBox()
29 self.group_box.setTitle( ' operation ' )
30
31 self.digit_buttons = []
32 for i in range(Calculate.number_digit_buttons):
33 self.digit_buttons.append(self.create_button(str(i),self.digit_clicked))
34
35 self.point_button = self.create_button( ' . ' ,self.point_clicked)
36 self.add_button = self.create_button( ' + ' ,self.additive)
37 self.cut_button = self.create_button( ' - ' ,self.additive)
38 self.times_button = self.create_button( ' * ' ,self.multiple)
39 self.division_button = self.create_button( ' / ' ,self.multiple)
40 self.equal_button = self.create_button( ' = ' ,self.equal_clicked)
41 self.backspace_button = self.create_button( ' <--backspace ' ,self.backspace_clicked)
42 # self.button = self.create_button('5',self.digit_clicked)
43 self.clear_button = self.create_button( ' clear ' ,self.clear)
44
45 self.layout_x = QtGui.QGridLayout()
46 self.layout_x.setSizeConstraint(QtGui.QLayout.SetFixedSize)
47
48
49 for i in range( 1 ,Calculate.number_digit_buttons):
50 row = (( 9 - i) / 3 ) + 1
51 column = ((i - 1 ) % 3 )
52 self.layout_x.addWidget(self.digit_buttons[i],row,column)
53 self.layout_x.addWidget(self.point_button, 3 , 3 )
54 self.layout_x.addWidget(self.digit_buttons[0], 4 ,0)
55 self.layout_x.addWidget(self.add_button, 4 , 1 )
56 self.layout_x.addWidget(self.cut_button, 4 , 2 )
57 self.layout_x.addWidget(self.times_button, 2 , 3 )
58 self.layout_x.addWidget(self.division_button, 1 , 3 )
59 self.layout_x.addWidget(self.equal_button, 4 , 3 )
60 self.layout_x.addWidget(self.backspace_button,0,0, 1 , 4 )
61 self.layout_x.addWidget(self.clear_button,0, 4 )
62
63 self.group_box.setLayout(self.layout_x)
64
65
66 self.layout = QtGui.QVBoxLayout()
67 self.layout.addWidget(self.line_edit)
68 self.layout.addWidget(self.group_box)
69 # self.layout.addWidget(self.button)
70
71 self.setLayout(self.layout)
72 def backspace_clicked(self):
73 if self.wait_for_operator:
74 return
75 string_a = self.line_edit.text()
76 string_b = string_a[ 1 :]
77 if not string_b:
78 string_b = ' 0 '
79 self.wait_for_operator = True
80 self.line_edit.setText(string_b)
81 def multiple(self):
82 pass
83 def digit_clicked(self):
84 clicked_button = self.sender()
85 digit_value = int(clicked_button.text())
86 if self.line_edit.text() == ' 0 ' and digit_value == 0.0 :
87 return
88 if self.wait_for_operator:
89 self.line_edit.clear()
90 self.wait_for_operator = False
91 self.line_edit.setText(self.line_edit.text() + str(digit_value))
92 def create_button(self,text,function):
93 # button = QtGui.QToolButton()
94 button = Button(text)
95 button.clicked.connect(function)
96 return button
97 def point_clicked(self):
98 if self.wait_for_operator:
99 self.line_edit.setText( ' 0 ' )
100 if ' . ' not in self.line_edit.text():
101 self.line_edit.setText(self.line_edit.text() + ' . ' )
102 self.wait_for_operator = False
103 def additive(self):
104 clicked_button = self.sender()
105 clicked_operator = clicked_button.text()
106 operator = float(self.line_edit.text())
107
108 # # if self.x:
109 # # if not self.calculate(operator,self.x):
110 # # self.abort_operation()
111 # # return
112 # # self.line_edit.setText(str(self.factor_sofar))
113 # # operator = self.factor_sofar
114 # # self.factor_sofar = 0.0
115 # # self.x = ''
116 if self.y:
117 if not self.calculate(operator,self.y):
118 self.abort_operation()
119 return
120 self.line_edit.setText(str(self.sum_sofar))
121 else :
122 self.sum_sofar = operator
123 self.y = clicked_operator
124 self.wait_for_operator = True
125 def equal_clicked(self):
126 operator = float(self.line_edit.text())
127 if self.y:
128 if not self.calculate(operator,self.y):
129 self.abort_operation()
130 return
131 # self.line_edit.setText(str(self.sum_sofar))
132 self.y = ''
133 else :
134 self.sum_sofar = operator
135 # self.y = clicked_operator
136 self.line_edit.setText(str(self.sum_sofar))
137 self.sum_sofar = 0.0
138 self.wait_for_operator = True
139
140 def calculate(self,number,tem):
141 if tem == ' + ' :
142 self.sum_sofar += number
143 elif tem == ' - ' :
144 self.sum_sofar -= number
145 return True
146 def abort_operation(self):
147 self.clear_all()
148 self.line_edit.setText( ' #### ' )
149 def clear_all(self):
150 self.sum_sofar = 0.0
151 self.factor_sofar = 0.0
152 self.x = ''
153 self.y = ''
154 self.line_edit.setText( ' 0 ' )
155 self.wait_for_operator = True
156 def clear(self):
157 if self.wait_for_operator:
158 return
159 self.line_edit.setText( ' 0 ' )
160 self.wait_for_operator = True
161
162 if __name__ == ' __main__ ' :
163 app = QtGui.QApplication(sys.argv)
164 calculate = Calculate()
165 calculate.show()
166 sys.exit(app.exec_())
// reference example from pyqt_example
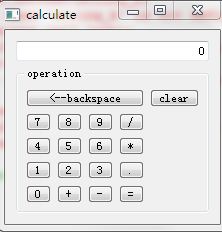
1 # coding = gbk
2 import sys
3 from PyQt4 import QtCore,QtGui
4
5 class Button(QtGui.QToolButton):
6 def __init__ (self,text,parent = None):
7 super(Button,self). __init__ (parent)
8 self.setSizePolicy(QtGui.QSizePolicy.Expanding,QtGui.QSizePolicy.Preferred)
9 self.setText(text)
10
11 class Calculate(QtGui.QWidget):
12 number_digit_buttons = 10
13 def __init__ (self,parent = None):
14 super(Calculate,self). __init__ (parent)
15 self.setWindowTitle( ' calculate ' )
16
17 self.x = ''
18 self.y = ''
19 self.sum_sofar = 0.0
20 self.factor_sofar = 0.0
21 self.wait_for_operator = True
22
23 self.line_edit = QtGui.QLineEdit( ' 0 ' )
24 self.line_edit.setAlignment(QtCore.Qt.AlignRight)
25 self.line_edit.setReadOnly(True)
26 self.line_edit.setMaxLength( 12 )
27
28 self.group_box = QtGui.QGroupBox()
29 self.group_box.setTitle( ' operation ' )
30
31 self.digit_buttons = []
32 for i in range(Calculate.number_digit_buttons):
33 self.digit_buttons.append(self.create_button(str(i),self.digit_clicked))
34
35 self.point_button = self.create_button( ' . ' ,self.point_clicked)
36 self.add_button = self.create_button( ' + ' ,self.additive)
37 self.cut_button = self.create_button( ' - ' ,self.additive)
38 self.times_button = self.create_button( ' * ' ,self.multiple)
39 self.division_button = self.create_button( ' / ' ,self.multiple)
40 self.equal_button = self.create_button( ' = ' ,self.equal_clicked)
41 self.backspace_button = self.create_button( ' <--backspace ' ,self.backspace_clicked)
42 # self.button = self.create_button('5',self.digit_clicked)
43 self.clear_button = self.create_button( ' clear ' ,self.clear)
44
45 self.layout_x = QtGui.QGridLayout()
46 self.layout_x.setSizeConstraint(QtGui.QLayout.SetFixedSize)
47
48
49 for i in range( 1 ,Calculate.number_digit_buttons):
50 row = (( 9 - i) / 3 ) + 1
51 column = ((i - 1 ) % 3 )
52 self.layout_x.addWidget(self.digit_buttons[i],row,column)
53 self.layout_x.addWidget(self.point_button, 3 , 3 )
54 self.layout_x.addWidget(self.digit_buttons[0], 4 ,0)
55 self.layout_x.addWidget(self.add_button, 4 , 1 )
56 self.layout_x.addWidget(self.cut_button, 4 , 2 )
57 self.layout_x.addWidget(self.times_button, 2 , 3 )
58 self.layout_x.addWidget(self.division_button, 1 , 3 )
59 self.layout_x.addWidget(self.equal_button, 4 , 3 )
60 self.layout_x.addWidget(self.backspace_button,0,0, 1 , 4 )
61 self.layout_x.addWidget(self.clear_button,0, 4 )
62
63 self.group_box.setLayout(self.layout_x)
64
65
66 self.layout = QtGui.QVBoxLayout()
67 self.layout.addWidget(self.line_edit)
68 self.layout.addWidget(self.group_box)
69 # self.layout.addWidget(self.button)
70
71 self.setLayout(self.layout)
72 def backspace_clicked(self):
73 if self.wait_for_operator:
74 return
75 string_a = self.line_edit.text()
76 string_b = string_a[ 1 :]
77 if not string_b:
78 string_b = ' 0 '
79 self.wait_for_operator = True
80 self.line_edit.setText(string_b)
81 def multiple(self):
82 pass
83 def digit_clicked(self):
84 clicked_button = self.sender()
85 digit_value = int(clicked_button.text())
86 if self.line_edit.text() == ' 0 ' and digit_value == 0.0 :
87 return
88 if self.wait_for_operator:
89 self.line_edit.clear()
90 self.wait_for_operator = False
91 self.line_edit.setText(self.line_edit.text() + str(digit_value))
92 def create_button(self,text,function):
93 # button = QtGui.QToolButton()
94 button = Button(text)
95 button.clicked.connect(function)
96 return button
97 def point_clicked(self):
98 if self.wait_for_operator:
99 self.line_edit.setText( ' 0 ' )
100 if ' . ' not in self.line_edit.text():
101 self.line_edit.setText(self.line_edit.text() + ' . ' )
102 self.wait_for_operator = False
103 def additive(self):
104 clicked_button = self.sender()
105 clicked_operator = clicked_button.text()
106 operator = float(self.line_edit.text())
107
108 # # if self.x:
109 # # if not self.calculate(operator,self.x):
110 # # self.abort_operation()
111 # # return
112 # # self.line_edit.setText(str(self.factor_sofar))
113 # # operator = self.factor_sofar
114 # # self.factor_sofar = 0.0
115 # # self.x = ''
116 if self.y:
117 if not self.calculate(operator,self.y):
118 self.abort_operation()
119 return
120 self.line_edit.setText(str(self.sum_sofar))
121 else :
122 self.sum_sofar = operator
123 self.y = clicked_operator
124 self.wait_for_operator = True
125 def equal_clicked(self):
126 operator = float(self.line_edit.text())
127 if self.y:
128 if not self.calculate(operator,self.y):
129 self.abort_operation()
130 return
131 # self.line_edit.setText(str(self.sum_sofar))
132 self.y = ''
133 else :
134 self.sum_sofar = operator
135 # self.y = clicked_operator
136 self.line_edit.setText(str(self.sum_sofar))
137 self.sum_sofar = 0.0
138 self.wait_for_operator = True
139
140 def calculate(self,number,tem):
141 if tem == ' + ' :
142 self.sum_sofar += number
143 elif tem == ' - ' :
144 self.sum_sofar -= number
145 return True
146 def abort_operation(self):
147 self.clear_all()
148 self.line_edit.setText( ' #### ' )
149 def clear_all(self):
150 self.sum_sofar = 0.0
151 self.factor_sofar = 0.0
152 self.x = ''
153 self.y = ''
154 self.line_edit.setText( ' 0 ' )
155 self.wait_for_operator = True
156 def clear(self):
157 if self.wait_for_operator:
158 return
159 self.line_edit.setText( ' 0 ' )
160 self.wait_for_operator = True
161
162 if __name__ == ' __main__ ' :
163 app = QtGui.QApplication(sys.argv)
164 calculate = Calculate()
165 calculate.show()
166 sys.exit(app.exec_())
// reference example from pyqt_example