原型设计模式(克隆一个苹果)
0. UML结构图
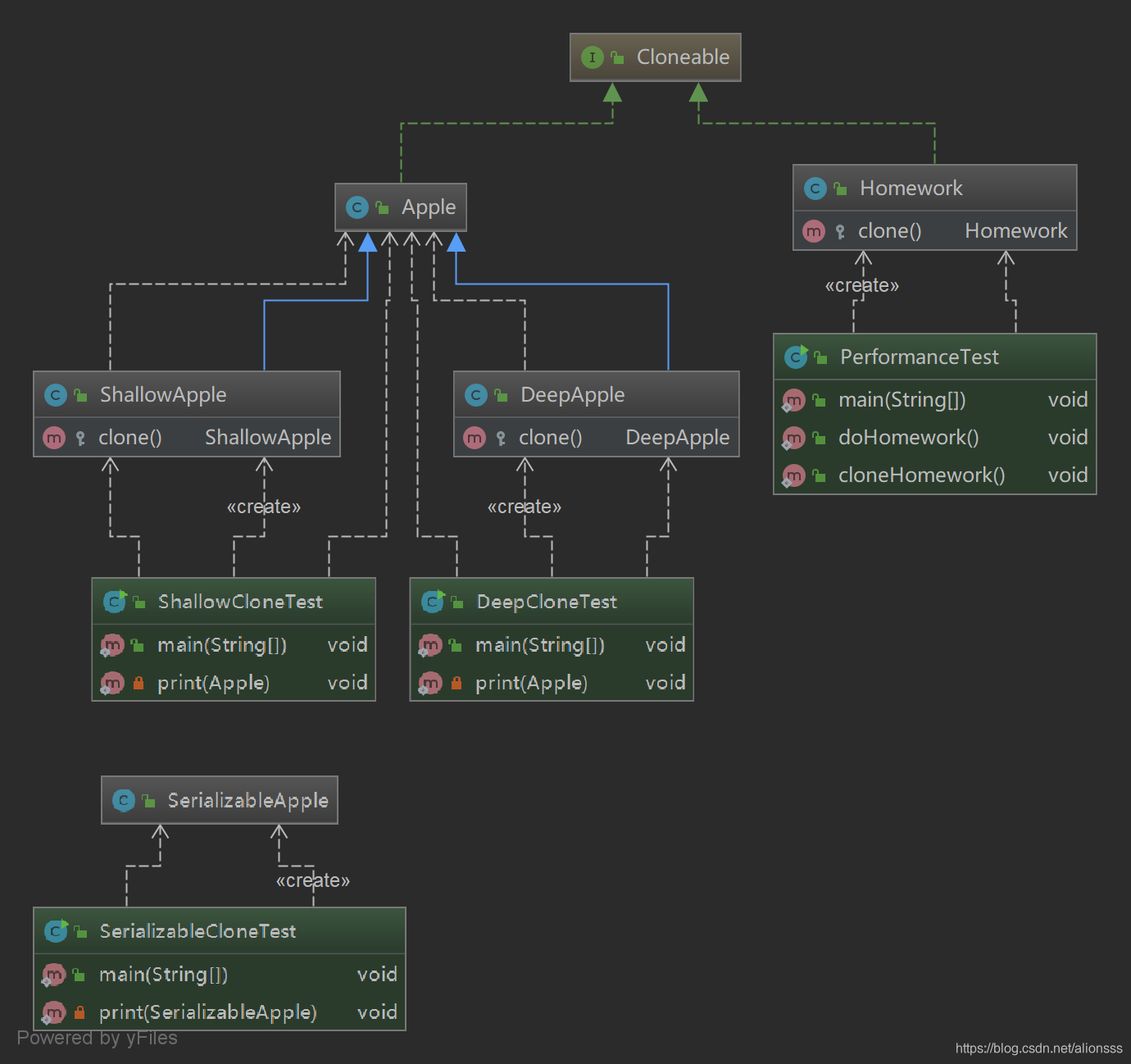
1. Code 克隆用的Apple类
1.1 苹果基类
import java.util.Date;
public class Apple implements Cloneable {
protected int size;
protected String place;
protected Date manufacture;
public Apple(int size, String place, Date manufacture) {
this.size = size;
this.place = place;
this.manufacture = manufacture;
}
}
1.2 可克隆的苹果(用于浅克隆)
import java.util.Date;
public class ShallowApple extends Apple {
public ShallowApple(int size, String place, Date manufacture) {
super(size, place, manufacture);
}
@Override
protected ShallowApple clone() throws CloneNotSupportedException {
return (ShallowApple) super.clone();
}
}
1.3 可克隆的苹果(用于深克隆)
import java.util.Date;
public class DeepApple extends Apple {
public DeepApple(int size, String place, Date manufacture) {
super(size, place, manufacture);
}
@Override
protected DeepApple clone() throws CloneNotSupportedException {
DeepApple apple = (DeepApple) super.clone();
apple.manufacture = (Date) this.manufacture.clone();
return apple;
}
}
1.4 可序列化的苹果(用于深克隆)
import java.io.Serializable;
import java.util.Date;
public class SerializableApple implements Serializable {
public int size;
public String place;
public Date manufacture;
public SerializableApple(int size, String place, Date manufacture) {
this.size = size;
this.place = place;
this.manufacture = manufacture;
}
}
1.5 家庭作业类(用于测试原型模式性能)
public class Homework implements Cloneable{
public Homework() {
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
@Override
protected Homework clone() throws CloneNotSupportedException {
return (Homework) super.clone();
}
}
2. 克隆Test
2.1 浅克隆测试
import java.util.Date;
public class ShallowCloneTest {
public static void main(String[] args) throws CloneNotSupportedException {
System.out.println("------+--浅克隆Test--+------");
ShallowApple apple = new ShallowApple(10, "China", new Date());
print(apple);
System.out.println("------+------+------");
ShallowApple cloneApple = apple.clone();
print(cloneApple);
System.out.println("修改manufacture");
apple.manufacture.setTime(10000000000L);
System.out.println("apple.manufacture = " + apple.manufacture);
System.out.println("cloneApple.manufacture = " + cloneApple.manufacture);
}
private static void print(Apple apple) {
System.out.println("apple = " + apple);
System.out.println("apple.size = " + apple.size);
System.out.println("apple.place = " + apple.place);
System.out.println("apple.manufacture = " + apple.manufacture);
}
}
2.2 深克隆测试
import java.util.Date;
public class DeepCloneTest {
public static void main(String[] args) throws CloneNotSupportedException {
System.out.println("------+--深克隆Test--+------");
DeepApple apple = new DeepApple(10, "China", new Date());
print(apple);
System.out.println("------+------+------");
DeepApple cloneApple = apple.clone();
print(cloneApple);
System.out.println("修改manufacture");
apple.manufacture.setTime(10000000000L);
System.out.println("apple.manufacture = " + apple.manufacture);
System.out.println("cloneApple.manufacture = " + cloneApple.manufacture);
}
private static void print(Apple apple) {
System.out.println("apple = " + apple);
System.out.println("apple.size = " + apple.size);
System.out.println("apple.place = " + apple.place);
System.out.println("apple.manufacture = " + apple.manufacture);
}
}
2.3 序列化、反序列化的方式实现深克隆 测试
import java.io.*;
import java.util.Date;
public class SerializableCloneTest {
public static void main(String[] args) throws IOException, ClassNotFoundException {
System.out.println("------+--深克隆Test--+------");
SerializableApple apple = new SerializableApple(10, "China", new Date());
print(apple);
System.out.println("------+------+------");
ByteArrayOutputStream bos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(bos);
oos.writeObject(apple);
byte[] bytes = bos.toByteArray();
ByteArrayInputStream bis = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bis);
SerializableApple cloneApple = (SerializableApple) ois.readObject();
print(cloneApple);
System.out.println("修改manufacture");
apple.manufacture.setTime(10000000000L);
System.out.println("apple.manufacture = " + apple.manufacture);
System.out.println("cloneApple.manufacture = " + cloneApple.manufacture);
}
private static void print(SerializableApple apple) {
System.out.println("apple = " + apple);
System.out.println("apple.size = " + apple.size);
System.out.println("apple.place = " + apple.place);
System.out.println("apple.manufacture = " + apple.manufacture);
}
}
2.4 原型模式的性能测试
- 注: new生成对象太慢时or同时间需要创建大量相同对象时,就使用原型模式
public class PerformanceTest {
public static void main(String[] args) throws CloneNotSupportedException {
doHomework();
cloneHomework();
}
public static void doHomework() {
long start = System.currentTimeMillis();
Homework homework = new Homework();
for (int i = 0; i < 100; i++) {
Homework homework2 = new Homework();
System.out.println("do homework = " + i);
}
long end = System.currentTimeMillis();
System.out.println("PerformanceTest.doHomework: 耗时" + (end - start) + "毫秒");
}
public static void cloneHomework() throws CloneNotSupportedException {
long start = System.currentTimeMillis();
Homework homework = new Homework();
for (int i = 0; i < 100; i++) {
Homework homework2 = homework.clone();
System.out.println("clone homework = " + i);
}
long end = System.currentTimeMillis();
System.out.println("PerformanceTest.cloneHomework: 耗时" + (end - start) + "毫秒");
}
}