package com.pdf.example;
import com.itextpdf.text.*;
import com.itextpdf.text.pdf.*;
import com.itextpdf.text.pdf.draw.DottedLineSeparator;
import com.itextpdf.text.pdf.draw.LineSeparator;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class PdfReport {// main测试
public static void main(String[] args) throws Exception {
try {
// 1.新建document对象
Document document = new Document(PageSize.A4);// 建立一个Document对象
// 2.建立一个书写器(Writer)与document对象关联
File file = new File("D:\\PDFDemo.pdf");
file.createNewFile();
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream(file));
// writer.setPageEvent(new Watermark("HELLO ITEXTPDF"));// 水印
// writer.setPageEvent(new MyHeaderFooter());// 页眉/页脚
// writer.setEncryption("123".getBytes(), "123".getBytes(), PdfWriter.ALLOW_COPY | PdfWriter.ALLOW_PRINTING, PdfWriter.ENCRYPTION_AES_128);
writer.setEncryption(null, null, PdfWriter.ALLOW_COPY | PdfWriter.ALLOW_PRINTING, PdfWriter.ENCRYPTION_AES_128);
// 3.打开文档
document.open();
document.addTitle("Title@PDF-Java");// 标题
document.addAuthor("Author@umiz");// 作者
document.addSubject("Subject@iText pdf sample");// 主题
document.addKeywords("Keywords@iTextpdf");// 关键字
document.addCreator("Creator@umiz`s");// 创建者
// 4.向文档中添加内容
new PdfReport().generatePDF(document);
// 5.关闭文档
document.close();
// createTablePdf(new File("D:\\PDFDemo1.pdf"));
} catch (Exception e) {
e.printStackTrace();
}
}
// 定义全局的字体静态变量
private static Font titlefont;
private static Font headfont;
private static Font keyfont;
private static Font textfont;
private static Font titleFont1;
private static Font subTitleFont1;
private static Font tableFont1;
// 最大宽度
private static int maxWidth = 520;
// 静态代码块
static {
try {
// 不同字体(这里定义为同一种字体:包含不同字号、不同style)
BaseFont bfChinese = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
titlefont = new Font(bfChinese, 16, Font.BOLD);
headfont = new Font(bfChinese, 14, Font.BOLD);
keyfont = new Font(bfChinese, 10, Font.BOLD);
textfont = new Font(bfChinese, 10, Font.NORMAL);
titleFont1 = new Font(bfChinese, 15, Font.BOLD);
subTitleFont1 = new Font(bfChinese, 10, Font.NORMAL);
tableFont1 = new Font(bfChinese, 13, Font.NORMAL);
} catch (Exception e) {
e.printStackTrace();
}
}
// 生成PDF文件
public void generatePDF(Document document) throws Exception {
// 段落
Paragraph paragraph = new Paragraph("美好的一天从早起开始!", titlefont);
paragraph.setAlignment(1); //设置文字居中 0靠左 1,居中 2,靠右
paragraph.setIndentationLeft(12); //设置左缩进
paragraph.setIndentationRight(12); //设置右缩进
paragraph.setFirstLineIndent(24); //设置首行缩进
paragraph.setLeading(20f); //行间距
paragraph.setSpacingBefore(5f); //设置段落上空白
paragraph.setSpacingAfter(10f); //设置段落下空白
// 直线
Paragraph p1 = new Paragraph();
p1.add(new Chunk(new LineSeparator()));
// 点线
Paragraph p2 = new Paragraph();
p2.add(new Chunk(new DottedLineSeparator()));
// 超链接
Anchor anchor = new Anchor("baidu");
anchor.setReference("www.baidu.com");
// 定位
Anchor gotoP = new Anchor("goto");
gotoP.setReference("#top");
// 添加图片
Image image = Image.getInstance("https://img-blog.csdn.net/20180801174617455?watermark/2/text/aHR0cHM6Ly9ibG9nLmNzZG4ubmV0L3dlaXhpbl8zNzg0ODcxMA==/font/5a6L5L2T/fontsize/400/fill/I0JBQkFCMA==/dissolve/70");
image.setAlignment(Image.ALIGN_CENTER);
image.scalePercent(40); //依照比例缩放
// 表格
PdfPTable table = getPdfPTable1();
document.add(paragraph);
document.add(anchor);
document.add(p2);
document.add(gotoP);
document.add(p1);
document.add(table);
document.add(image);
// ==============色块===================
Paragraph paragraph1 = new Paragraph();
Font f = new Font(Font.FontFamily.TIMES_ROMAN, 11.0f, Font.BOLD, BaseColor.WHITE);
Chunk chunk = new Chunk("Total Cost:", f);
// chunk.setBackground(BaseColor.RED, 0.0f, 0.0f, 470.0f, 0.0f);
// red、green、blue
BaseColor baseColor = new BaseColor(255, 0, 0);
chunk.setBackground(baseColor, 0.0f, 0.0f, 470.0f, 0.0f);
//设置段落前后间距
// paragraph1.setSpacingAfter(25);
// paragraph1.setSpacingBefore(25);
// //设置段段落居中
// paragraph1.setAlignment(Element.ALIGN_CENTER);
// //设置缩进
// paragraph1.setIndentationLeft(250);
// paragraph1.setIndentationRight(50);
paragraph1.add(chunk);
document.add(paragraph1);
// 表格2
PdfPTable table2 = createTable2();
document.add(table2);
// 表格2
PdfPTable table3 = createTable3();
document.add(table3);
// 表格4
Paragraph title = new Paragraph("柔柔弱弱柔柔弱弱让", titleFont1);
title.setAlignment(Element.ALIGN_CENTER);
Paragraph subTitle = new Paragraph("eeeeee\n\n\n", subTitleFont1);
subTitle.setAlignment(Element.ALIGN_CENTER);
document.add(title);
document.add(subTitle);
document.add(createTable(tableFont1));
}
private PdfPTable createTable2() throws DocumentException, IOException {
// 添加表格,4列
PdfPTable table = new PdfPTable(4);
// 设置表格宽度比例为100%
table.setWidthPercentage(100);
// 设置表格的宽度
table.setTotalWidth(300);
// 也可以每列分别设置宽度
float[] width = new float[] { 120, 120, 120, 120 };
table.setTotalWidth(width);
// 锁住宽度
table.setLockedWidth(true);
// 设置表格上面空白宽度
table.setSpacingBefore(10f);
// 设置表格下面空白宽度
table.setSpacingAfter(10f);
// 设置表格默认为无边框
// table.getDefaultCell().setBorder(0);
// 构建每个单元格
// String xx = "Cell 1";
// String xx = "Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1 Cell 1";
// PdfPCell cell1 = new PdfPCell(new Paragraph(xx));
Chunk chunk1 = new Chunk("Name 1");
Paragraph phrase = new Paragraph();
phrase.add(chunk1);
PdfPCell cell1 = new PdfPCell(phrase);
// 边框颜色
// cell1.setBorderColor(BaseCo
itextpdf
最新推荐文章于 2023-03-29 09:34:00 发布
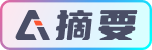