路由使用
App.tsx
import { BrowserRouter as Router } from "react-router-dom";
import { Suspense } from 'react';
const App = () => {
return (
<Router>
<Suspense fallback={<div>222</div>}>
{}
<RoutesComponents></RoutesComponents>
</Suspense>
</Router>
)
}
export default App
routes.tsx
import {Routes, Route} from 'react-router-dom'
import React from 'react'
const Home = React.lazy(() => import('@/pages/home'))
const Note = React.lazy(() => import('@/pages/note'))
const RoutesComponents = () => {
return (
<Routes>
<Route path="home/:id" element={<Home />} />
<Route path="note" element={<Note />} />
{}
<Route path="*" element={<Home />} />
</Routes>
)
}
export default RoutesComponents
Home.tsx
import styles from './index.module.less'
import { useNavigate, useParams, useLocation, Link } from "react-router-dom";
import { Button } from 'antd';
const Home = () => {
const navigate = useNavigate()
const params = useParams()
console.log("🚀 ~ file: index.tsx ~ line 10 ~ Home ~ params", params)
const location = useLocation()
console.log("🚀 ~ file: index.tsx ~ line 14 ~ Home ~ location", location)
const toHome = () => {
navigate('/note', { state: { name: 'zf' }})
}
return (
<div className={styles.homeContainer}>
<div>
<Button onClick={toHome}>to Note</Button>
<Link to={'/note'}>to Note</Link>
</div>
<div>Home</div>
</div>
)
}
export default Home
嵌套路由使用及父路由传参给子路由
路由文件 routes.tsx
import {Routes, Route} from 'react-router-dom'
import React from 'react'
const Home = React.lazy(() => import('@/pages/home'))
const Note = React.lazy(() => import('@/pages/note'))
const Child = React.lazy(() => import('@/pages/home/components/child'))
const RoutesComponents = () => {
return (
<Routes>
<Route path="home" element={<Home />}>
{}
{}
<Route path="child" element={<Child />} />
<Route path="" element={<Child />} />
</Route>
<Route path="note" element={<Note />} />
{}
<Route path="*" element={<Home />} />
</Routes>
)
}
export default RoutesComponents
父组件
import styles from './index.module.less'
import { useNavigate, useParams, useLocation, Link, Route, Routes } from "react-router-dom";
import { Button } from 'antd';
import React from 'react';
import { Outlet } from 'react-router-dom';
const Home = () => {
const [count, setCount] = useState<number>(0)
const toHome = () => {
navigate('/note', { state: { name: 'zf' }})
}
return (
<div className={styles.homeContainer}>
<div>
<Button onClick={toHome}>to Note</Button>
<Link to={'/note'}>to Note</Link>
</div>
<div>Home</div>
{}
<Outlet context={[count, setCount]}></Outlet>
</div>
)
}
export default Home
子组件
const Child = () => {
const [count, setCount] = useOutletContext<OutletType>();
const onClick = () => {
setCount((n: number) => n + 1)
}
return (
<div>
<div>Child</div>
<div>
<button onClick={onClick}>child deal count</button>
<div>
Child count {count}
</div>
</div>
</div>
)
}
export default Child
效果图
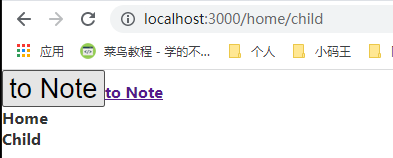
useSearchParams
import { Input } from "antd";
import React from "react";
import { useOutletContext, useSearchParams } from "react-router-dom";
type OutletType = [number, React.Dispatch<React.SetStateAction<number>>]
const Child = () => {
const [count, setCount] = useOutletContext<OutletType>();
const [searchParams, setSearchParams] = useSearchParams();
console.log("🚀 ~ file: child.tsx ~ line 10 ~ Child ~ searchParams", searchParams)
const handleSubmit = (event: any) => {
event.preventDefault();
setSearchParams({
name: event.target.value
})
}
return (
<div>
<div>Child</div>
<div>
<Input onChange={handleSubmit}></Input>
</div>
</div>
)
}
export default Child
示例代码地址