在vue项目中,我们可以通过vue.use()的形式注册全局组件,本篇来讲一下如何通过install的形式开发全局插件;
目录结构:
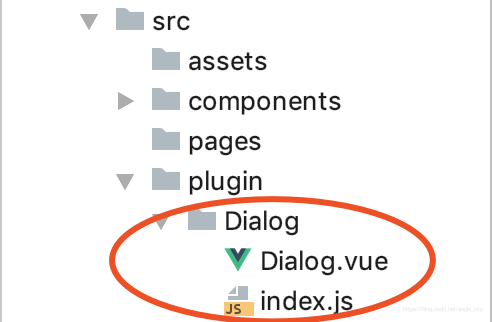
实现效果:
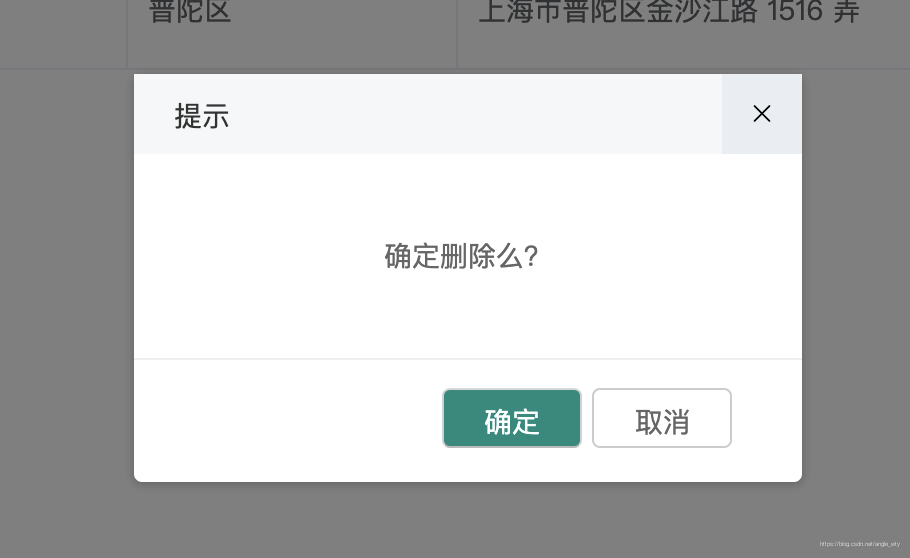
Dialog.vue
<template>
<div class="messageBox" v-if="show">
<div class="inner">
<div class="top">
<div class="tit">{{title}}</div>
<div class="smallIcon" @click="show = false">
<i class="el-icon-close"></i>
</div>
</div>
<div class="center">
{{text}}
</div>
<div class="bottom">
<div class="bottonW">
<button class="makeSure" ref="submit" @click="makeSureBtn">{{btnText1}}</button>
<button class="cancle" @click="show = false">{{btnText2}}</button>
</div>
</div>
</div>
</div>
</template>
<script>
export default {
name: "messagePopUp",
data(){
return{
show:false,
text:'',
title:'',
btnText1:'',
btnText2:'',
}
},
methods:{
makeSureBtn(){
}
}
}
</script>
<style scoped lang="scss">
.messageBox{
position: fixed;
left: 0;
top: 0;
width: 100%;
height: 100%;
background: rgba(0,0,0,0.5);
z-index: 1000;
.inner{
width: 334px;
height: 204px;
position: fixed;
top:50%;
left: 50%;
transform: translate(-50%,-50%);
background:rgba(255,255,255,1);
box-shadow:0px 1px 4px 1px rgba(0,0,0,0.16);
border-radius:4px 4px;
font-size:14px;
font-family:MicrosoftYaHei;
.top{
width:100%;
height:40px;
line-height: 40px;
background:rgba(245,247,248,1);
box-sizing: border-box;
padding-left: 20px;
.tit{
display: inline-block;
font-size:14px;
font-family:MicrosoftYaHei;
color:rgba(51,51,51,1);
}
.smallIcon{
display: inline-block;
float: right;
width:40px;
height:40px;
background:rgba(233,238,242,1);
text-align: center;
font-size: 14px;
cursor: pointer;
}
}
.center{
width: 100%;
height: 102px;
line-height: 102px;
box-sizing: border-box;
padding: 0 20px;
text-align: center;
color:rgba(102,102,102,1);
}
.bottom{
width: 100%;
height: 60px;
line-height: 60px;
box-sizing: border-box;
border-top:1px solid rgba(238,238,238,1);
padding: 0 20px;
.bottonW{
width: 160px;
height: 30px;
float: right;
button{
width:70px;
height:30px;
line-height: 30px;
border-radius:4px;
box-sizing: border-box;
cursor: pointer;
outline: none;
}
.makeSure{
color:rgba(255,255,255,1);
background:rgba(0,138,124,1);
}
.cancle{
background:rgba(255,255,255,1);
color:rgba(102,102,102,1);
border:1px solid rgba(204,204,204,1) !important;
}
}
}
}
}
</style>
index.js
import Vue from 'vue'
import DialogVue from './Dialog'
//创建构造器
const Dialog = Vue.extend(DialogVue)
DialogVue.install = function (options ={}) {
//创建实例
let instance = new Dialog({
data: options
})
instance.makeSureBtn = () => {
options.makeSure()
options.show = false
}
instance.$mount()
document.body.appendChild(instance.$el)
Vue.nextTick(()=>{
instance.show = true
})
}
export default DialogVue;
main.js
import Vue from 'vue'
import App from './App'
import router from './router'
//按需加载 element-ui
import {
Button,
Table,
TableColumn,
Message,
} from 'element-ui';
//注册全局插件
import Dialog from './plugin/Dialog/index'
Vue.prototype.$Dialog = Dialog.install;
Vue.use(Button)
Vue.use(Table)
Vue.use(TableColumn)
Vue.config.productionTip = false
new Vue({
el: '#app',
router,
components: { App },
template: '<App/>'
})
使用方式:
this.$Dialog({
text:'确定删除么?',
title:'提示',
btnText1:'确定',
btnText2:'取消',
makeSure:()=>{
console.log('删除')
}
})