8/6诺瓦星云
修改程序
void point(int *p){*p = p[2];};
int main() {
int c[] ={1,2,3,4,5},*p = c;
point(p+1);
for(;p <c+5;)
{
printf("%d",*p++);
}
return 0;
}
1、分隔字符串 strtok
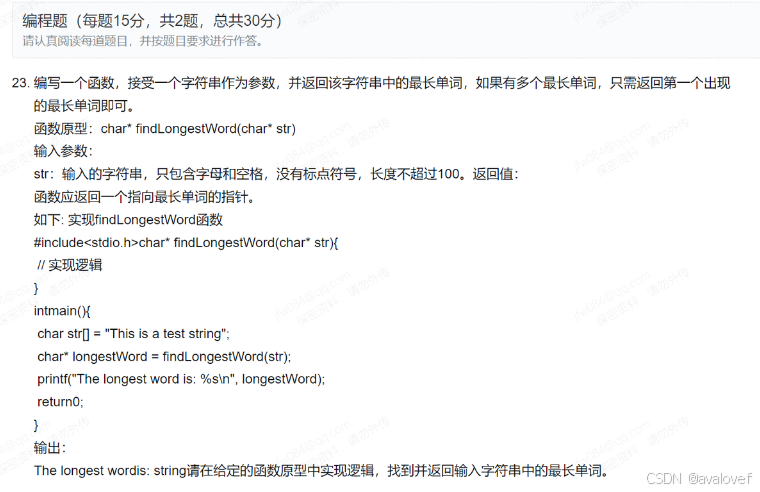
#include <stdio.h>
#include <string.h>
char* findLongestWord(char* str);
int main() {
char* str = "This is a test string";
char* longestWord = findLongestWord(str);
if (longestWord != NULL) {
printf("The longest word is: %s\n", longestWord);
} else {
printf("No words found in the string.\n");
}
return 0;
}
char* findLongestWord(char* str) {
if (str == NULL) return NULL;
char *token;
char *tokens[100];
int i = 0;
char delimiters[] = " ";
char *strCopy = strdup(str);
token = strtok(strCopy, delimiters);
while (token != NULL) {
tokens[i++] = token;
token = strtok(NULL, delimiters);
}
int max = -1;
char* longestWord = NULL;
for (int j = 0; j < i; j++) {
int len_tmp = strlen(tokens[j]);
if(len_tmp>max){
max = len_tmp;
longestWord = tokens[j];
}
}
free(strCopy);
return longestWord;
}
2、后缀法解 最长无重复子串
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int longestlen(char * p)
{
int hash[256];
int len = 0;
memset(hash,0,sizeof(hash));
while (*p && !hash[*p])
{
hash[*p] = 1;
++ len;
++ p;
}
return len;
}
int max_unique_substring4(char * str)
{
int maxlen = -1;
int begin = 0;
char *a[99999];
int n = 0;
while(*str != '\0')
{
a[n++] = str++;
}
for (int i=0; i<n; i++)
{
int temlen = longestlen(a[i]);
if (temlen > maxlen)
{
maxlen = temlen;
begin = i;
}
}
printf("%.*s\n", maxlen, a[begin]);
return maxlen;
}
int main() {
char *test_str = "abcabcbb";
printf("Length of the longest substring without repeating characters: %s\n", max_unique_substring4(test_str));
return 0;
}
3、双向链表
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <string.h>
typedef struct list_node_t {
struct list_node_t *p_before;
struct list_node_t *p_next;
void *p_data;
uint32_t data_size;
} list_node_t;
int insert_data_to_list(void *p_list_head, const void *data, uint32_t data_size) {
if (!p_list_head || !data) {
return -2;
}
list_node_t *new_node = (list_node_t *)malloc(sizeof(list_node_t));
if (!new_node) {
return -1;
}
new_node->p_data = malloc(data_size);
if (!new_node->p_data) {
free(new_node);
return -1;
}
memcpy(new_node->p_data, data, data_size);
new_node->data_size = data_size;
new_node->p_before = NULL;
new_node->p_next = NULL;
list_node_t *current = (list_node_t *)p_list_head;
while (current->p_next) {
current = current->p_next;
}
current->p_next = new_node;
new_node->p_before = current;
return 0;
}
int delete_list_node(void *p_node) {
if (!p_node) {
return -2;
}
list_node_t *node = (list_node_t *)p_node;
if (node->p_before) {
node->p_before->p_next = node->p_next;
}
if (node->p_next) {
node->p_next->p_before = node->p_before;
}
free(node->p_data);
free(node);
return 0;
}
int main() {
list_node_t head = {NULL, NULL, NULL, 0};
int data1 = 100;
int data2 = 20;
insert_data_to_list(&head, &data1, sizeof(data1));
insert_data_to_list(&head, &data2, sizeof(data2));
list_node_t *current = head.p_next;
while (current) {
printf("%d\n", *(int *)current->p_data);
current = current->p_next;
}
current = head.p_next;
while (current) {
list_node_t *next = current->p_next;
delete_list_node(current);
current = next;
}
return 0;
}
4、背包问题
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <string.h>
typedef struct list_node_t {
struct list_node_t *p_before;
struct list_node_t *p_next;
void *p_data;
uint32_t data_size;
} list_node_t;
int insert_data_to_list(void *p_list_head, const void *data, uint32_t data_size) {
if (!p_list_head || !data) {
return -2;
}
list_node_t *new_node = (list_node_t *)malloc(sizeof(list_node_t));
if (!new_node) {
return -1;
}
new_node->p_data = malloc(data_size);
if (!new_node->p_data) {
free(new_node);
return -1;
}
memcpy(new_node->p_data, data, data_size);
new_node->data_size = data_size;
new_node->p_before = NULL;
new_node->p_next = NULL;
list_node_t *current = (list_node_t *)p_list_head;
while (current->p_next) {
current = current->p_next;
}
current->p_next = new_node;
new_node->p_before = current;
return 0;
}
int delete_list_node(void *p_node) {
if (!p_node) {
return -2;
}
list_node_t *node = (list_node_t *)p_node;
if (node->p_before) {
node->p_before->p_next = node->p_next;
}
if (node->p_next) {
node->p_next->p_before = node->p_before;
}
free(node->p_data);
free(node);
return 0;
}
int main() {
list_node_t head = {NULL, NULL, NULL, 0};
int data1 = 100;
int data2 = 20;
insert_data_to_list(&head, &data1, sizeof(data1));
insert_data_to_list(&head, &data2, sizeof(data2));
list_node_t *current = head.p_next;
while (current) {
printf("%d\n", *(int *)current->p_data);
current = current->p_next;
}
current = head.p_next;
while (current) {
list_node_t *next = current->p_next;
delete_list_node(current);
current = next;
}
return 0;
}