1.核心代码
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>vue2.0-1</title>
<style>
/*解决闪烁问题*/
[v-clock]{
display:none;
}
</style>
</head>
<body>
<script src="https://cdn.bootcss.com/vue/2.5.16/vue.min.js"></script>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<!--下拉框-->
<div id="app1" v-clock>
<select v-model="selected">
<option value="" disabled>---请选择---</option>
<option>A</option>
<option>B</option>
<option>C</option>
</select>
<br />
你选择的是{{selected}}
</div>
<!--单选框-->
<div id="app2">
性别:
<input type="radio" value="男" v-model="sex">男
<input type="radio" value="女" v-model="sex">女
<br/>
你选择了:{{sex}}
</div>
<!--axios-->
<div id="app3">
<div>{{info.name}}</div>
<div>{{info.address.city}}</div>
<a v-bind:href="info.url">Click me to jump to Baidu</a>
</div>
<!--methods和computed-->
<div id="app4">
Now:{{getTime()}}
Now2:{{getTime2}}
</div>
<!--v-for-->
<div id="app5">
<ol>
<li v-for="todo in todos">{{todo.text}}</li>
</ol>
</div>
<!--v-bind-->
<div id="app6">
<span v-bind:title="message">
鼠标悬停几秒钟查看此处动态绑定的提示信息!
</span>
</div>
<!--v-if-->
<div id="app7">
<p v-if="seen">现在你看到我了</p>
</div>
<!--插槽slot-->
<div id="app8">
<to_do>
<to_title slot="to_title" v-bind:title="title"></to_title>
<to_items slot="to_items" v-for="item in todoItems" v-bind:item="item"></to_items>
</to_do>
</div>
<!--v-on-->
<div id="app9">
<p>{{message}}</p>
<button v-on:click="reverseMessage">反转消息</button>
</div>
<!--v-model-->
<div id="app10">
<p>{{message}}</p>
<input v-model="message"/>
</div>
<!--v-once-->
<div id="app11">
<h3>{{message}}</h3>
<span v-once>你将不会改变:{{message}}</span>
</div>
<!--v-html-->
<div id="app12">
<p>Using mustaches: {{rawHtml}}</p>
<p>Using v-html directive: <span v-html="rawHtml"></span></p>
</div>
<script>
var vm1 = new Vue({
el: "#app1",
data:{
selected: "A"
}
});
var vm2 = new Vue({
el: "#app2",
data:{
sex: ""
}
});
var vm3 = new Vue({
el: "#app3",
data(){
return{
info:{
name:null,
url:null,
address:{
street:null,
city:null,
country:null
}
}
}
},
mounted(){
axios.get('data.json').then(response=>(this.info=response.data));
}
//使用钩子函数mounted方法,在vue实例挂载的时候将json里的数据,
//加到示例的data函数的info属性中,利用mounted会将el替换成实例下的el的特性,把数据渲染到app中
});
var vm4 = new Vue({
el: "#app4",
data:{
message:'123'
},
methods:{
getTime:function(){
return Date.now();
}
},
computed:{
getTime2:function(){
this.message;
return Date.now();
}
}
});
var vm5 = new Vue({
el: "#app5",
data: {
todos:[
{text:'这是第一个'},
{text:'这是第二个'},
{text:'这是第三个'}
]
}
})
var vm6 = new Vue({
el: "#app6",
data: {
message: '页面加载于 ' + new Date().toLocaleString()
}
})
var vm7 = new Vue({
el: "#app7",
data: {
seen: true
}
})
Vue.component("to_do",{
template: '<div>' + '<slot name="to_title"></slot>' + '<ul>' + '<slot name="to_items"></slot>' + '</ul>'+ '</div>'
});
Vue.component("to_title",{
props: ['title'],
template: '<p>{{title}}</p>'
});
Vue.component("to_items",{
props: ['item'],
template: '<li>{{item}}</li>'
});
var vm8 = new Vue({
el: "#app8",
data: {
title: '课程列表',
todoItems: ['数据结构','操作系统']
}
});
var vm9 = new Vue({
el: "#app9",
data: {
message: 'Hello Vue.js!'
},
methods: {
reverseMessage: function(){
this.message = this.message.split('').reverse().join('')
}
}
})
var vm10 = new Vue({
el: "#app10",
data: {
message:'双向绑定'
}
})
var vm11 = new Vue({
el: "#app11",
data: {
message: '打开控制台修改vm11.message'
}
})
var vm12 = new Vue({
el: "#app12",
data: {
rawHtml: '<span style="color: red">This should be red.</span>'
},
created: function() {
console.log('message is: ' + this.message)
}
})
</script>
</body>
</html>
2.data.json文件
{
"name": "EDC",
"url": "https://www.baidu.com",
"page": 1,
"isNonProfit": true,
"address": {
"street": "白云路",
"city": "湖北武汉",
"country": "中国"
},
"links":[{
"name": "bilibili",
"url": "https://space.bilibili.com"
},
{
"name": "Vue",
"url": "https://cn.vuejs.org/"
},
{
"name": "Vuex",
"url": "https://vuex.vuejs.org/"
}]
}
3.运行结果截图
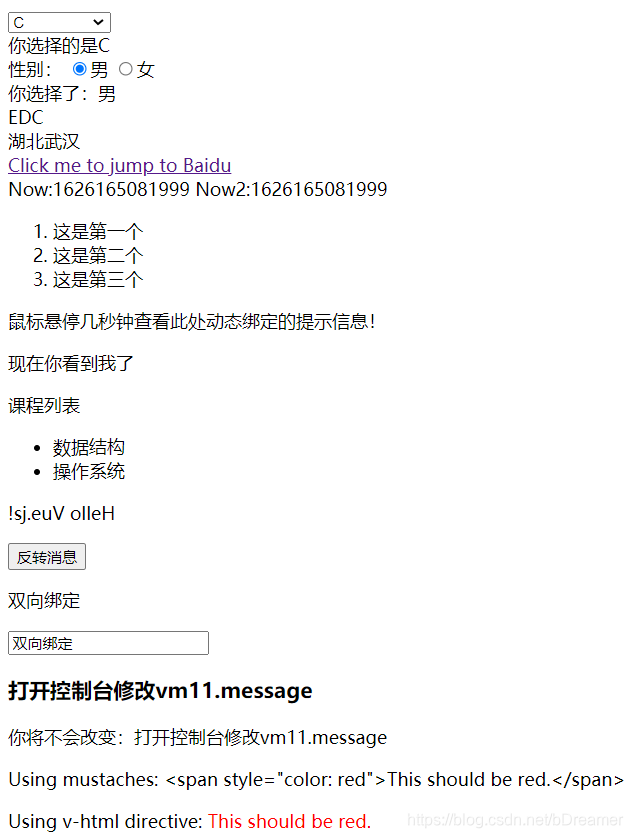