效果如下:
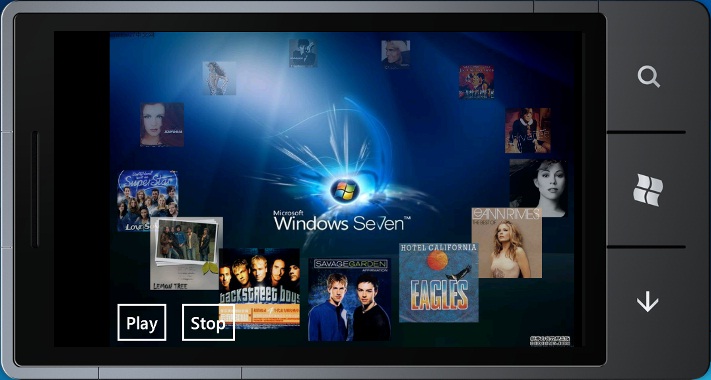
MainPage.xaml


<
phone:PhoneApplicationPage
x:Class = " ShopShow.MainPage "
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
xmlns:phone = " clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone "
xmlns:shell = " clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone "
xmlns:d = " http://schemas.microsoft.com/expression/blend/2008 "
xmlns:mc = " http://schemas.openxmlformats.org/markup-compatibility/2006 "
mc:Ignorable = " d " d:DesignWidth = " 480 " d:DesignHeight = " 768 "
FontFamily = " {StaticResource PhoneFontFamilyNormal} "
FontSize = " {StaticResource PhoneFontSizeNormal} "
Foreground = " {StaticResource PhoneForegroundBrush} "
SupportedOrientations = " PortraitOrLandscape " Orientation = " Portrait "
shell:SystemTray.IsVisible = " True " >
<!-- LayoutRoot is the root grid where all page content is placed -->
< Grid x:Name = " LayoutRoot " Background = " Transparent " >
< Grid.RowDefinitions >
< RowDefinition Height = " Auto " />
< RowDefinition Height = " * " />
</ Grid.RowDefinitions >
<!-- ContentPanel - place additional content here -->
< Grid x:Name = " ContentPanel " Grid.Row = " 1 " Margin = " 12,0,12,0 " >
< Grid.Background >
< ImageBrush ImageSource = " Images/win7-6.jpg "
Stretch = " Fill " />
</ Grid.Background >
< Image x:Name = " shower " Width = " 180 " Height = " 180 " Stretch = " Fill " Visibility = " Collapsed " >
</ Image >
< Canvas x:Name = " moveCanvas " >
</ Canvas >
< StackPanel Orientation = " Horizontal "
Margin = " 0 400 0 0 " >
< Button Height = " 80 " Padding = " 10 0 "
Content = " Play " Margin = " 0 "
x:Name = " btnStart "
Click = " btnStart_Click " ></ Button >
< Button Height = " 80 " Padding = " 10 0 "
Content = " Stop " Margin = " 0 "
x:Name = " btnStop "
Click = " btnStop_Click " ></ Button >
</ StackPanel >
</ Grid >
</ Grid >
</ phone:PhoneApplicationPage >
x:Class = " ShopShow.MainPage "
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
xmlns:phone = " clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone "
xmlns:shell = " clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone "
xmlns:d = " http://schemas.microsoft.com/expression/blend/2008 "
xmlns:mc = " http://schemas.openxmlformats.org/markup-compatibility/2006 "
mc:Ignorable = " d " d:DesignWidth = " 480 " d:DesignHeight = " 768 "
FontFamily = " {StaticResource PhoneFontFamilyNormal} "
FontSize = " {StaticResource PhoneFontSizeNormal} "
Foreground = " {StaticResource PhoneForegroundBrush} "
SupportedOrientations = " PortraitOrLandscape " Orientation = " Portrait "
shell:SystemTray.IsVisible = " True " >
<!-- LayoutRoot is the root grid where all page content is placed -->
< Grid x:Name = " LayoutRoot " Background = " Transparent " >
< Grid.RowDefinitions >
< RowDefinition Height = " Auto " />
< RowDefinition Height = " * " />
</ Grid.RowDefinitions >
<!-- ContentPanel - place additional content here -->
< Grid x:Name = " ContentPanel " Grid.Row = " 1 " Margin = " 12,0,12,0 " >
< Grid.Background >
< ImageBrush ImageSource = " Images/win7-6.jpg "
Stretch = " Fill " />
</ Grid.Background >
< Image x:Name = " shower " Width = " 180 " Height = " 180 " Stretch = " Fill " Visibility = " Collapsed " >
</ Image >
< Canvas x:Name = " moveCanvas " >
</ Canvas >
< StackPanel Orientation = " Horizontal "
Margin = " 0 400 0 0 " >
< Button Height = " 80 " Padding = " 10 0 "
Content = " Play " Margin = " 0 "
x:Name = " btnStart "
Click = " btnStart_Click " ></ Button >
< Button Height = " 80 " Padding = " 10 0 "
Content = " Stop " Margin = " 0 "
x:Name = " btnStop "
Click = " btnStop_Click " ></ Button >
</ StackPanel >
</ Grid >
</ Grid >
</ phone:PhoneApplicationPage >
MainPage.xaml.cs


using
System;
using System.Collections.Generic;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Input;
using System.Windows.Media;
using Microsoft.Phone.Controls;
using System.Windows.Threading;
using System.Windows.Media.Imaging;
namespace ShopShow
{
public partial class MainPage : PhoneApplicationPage
{
private double centerX = 360 ;
private double centerY = 200 ;
private double width = 300 ;
private double height = 180 ;
private double degree = 0 ;
// 项集合类
List < ShopItem > objList = new List < ShopItem > ();
private double itemWidth = 160 ;
private double itemHeight = 80 ;
private double count = 14 ;
private double currentOpacity = 0 ;
private DispatcherTimer timer;
// Constructor
public MainPage()
{
InitializeComponent();
this .Loaded += new RoutedEventHandler(ShopShow_Loaded);
}
private void ShopShow_Loaded( object sender, RoutedEventArgs e)
{
timer = new DispatcherTimer();
for (var i = 1 ; i < count; i ++ )
{
ShopItem item = new ShopItem();
Image image = item.obj;
Uri uri = new Uri( string .Format( " Images/album{0}.png " , i)
, UriKind.RelativeOrAbsolute);
BitmapImage bitmap = new BitmapImage(uri);
image.Source = bitmap;
image.MouseEnter += new MouseEventHandler(image_MouseEnter);
image.MouseLeave += new MouseEventHandler(image_MouseLeave);
image.MouseLeftButtonDown += new MouseButtonEventHandler(image_MouseLeftButtonDown);
objList.Add(item);
moveCanvas.Children.Add(item);
}
timer.Tick += new EventHandler(timer_Tick);
TimeSpan sp = new TimeSpan( 0 , 0 , 0 , 0 , 20 );
timer.Interval = sp;
timer.Start();
}
private void image_MouseLeftButtonDown( object sender, MouseButtonEventArgs e)
{
Image img = sender as Image;
shower.Visibility = Visibility.Visible;
shower.Source = img.Source;
}
private void image_MouseLeave( object sender, MouseEventArgs e)
{
timer.Start();
Image img = (Image)sender;
img.Opacity = currentOpacity;
}
private void timer_Tick( object sender, EventArgs e)
{
StartMove();
}
private void image_MouseEnter( object sender, MouseEventArgs e)
{
timer.Stop();
Image img = (Image)sender;
currentOpacity = img.Opacity;
img.Opacity = 1 ;
}
private void btnStart_Click( object sender, RoutedEventArgs e)
{
timer.Start();
}
private void btnStop_Click( object sender, RoutedEventArgs e)
{
timer.Stop();
}
private void StartMove()
{
for (var i = 0 ; i < objList.Count; i ++ )
{
// 创建一个圆周
var tmp = (degree + ( 360 / objList.Count) * i) % 360 ;
tmp = tmp * Math.PI / 180 ;
var posX = (width) * Math.Sin(tmp); // 更新x
var posY = (height) * Math.Cos(tmp); // 更新y
ShopItem obj = objList[i];
// 根据宽高计算缩放比例
double scale = ( 2 * height - posY) / ( 3 * height + itemHeight / 2 );
Canvas.SetLeft(obj, centerX + posX - (itemWidth / 2 ) * scale);
Canvas.SetTop(obj, centerY - posY - (itemHeight / 2 ) * scale);
Canvas.SetZIndex(obj, int .Parse(Math.Ceiling(objList.Count * scale).ToString()));
// 创建并应用变形属性
ScaleTransform st = new ScaleTransform();
st.ScaleX = scale;
st.ScaleY = scale;
obj.RenderTransform = st;
obj.Opacity = scale;
}
degree = degree - 0.4 ;
}
}
}
using System.Collections.Generic;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Input;
using System.Windows.Media;
using Microsoft.Phone.Controls;
using System.Windows.Threading;
using System.Windows.Media.Imaging;
namespace ShopShow
{
public partial class MainPage : PhoneApplicationPage
{
private double centerX = 360 ;
private double centerY = 200 ;
private double width = 300 ;
private double height = 180 ;
private double degree = 0 ;
// 项集合类
List < ShopItem > objList = new List < ShopItem > ();
private double itemWidth = 160 ;
private double itemHeight = 80 ;
private double count = 14 ;
private double currentOpacity = 0 ;
private DispatcherTimer timer;
// Constructor
public MainPage()
{
InitializeComponent();
this .Loaded += new RoutedEventHandler(ShopShow_Loaded);
}
private void ShopShow_Loaded( object sender, RoutedEventArgs e)
{
timer = new DispatcherTimer();
for (var i = 1 ; i < count; i ++ )
{
ShopItem item = new ShopItem();
Image image = item.obj;
Uri uri = new Uri( string .Format( " Images/album{0}.png " , i)
, UriKind.RelativeOrAbsolute);
BitmapImage bitmap = new BitmapImage(uri);
image.Source = bitmap;
image.MouseEnter += new MouseEventHandler(image_MouseEnter);
image.MouseLeave += new MouseEventHandler(image_MouseLeave);
image.MouseLeftButtonDown += new MouseButtonEventHandler(image_MouseLeftButtonDown);
objList.Add(item);
moveCanvas.Children.Add(item);
}
timer.Tick += new EventHandler(timer_Tick);
TimeSpan sp = new TimeSpan( 0 , 0 , 0 , 0 , 20 );
timer.Interval = sp;
timer.Start();
}
private void image_MouseLeftButtonDown( object sender, MouseButtonEventArgs e)
{
Image img = sender as Image;
shower.Visibility = Visibility.Visible;
shower.Source = img.Source;
}
private void image_MouseLeave( object sender, MouseEventArgs e)
{
timer.Start();
Image img = (Image)sender;
img.Opacity = currentOpacity;
}
private void timer_Tick( object sender, EventArgs e)
{
StartMove();
}
private void image_MouseEnter( object sender, MouseEventArgs e)
{
timer.Stop();
Image img = (Image)sender;
currentOpacity = img.Opacity;
img.Opacity = 1 ;
}
private void btnStart_Click( object sender, RoutedEventArgs e)
{
timer.Start();
}
private void btnStop_Click( object sender, RoutedEventArgs e)
{
timer.Stop();
}
private void StartMove()
{
for (var i = 0 ; i < objList.Count; i ++ )
{
// 创建一个圆周
var tmp = (degree + ( 360 / objList.Count) * i) % 360 ;
tmp = tmp * Math.PI / 180 ;
var posX = (width) * Math.Sin(tmp); // 更新x
var posY = (height) * Math.Cos(tmp); // 更新y
ShopItem obj = objList[i];
// 根据宽高计算缩放比例
double scale = ( 2 * height - posY) / ( 3 * height + itemHeight / 2 );
Canvas.SetLeft(obj, centerX + posX - (itemWidth / 2 ) * scale);
Canvas.SetTop(obj, centerY - posY - (itemHeight / 2 ) * scale);
Canvas.SetZIndex(obj, int .Parse(Math.Ceiling(objList.Count * scale).ToString()));
// 创建并应用变形属性
ScaleTransform st = new ScaleTransform();
st.ScaleX = scale;
st.ScaleY = scale;
obj.RenderTransform = st;
obj.Opacity = scale;
}
degree = degree - 0.4 ;
}
}
}
ShopItem.xaml


<
phone:PhoneApplicationPage
x:Class = " ShopShow.ShopItem "
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
xmlns:phone = " clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone "
xmlns:shell = " clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone "
xmlns:d = " http://schemas.microsoft.com/expression/blend/2008 "
xmlns:mc = " http://schemas.openxmlformats.org/markup-compatibility/2006 "
FontFamily = " {StaticResource PhoneFontFamilyNormal} "
FontSize = " {StaticResource PhoneFontSizeNormal} "
Foreground = " {StaticResource PhoneForegroundBrush} "
SupportedOrientations = " Portrait " Orientation = " Portrait "
mc:Ignorable = " d " d:DesignHeight = " 768 " d:DesignWidth = " 480 "
shell:SystemTray.IsVisible = " True " >
<!-- LayoutRoot is the root grid where all page content is placed -->
< Grid x:Name = " LayoutRoot " Background = " Transparent " >
<!-- ContentPanel - place additional content here -->
< Grid x:Name = " ContentPanel " Margin = " 0 " >
< Image x:Name = " obj "
Width = " 135 "
Height = " 135 "
Stretch = " Fill " />
</ Grid >
</ Grid >
</ phone:PhoneApplicationPage >
x:Class = " ShopShow.ShopItem "
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
xmlns:phone = " clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone "
xmlns:shell = " clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone "
xmlns:d = " http://schemas.microsoft.com/expression/blend/2008 "
xmlns:mc = " http://schemas.openxmlformats.org/markup-compatibility/2006 "
FontFamily = " {StaticResource PhoneFontFamilyNormal} "
FontSize = " {StaticResource PhoneFontSizeNormal} "
Foreground = " {StaticResource PhoneForegroundBrush} "
SupportedOrientations = " Portrait " Orientation = " Portrait "
mc:Ignorable = " d " d:DesignHeight = " 768 " d:DesignWidth = " 480 "
shell:SystemTray.IsVisible = " True " >
<!-- LayoutRoot is the root grid where all page content is placed -->
< Grid x:Name = " LayoutRoot " Background = " Transparent " >
<!-- ContentPanel - place additional content here -->
< Grid x:Name = " ContentPanel " Margin = " 0 " >
< Image x:Name = " obj "
Width = " 135 "
Height = " 135 "
Stretch = " Fill " />
</ Grid >
</ Grid >
</ phone:PhoneApplicationPage >