今天为大家分享一个java语言编写的图书管理程序-003,目前系统功能已经很全面,后续会进一步完善。整个系统界面漂亮,有完整得源码,希望大家可以喜欢。喜欢的帮忙点赞和关注。一起编程、一起进步
开发环境
开发语言为Java,开发环境Eclipse或者IDEA都可以,数据为MySQL。运行主程序,或者执行打开JAR文件即可以运行本程序。
系统框架
利用JDK自带的SWING框架开发。纯窗体模式,直接运行Main文件即可以。同时带有详细得设计文档。
主要功能
Java编写的画图板,功能非常齐全,代码可以执行运行运行。
本次课程设计是利用Java 语言来进行开发画图面板,主要是利用Java语言中自带的Graphics2D强大的画图功能来进行画图的。
Graphics2D继承自Graphics,它扩展了Graphics的绘图功能,拥有更强大的二维图形处理能力,提供对几何形状、坐标转换、颜色管理以及文字布局等更精确的控制。
Graphics2D定义了几种方法,用于添加或改变图形的状态属性。可以通过设定和修改状态属性,指定画笔宽度和画笔的连接方式,设定平移、旋转、缩放或修剪变换图形,以及设定填充图形的颜色和图案等。本次画图面板的实现逻辑如下:
步骤1 定一个基类Shape,继承MouseMotionListener,里面记录了
/** 画笔大小 */
protected float stroke;
/** 画笔颜色 */
protected Color color;
/** 图形类型 */
protected String type;//要画的图形类型
protected int startX = 0;//起始点X坐标
protected int startY = 0;//起始点X坐标
protected int endX = 0;//终止点X坐标
protected int endY = 0;//终止点Y坐标
protected int currentX = 0;//当前X坐标
protected int currentY = 0;//当前Y坐标
protected int currentD = 0;//移动距离
public List<Point> pointList=new ArrayList<Point>();//中途所有经过点的坐标
步骤2 所有类型的图形(文字,直线),都继承Shape
步骤3 当点击不同图标的时候,调用不同的类型的Draw方法,
(1)画直线的时候调用
graphics2d.drawLine(startX, startY, endX, endY);
(2) 画矩形时候调用
xS[0] = currentX;
yS[0] = currentY;
xS[1] = currentX + currentD;
yS[1] = currentY;
xS[2] = currentX+ currentD / 2;
yS[2] = (int)(currentY - currentD / 2 * 1.732);
graphics2d.setColor(color);
graphics2d.setStroke(new BasicStroke(stroke));
graphics2d.drawRect(currentX, currentY, currentD, currentD);
画圆形的时候调用
graphics2d.drawOval(currentX, currentY, currentD, currentD);
(4) 画笔画的时候
int[] arrayx = new int[getPointList().size()];
int[] arrayy = new int[getPointList().size()];
for (int i = 0; i < getPointList().size(); i++) {
Point point = (Point)getPointList().get(i);
arrayx[i]=point.getX();
arrayy[i]=point.getY();
}
graphics2d.setColor(color);
graphics2d.setStroke(new BasicStroke(stroke));
graphics2d.drawPolyline(arrayx,arrayy,getPointList().size());
画橡皮檫的时候
graphics2d.setColor(Color.WHITE);
graphics2d.setStroke(new BasicStroke(8.5f));
int[] arrayx = new int[getPointList().size()];
int[] arrayy = new int[getPointList().size()];
for (int i = 0; i < getPointList().size(); i++) {
Point point = (Point)getPointList().get(i);
arrayx[i]=point.getX();
arrayy[i]=point.getY();
}
graphics2d.setColor(color);
graphics2d.setStroke(new BasicStroke(stroke));
graphics2d.drawPolyline(arrayx,arrayy,getPointList().size());
步骤4 所有类型的图形(文字,直线),都继承Shape,当鼠标按键,或者拖动的时候,在面板上进行重绘,把存储在List中的Shape,重新绘制一遍。得到我们要画的最终的图形,保存图片即可。
1 画直线和文字
2画曲线
3 画矩形
4 画椭圆
5 橡皮擦
6 撤销和重做功能
7 从画图板导入图片,并且对图片进行保存
8 设置画图的颜色和像素大小
这个项目涵盖了java 窗体编程的各种知识,包括UI界面设计、时间处理、文件操作等。通过这个项目能快速提升java 窗体编程,是非常好的一个项目。代码可以直接运行,没有任何bug。有详细的操作手册
实现效果
1 主界面
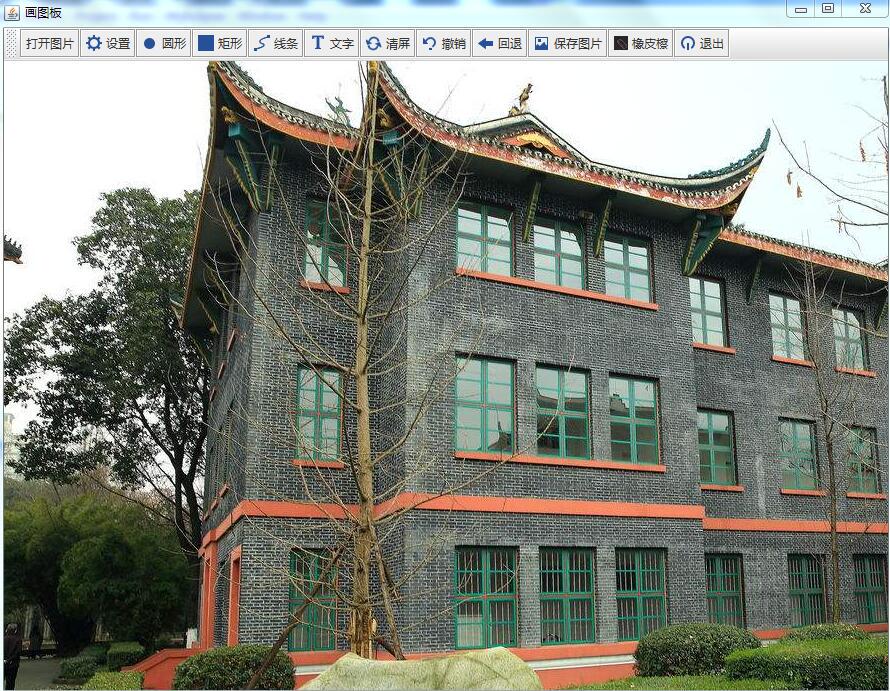
2 画直线和圆圈
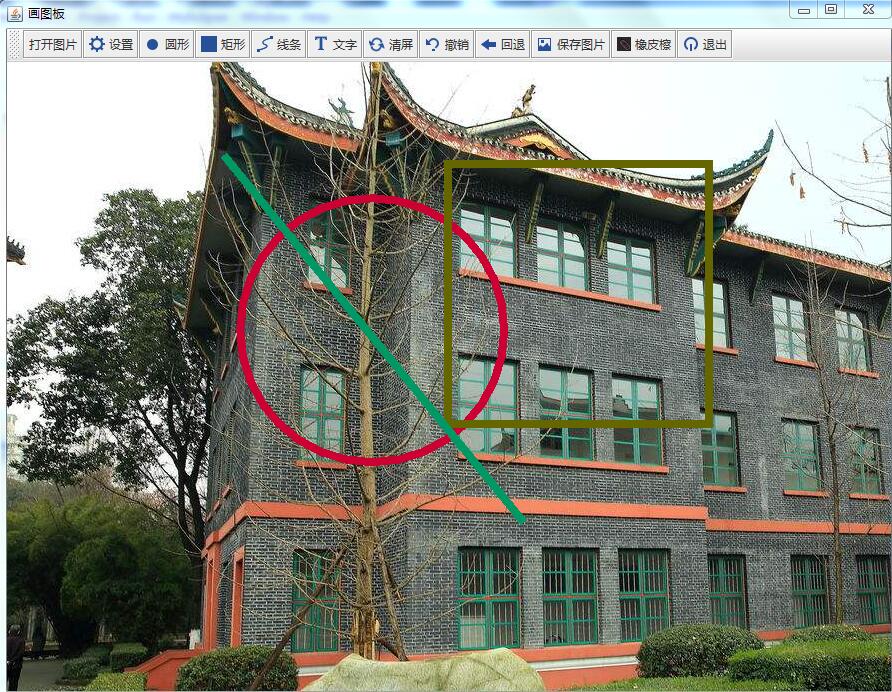
3 设置属性(颜色、字体大小)
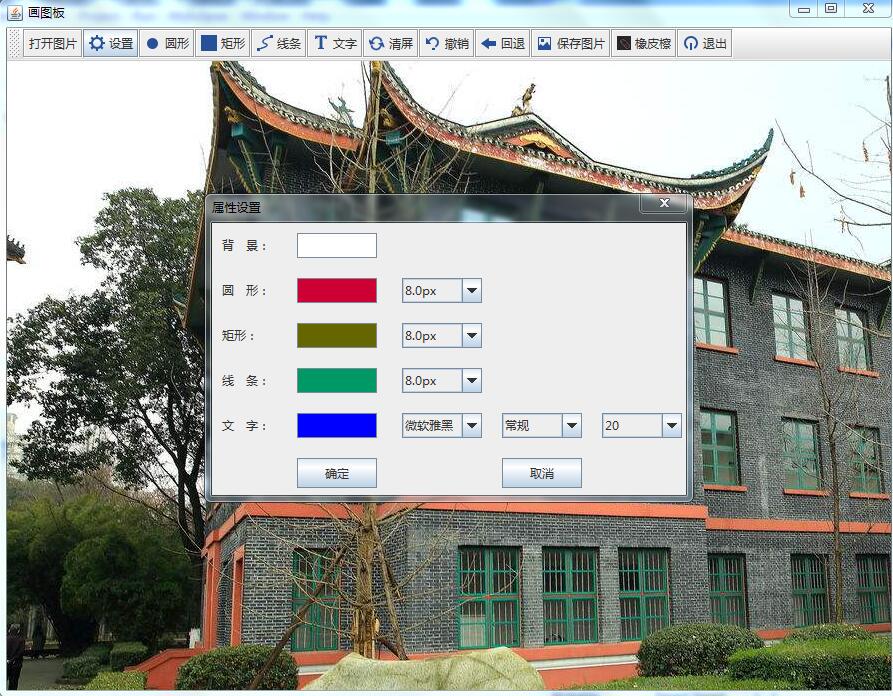
4 保存结果
点击顶部边工具栏上的保存图标,出现保存图片的对话框,选择保存路径,完成图片的保存。
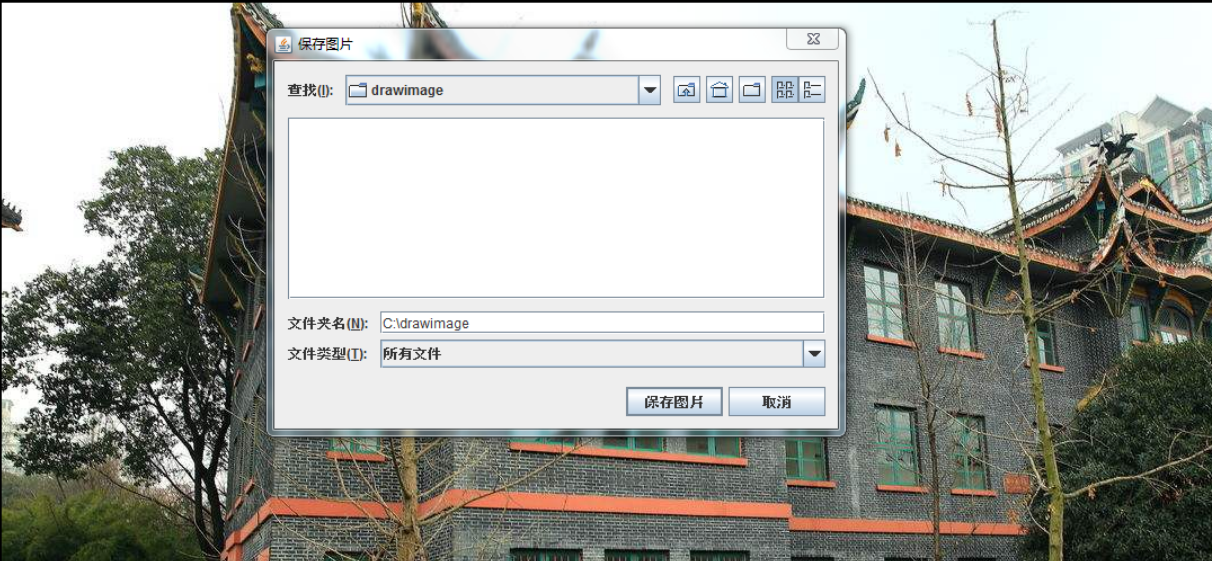
关键代码实现
package com.huatu.ui;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.event.MouseEvent;
import java.awt.event.MouseMotionListener;
import javax.swing.JLabel;
/**
*/
public abstract class Shape extends JLabel implements MouseMotionListener {
private static final long serialVersionUID = 1L;
/** 画笔大小 */
protected float stroke;
/** 画笔颜色 */
protected Color color;
/** 图形类型 */
protected String type;
protected int startX = 0;
protected int startY = 0;
protected int endX = 0;
protected int endY = 0;
protected int currentX = 0;
protected int currentY = 0;
protected int currentD = 0;
protected Shape(Color color1, float stroke1, String type1, int x, int y) {
type = type1;
color = color1;
stroke = stroke1;
startX = endX = currentX = x;
startY = endY = currentY = y;
addMouseMotionListener(this);
}
public void mouseDragged(MouseEvent mouseevent) {
// 变成十字架拖放的时候,需要计算圆形和矩形
if (DrawingBoard.cursor == 1) {
endX = mouseevent.getX();
endY = mouseevent.getY();
currentD = Math.abs(startX - endX);
if (startX > endX) {
currentX = endX;
}
if (startY > endY) {
currentY = endY;
}
} else {// 拖放完毕之后再次点击只需要控制位置即可
currentX = mouseevent.getX();
currentY = mouseevent.getY();
}
}
public void mouseMoved(MouseEvent mouseevent) {
// 鼠标移动
}
/**
* 调用画板来画图
* @param graphics2d 画板的graphics
*/
public abstract void draw(Graphics2D graphics2d);
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
}