[spring学习]使用maven创建spring项目
首先创建好maven项目
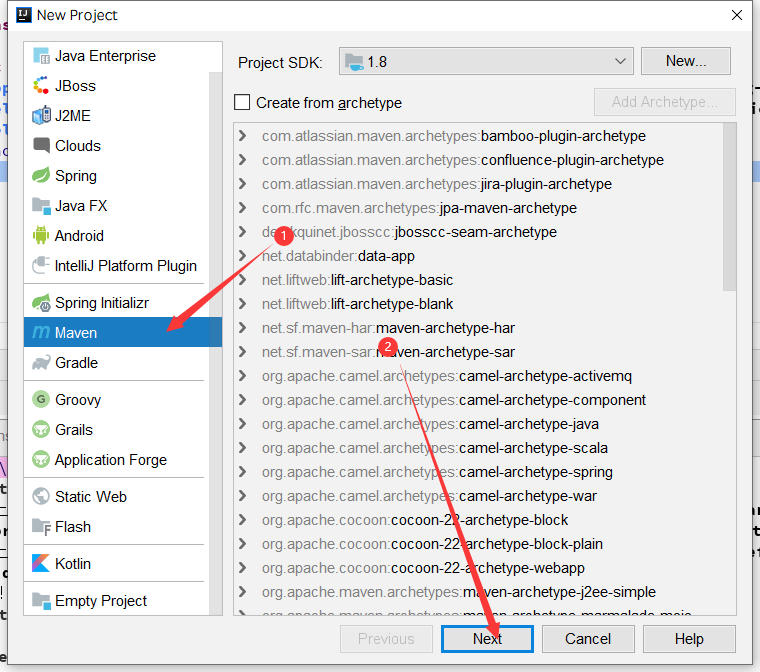
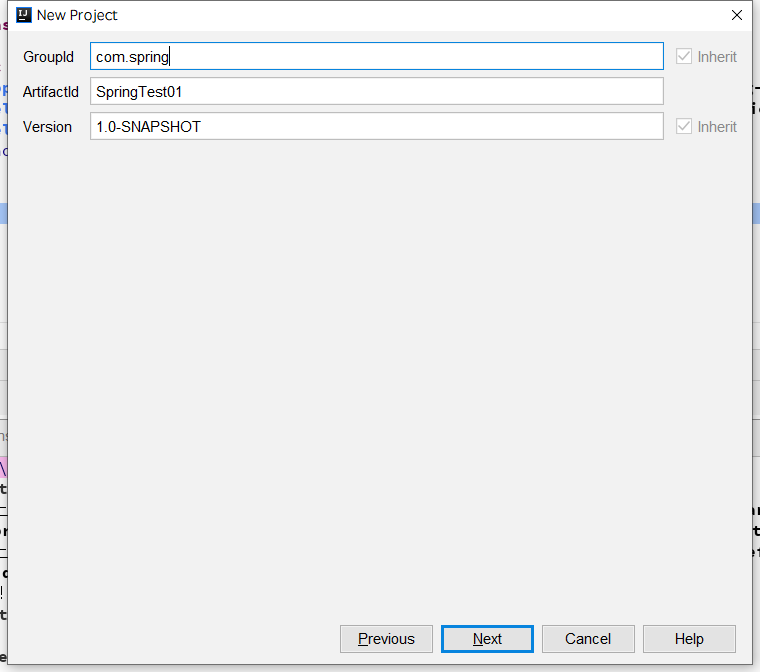
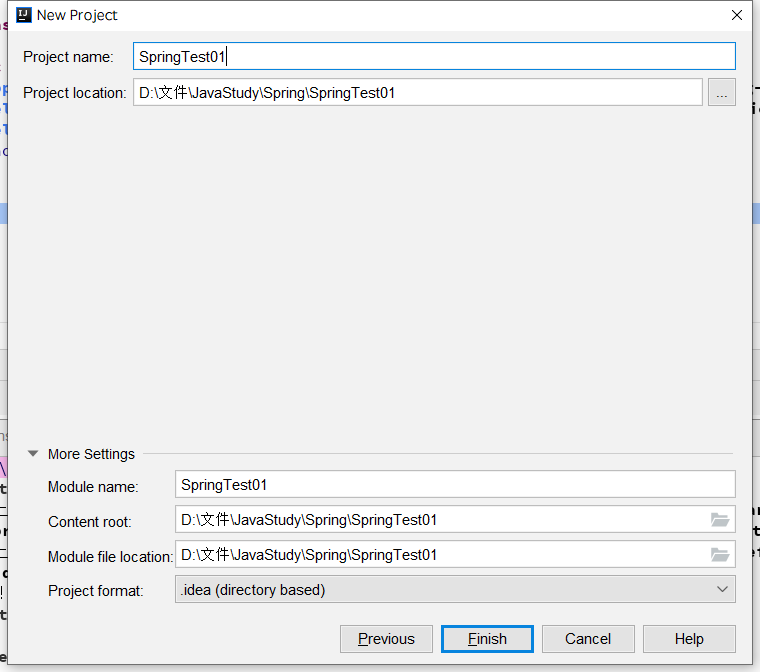
创建好项目之后,项目结构为这样:
配置pom.xml
<dependencies>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-beans -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>4.3.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.3.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>4.3.0.RELEASE</version>
</dependency>
<!-- https://mvnrepository.com/artifact/junit/junit -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13</version>
</dependency>
</dependencies>
此时右键项目,创建spring配置文件
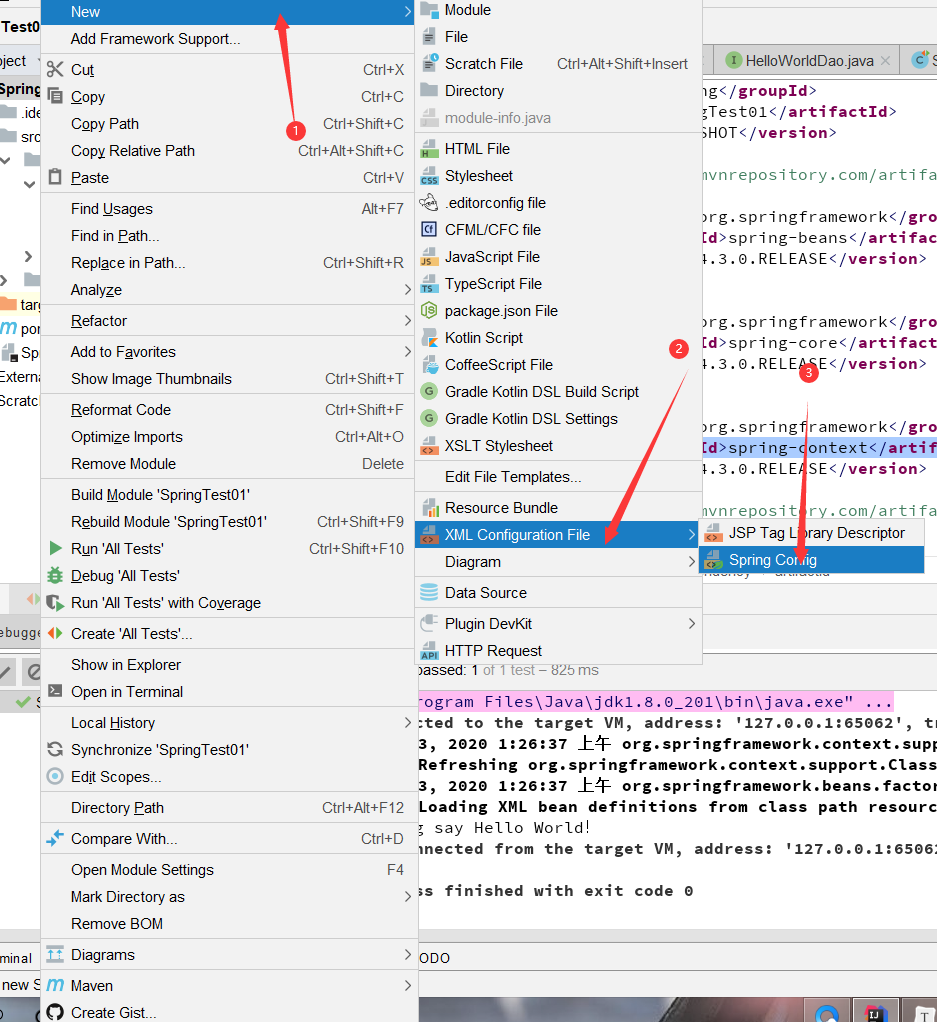
目录结构如下:
HelloWorldDao.java
package com.spring.dao;
public interface HelloWorldDao {
public void sayHello();
}
HelloWorldDaoImpl.java
package com.spring.dao.impl;
import com.spring.dao.HelloWorldDao;
public class HelloWorldDaoImpl implements HelloWorldDao {
public void sayHello() {
System.out.println("Spring say Hello World!");
}
}
HelloWorldService.java
package com.spring.service;
import com.spring.dao.HelloWorldDao;
public class HelloWorldService {
HelloWorldDao helloWorldDao;
public HelloWorldDao getHelloWorldDao() {
return helloWorldDao;
}
public void setHelloWorldDao(HelloWorldDao helloWorldDao) {
this.helloWorldDao = helloWorldDao;
}
}
spring-config.xml
<bean id="HelloWorldDao" class="com.spring.dao.impl.HelloWorldDaoImpl" />
<bean id="HelloWorldService" class="com.spring.service.HelloWorldService" >
<property name="helloWorldDao" ref="HelloWorldDao"/>
</bean>
测试Spring
package com.spring.app;
import com.spring.dao.HelloWorldDao;
import com.spring.service.HelloWorldService;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class SpringTest {
@Test
public void sayHello(){
ApplicationContext ac = new ClassPathXmlApplicationContext("spring-conf.xml");
HelloWorldService service = (HelloWorldService) ac.getBean("HelloWorldService");
HelloWorldDao dao = service.getHelloWorldDao();
dao.sayHello();
}
}
运行结果:
八月 13, 2020 1:26:37 上午 org.springframework.context.support.ClassPathXmlApplicationContext prepareRefresh
信息: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@4ae82894: startup date [Thu Aug 13 01:26:37 CST 2020]; root of context hierarchy
八月 13, 2020 1:26:37 上午 org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions
信息: Loading XML bean definitions from class path resource [spring-conf.xml]
Spring say Hello World!
Disconnected from the target VM, address: '127.0.0.1:65062', transport: 'socket'
Process finished with exit code 0