<template>
<div class="pie-wrap">
<div class="pie" ref="pieEchart"></div>
</div>
</template>
<script>
import * as $echarts from 'echarts'
export default {
name: 'pieModule',
props: {
data: {
type: String,
required: true,
default: '90%'
},
name: {
type: String,
required: true
},
radius: {
type: Array,
required: false,
default: () => [
['50%', '60%']
]
},
fontSize: {
type: Number,
required: false,
default: 14
},
outColorList: {
type: Array,
default: () => ['#000', '#fff']
}
},
watch: {
data: {
deep: true,
handler() {
this.init();
}
}
},
data() {
return {
// 图表类型
myEchart: null,
// 图标配置项
options: {
tooltip: {show: false},
title: {
textAlign: 'center',
x: '48%',
y: '30%',
textStyle: {
fontWeight: 'bold',
color: this.outColorList[0],
fontSize: '14'
}
},
legend: {
show: true,
data: [this.name],
icon: 'circle',
orient: 'horizontal',
itemWidth: 0,
itemHeight: 0,
right: 'center',
bottom: 'bottom',
align: 'right',
textStyle: {
color: "#081735"
}
},
series: [
{
name: this.name,
type: 'pie',
hoverAnimation: false,
center: ['50%', '40%'],
emphasis: {scale: false},
itemStyle: {},
label: {show: false},
labelLine: {show: false}
}
]
}
};
},
// 创建元素
mounted() {
this.init();
},
// 销毁
destroyed() {
// 销毁图表
this.destroyedChart();
},
methods: {
/**
* @description 初始化的方法
* @name init
* @return {*} 无
*/
init() {
// 更新数据
this.update(this.data);
},
/**
* @description 刷新图表
* @return {*} 无
*/
refresh() {
if (this.myEchart) {
this.myEchart.resize();
}
},
/**
* @description 更新
* @param {Object} data 图标数据
* @name update
* @return {*} 无
*/
update(data) {
// 如果不存在echarts
if (!this.myEchart) {
// 图表对象
this.myEchart = $echarts.init(this.$refs.pieEchart);
// 绑定resize 事件
this.bindResizeEvent();
}
// 更新数据
this.updateData(data);
// 清空图表
this.myEchart.clear();
// 生成图表
this.myEchart.setOption(this.options);
},
/**
* @description 更新数据
* @name updateData
* @param {object} data 参数
* @return {*} 无
*/
updateData(data) {
// 对数据进行赋值
const colorName = ['outColorList'];
let tempData = [];
tempData.push(data.substring(0, data.length-1));
tempData.push({value: 100 - this.data.substring(0, data.length-1)});
this.options.series.forEach((item, index) => {
item.data = tempData;
item.itemStyle.color = (params) => this[colorName[index]][params.dataIndex];
item.radius = this.radius[index];
});
this.options.title.text = `${this.data}`;
this.options.title.textStyle.fontSize = this.fontSize;
},
/**
* @description 事件处理函数
* @name resizeHandler
* @return {*} 无
*/
resizeHandler() {
if (this.myEchart) {
this.myEchart.resize();
}
},
/**
* @description 绑定resize 事件
* @name init
* @return {*} 无
*/
bindResizeEvent() {
// 绑定resize事件
window.addEventListener('resize', this.resizeHandler);
},
// 取消事件绑定
unbindResizeEvent() {
// 取消绑定resize事件
window.removeEventListener('resize', this.resizeHandler);
},
/**
* @description 销毁图表
* @name destroyedChart
* @return {*} 无
*/
destroyedChart() {
// 如果存在echarts
if (this.myEchart) {
// 销毁实例,销毁后实例无法再被使用。
this.myEchart.dispose();
this.myEchart = null;
// 取消事件绑定
this.unbindResizeEvent();
}
}
}
};
</script>
<style lang="scss">
.pie-wrap {
position: relative;
width: 100%;
height:100%;
.pie {
margin: 0 auto;
width: 100px;
height: 100px;
}
}
</style>
<pie :name="'学习占比'" :data="'69%'" :out-color-list="['#0370ff', '#fff']"></pie>
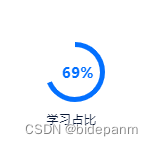