实现效果
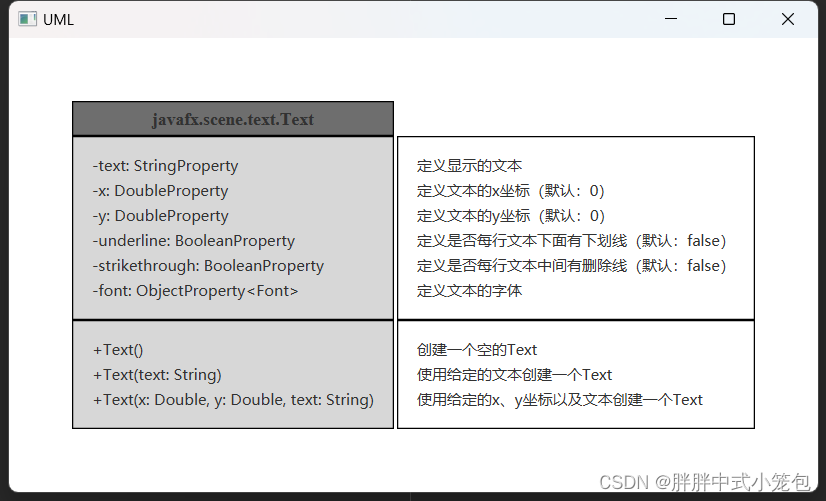
代码
package com.boulete.fxdemo;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.scene.text.Font;
import javafx.scene.text.FontPosture;
import javafx.scene.text.FontWeight;
import javafx.stage.Stage;
import java.util.Arrays;
import java.util.Iterator;
import java.util.LinkedList;
public class UMLApplication extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
UMLClass umlClassText = new UMLClass("javafx.scene.text.Text");
umlClassText.addProperties(
new Property("text", "StringProperty", "定义显示的文本"),
new Property("x", "DoubleProperty", "定义文本的x坐标(默认:0)"),
new Property("y", "DoubleProperty", "定义文本的y坐标(默认:0)"),
new Property("underline", "BooleanProperty", "定义是否每行文本下面有下划线(默认:false)"),
new Property("strikethrough", "BooleanProperty", "定义是否每行文本中间有删除线(默认:false)"),
new Property("font", "ObjectProperty<Font>", "定义文本的字体")
);
umlClassText.addMethods(
new Method("Text", "创建一个空的Text"),
new Method("Text", "使用给定的文本创建一个Text",
new Property("text", "String")),
new Method("Text", "使用给定的x、y坐标以及文本创建一个Text",
new Property("x", "Double"),
new Property("y", "Double"),
new Property("text", "String"))
);
Scene scene = new Scene(getPaneByUMLClass(umlClassText));
primaryStage.setTitle("UML");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch();
}
public GridPane getPaneByUMLClass(UMLClass umlClass){
final double paddingOfWholePane = 50;
final double paddingOfInnerPane = 15;
final double paddingOfTitle = 5;
final double hGap = 2;
final double vGap = 5;
final Font fontTitle = Font.font("Times New Roman", FontWeight.BOLD, FontPosture.REGULAR, 14);
GridPane pane = new GridPane();
StackPane paneClassName = new StackPane();
VBox vBoxProperties = new VBox(vGap);
VBox vBoxPropertiesDesc = new VBox(vGap);
VBox vBoxMethods = new VBox(vGap);
VBox vBoxMethodsDesc = new VBox(vGap);
pane.setStyle("-fx-background-color: white");
paneClassName.setStyle("-fx-border-color: black; -fx-background-color: rgb(110,110,110)");
vBoxProperties.setStyle("-fx-border-color: black; -fx-background-color: rgb(215,215,215)");
vBoxMethods.setStyle("-fx-border-color: black; -fx-background-color: rgb(215,215,215)");
vBoxPropertiesDesc.setStyle("-fx-border-color: black");
vBoxMethodsDesc.setStyle("-fx-border-color: black");
pane.setAlignment(Pos.CENTER);
pane.setPadding(new Insets(paddingOfWholePane,paddingOfWholePane,paddingOfWholePane,paddingOfWholePane));
pane.setHgap(hGap);
Label labelClassName = new Label(umlClass.name);
labelClassName.setFont(fontTitle);
paneClassName.getChildren().add(labelClassName);
paneClassName.setPadding(new Insets(paddingOfTitle, 0, paddingOfTitle, 0));
vBoxProperties.setPadding(new Insets(paddingOfInnerPane,paddingOfInnerPane,paddingOfInnerPane,paddingOfInnerPane));
vBoxPropertiesDesc.setPadding(new Insets(paddingOfInnerPane,paddingOfInnerPane,paddingOfInnerPane,paddingOfInnerPane));
vBoxMethods.setPadding(new Insets(paddingOfInnerPane,paddingOfInnerPane,paddingOfInnerPane,paddingOfInnerPane));
vBoxMethodsDesc.setPadding(new Insets(paddingOfInnerPane,paddingOfInnerPane,paddingOfInnerPane,paddingOfInnerPane));
for (Property property : umlClass.properties){
vBoxProperties.getChildren().add(new Label("-" + property));
vBoxPropertiesDesc.getChildren().add(new Label(property.desc));
}
for (Method method : umlClass.methods){
vBoxMethods.getChildren().add(new Label(method.toString()));
vBoxMethodsDesc.getChildren().add(new Label(method.desc));
}
pane.add(paneClassName, 0, 0);
pane.addRow(1, vBoxProperties, vBoxPropertiesDesc);
pane.addRow(2, vBoxMethods, vBoxMethodsDesc);
return pane;
}
public static class Property{
String name;
String type;
String desc;
public Property(String n, String t, String d){
name = n;
type = t;
desc = d;
}
public Property(String n, String t){
this(n, t, "");
}
@Override
public String toString() {
return name + ": " + type;
}
}
public static class Method{
String name;
String desc;
String methodReturn = "";
LinkedList<Property> properties = new LinkedList<>();
public Method(String n, String d){
name = n;
desc = d;
}
public Method(String n){
this(n, "");
}
public Method(String n, String d, String r){
this(n, d);
methodReturn = r;
}
public Method(String n, String d, Property...ps){
this(n, d);
this.addProperties(ps);
}
public Method(String n, String d, String r, Property...ps){
this(n, d, r);
this.addProperties(ps);
}
public void addProperty(Property p){
properties.add(p);
}
public void addProperties(Property...ps){
properties.addAll(Arrays.asList(ps));
}
@Override
public String toString() {
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append("+").append(name).append('(');
Iterator<Property> iterator = properties.iterator();
while (iterator.hasNext()){
stringBuilder.append(iterator.next());
if (iterator.hasNext())stringBuilder.append(", ");
}
stringBuilder.append(')');
if (!methodReturn.equals("")) stringBuilder.append(": ").append(methodReturn);
return stringBuilder.toString();
}
}
public static class UMLClass{
String name;
LinkedList<Property> properties = new LinkedList<>();
LinkedList<Method> methods = new LinkedList<>();
public UMLClass(String n){
name = n;
}
public void addProperties(Property...ps){
properties.addAll(Arrays.asList(ps));
}
public void addMethods(Method...ms){
methods.addAll(Arrays.asList(ms));
}
}
}