前言
通过文件url批量下载文件,并且每一个文件创建一个文件夹,以此分类,最后统一打成一个压缩包
一、代码示例
public void download(List<DownloadContractFileDTO> list) {
File templateFile = FileUtils.createTemplateFile("zip");
// 创建一个临时目录用于保存文件夹和文件
Path tempDir = null;
try {
tempDir = Files.createTempDirectory("tempDir");
for (DownloadContractFileDTO downloadContractFile : list) {
String name = downloadContractFile.getContractName();
String code = downloadContractFile.getContractCode();
List<String> urlList = downloadContractFile.getContractFileUrl();
// 创建以keyName为名称的文件夹
String fileName = FileUtil.getFileSubstring(name);
Path folderPath = Files.createDirectory(tempDir.resolve(fileName + "_" + code));
for (String url : urlList) {
// 下载PDF文件并保存到文件夹中
FileUtil.downloadFile(url, folderPath.resolve(name).toString());
}
}
// 压缩文件夹
FileUtil.zipDirectory(tempDir.toString(),templateFile);
log.info("文件下载、压缩!完成");
} catch (Exception e) {
AssertUtil.notNull(true, "下载失败");
log.error("下载失败==={}", e.getMessage());
} finally {
if (tempDir != null) {
FileUtil.deleteFilesInDirectory(tempDir.toFile());
}
log.info("文件删除完成!");
}
}
文件工具类
@Slf4j
public class FileUtil {
/**
* 下载文件
*
* @param url url
* @param filePath filePath
* @throws IOException
*/
public static void downloadFile(String url, String filePath) throws IOException {
try (BufferedInputStream in = new BufferedInputStream(new URL(url).openStream());
FileOutputStream fileOutputStream = new FileOutputStream(filePath)) {
byte[] dataBuffer = new byte[1024];
int bytesRead;
while ((bytesRead = in.read(dataBuffer, 0, 1024)) != -1) {
fileOutputStream.write(dataBuffer, 0, bytesRead);
}
}
}
/**
* 压缩文件
*
* @param sourceDir 文件
* @param targetFile 文件
* @throws IOException
*/
public static void zipDirectory(String sourceDir, File targetFile) throws IOException {
try (FileOutputStream fos = new FileOutputStream(targetFile);
ZipOutputStream zos = new ZipOutputStream(fos);
Stream<Path> paths = Files.walk(Paths.get(sourceDir))) {
paths.filter(path -> !Files.isDirectory(path))
.forEach(path -> {
try {
Path relativePath = Paths.get(sourceDir).relativize(path);
ZipEntry zipEntry = new ZipEntry(relativePath.toString());
zos.putNextEntry(zipEntry);
byte[] buffer = new byte[1024];
int length;
try (InputStream is = Files.newInputStream(path)) {
while ((length = is.read(buffer)) > 0) {
zos.write(buffer, 0, length);
}
}
zos.closeEntry();
} catch (IOException e) {
e.printStackTrace();
}
});
}
}
/**
* @param directory 文件
*/
public static void deleteFilesInDirectory(File directory) {
File[] files = directory.listFiles();
if (files != null) {
for (File file : files) {
if (file.isDirectory()) {
deleteFilesInDirectory(file);
} else {
boolean delete = file.delete();
log.info("====delete_file=={},==file=={}", delete, JsonUtils.writeAsJson(file));
}
}
}
boolean delete = directory.delete();
log.info("===delete_file=={},==file=={}", delete, JsonUtils.writeAsJson(directory));
}
/**
* 获取文件夹名称
*
* @return
*/
public static String getFileSubstring(String name) {
if (StringUtils.isBlank(name)) {
return String.valueOf(System.currentTimeMillis());
}
int lastDotIndex = name.lastIndexOf(".");
if (lastDotIndex != -1) {
return name.substring(0, lastDotIndex);
} else {
return name;
}
}
/**
* 创建临时文件
*/
public static File createTemplateFile(String fileType) {
String s = UUID.randomUUID() + "." + fileType;
String filepath = Thread.currentThread().getContextClassLoader().getResource("").getPath()
+ File.separator + "temp";
File dir = new File(filepath);
if (!dir.exists()) {
dir.mkdirs();
}
return createSubFile(dir, s);
}
/**
* 创建子文件
*
* @param parentDir 父文件夹
* @param filename 子文件名
*/
public static File createSubFile(File parentDir, String filename) {
return new File(parentDir.getAbsolutePath() + File.separator + filename);
}
}
实体Bean
@Data
@AllArgsConstructor
@NoArgsConstructor
public class DownloadContractFileDTO implements Serializable {
/**
* 合同code
*/
private String contractCode;
/**
* 合同名称
*/
private String contractName;
/**
* 合同问 url
*/
private List<String> contractFileUrl;
}```
# 二、最后效果
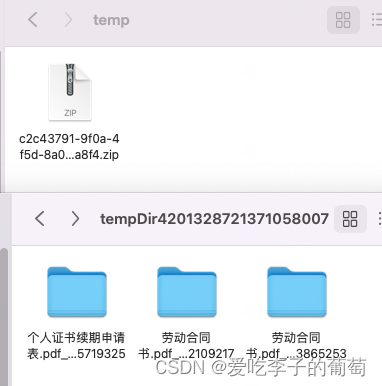
---
# 总结
提示:具体根据自己需求修改代码