package com.baofoo.admin.utils;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* Created by caox on 2018/3/30.
*/
public class ReflectUtils {
/**
* 根据属性名设置 属性值
* @param object 对象类
* @param properityName 属性名
* @param properityType 属性类型 全类名 e.g:java.lang.String
* @param realProperties 要改变的属性值
* @return
*/
public static Object setProperityByName(Object object, String properityName, String properityType, String realProperties){
Field[] fieldsAll = getAllFields(object);
for(Field fAll : fieldsAll){
try {
// 获取属性的类型
String type = fAll.getGenericType().toString();
if (properityType.equals(type) && properityName.equals(fAll.getName())){
fAll.setAccessible(true);
fAll.set(object, realProperties);
}
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
return object;
}
/**
* 递归取类的属性名
* @param object
* @return
*/
public static Field[] getAllFields(Object object){
Class clazz = object.getClass();
List<Field> fieldList = new ArrayList<Field>();
while (clazz != null){
fieldList.addAll(new ArrayList<Field>(Arrays.asList(clazz.getDeclaredFields())));
clazz = clazz.getSuperclass();
}
Field[] fields = new Field[fieldList.size()];
fieldList.toArray(fields);
return fields;
}
/**
* 获取类对象对应属性名 的 对应值
* @param object
* @return
*/
public static String getPropertiesNameAndValue(Object object,String propertiesName){
Method[] methods = object.getClass().getMethods();
String result = "";
for(int i = 0; i < methods.length; i++){
Method method = methods[i];
if(method.getName().startsWith("get") &&
method.getName().equals("get" + (new StringBuilder()).append(Character.toUpperCase(propertiesName.charAt(0))).append(propertiesName.substring(1)).toString())){
// System.out.print("methodName:"+method.getName()+"\t");
try {
//得到get 方法的值
// System.out.println("value:"+method.invoke(object));
result = (String) method.invoke(object);
} catch (Exception e) {
e.printStackTrace();
}
}else{
}
}
return result;
}
}
Object : 具体的实例类带入
利用上述自定义反射方法 在排除类内含有List类型的属性(这里除去类内的List类型属性其他属性都为String类型,但凡这些属性后面带有多余“,”进行批量去除):
try
{
BFA00202 bfa00202 = new BFA00202();
bfa00202.setiDType("iDType,");
bfa00202.setApplicationID("applicationID,");
bfa00202.setMessageFrom("messageFrom,");
System.out.println(bfa00202.toString());
Field[] fields = ReflectUtils.getAllFields(bfa00202);
for (Field field :fields) {
if (!("java.util.List".equals(field.getType().getName()))) {
// 如果type是类类型,则前面包含"class ",后面跟类名
if (field.getGenericType().toString().equals(
"class java.lang.String")) {
// 拿到该属性的gettet方法
Method m ;
try{
m = (Method) bfa00202.getClass().getMethod(
"get" + getMethodName(field.getName()));
}catch (Exception e){
m = (Method) bfa00202.getClass().getMethod(
"get" + (field.getName()));
}
// 调用getter方法获取属性值
String val = (String) m.invoke(bfa00202);
if (val != null) {
// 但凡属性值里面有非ArryList型且带后面多余","均去掉
String valEnd = delStringFrom(val);
System.out.println("String type:" + valEnd);
field.setAccessible(true);
field.set(bfa00202, valEnd);
}
}
}
}
System.out.println(bfa00202.toString());
}
catch (Exception e) {
e.printStackTrace();
}
复杂情况二:处理BFA002类中有List型的属性,本例中处理List中内属性字段带",noData"和是noData两种数据情况处理,第一种去掉,第二种置为null:
/**
* 把一个字符串的第一个字母大写、效率是最高的
*/
private static String getMethodName(String fildeName) throws Exception{
byte[] items = fildeName.getBytes();
items[0] = (byte) ((char) items[0] - 'a' + 'A');
return new String(items);
}
/**
* 判断字符串是否含有“,”去掉逗号以后的字符
*/
public static String delStringFrom(String str){
if(str.contains(",")){
int pos = str.indexOf(",");
str = str.substring(0,pos);
}
return str;
}
/**
* 对应处理类内List中第二种情况
* @param str
* @return
*/
public static String delNullFrom(String str){
if("noData".equals(str)){
str = null;
}
return str;
}
/**
* 对应处理类内List中第一种情况
* @param str
* @return
*/
public static String delNoDateFrom(String str){
if(null != str && str.contains(",noData")){
int pos = str.indexOf(",noData");
str = str.substring(0,pos);
}
return str;
}
/**
* 处理BFA002类中有List型的属性
* 本例中处理List中内属性字段带",noData"和是noData两种数据情况处理,第一种去掉,第二种置为null
* @param objects
* @return
* @throws Exception
*/
public static List<Object> dealStringFromArrayList(List<Object> objects) throws Exception{
for(Object object : objects){
Field[] fields = ReflectUtils.getAllFields(object);
for (Field field :fields) {
Method m ;
try{
m = (Method) object.getClass().getMethod(
"get" + getMethodName(field.getName()));
}catch (Exception e){
m = (Method) object.getClass().getMethod(
"get" + (field.getName()));
}
// 调用getter方法获取属性值
String val = (String) m.invoke(object);
if (val != null) {
String valEnd = delNullFrom(val);
System.out.println("valEnd : " + valEnd);
String valEnd_End = delNoDateFrom(valEnd);
field.setAccessible(true);
field.set(object, valEnd_End);
}
}
}
return objects;
}
public static void main(String[] args) {
try
{
BFA00202 bfa00202 = new BFA00202();
bfa00202.setiDType("iDType,");
bfa00202.setApplicationID("applicationID,");
bfa00202.setMessageFrom("messageFrom,");
List<Association> associationList = new ArrayList<Association>();
Association association = new Association();
association.setBindingDataType("01,noData");
association.setBindingData("02,noData");
Association association1 = new Association();
association1.setBindingDataType("noData");
association1.setBindingData("noData");
associationList.add(association);
associationList.add(association1);
bfa00202.setAssociations(associationList);
System.out.println(bfa00202.toString());
for(Association accoc : bfa00202.getAssociations()){
System.out.println(ToStringBuilder.reflectionToString(accoc));
}
Field[] fields = ReflectUtils.getAllFields(bfa00202);
for (Field field :fields) {
if (!("java.util.List".equals(field.getType().getName()))) {
// 如果type是类类型,则前面包含"class ",后面跟类名
if (field.getGenericType().toString().equals(
"class java.lang.String")) {
// 拿到该属性的gettet方法
Method m ;
try{
m = (Method) bfa00202.getClass().getMethod(
"get" + getMethodName(field.getName()));
}catch (Exception e){
m = (Method) bfa00202.getClass().getMethod(
"get" + (field.getName()));
}
// 调用getter方法获取属性值
String val = (String) m.invoke(bfa00202);
if (val != null) {
// 但凡属性值里面有非ArryList型且带后面多余","均去掉
String valEnd = delStringFrom(val);
System.out.println("String type:" + valEnd);
field.setAccessible(true);
field.set(bfa00202, valEnd);
}
}
}else{
// 处理BFA002类中有List型的属性,本例中处理List中内属性字段带",noData"和是noData两种数据情况处理,第一种去掉,第二种置为null
Method m ;
try{
m = (Method) bfa00202.getClass().getMethod(
"get" + getMethodName(field.getName()));
List<Object> val = (List<Object> ) m.invoke(bfa00202);
List<Object> valEnd = dealStringFromArrayList(val);
field.setAccessible(true);
field.set(bfa00202, valEnd);
}catch (Exception e){
e.printStackTrace();
}
}
}
System.out.println(bfa00202.toString());
for(Association accoc : bfa00202.getAssociations()){
System.out.println(ToStringBuilder.reflectionToString(accoc));
}
}
catch (Exception e) {
e.printStackTrace();
}
}
结果如下:
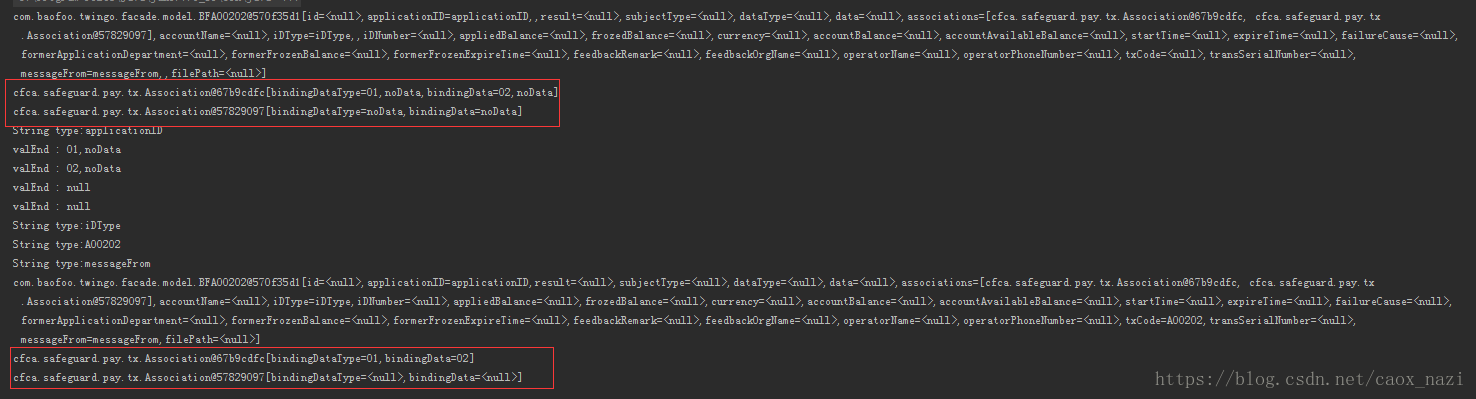