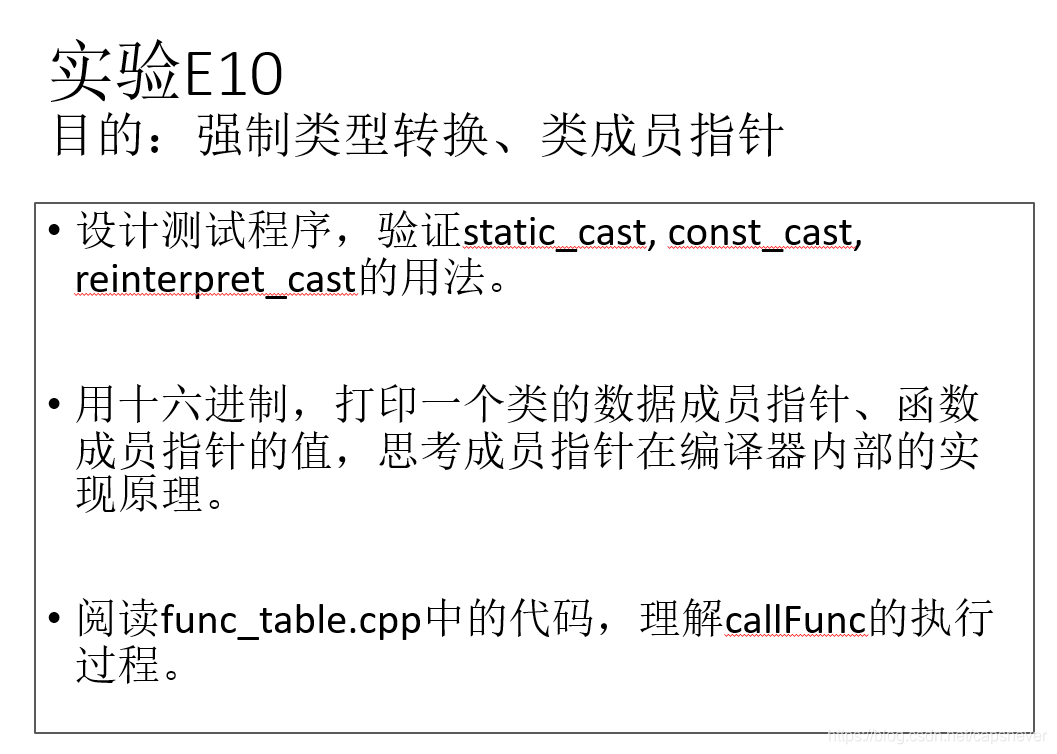
#include <iostream>
using namespace std;
class base {
public:
int _val = 0;
string name = "name";
int _num = 5;
bool _jud = true;
base() {}
base(int toval)
{
_val = toval;
}
void show() const
{
cout << "_val的值为" << _val << endl;
}
void change(int toval)
{
_val = toval;
}
int get_Zero()
{
return 0;
}
void f()
{
cout << "calling f()" << endl;
}
};
template<typename T>
void print(base& _base, T base::* ptr)
{
cout << (_base.*ptr) << endl;
}
typedef void (base::* ptr1)();
typedef void (base::* ptr2)()const;
typedef void (base::* ptr3)(int);
typedef int (base::* ptr4)();
class Derived : public base
{
public:
void test()
{
cout << "calling Derived::test()" << endl;
}
};
class compute
{
public:
static double add(double a, double b)
{
return a + b;
}
};
typedef double (*Compute)(double, double);
int main() {
Derived d1;
base b1 = static_cast<base>(d1);
d1.show();
d1.test();
char ch = 'a';
int t1 = static_cast<int>(ch);
cout << t1 << endl << ch << endl;
if (t1 == 0x61)
{
cout << "t1==0x61" << endl;
}
else
{
cout << "t1!=0x61" << endl;
}
int b2 = 5;
int* ptr = &b2;
int t2 = reinterpret_cast<int>(ptr);
printf("%d\n%d\n", ptr, t2);
base _re_b(1);
int& n1 = reinterpret_cast<int&>(_re_b);
cout << "原来的_val值是" << _re_b._val << endl;
n1 = -100;
cout << "修改n1为-100后,_val值为" << _re_b._val << endl;
int m1 = 1;
cout << "原来的m1值为" << m1 << endl;
base* _re_a = reinterpret_cast<base*>(&m1);
_re_a->_num = -100;
cout << "修改类内的第二个声明定义的_num变量为-100后,m1的值为" << m1 << endl;
_re_a->_val = -100;
cout << "修改类内的第一个声明定义的_val变量为-100后,m1的值为" << m1 << endl;
printf("_re_a->_val的地址为%p\n_re_a->_num的地址为%p\n", &_re_a->_val, &_re_a->_num);
printf("m1的地址为%p\n", &m1);
long long _re_l = 0x123456789abcd;
cout << "_re_l的值为" << hex << _re_l << endl;
base* _re_c = reinterpret_cast<base*>(_re_l);
long long _re_r = reinterpret_cast<long long>(_re_c);
cout << "经过指针转化后的_re_r是" << hex << _re_r << endl;
{
const int a = 12;
const int* ap = &a;
int* tmp = const_cast<int*>(ap);
*tmp = 11;
cout << "test1中a最后的值为" << a << endl;
}
{
int a = 12;
const int* ap = &a;
int* tmp = const_cast<int*>(ap);
*tmp = 11;
cout << "test2中a最后的值为" << a << endl;
}
base _co_b1(5);
const base* bptr = &_co_b1;
base* base2 = const_cast<base*>(bptr);
base2->change(-9);
bptr->show();
{
const base b3;
base b4 = const_cast<base&>(b3);
b4.change(7);
b4.show();
b3.show();
printf("b4的地址为%d\n", &b4);
printf("b3的地址为%d\n", &b3);
}
{
base b3;
base& b4 = b3;
b4.change(7);
b4.show();
b3.show();
printf("b4的地址为%d\n", &b4);
printf("b3的地址为%d\n", &b3);
}
string base::* nptr = &base::name;
int base::* nptr1 = &base::_num;
bool base::* nptr2 = &base::_jud;
printf("%x\n", nptr);
printf("%x\n", nptr1);
printf("%x\n", nptr2);
base temp;
print(temp, nptr);
print(temp, nptr1);
print(temp, nptr2);
ptr1 _ptr1 = &base::f;
ptr2 _ptr2 = &base::show;
ptr3 _ptr3 = &base::change;
ptr4 _ptr4 = &base::get_Zero;
printf("%x\n", _ptr1);
printf("%x\n", _ptr2);
printf("%x\n", _ptr3);
printf("%x\n", _ptr4);
base b5;
(b5.*_ptr1)();
(b5.*_ptr2)();
(b5.*_ptr3)(9);
cout << "调用(b5.*_ptr3)(9)后" << endl;
(b5.*_ptr2)();
cout << (b5.*_ptr4)() << endl;
Compute _Com = &compute::add;
cout << "调用普通函数指针的结果为" << _Com(9.2, 6) << endl;
return 0;
}