一、使用maven中的web模板创建一个web项目
1、项目信息
自己定,项目建好后,等待相关插件、依赖、目录加载
2、初始文件目录
3、目录补全
新建目录(package)
打开配置界面
设置目录用途
二、配置编译路径
1、给项目添加web
添加,如果已经有了,可以删了重新添,也可以跳过这一步,直接修改指定目录
配置路径
2、配置Artifacts
3、在pom.xml中配置项目编码和编译环境
<properties>
<!--字符集-->
<project.build.sourceEncoding>GBK</project.build.sourceEncoding>
<!--编译环境-->
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
4、在pom.xml中配置maven生命周期中编译插件的编码和编译环境
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
<configuration>
<!--编译环境-->
<source>1.8</source>
<target>1.8</target>
<!--编译编码-->
<encoding>GBK</encoding>
</configuration>
</plugin>
5、解决maven生命周期中test控制台乱码问题(pom.xml)
修改pom.xml
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.16</version>
<configuration>
<forkMode>once</forkMode>
<argLine>-Dfile.encoding=UTF-8</argLine>
</configuration>
</plugin>
修改maven启动参数
-Dfile.encoding=UTF-8
6、修改web.xml头
增加后才可以支持EL表达式
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
三、配置tomcat
1、增加tomcat启动项
2、 选择tomcat版本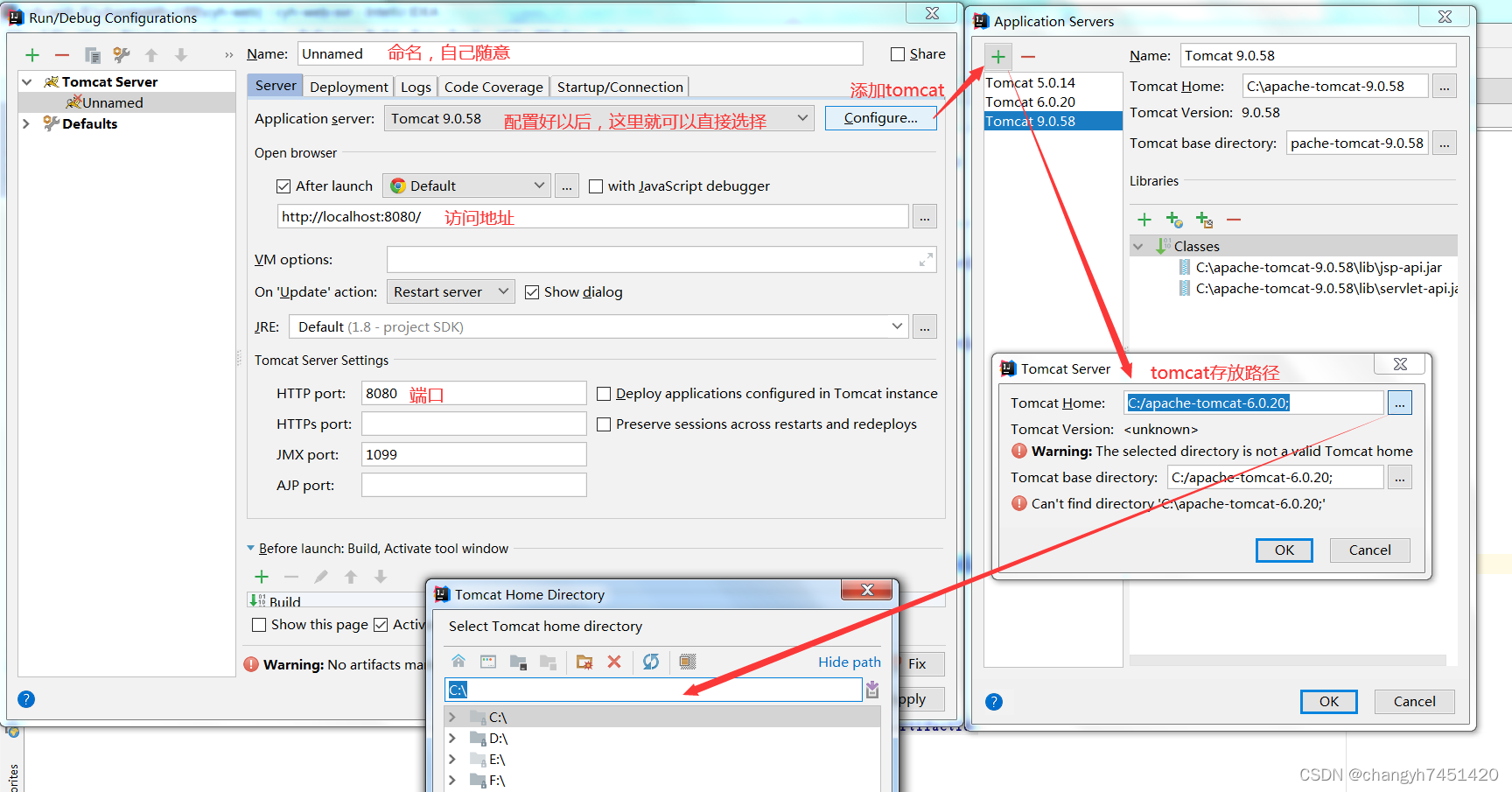
3、配置deployment
4、配置完成,启动tomcat
显示下面页面,代表环境没有问题
5、坑
tomcat6配jdk1.8,服务启动会报错,要配jdk1.6
四、jsp通过form表单向servlet传值
1、添加servlet依赖
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>3.0-alpha-1</version>
</dependency>
2、设置jsp编码
<%--pageEncoding是jsp文件本身的编码
contentType的charset是指服务器发送给客户端时的内容编码--%>
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8" %>
3、添加form标签
<%--action:发起提交请求时指向的servlet
method:请求调用servlet的doGet方法还是doPost方法
get是form表单的默认方法
get数据放在请求的URL中:http://localhost:8080/login?aaa=%E5%A4%A9%E5%A4%A9&bbb=%E5%9C%B0%E5%9C%B0
post是不可见的:http://localhost:8080/login
post可以传输大量的数据,所有上传文件只能用post提交--%>
<form action="login" method="post">
<%--input的name用于servlet从请求中取参数的key--%>
<input type="text" name="aaa" id="bbb">
<input type="text" name="bbb" id="aaa">
<input type="submit" value="确定">
</form>
4、编写servlet接收类
public class LoginServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//取到form表单传入的参数
String aaa = req.getParameter("aaa");
//在控制台打印
System.out.println(aaa);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//设置接收和返回的字符集
req.setCharacterEncoding("utf-8");
resp.setCharacterEncoding("utf-8");
String aaa = req.getParameter("aaa");
System.out.println(aaa);
}
}
5、配置web.xml
<!--配置servlet-->
<servlet>
<!--自定义servlet名-->
<servlet-name>LoginServlet</servlet-name>
<!--servlet类全路径:包+类名-->
<servlet-class>com.cyh.servlet.LoginServlet</servlet-class>
</servlet>
<!--配置servlet映射-->
<servlet-mapping>
<!--要映射的servlet名,与前面自定义的名一样,注意大小写-->
<servlet-name>LoginServlet</servlet-name>
<!--映射的sevlet访问路径(注:/一定要加)-->
<url-pattern>/login</url-pattern>
</servlet-mapping>
五、jsp通过session向servlet传值
1、jsp将数据写入session
<%
//通过session传值:注:这里不能用 小于号+百分号+--注释,有冲突
session.setAttribute("ccc", "ccc");
List<User> users = new ArrayList<User>();
User user = new User();
user.setId("1");
user.setName("a");
user.setPassword("1a");
users.add(user);
//通过session传对象
session.setAttribute("users",users);
%>
2、servlet接收数据
HttpSession session = req.getSession();
String ccc = session.getAttribute("ccc").toString();
System.out.println(ccc);
List<User> users = (List<User>) session.getAttribute("users");
for (User user:users) {
System.out.println(user.toString());
}
六、jsp上传文件
1、导入依赖
文件上传依赖
<!--文件上传-->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency>
读写excel依赖
<!-- 引入poi,解析workbook视图 -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.16</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.14</version>
</dependency>
<!-- 处理excel和上面功能是一样的-->
<dependency>
<groupId>net.sourceforge.jexcelapi</groupId>
<artifactId>jxl</artifactId>
<version>2.6.10</version>
</dependency>
2、写一个接收上传文件的方法
private String fileUpload(HttpServletRequest req, HttpServletResponse resp) throws IOException{
String errorMessage = null;
//判断是否是muitipart/form-data类型
if(!ServletFileUpload.isMultipartContent(req)) {
errorMessage = "表单的enctype属性不是multipart/form-data类型";
return errorMessage;
}
//核心Api
//创建file items工厂
FileItemFactory factory = new DiskFileItemFactory();
//创建文件上传处理器
ServletFileUpload fileUpload = new ServletFileUpload(factory);
//设置单个文件上传大小 2M
fileUpload.setFileSizeMax(2*1024*1024);
//设置总上传文件大小(有时候一次性上传多个文件,需要有一个上限,此处为10M)
fileUpload.setSizeMax(10*1024*1024);
//解析请求
try {
// 解析请求的内容提取文件数据
List<FileItem> parseRequest = fileUpload.parseRequest(req);
//获取数据
for (FileItem fileItem : parseRequest) {
//判断数据类型是不是普通的form表单字段
if(!fileItem.isFormField()) {
//获得上传文件名
String fileName = fileItem.getName();
//获得文件输入流
InputStream fileStream = fileItem.getInputStream();
//取得文件后缀名
String extString = fileName.substring(fileName.lastIndexOf("."));
//判断扩展名是否合法
if (!(extString.equals(".xlsx"))) {
errorMessage = "上传文件非法!";
fileItem.delete();
return errorMessage;
}
User user = new User();
UserDao userDao = new UserDaoImpl();
//文件流转为excel流
XSSFWorkbook workbook=new XSSFWorkbook(fileStream);
//取第一个sheet页数据
Sheet sheet=workbook.getSheetAt(0);
//获取sheet中最后一行行号
int lastRowNum=sheet.getLastRowNum();
//第1行表头数据不读
for (int i=1;i<=lastRowNum;i++){
//获取行数据
Row row=sheet.getRow(i);
//获取指定行,指定列的数据
Cell cell1=row.getCell(1);
Cell cell2=row.getCell(2);
Cell cell3=row.getCell(3);
//数据写入对象
user.setId(cell1.getStringCellValue());
user.setName(cell2.getStringCellValue());
user.setPassword(cell3.getStringCellValue());
//控制台打印读取结果
System.out.println(user.toString());
//调用方法,将数据插入数据库
userDao.addAc01(user);
}
//关闭输入流
fileStream.close();
//重新获取文件输入流,注:如果不重新获取,下面读取文件流就会报错
fileStream = fileItem.getInputStream();
//得到保存文件的名称
String saveFileName = UUID.randomUUID().toString() + "_" + fileName;
//得到文件保存路径
//设置文件上传基本路径
String savePath = this.getServletContext().getRealPath("/WEB-INF/uploadFiles")+File.separator;
File uploadFolder = new File(savePath);
//如果文件路径不存在,则新建一个路径
if (!uploadFolder.exists()) {
uploadFolder.mkdirs();
}
//获得保存文件地址
String realFilePath = savePath + saveFileName;
//创建文件输出流
FileOutputStream out = new FileOutputStream(realFilePath);
//创建缓冲区
byte buffer[] = new byte[1024];
int len = 0;
while ((len = fileStream.read(buffer)) > 0) {
//写文件
out.write(buffer, 0, len);
}
//关闭输出流
out.close();
//关闭输入流
fileStream.close();
}
}
} catch (FileUploadException e) {
e.printStackTrace();
}
errorMessage = "上传成功";
return errorMessage;
}
3、写jsp上传界面
<%--文件传输,enctype就这么写--%>
<form action="login" method="post" enctype="multipart/form-data" >
<input type="file" name="uploadFile">
<button type="submit">上传</button>
<h1>${errmsg}</h1>
</form>
4、坑
文件上传只能用post,get只适用于小量数据传输,因为get的传值是在url里的,详见“四、3、添加form标签”
七、jsp下载文件
1、根据数组对象生成excel文件
//创建Excel文件薄
XSSFWorkbook workbook=new XSSFWorkbook();
//创建工作表sheet
Sheet sheet=workbook.createSheet();
//创建第一行
Row row=sheet.createRow(0);
String[] title={"用户id","用户姓名","用户密码"};
Cell cell=null;
for (int i=0;i<title.length;i++){
cell=row.createCell(i);
cell.setCellValue(title[i]);
}
//追加数据
for (int i=0;i<userlist.size();i++){
Row nextrow=sheet.createRow(i+1);
Cell cell2=nextrow.createCell(0);
cell2.setCellValue(userlist.get(i).getId());
cell2=nextrow.createCell(1);
cell2.setCellValue(userlist.get(i).getName());
cell2=nextrow.createCell(2);
cell2.setCellValue(userlist.get(i).getPassword());
}
2、文件写入服务器
//文件名
String fileName="users.xlsx";
//设置文件在服务器保存基本路径
String savePath = this.getServletContext().getRealPath("/WEB-INF/downloadFiles")+File.separator;
File downloadFolder = new File(savePath);
//如果文件路径不存在,则新建一个路径
if (!downloadFolder.exists()) {
downloadFolder.mkdirs();
}
//获得保存文件地址
String realFilePath = savePath + fileName;
//创建文件输出流
FileOutputStream out = new FileOutputStream(realFilePath);
//写好的excel文件写入输出流
workbook.write(out);
//关闭输出流
out.close();
//关闭excel文件流
workbook.close();
3、生成文件下载链接
//设置消息头,告诉浏览器下载文件名
resp.setHeader("Content-Disposition", "attachment; filename="+fileName);
//取到返回的输出流
ServletOutputStream servletOutputStream=resp.getOutputStream();
//数据写入输出流
workbook.write(servletOutputStream);
//关闭excel文件流
workbook.close();
//释放输出流
servletOutputStream.flush();
//关闭输出流
servletOutputStream.close();
4、数据直接写入本地文件
//创建一个文件
File file=new File("D:/user.xlsx");
file.createNewFile();
FileOutputStream stream= FileUtils.openOutputStream(file);
//数据直接写入本地文件
workbook.write(stream);
stream.close();
5、服务器文件下载
//获取文件路径
String filePath="D:"+File.separator+fileName;
File downloadFile=new File(filePath);
//文件输入流读取资源
FileInputStream fileInputStream=new FileInputStream(downloadFile);
//新建缓存流
BufferedInputStream bufferedInputStream=new BufferedInputStream(fileInputStream);
//取到返回的输出流
ServletOutputStream servletOutputStream=resp.getOutputStream();
int size=0;
byte[] b=new byte[4096];
//文件写入输出流
while ((size=bufferedInputStream.read(b))!=-1) {
servletOutputStream.write(b, 0, size);
}
八、参考资料
关于JSP页面中的pageEncoding和contentType两种属性的区别_dragon4s的专栏-CSDN博客_pageencodingJSP指令标签中这句有什么用途? 关于JSP页面中的pageEncoding和contentType两种属性的区别: pageEncoding是jsp文件本身的编码 contentType的charset是指服务器发送给客户端时的内容编码 JSP要经过两次的“编码”,第一阶https://blog.csdn.net/dragon4s/article/details/6604624form表单中的enctype属性,form表单中的enctype="multipart/form-data"什么意思_jiang7701037的博客-CSDN博客一、form表单的作用1、<form>表单标签使用在一个网页中数据提交标签,这就不用多说了2、form表单在提交时: 1)、先把form表单里的表单元素的name属性和value属性进行收集。 2)、按照enctype属性的设置,选择合适的编码方式,对数据进行编码,放在请求头里 3)、浏览器进行发送。二、enctype属性enc...
https://blog.csdn.net/jiang7701037/article/details/102617481Java 读取Excel ( xls 和 xlsx 格式 )_繁星-CSDN博客_java读取xlsx格式的excel在项目中遇到需要读取 Excel中的数据并导入 Word中的需求,研究了一下在这里做个笔记编写思路:我这里使用的是 poi,其他读取Excel的工具也差不多都是这个思路把表格想象成一个二维数组,然后逐一遍历每一个对象。其实我根据感觉直接看 Excel的格式更好理解,就是使用 Java取出第几行第几列的值而已。这里容易忽略的问题就是一个Excel文件可能会有...
https://blog.csdn.net/qq_36623327/article/details/89236029EL表达式详解_浅然的专栏-CSDN博客_el表达式该篇博客主要关于EL表达式,废话不多说=-=进入正题博客目录1、EL表达式介绍2、EL获取数据3、EL中的内置对象4、EL访问Bean的属性5、EL访问数组中的数据6、EL获取list中数据7、EL访问Map8、EL中的运算符(empty)9、自定义EL函数10、总结一、EL表达式介绍Ex...
https://xulinjie.blog.csdn.net/article/details/79850223