package com.shiwusuo.ReadHdfsToClickHouse
import com.alibaba.fastjson.JSON
import org.apache.http.client.methods.{CloseableHttpResponse, HttpGet, HttpPost, HttpPut}
import org.apache.http.impl.client.{CloseableHttpClient, HttpClients}
import org.apache.http.client.ClientProtocolException
import org.apache.http.client.config.RequestConfig
import org.apache.http.entity.{ContentType, StringEntity}
import org.apache.http.util.EntityUtils
import java.io.IOException
import org.apache.http.HttpEntity
import scala.collection.mutable
/*
* get
* psot
* 请求调用
* */
object InterfaceUtils {
def getResponse(url: String): String = {
val httpClient = HttpClients.createDefault() // 创建 client 实例
val httpGet = new HttpGet(url) // 创建 get 实例
var resPonseJson: String = null
try {
val response = httpClient.execute(httpGet) // 发送请求
resPonseJson = EntityUtils.toString(response.getEntity)
} catch {
case e: Throwable => e.printStackTrace()
}
resPonseJson
// 获取返回结果
}
//Put请求
def putResponse(url: String, jsonStr: String ): String ={
val httpClient = HttpClients.createDefault()
val httpPut = new HttpPut(url)
val requestConfig = RequestConfig.custom.setConnectTimeout(35000).setConnectionRequestTimeout(35000).setSocketTimeout(60000).build
httpPut.setConfig(requestConfig)
httpPut.setHeader("Content-type", "application/json")
httpPut.setHeader("DataEncoding", "UTF-8")
try {
httpPut.setEntity(new StringEntity(jsonStr))
var httpResponse = httpClient.execute(httpPut)
val entity = httpResponse.getEntity
val result = EntityUtils.toString(entity, "UTF-8")
return result
} catch {
case e: ClientProtocolException =>
e.printStackTrace()
return null
case e: IOException =>
e.printStackTrace()
return null
}
}
//Post请求
def postResponse(url: String, params: String = null, header: String = null): String ={
val httpClient = HttpClients.createDefault() // 创建 client 实例
val post = new HttpPost(url) // 创建 post 实例
// 设置 header
if (header != null) {
val json = JSON.parseObject(header)
json.keySet().toArray.map(_.toString).foreach(key => post.setHeader(key, json.getString(key)))
}
if (params != null) {
post.setEntity(new StringEntity(params, "UTF-8"))
}
val response = httpClient.execute(post) // 创建 client 实例
val str: String = EntityUtils.toString(response.getEntity, "UTF-8")
str // 获取返回结果
}
def post(posturi: String, data: String) = {
val httpClient = HttpClients.createDefault() // 创建 client 实例
val httpPost = new HttpPost(posturi);
//httpPost.setConfig(requestConfig)
val entity = new StringEntity(data, ContentType.APPLICATION_JSON)
var res: String = ""
//httpPost.setEntity(new UrlEncodedFormEntity());
httpPost.setEntity(entity)
val response2 = httpClient.execute(httpPost);
try {
System.out.println(response2.getStatusLine());
val entity2 = response2.getEntity();
res = EntityUtils.toString(entity2)
// do something useful with the response body
// and ensure it is fully consumed
EntityUtils.consume(entity2);
} finally {
response2.close();
}
res
}
/*def put_test(): Unit ={
val closeableHttpClient = HttpClients.createDefault()
val httpput = new HttpPut("")
// 5、设置header信息
//header中通用属性
httpput.setHeader("Accept","*")
httpput.setHeader("Accept-Encoding","gzip, deflate")
httpput.setHeader("Cache-Control","no-cache")
httpput.setHeader("Connection", "keep-alive")
httpput.setHeader("Content-Type", "application/json;charset=UTF-8")
//业务参数
val map: mutable.HashMap[String, String] = new mutable.HashMap[String, String]()
// TODO map 的 值
map.put("", "")
//组织请求参数
val stringEntity = new StringEntity(JSON.toJSONString(map), "UTF-8")
httpput.setEntity(stringEntity)
var content = ""
var httpResponse = null
try {
//响应信息
val httpResponse = closeableHttpClient.execute(httpput)
val entity: HttpEntity = httpResponse.getEntity()
content = EntityUtils.toString(entity, "UTF-8");
} catch {
case e: Throwable => e.printStackTrace()
}
}*/
def main(args: Array[String]): Unit = {
//测试get请求
val str: String = getResponse("http://ip:端口/test/resource/getResourceTagsByResourceId?")
println(str)
/*//测试post请求
val postUrl = "http://192.168.12.151:33333/Status"
val params = """{"taskId":"task08261708"}"""
println("This post response: ")
val header =
"""
{
"Accept": "application/json;charset=UTF-8",
"Access-Control-Allow-Headers": "Origin, X-Requested-With, Content-Type, Accept",
"Connection": "keep-alive",
"Content-Type": "application/json; charset=utf-8"
}
""".stripMargin
val str: String = postResponse(postUrl, params, """{"Content-Type": "application/json"}""")
val json = JSON.parseObject(str)
println(json)*/
/* //测试put
val url = "http://192.168.12.51:8080/TaskEngine/api/v1/job/" + "jobId"
val params=
"""
{
"jobInfo": {
“status”:”completed”,
“endTime”:”2020-11-05 12:00:00 ”
}
}
""".stripMargin
putResponse(url , params)*/
}
}
HttpClient Get、Post请求
最新推荐文章于 2024-11-07 11:39:01 发布
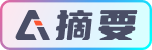